Tackling Fragment Lifecycle Issues in Java Applications
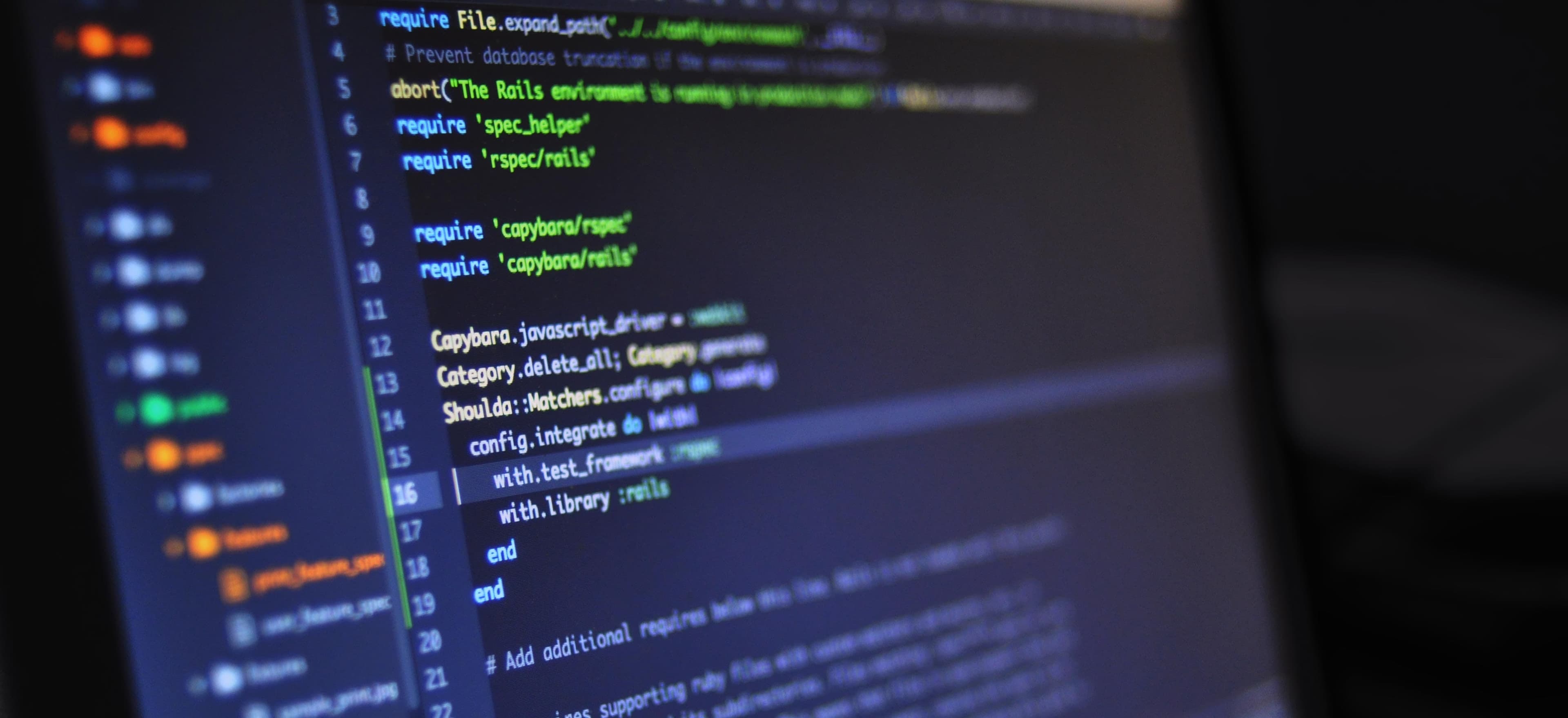
- Published on
Tackling Fragment Lifecycle Issues in Java Applications
When developing Java applications, particularly in Android, understanding the Fragment lifecycle is crucial. The Fragment lifecycle poses unique challenges and complexities that developers must navigate. In this blog post, we will explore these challenges in depth, looking at how to effectively manage Fragment states while developing robust and user-friendly applications.
Understanding Fragment Lifecycles
Before we dive deeper into the issues, let's summarize the Fragment lifecycle. A Fragment goes through several states, including:
- Created: The Fragment is being created.
- Started: The Fragment is visible to the user.
- Resumed: The Fragment is in the foreground and interactive.
- Paused: The Fragment is partially obscured.
- Stopped: The Fragment is no longer visible.
- Destroyed: The Fragment is being removed.
