Tackling Java Framework Hurdles: Lessons from HydePHP
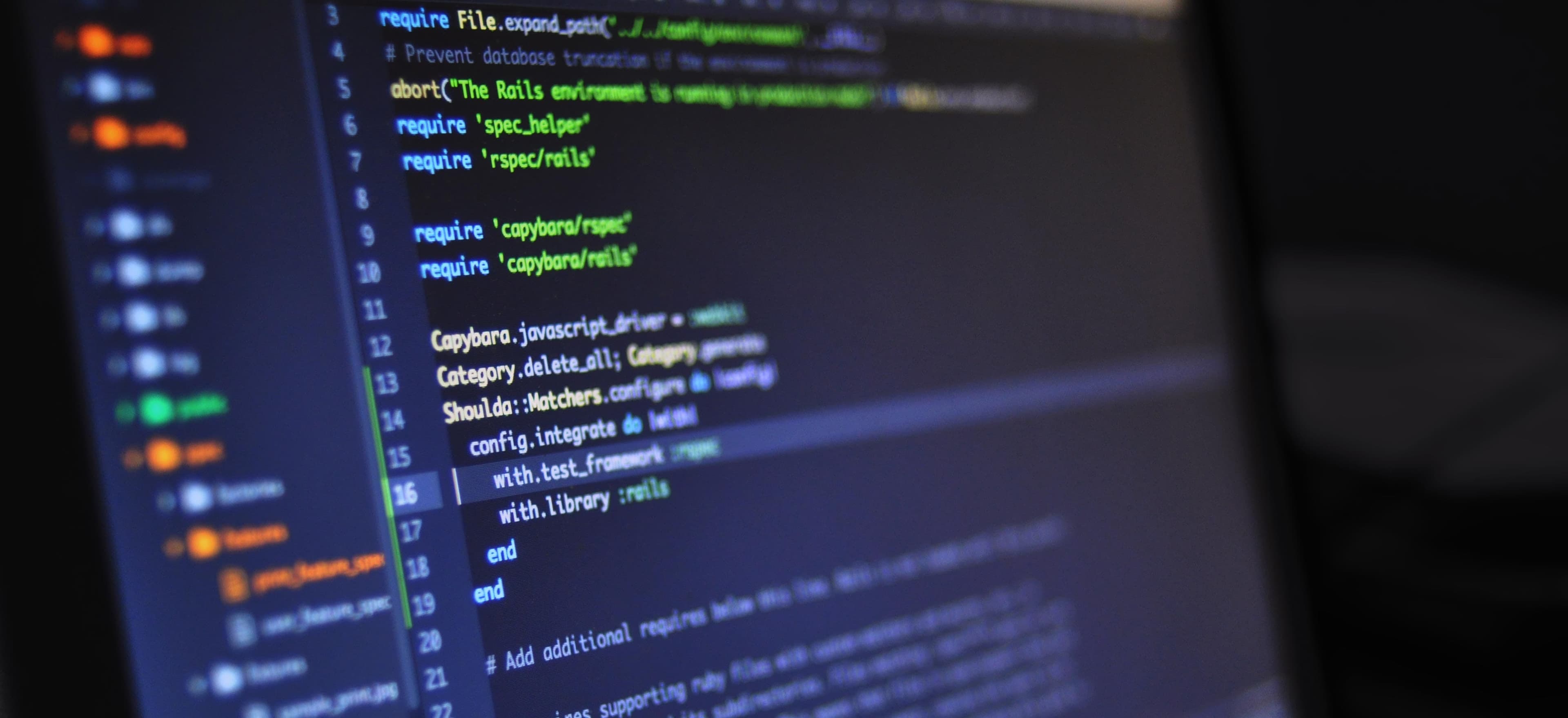
- Published on
Tackling Java Framework Hurdles: Lessons from HydePHP
When navigating the landscape of software development, developers often encounter various challenges associated with frameworks. Java, one of the most popular programming languages, has a myriad of frameworks that simplify development yet introduce complexity in their own way. In this post, we will explore several hurdles faced when using Java frameworks and draw parallels to lessons learned from HydePHP, as outlined in the article Overcoming Common Challenges with HydePHP's First Release.
Understanding Framework Hurdles
Before diving into solutions, it's critical to understand the common hurdles developers face when working with frameworks. Common issues include:
- Complexity and Steep Learning Curves: Many frameworks come with a myriad of features and components that can overwhelm beginners.
- Integration Challenges: Integrating third-party libraries or tools is not always straightforward.
- Performance Issues: Some frameworks add overhead, impacting the application’s performance.
- Inadequate Documentation: Insufficient documentation can leave developers in the dark regarding functionality.
Navigating these challenges demands a well-informed approach and effective strategies.
