Integrating Java with Django for Enhanced Data Science Solutions
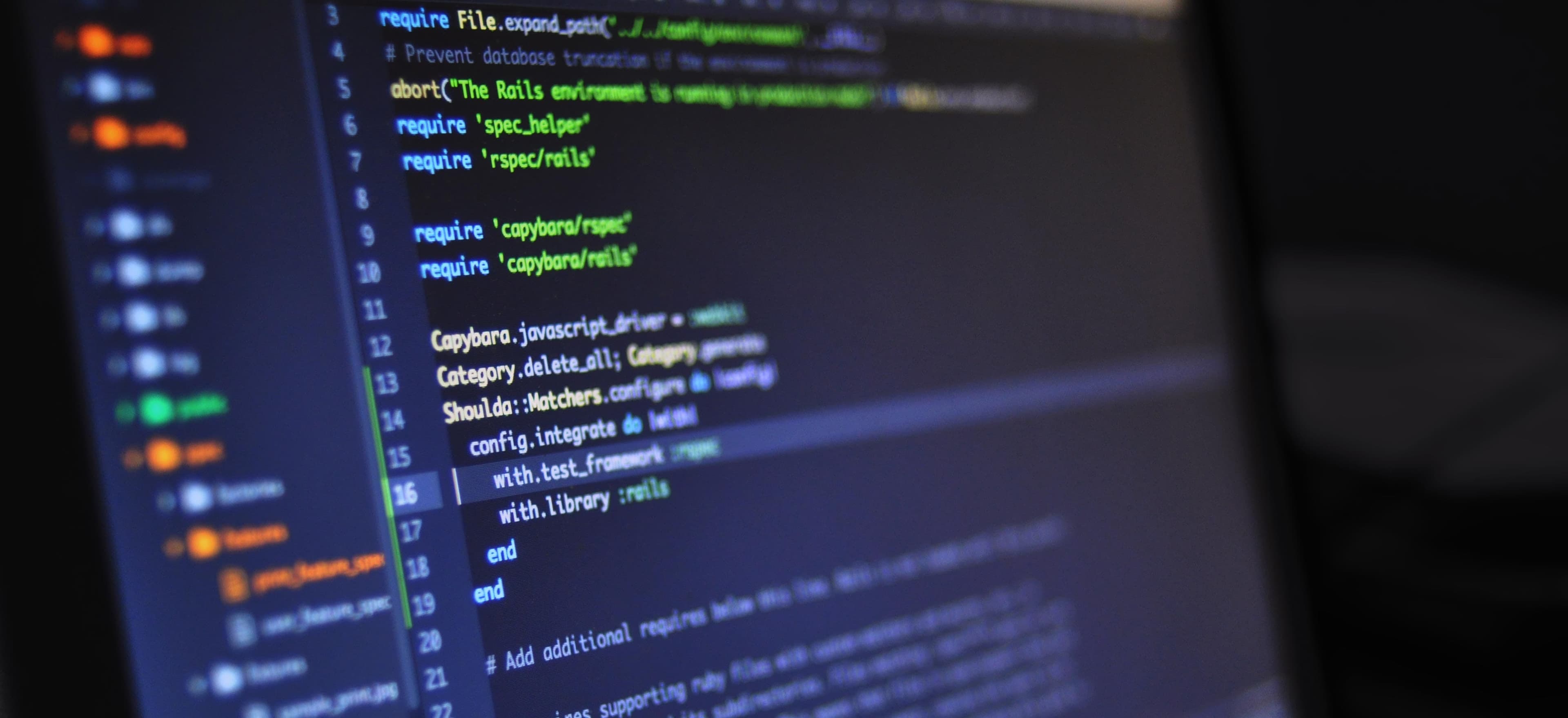
- Published on
Integrating Java with Django for Enhanced Data Science Solutions
In the ever-evolving world of data science, leveraging the strengths of multiple programming languages can greatly enhance your data-oriented projects. While Python is often the go-to language for many data scientists—thanks to its abundant libraries and frameworks—Java also offers powerful capabilities, especially in applications that demand performance and scalability. In this blog post, we will delve into how integrating Java with Django can provide robust solutions for data science projects.
Why Integrate Java with Django?
Django, a high-level Python web framework, allows for quick development and clean pragmatic design. However, Java brings its own set of strengths to the table:
- Performance: Java is known for its speed in processing large data sets.
- Scalability: Java applications can easily scale up as your data needs grow.
