Transforming Java Arrays: Effective Use of Streams to Simplify
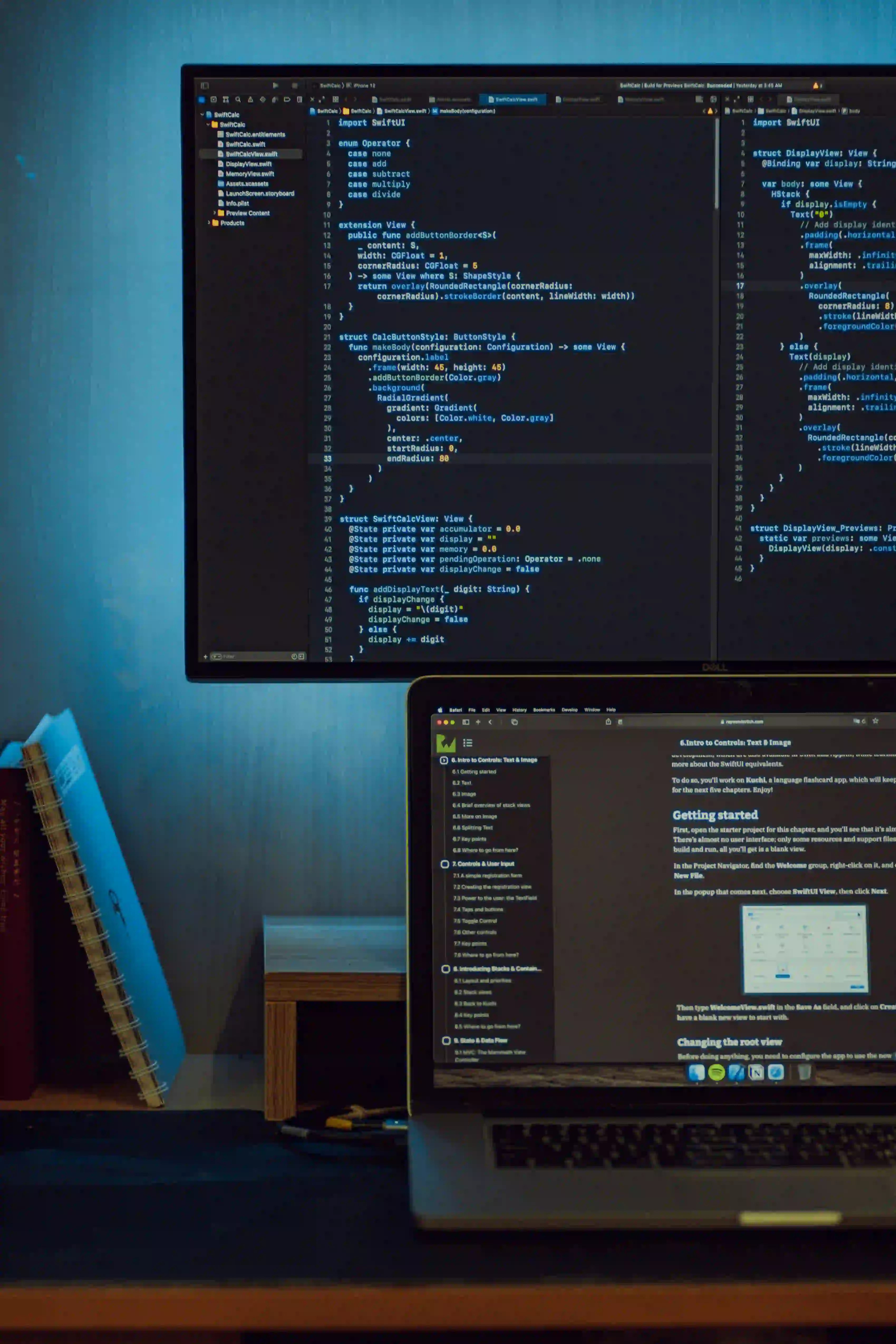
Transforming Java Arrays: Effective Use of Streams to Simplify
Java has long provided us with robust tools for handling arrays, but many developers are unaware of the power of Java Streams for simplifying array operations. First introduced in Java 8, Streams have revolutionized the way we process collections of data, offering a more readable and efficient way of handling arrays. In this blog post, we'll explore how to leverage streams to transform arrays effectively while exploring why these operations can lead to cleaner and more maintainable code.