Learning from HydePHP
HydePHP's inaugural release offered valuable insights that can significantly benefit Java developers when dealing with framework challenges. Chief among these insights is the concept of simplicity and community involvement. The following examples illustrate how HydePHP's strategies can be adapted to Java development.
1. Embrace Simplicity Over Complexity
In HydePHP, simplicity led to a straightforward learning process. Similar to how it's important to grasp the fundamentals of HydePHP, Java developers should focus on mastering basic concepts before jumping into frameworks.
Example: Before adopting frameworks like Spring or Hibernate, ensure a solid understanding of core Java concepts like OOP (Object-Oriented Programming), collections, and interfaces.
// Example: Basic OOP Concepts in Java
class Animal {
String name;
public Animal(String name) {
this.name = name;
}
public void sound() {
System.out.println("Animal sound");
}
}
class Dog extends Animal {
public Dog(String name) {
super(name);
}
@Override
public void sound() {
System.out.println(name + " says Woof!");
}
}
public class Main {
public static void main(String[] args) {
Dog dog = new Dog("Buddy");
dog.sound(); // Outputs: Buddy says Woof!
}
}
Why: Understanding Java's OOP principles is paramount, as most frameworks leverage these concepts. A strong foundation enables better comprehension and utilization of complex frameworks.
2. Utilize Community Resources
HydePHP's community-driven development showcased the importance of collaboration. Similarly, leveraging community resources can be a game-changer for Java developers.
Resources such as GitHub, Stack Overflow, and Java forums can serve as gold mines for solving framework-related issues. For instance, if you're using Spring and run into configuration issues:
- Search on Stack Overflow: Many developers have faced similar issues and shared solutions.
- Refer to GitHub repositories: Often, others will have submitted PRs addressing specific bugs or pain points in frameworks.
3. Read and Contribute to Documentation
In HydePHP’s development, involving the community helped produce comprehensive documentation. As a Java developer, investing time in reading the documentation can save a considerable amount of frustration.
Example: The Spring Framework has extensive documentation. When struggling to configure a project, refer to:
Moreover, consider contributing to the documentation, as writing about learned solutions helps reinforce your knowledge.
4. Performance Assessment Tools
HydePHP initiated practical performance assessments, a practice that is equally applicable to Java development. Tools such as JProfiler, VisualVM, and Spring Boot Actuator provide insights into application performance.
Example: If you're observing slow response times within a Spring application:
- Use Spring Boot Actuator to analyze the health and metrics of your application.
<!-- Example: Adding Spring Boot Actuator in Maven -->
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-actuator</artifactId>
</dependency>
Why: Monitoring metrics and performance details allows for effective optimization by identifying bottlenecks and optimizing the necessary components.
5. Break Down the Problem
Large applications can seem daunting. HydePHP encourages a modular approach to development. Developers should break down their applications into manageable components.
Example: In Java applications, leverage Spring's modular features:
@Configuration
@EnableWebMvc
public class WebConfig implements WebMvcConfigurer {
@Bean
public ViewResolver viewResolver() {
InternalResourceViewResolver resolver = new InternalResourceViewResolver();
resolver.setPrefix("/WEB-INF/views/");
resolver.setSuffix(".jsp");
return resolver;
}
}
Why: Modular design encourages better organization of code, making it easier to manage and understand. This directly addresses complexity in frameworks by limiting the amount of code you need to navigate at once.
6. Set Up a Continuous Feedback Loop
HydePHP emphasized dynamic feedback through community engagement. For Java developers, creating a similar feedback mechanism is essential. Continuous integration and delivery (CI/CD) tools such as Jenkins, Travis CI, or GitHub Actions can streamline feedback.
Example: Configure a Jenkins pipeline to run tests automatically on every commit. Here’s a brief look at a simple Jenkinsfile configuration:
pipeline {
agent any
stages {
stage('Build') {
steps {
echo 'Building ...'
sh './gradlew build'
}
}
stage('Test') {
steps {
echo 'Testing ...'
sh './gradlew test'
}
}
}
}
Why: Continuous feedback allows developers to identify issues early, facilitating a more agile approach to overcoming challenges.
In Conclusion, Here is What Matters
Tackling Java framework hurdles can seem overwhelming, but by emulating the strategies employed in HydePHP's development, Java developers can adapt and succeed. Embracing simplicity, engaging with the community, thoroughly understanding documentation, utilizing performance assessment tools, breaking down complexities, and establishing continuous feedback mechanisms are foundational practices that can significantly enhance your development experience.
As you explore the depths of Java frameworks, remember that every challenge presents an opportunity. By learning from HydePHP and implementing these strategies, you can not only overcome hurdles but flourish as a proficient Java developer. For further insights into how frameworks can address challenges in web development, consider reading Overcoming Common Challenges with HydePHP's First Release.
By equipping yourself with knowledge and adaptability, you'll be well on your way to mastering Java frameworks with confidence. Happy coding!