Solving Cache Inconsistency Issues with Ehcache in Spring
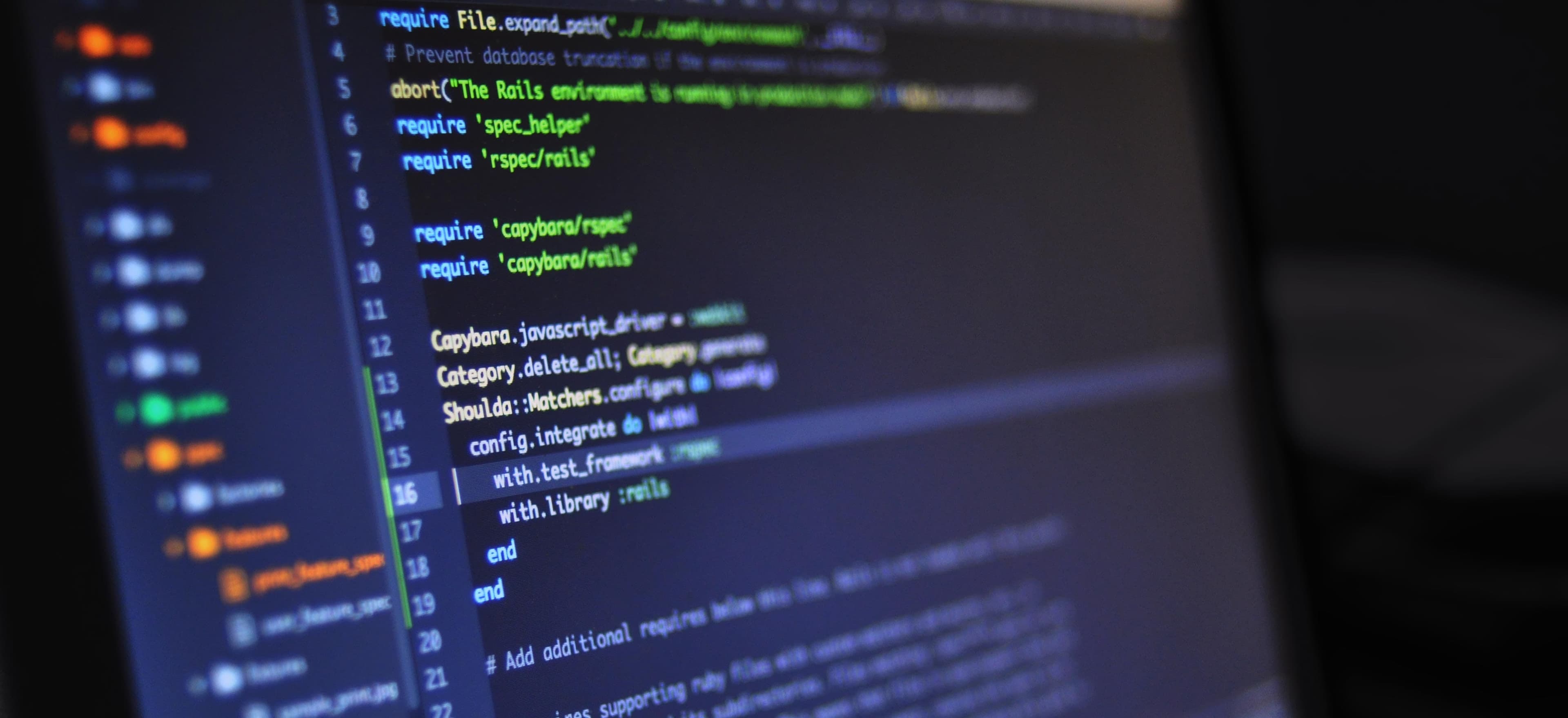
- Published on
Solving Cache Inconsistency Issues with Ehcache in Spring
Caching is a crucial aspect of modern software development, aimed at enhancing performance and reducing latency. However, it can introduce complexity, particularly when dealing with cache inconsistency issues. In this blog post, we will explore how to effectively address cache inconsistency problems using Ehcache in a Spring application.
What is Cache Inconsistency?
Cache inconsistency occurs when the data in the cache gets out of sync with the data in the underlying data store. This situation can lead to stale data being served to users, which can distort business decisions or user experiences. Cache inconsistency can arise due to multiple reasons, such as:
- Concurrent updates to data.
- Data eviction policies that do not align with the update frequency.
- Manual cache invalidation processes that fail.
Why Use Ehcache with Spring?
Ehcache is a widely-used caching framework for Java. It provides an easy-to-use API and integrates seamlessly with the Spring framework, offering features like:
- Ease of Configuration: Ehcache can be configured via XML or Java annotations, making it flexible.
- Support for Annotations: Spring offers a simple way to apply caching to methods with annotations (@Cacheable, @CachePut, @CacheEvict).