Understanding Java Streams
Java Streams are part of the java.util.stream
package and provide a high-level abstraction for performing functional operations on sequences of elements. This includes manipulating arrays and collections, filtering data, and executing operations using functional programming principles.
Why Use Streams?
Streams can be beneficial over traditional approaches for several reasons:
- Readability: Stream operations can often read more like plain English.
- Conciseness: You can achieve complex transformations in less code.
- Laziness: Streams are computed lazily, meaning they only compute results when required.
- Parallel processing: Streams can easily leverage multicore processors for parallel operations using
.parallelStream()
.
Getting Started with Streams
To use Streams, we first need to import the necessary classes:
import java.util.Arrays;
import java.util.List;
import java.util.stream.Collectors;
Next, let's take a look at a simple array of integers and perform some common transformations.
int[] numbers = {1, 2, 3, 4, 5};
Transforming Arrays to Lists
One of the common tasks in programming is converting arrays into lists. Using streams, this task can be performed elegantly. Here’s how to convert an array into a list:
List<Integer> numberList = Arrays.stream(numbers)
.boxed()
.collect(Collectors.toList());
Why This Works:
- The
Arrays.stream(numbers)
converts theint
array into a stream ofint
. - The
.boxed()
method converts theint
primitive type intoInteger
objects. - Finally,
.collect(Collectors.toList())
gathers the elements into aList
.
Filtering with Streams
Filtering arrays is another powerful feature of Streams. Let's say we want to filter the even numbers from our initial array.
int[] evenNumbers = Arrays.stream(numbers)
.filter(num -> num % 2 == 0)
.toArray();
Why Use filter()
:
- The
filter()
method allows for the application of a predicate—a condition that each element must satisfy to be included in the result.
To see the filtered result, you can iterate through the evenNumbers
array:
System.out.println(Arrays.toString(evenNumbers)); // Outputs: [2, 4]
Mapping Elements
Mapping is another essential operation that transforms each element in a stream. Suppose we want to square each number in our original array.
int[] squaredNumbers = Arrays.stream(numbers)
.map(num -> num * num)
.toArray();
Why Mapping Is Useful:
- The
.map()
method applies the specified function to each element, transforming them into a new form.
Chaining Operations
One of the most powerful aspects of Java Streams is method chaining:
int[] processedNumbers = Arrays.stream(numbers)
.filter(num -> num > 2)
.map(num -> num * num)
.toArray();
This performs a filter and a transformation in a single, succinct line. Understanding method chaining can boost productivity and clarity in your code.
Reducing with Streams
Reduction operations like summing or finding maximum values are also efficient with Streams. For instance, to sum up all the numbers in our array:
int sum = Arrays.stream(numbers)
.reduce(0, Integer::sum);
Why Use Reduce?:
- The
reduce()
method collapses a stream of values into a single value. Here, the first argument (0) is the identity value, andInteger::sum
tells the stream to sum the elements.
Real-World Example
Imagine an application that receives data about users and needs to filter, transform, and collect that data. Here's a more complex example:
Assuming we have a user class defined as follows:
public class User {
private String name;
private int age;
// constructor, getters, and setters
}
List<User> users = Arrays.asList(
new User("Alice", 30),
new User("Bob", 20),
new User("Charlie", 35)
);
We can use streams to filter users older than 25, transform their names to uppercase, and collect names into a list:
List<String> userNames = users.stream()
.filter(user -> user.getAge() > 25)
.map(User::getName)
.map(String::toUpperCase)
.collect(Collectors.toList());
Why Is This Beneficial?
- This segment of code is concise and readable. Stream operations clearly define the flow from filtering to transformation without the need for traditional loops and conditionals.
Lessons Learned
Java Streams present a powerful and flexible way to transform arrays and collections, promoting cleaner and more maintainable code. Learning to utilize Streams effectively—not only in transforming but also filtering and aggregating data—can greatly enhance your Java programming skills.
For further reading about handling arrays and common pitfalls, you may find this article useful: Mastering Arrays: Avoid Common Pitfalls with Map, Filter & Reduce.
Next Steps
- Experiment with Stream operations in your projects.
- Explore more complex transformations with nested data.
- Look into parallel streams for performance improvements on large datasets.
Your journey into Java Streams can make your code more efficient, maintainable, and elegant. Start applying these principles today and simplify your Java programming!