Troubleshooting JavaScript Compatibility in Internet Explorer
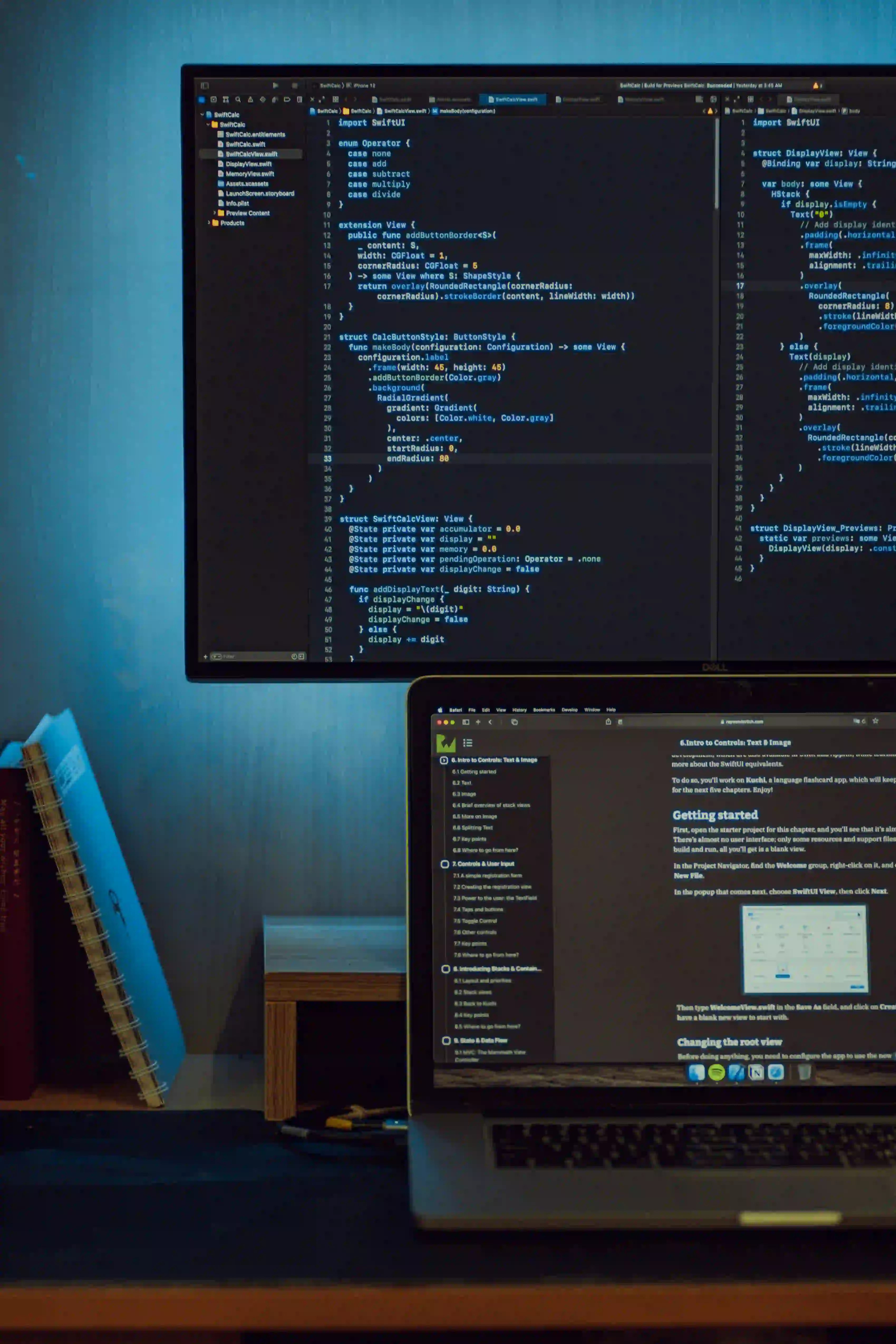
Troubleshooting JavaScript Compatibility in Internet Explorer
JavaScript is the backbone of modern web development, but dealing with Internet Explorer (IE) can be a headache for developers. Known for its quirks and inconsistent support for web standards, IE can turn simple tasks into major challenges. In this blog post, we will delve into how to troubleshoot JavaScript compatibility issues in Internet Explorer and provide solutions that will save you time and frustration.
