Resolving JavaScript Conflicts in Wow Slider for IE Users
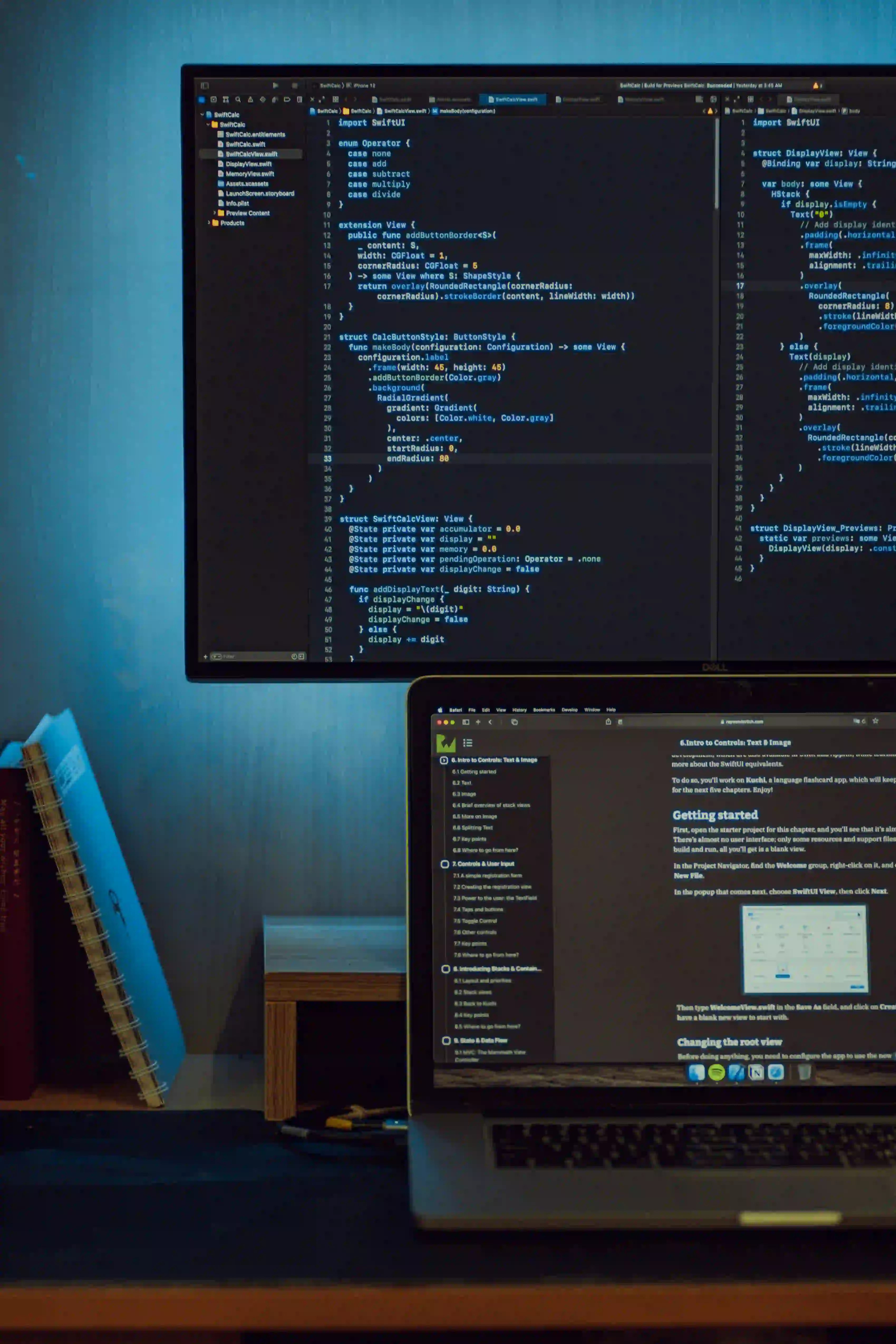
Resolving JavaScript Conflicts in Wow Slider for IE Users
When dealing with web development, cross-browser compatibility always poses a significant challenge. One specific area of concern is JavaScript conflicts, particularly with Internet Explorer (IE). This blog post will delve into effective strategies for resolving JavaScript conflicts in the Wow Slider, a popular jQuery plugin used to create stunning image slideshows.
Understanding Wow Slider
Wow Slider is an advanced jQuery slider plugin that offers dynamic effects, user-friendly features, and rich visual presentation options. Users often resort to it to enhance their websites' aesthetic appeal. However, many developers face issues, particularly when their websites are accessed via Internet Explorer.
