Overcoming TDD Hurdles: Java Best Practices for Success
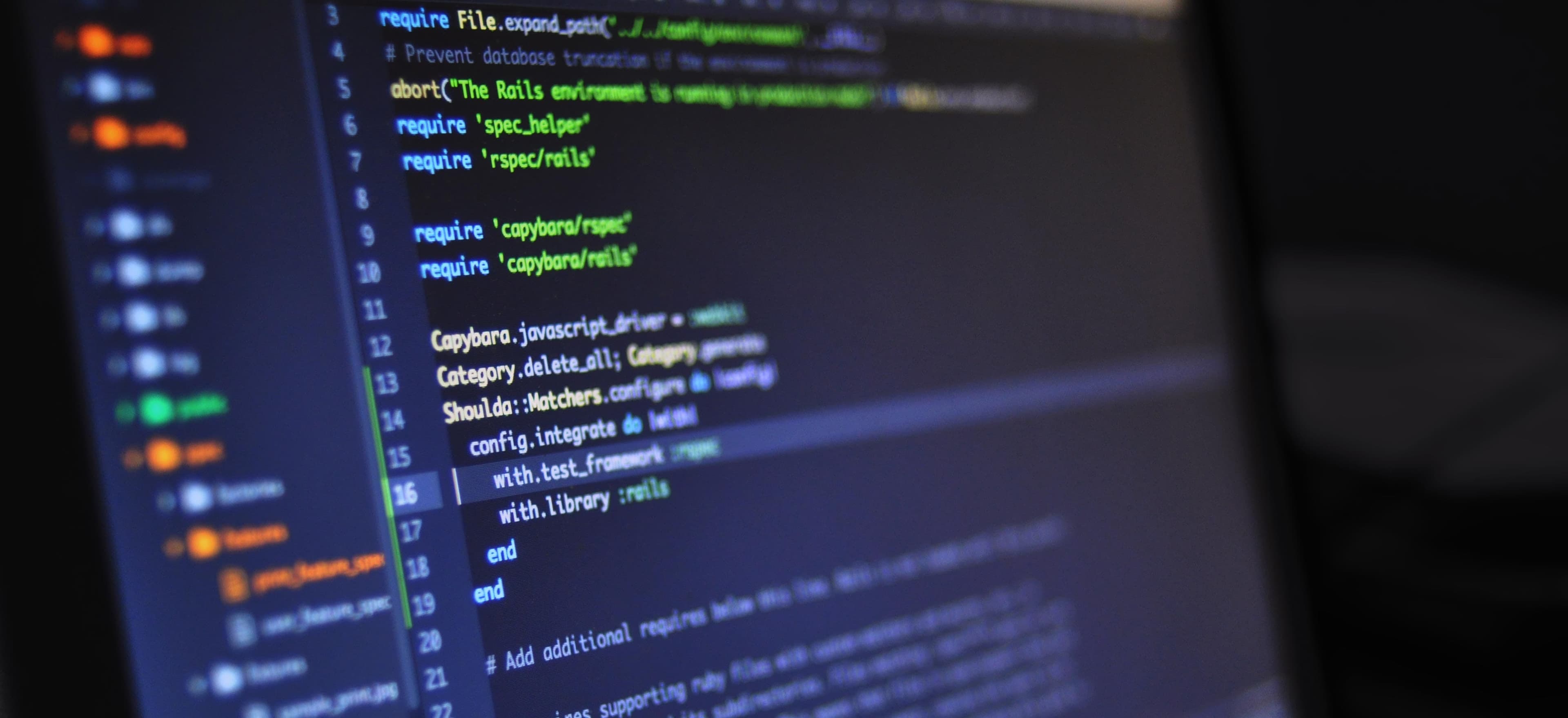
- Published on
Overcoming TDD Hurdles: Java Best Practices for Success
Test-Driven Development (TDD) continues to attract developers worldwide due to its promise of delivering more reliable and maintainable code. However, as outlined in the article "Common Challenges Faced in Test-Driven Development", the journey isn't free of obstacles. This blog post aims to delve into effective strategies to overcome these challenges, specifically in the context of Java programming.
What is Test-Driven Development?
Before we dive into solutions, let’s briefly review what TDD entails. TDD is a software development approach where tests are written before the actual code. The process is defined by a simple mantra: Red-Green-Refactor.
- Red: Write a failing test.
- Green: Write just enough code to pass the test.
- Refactor: Improve the code without changing its behavior.
Following this cycle helps in ensuring that your code meets the required specifications right from the start. However, developers often encounter hurdles when implementing TDD, particularly in a robust language like Java.