- Scalability: Ehcache can handle different caching strategies.
Configuring Ehcache in Spring
To start using Ehcache in your Spring application, you need to set up your project with the appropriate dependencies. If you are using Maven, add the following to your pom.xml
:
<dependency>
<groupId>org.springframework</groupId>
<artifactId>spring-context-support</artifactId>
<version>5.3.12</version>
</dependency>
<dependency>
<groupId>org.ehcache</groupId>
<artifactId>ehcache</artifactId>
<version>3.10.2</version>
</dependency>
Ehcache Configuration
To configure Ehcache, create an ehcache.xml
file as follows:
<ehcache xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:noNamespaceSchemaLocation="http://www.ehcache.org/ehcache.xsd">
<cache name="myCache"
maxEntriesLocalHeap="1000"
eternal="false"
timeToIdleSeconds="3600"
timeToLiveSeconds="3600">
</cache>
</ehcache>
- maxEntriesLocalHeap: Limits the number of entries in cache.
- eternal: Indicates whether the cache entries are permanent.
- timeToIdleSeconds: Configures how long the cache entry remains valid if not accessed.
- timeToLiveSeconds: Configures how long a cache entry is available.
Enabling Caching in Spring
Next, enable caching in your Spring configuration:
import org.springframework.cache.annotation.EnableCaching;
import org.springframework.context.annotation.Bean;
import org.springframework.context.annotation.Configuration;
@Configuration
@EnableCaching
public class AppConfig {
// Other bean configurations
@Bean
public EhCacheManagerFactoryBean ehCacheManager() {
EhCacheManagerFactoryBean factoryBean = new EhCacheManagerFactoryBean();
factoryBean.setConfigLocation(new ClassPathResource("ehcache.xml"));
factoryBean.setShared(true);
return factoryBean;
}
}
This configuration initializes Ehcache and enables caching support in your Spring application.
Using Caching Annotations
Once everything is set up, you can start using caching in your services. Here is a simple example of a service that retrieves user information:
import org.springframework.cache.annotation.Cacheable;
import org.springframework.stereotype.Service;
@Service
public class UserService {
@Cacheable("myCache")
public User getUserById(Long userId) {
// Simulate a slow database call
simulateSlowService();
return fetchUserFromDatabase(userId);
}
private User fetchUserFromDatabase(Long userId) {
// Code to fetch user from database
}
private void simulateSlowService() {
try {
Thread.sleep(3000);
} catch (InterruptedException e) {
throw new IllegalStateException(e);
}
}
}
In this example, the getUserById
method caches the user object. The next time getUserById
is called with the same userId
, it retrieves the user object from the cache instead of querying the database again.
Addressing Cache Inconsistency
To effectively manage cache consistency with Ehcache in Spring, you can utilize the following strategies:
1. Cache Eviction
Using the @CacheEvict
annotation allows you to remove cache entries when the underlying data changes. This ensures that any updates are reflected in the cache:
import org.springframework.cache.annotation.CacheEvict;
@CacheEvict(value = "myCache", key = "#user.id")
public void updateUser(User user) {
// Code to update user in database
}
In this case, every time updateUser
is called, it will evict the corresponding entry from the cache, ensuring that the next retrieval calls getUserById
will fetch fresh data.
2. Cache Put
The @CachePut
annotation is another tool for cache management. It updates the cache directly when a method is called:
import org.springframework.cache.annotation.CachePut;
@CachePut(value = "myCache", key = "#user.id")
public User updateUser(User user) {
// Code to update user in database
return user;
}
3. Versioning Cache Entries
Another advanced technique is to use versioning in your cache keys. This method can prevent stale data from being returned when data changes. Here is a simple example of how to implement versioning:
@Cacheable(value = "myCache", key = "#userId + '-' + #version")
public User getUserById(Long userId, String version) {
return fetchUserFromDatabase(userId);
}
4. Distributing the Cache
In a distributed system, caching inconsistencies can arise due to multiple application instances. For larger setups, consider using distributed cache systems such as Hazelcast or Redis, which offer better consistency across multiple servers.
Key Takeaways
Cache inconsistency is a complex issue, but with a proper understanding of Ehcache and effective strategies, it can be effectively managed. The integration of Ehcache with Spring gives developers powerful tools to ensure data consistency while maintaining performance.
To summarize, you can:
- Configure and enable Ehcache in your Spring application.
- Use caching annotations like
@Cacheable
,@CacheEvict
, and@CachePut
to manage cache behavior. - Implement techniques like versioning and distributed caching for more extensive systems.
By following these best practices, you can ensure your application remains performant and provides accurate data to its users.
For further reading on caching strategies in Spring, you may find these articles helpful:
Now that you have the tools to tackle cache inconsistency, you can enhance your application's performance while ensuring data accuracy. Happy coding!
Checkout our other articles