- Multi-threading: With inherent support for multithreading, Java can handle multiple tasks simultaneously without performance dips.
By combining these two languages, you can create applications that harness the best of both worlds—ease of web development with Django and high-performance computations from Java.
Setting Up the Project
To kick off our integration process, you need a Django project alongside a Java application.
Prerequisites
-
Python 3.x: Make sure Python is installed on your system.
-
Java Development Kit (JDK): Install the latest version of the JDK.
-
Django: Install Django using pip:
pip install django
-
Java Libraries: Include any Java libraries necessary for your project in your Java IDE.
Creating the Django App
Begin by creating a new Django project. Here’s how to set it up:
django-admin startproject myproject
cd myproject
python manage.py startapp myapp
This will create the basic scaffolding for your Django project. Your directory structure will look something like this:
myproject/
manage.py
myproject/
settings.py
urls.py
...
myapp/
models.py
views.py
...
Coding the Java Application
Now that the Django application is set up, let’s create a simple Java application that we can interact with. Below is an example of a Java class that performs data statistics calculations.
public class Statistics {
// Method to calculate mean of an array of doubles
public double calculateMean(double[] data) {
double sum = 0.0;
for (double num : data) {
sum += num;
}
return sum / data.length; // Average calculation
}
// Method to calculate median of an array
public double calculateMedian(double[] data) {
Arrays.sort(data); // Sorting array for median calculation
int length = data.length;
if (length % 2 == 0) {
return (data[length / 2 - 1] + data[length / 2]) / 2.0; // Even case
} else {
return data[length / 2]; // Odd case
}
}
}
Why This Java Approach?
- Efficiency: The array sorting and calculations are handled in Java, taking advantage of its performance efficiency.
- Scalability: Java can easily handle large datasets without causing lag or excessive memory usage.
Communication Between Java and Django
To enable communication between Django and Java, we can use HTTP requests. This allows your Django application to make API calls to your Java backend.
Creating a Simple API with Django
First, ensure your Django app is configured to handle API requests. You can use Django REST framework for this:
-
Install Django REST framework:
pip install djangorestframework
-
Update your Django settings to include it:
INSTALLED_APPS = [ ... 'rest_framework', 'myapp', ]
-
Create an API endpoint in
views.py
:
from rest_framework.views import APIView
from rest_framework.response import Response
import requests
class StatisticsView(APIView):
def post(self, request, format=None):
# Extract data from incoming request
data = request.data.get("data")
# Call the Java service (Make sure you have Java running an API)
response = requests.post("http://localhost:8080/calculate", json={'data': data})
# Handle the result from Java, making sure you parse it correctly
result = response.json()
return Response(result)
Setting Up the Java API
For the Java part, you can develop a lightweight REST API using Spring Boot. Here is a simple controller that provides statistical calculations:
@RestController
public class StatsController {
@PostMapping("/calculate")
public ResponseEntity<Map<String, Double>> calculateStats(@RequestBody double[] data) {
Statistics stats = new Statistics();
double mean = stats.calculateMean(data);
double median = stats.calculateMedian(data);
Map<String, Double> result = new HashMap<>();
result.put("mean", mean);
result.put("median", median);
return ResponseEntity.ok(result); // Send response back to Django
}
}
Testing the Integration
Make sure your Java application is running and listening for requests.
You can use a simple curl command to test the integrated setup from your Django app:
curl -X POST http://localhost:8000/api/stats/ -d '{"data": [1, 2, 3, 4, 5]}' -H "Content-Type: application/json"
Ensure to update your urls.py
to include a path for the StatisticsView
so the request can be properly routed.
from django.urls import path
from myapp.views import StatisticsView
urlpatterns = [
path('api/stats/', StatisticsView.as_view(), name='stats'),
]
My Closing Thoughts on the Matter
Integrating Java with Django can yield a powerful setup for data science applications. By utilizing Django's quick web-development capabilities alongside Java's performance and scalability, developers can create robust solutions that handle significant data loads efficiently.
For those interested in data science workflows, consider checking out the existing article, "Boosting Data Science Work with Django: Key Benefits" from configzen.com, for more understanding of how Django can assist in your data science projects.
Final Thoughts
As we’ve explored, marrying the capabilities of Java and Django offers promising advantages. Whether you’re building high-performance data applications or creating scalable web interfaces, this integration will significantly enhance your project's capabilities. By selecting the right tools and implementing efficient methodologies, you can ensure success in the fast-paced world of data science.
Checkout our other articles