Key Challenges in TDD
1. Understanding the Requirements
One of the most significant challenges developers face is unclear or shifting requirements. If you’re not sure what the final product should look like, writing tests becomes almost impossible.
Solution: Invest time in gathering clear requirements from stakeholders. Engage in discussions, use user stories, and apply acceptance criteria to have a well-defined roadmap.
2. Writing Meaningful Tests
Many developers fall into the trap of writing tests that are either too broad or too narrow. Tests that are too broad can miss edge cases, while overly narrow tests can lead to a false sense of security.
Solution: Aim for the AAA pattern: Arrange, Act, and Assert. This structure makes your tests more readable and reliable.
Example: Writing a Simple Unit Test in Java
Here's a simple example demonstrating the AAA pattern using JUnit, a popular testing framework for Java:
import org.junit.jupiter.api.Test;
import static org.junit.jupiter.api.Assertions.*;
class CalculatorTest {
private Calculator calculator = new Calculator();
@Test
void testAdd() {
// Arrange
int a = 5;
int b = 3;
// Act
int result = calculator.add(a, b);
// Assert
assertEquals(8, result, "5 + 3 should equal 8");
}
}
Why This Code?:
- The
Arrange
phase sets up your variables and objects. - The
Act
phase executes the method you're testing. - The
Assert
phase validates that the output meets the expectations.
This well-structured format makes your tests easier to read, understand, and maintain.
3. Too Many Tests
While having tests is great, an overwhelming number of tests can lead to longer feedback cycles and negatively affect productivity.
Solution: Focus on writing meaningful and critical tests, particularly unit tests that truly validate the core functionality. Avoid redundancy where possible.
4. Test Maintenance
As your code evolves, tests may need to change as well. Letting tests go stale can lead to increasing maintenance burdens.
Solution: Regularly review and update your tests to keep them in sync with your application logic. Implement Continuous Integration/Continuous Deployment (CI/CD) practices to ensure tests are run automatically.
Practical Example: Refactoring Code
Refactoring your code while ensuring tests still pass is crucial in TDD. Let’s consider an example where we have a simple method in our Calculator
class that grows in complexity.
class Calculator {
public int add(int a, int b) {
return a + b;
}
}
If we need to optimize this addition in the future, we can easily refactor it while relying on the existing tests to ensure new changes don’t break old functionality.
5. Lack of Support
Sometimes, development teams are not familiar with TDD practices, leading to inconsistency and confusion.
Solution: Promote TDD as part of the team culture. Conduct workshops and share success stories highlighting the benefits of TDD. Create a conducive environment for learning and growth.
Best Practices for Successful TDD in Java
1. Leverage Mocking Frameworks
Mocking frameworks, like Mockito, can be game-changers in simulating components and dependencies during testing. They allow you to isolate the unit of code you want to test.
import static org.mockito.Mockito.*;
import org.junit.jupiter.api.Test;
class UserServiceTest {
private UserRepository userRepository = mock(UserRepository.class);
private UserService userService = new UserService(userRepository);
@Test
void testFetchUser() {
// Arrange
User user = new User("John Doe");
when(userRepository.findUserById(1)).thenReturn(user);
// Act
User result = userService.fetchUser(1);
// Assert
assertEquals("John Doe", result.getName());
}
}
Why This Code?: Mocking helps you test the UserService
without having to rely on the implementation of UserRepository
, allowing you to validate the service logic independently.
2. Write Tests that are Independent
Keep your tests independent so that one failing test does not impact others. This can be achieved by avoiding shared state and using dependency injection.
3. Continuously Educate Your Team
Training and shared knowledge can go a long way in overcoming common hurdles faced by TDD.
4. Proper Exception Handling in Tests
Testing can sometimes uncover exceptions in unexpected areas. Always be prepared to write tests that handle these cases gracefully.
@Test
void testDivisionByZero() {
assertThrows(ArithmeticException.class, () -> {
calculator.divide(5, 0);
});
}
Why This Code?: Here, we ensure our divide
method handles division by zero scenarios gracefully, which is a common edge case.
5. Keep Your Tests Up to Date
As previously mentioned, refactoring and updating your tests are critical as requirements change. Make a habit of revisiting and improving your tests regularly.
Lessons Learned
TDD may present several challenges, but with well-defined practices and techniques, developers can navigate through these hurdles, particularly in Java development. By implementing the best practices discussed in this blog post, you can not only improve the quality of your code but also create a more efficient software development process.
For those exploring TDD, remember to consult the article "Common Challenges Faced in Test-Driven Development" for further insights.
In the world of software development, embracing TDD is a step toward building resilient, maintainable, and high-quality software. Happy coding!
Checkout our other articles