Each of these states has associated lifecycle callbacks, such as onCreate()
, onStart()
, onResume()
, onPause()
, onStop()
, and onDestroy()
. Understanding these states and their transitions is vital for managing UI and data within Fragments effectively.
Common Fragment Lifecycle Issues
The Fragment lifecycle is inherently complex, leading to several common challenges:
-
State Management: Retaining data across configuration changes, such as screen rotations, can be a nightmare if not handled correctly.
-
Memory Leaks: Improper management of Fragments can lead to leaked instances, which might hold onto references that prevent garbage collection.
-
Improper Lifecycle Handling: Calling UI operations in the wrong lifecycle method can lead to crashes or unexpected behavior.
-
Navigational Challenges: Moving back and forth between Fragments can lead to instantiation issues and incorrect Fragment states.
Detailed Exploration of Challenges
1. State Management
Many developers tend to use static variables or singleton patterns to retain state; however, both come with risks of leaks and inconsistency. A better approach is to use the onSaveInstanceState
method. This allows you to store necessary data safely.
@Override
public void onSaveInstanceState(Bundle outState) {
super.onSaveInstanceState(outState);
outState.putString("key", "value");
}
The outState
Bundle can be retrieved in onCreate()
of the Fragment:
@Override
public void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
if (savedInstanceState != null) {
String value = savedInstanceState.getString("key");
// Use the value to restore UI state
}
}
By leveraging onSaveInstanceState
, we can store transient UI state effectively and retrieve it when the Fragment is recreated.
2. Memory Leaks
Memory leaks can become a significant issue, especially with Fragments retaining references to Activity contexts. This commonly arises when using static references or callbacks. You can avoid leaks by using WeakReference
.
Example:
public class MyFragment extends Fragment {
private WeakReference<Context> contextRef;
@Override
public void onAttach(Context context) {
super.onAttach(context);
contextRef = new WeakReference<>(context);
}
// Use contextRef.get() when necessary, ensuring context has not been GC'd
}
Using WeakReference
allows for garbage collection of context when it's no longer in use, mitigating memory leaks.
3. Improper Lifecycle Handling
Calling UI modifications in the wrong lifecycle methods is a common pitfall. A good rule of thumb is to perform UI updates in onResume()
to ensure that they are only done when the Fragment is visible and not in the process of being created or destroyed.
@Override
public void onResume() {
super.onResume();
updateUI();
}
private void updateUI() {
// UI update logic
// Safe to run as the Fragment is in the foreground
}
This strategy not only improves the stability of your application but ensures that user interactions are seamless.
4. Navigational Challenges
Fragment back stack management can often be troublesome if not meticulously handled. When replacing Fragments, always ensure that you are managing the back stack correctly.
Here is a sample of how to add to the back stack during a Fragment transaction:
Fragment newFragment = new MyFragment();
FragmentTransaction transaction = getSupportFragmentManager().beginTransaction();
transaction.replace(R.id.fragment_container, newFragment);
transaction.addToBackStack(null); // This ensures that you can navigate back
transaction.commit();
The addToBackStack
method adds the transaction to the back stack, allowing for consistent navigation without unexpected behavior.
Best Practices for Fragment Lifecycle Management
To manage Fragment lifecycles effectively, consider these best practices:
-
Use
onSaveInstanceState
for State Management: Always save transient state inonSaveInstanceState()
. This practice frees you from maintaining state manually and reduces the risk of errors. -
Avoid Long Process in Lifecycle Methods: Heavy computations should be deferred to worker threads to avoid blocking UI updates.
-
Lifecycle-Aware Components: Utilize Android’s architecture components, such as LiveData and ViewModel, for efficient state management.
-
Regularly Reference Mastering Dynamic Fragments: Common Challenges Uncovered for further insights into handling dynamic Fragments.
-
Clear Navigation Flow: Maintain a clear navigation flow using the Navigation component to ease the complexities associated with Fragment transitions.
The Last Word
As we’ve discovered, the Fragment lifecycle in Java applications introduces several challenges, but managing them is possible with a solid grasp of lifecycle callbacks, best practices, and proper state management techniques. By following the strategies outlined in this article, you will steer clear of common pitfalls and ensure smooth user experiences.
Remember, the goal is to create applications that not only function correctly but also provide a seamless interaction experience for your users. With diligent care in managing Fragment lifecycle issues, you can achieve that balance.
For further reading on related topics, don't forget to check out existing literature like "Mastering Dynamic Fragments: Common Challenges Uncovered" at infinitejs.com/posts/mastering-dynamic-fragments-challenges. Happy coding!
Checkout our other articles