For more comprehensive details about the specific Wow Slider issues encountered in IE, check out the article titled Fixing Wow Slider Issues in Internet Explorer.
Why JavaScript Conflicts Occur
JavaScript conflicts can arise from multiple sources, including:
- Library Compatibility: Different JavaScript libraries may not work harmoniously together.
- DOM Manipulation: Changes on the Document Object Model (DOM) by one script can interfere with others.
- Event Handling: Conflicting event listeners can disrupt functionality.
In Internet Explorer, these conflicts can be exacerbated due to its unique implementation of JavaScript and CSS.
Analyzing the Issue
Before implementing any solutions, it's essential to analyze the specific problem. Use the built-in developer tools in IE (press F12) to access the console and check for any error messages.
A typical error related to the Wow Slider might look like this:
Uncaught TypeError: 'undefined' is not a function
This error suggests that a required function is either not defined or is overwritten by another library.
Solutions for JavaScript Conflicts in Wow Slider
1. Use jQuery No Conflict Mode
One common resolution is using jQuery's no conflict mode. When you load jQuery, it uses $
as a shortcut to reference its functions. However, if another library uses $
, you may encounter conflicts.
Here’s how to implement no conflict mode:
// Initialize jQuery in no conflict mode
jQuery.noConflict();
jQuery(document).ready(function($) {
// Your Wow Slider initialization code here
$("#wowslider-container").wowSlider({
// Slider options
});
});
By placing your initialization code within the ready()
method of jQuery, you can ensure that the $
can be referenced safely.
2. Load Scripts in the Correct Order
Loading scripts in the wrong order can also cause issues. Ensure that jQuery is loaded before other libraries and plugins, especially Wow Slider. Here’s how your HTML <head>
section should look:
<head>
<script src="https://code.jquery.com/jquery-3.6.0.min.js"></script>
<script src="path/to/wowslider.js"></script>
<link rel="stylesheet" type="text/css" href="path/to/wowslider.css">
</head>
Make sure jQuery is at the top, followed by Wow Slider scripts and styles.
3. Ensure Consistent jQuery Versions
Using inconsistent versions of jQuery can lead to functionality issues. Always check the Wow Slider documentation to ensure you are using a compatible version of jQuery.
Here’s a simple snippet to confirm the version once loaded:
console.log("jQuery Version: " + jQuery.fn.jquery);
The above will log the current version of jQuery in the console, which can help identify whether it's compatible with other libraries.
4. Handle Event Conflicts
Conflicts can also occur with event handlers. When attaching events, ensure to use delegation where applicable:
jQuery(document).on('click', '.your-class', function() {
// Your click handling code
});
Using .on()
allows for event delegation and reduces conflicts with other scripts.
5. Graceful Handling of Errors
Implementing error handling in your JavaScript can aid in troubleshooting. Here is an example of how to handle JavaScript errors:
window.onerror = function(message, source, lineno, colno, error) {
console.log("Error message: " + message +
"\nSource: " + source +
"\nLine number: " + lineno +
"\nColumn number: " + colno);
};
This function will allow you to log errors in the console, giving insight into any problems that arise.
6. Utilize Fallbacks for IE
Internet Explorer may not support modern JavaScript functions, leading to further compatibility issues. For this reason, utilizing feature detection can provide fallbacks:
if (!Array.prototype.forEach) {
// Polyfill for forEach
}
This checks if the forEach
method is available on the Array
prototype. If not, it allows you to implement alternative logic.
Testing and Debugging
Testing extensively across all browsers is crucial. Make use of IE’s debugging tools:
- Use the F12 Developer Tools to check console logs.
- Employ network tracing to ensure all resources load correctly.
- Utilize the timing tab to see if any scripts are causing delays.
By following a systematic approach, developers can easily identify and resolve conflicts.
My Closing Thoughts on the Matter
Resolving JavaScript conflicts in the Wow Slider for IE users can be manageable with the right strategies. Using no conflict mode, ensuring proper script order, consistent jQuery versions, delegation, and implementing error handling will greatly increase compatibility across browsers.
By being proactive in testing and debugging, you can provide a seamless experience for users, enhancing your website's quality.
For any specific Wow Slider issues you're facing, I refer you to an excellent resource, the article titled Fixing Wow Slider Issues in Internet Explorer, which dives deeper into common challenges.
Now you’re ready to tackle those pesky JavaScript conflicts head-on while leveraging Wow Slider on your site! Happy coding!