Understanding Internet Explorer's JavaScript Limitations
Before we dive into troubleshooting, let's understand some common JavaScript compatibility issues in Internet Explorer:
-
ES5 and ES6 Support: Internet Explorer only fully supports ECMAScript 5. Features introduced in ES6 and beyond, such as promises, modules, and arrow functions, are not natively available.
-
Event Handling: The difference in how events are handled can lead to unexpected behavior in IE. Functions like
addEventListener
are missing. -
CSS Manipulation: Many CSS properties and selectors are not fully supported, impacting the user interface.
-
AJAX Calls: The
XMLHttpRequest
object has nuances that behave differently in IE compared to modern browsers.
Now that we have identified the issues, let's explore how to troubleshoot these problems.
1. Use Feature Detection
One of the best practices in JavaScript development is to use feature detection. Rather than checking for browser versions, we can check if specific features or functions are available. For example, if your code uses addEventListener
, you can write:
if (window.addEventListener) {
// Modern browsers
element.addEventListener('click', function() {
// Your code here
});
} else {
// IE fallback
element.attachEvent('onclick', function() {
// Your code here
});
}
Why Use Feature Detection?
Using feature detection instead of browser detection helps ensure that your code remains compatible with future versions of browsers that might add support for previously unsupported features.
2. Use Polyfills for Missing Functionality
When writing code for modern features that Internet Explorer does not support, using polyfills can help bridge the gap. A polyfill is a piece of code that provides a fallback for features that are missing.
For instance, if you are using Promise
, you can include a polyfill like this:
<script src="https://cdnjs.cloudflare.com/ajax/libs/es6-promise/4.2.8/dist/es6-promise.auto.min.js"></script>
Why Use Polyfills?
Polyfills allow you to write modern JavaScript while maintaining compatibility with older browsers. This means less duplicate code and a cleaner codebase.
3. Transpiling Your Code
Another effective solution is to use a transpiler, such as Babel. This converts ES6+ code into ES5 code that is compatible with Internet Explorer. Here’s how to set it up:
-
Install Babel CLI and Preset:
🔧snippet.shnpm install --save-dev @babel/core @babel/cli @babel/preset-env
-
Configure Babel: Create a file named
.babelrc
in your project directory and add the following:📋snippet.json{ "presets": [ ["@babel/preset-env", { "targets": { "ie": "11" } }] ] }
-
Transpile Your Code: Run Babel to transpile your JavaScript files:
🔧snippet.shnpx babel src --out-dir lib
Why Transpile Your Code?
Transpilation makes it easier to use modern JavaScript features while ensuring that your code runs seamlessly across all browsers, including older versions like Internet Explorer.
4. Debugging with Developer Tools
Internet Explorer provides developer tools to help debug your JavaScript. By pressing F12, you can access the console, profiling, and other useful features. Pay attention to command errors and performance bottlenecks.
Tips for Using Developer Tools
-
Console Logging: Use
console.log
to trace function calls, variable values, and identify where the issues occur. -
Debugging Breakpoints: Set breakpoints to pause execution and inspect the current state of your application.
5. Testing in Internet Explorer
To properly troubleshoot JavaScript compatibility, you must test your application in Internet Explorer. Try these methods:
-
Virtual Machines: Microsoft provides free Virtual Machines for testing different versions of IE.
-
BrowserStack: Use services such as BrowserStack for cross-browser testing on actual IE environments.
The Last Word
Troubleshooting JavaScript compatibility in Internet Explorer can seem daunting, but understanding the limitations and applying the strategies above will help you create robust applications. Remember that while fixing compatibility issues may take initial effort, it will pay dividends in the long run by providing a seamless experience for all users.
If you encounter specific issues, consider consulting resources like the article on Fixing Wow Slider Issues in Internet Explorer which covers troubleshooting specific libraries.
By incorporating best practices like feature detection, polyfilling, and testing, you can navigate the challenges of Internet Explorer effectively. Happy coding!