Handling Dynamic Data in Java with JavaScript Integration
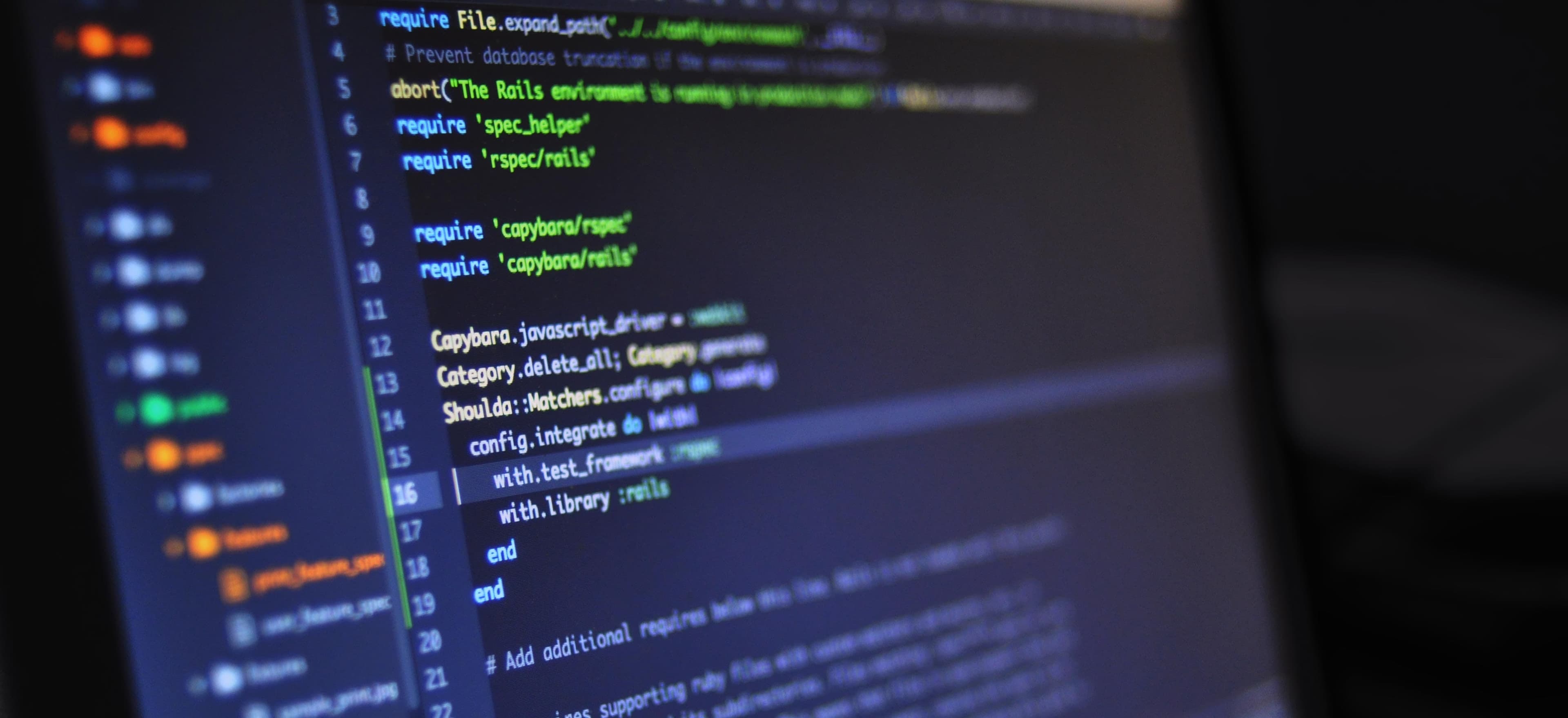
- Published on
Handling Dynamic Data in Java with JavaScript Integration
In modern web applications, the interplay between front-end and back-end technologies is crucial to delivering dynamic and interactive user experiences. One common scenario is managing dynamic data, especially when dealing with input fields generated at runtime. In this blog post, we will explore how to manage dynamic data in Java and facilitate integration with JavaScript, particularly focusing on how to work with values collected from dynamically created text boxes. This discussion will include code examples and best practices.
Understanding Dynamic Data and Its Importance
Dynamic data refers to information that is generated in real-time and can change based on user interaction, such as input from a text box. Managing dynamic data efficiently is vital for enhancing user experience and application performance.
For instance, imagine a form where users can add multiple text fields for notes. As users create and fill in these fields, the application needs to collect, process, and potentially store this information.
Why Integrate Java and JavaScript?
Java is predominantly used on the server side, while JavaScript (JS) is favored for front-end development. Integrating the two allows the server to handle business logic while JS manages user interactions. This makes your application more responsive. As a result, understanding how to pass data back and forth between Java and JavaScript will enhance your web application’s capabilities.
Collecting Dynamic Data in JavaScript
Before we delve into the Java aspects, let’s look at how to gather dynamic text box values using JavaScript. We can refer to an existing article, Storing Dynamic Text Box Values in JavaScript Arrays, which details the JavaScript side of things.
Here’s a simple example of how you can gather values from dynamically created text boxes.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Dynamic Text Boxes</title>
</head>
<body>
<div id="dynamicTextBoxes"></div>
<button id="addTextBox">Add Text Box</button>
<button id="submit">Submit</button>
<script>
document.getElementById('addTextBox').addEventListener('click', function() {
const textBox = document.createElement('input');
textBox.type = 'text';
textBox.name = 'dynamicTextBox[]';
document.getElementById('dynamicTextBoxes').appendChild(textBox);
});
document.getElementById('submit').addEventListener('click', function() {
const inputs = document.getElementsByName('dynamicTextBox[]');
const values = [];
for (let input of inputs) {
values.push(input.value);
}
console.log(values); // For demonstration, we simply log the values
});
</script>
</body>
</html>
Explanation of the JavaScript Code
-
Dynamic Text Box Creation: When the "Add Text Box" button is clicked, a new
input
element is created and appended to thediv
element. -
Collecting Values: Upon clicking the "Submit" button, we loop through all input elements with the name
dynamicTextBox[]
. Each value is pushed into thevalues
array. -
Outputting Values: Finally, we simply log the collected values to the console.
This JavaScript handles user-generated input in a seamless and dynamic way. The next step involves what happens on the server side when this dynamic data is sent to a Java application.
Handling Data on the Java Side
Once we gather values on the front end, the next logical step is to pass this information to a Java backend for processing or storage. Let's simulate this with a Spring Boot application.
Example Java Controller in Spring Boot
Below is a simple controller that can handle the incoming data.
import org.springframework.web.bind.annotation.*;
import java.util.List;
@RestController
@RequestMapping("/api")
public class DynamicDataController {
@PostMapping("/submitDynamicData")
public String handleDynamicData(@RequestBody List<String> dynamicValues) {
// Process the received values
StringBuilder responseBuilder = new StringBuilder("Received Values: ");
for(String value : dynamicValues) {
responseBuilder.append(value).append(" ");
}
// Here you can also save the values into a database or perform other logic
return responseBuilder.toString();
}
}
Explanation of the Java Code
-
Controller Annotation: We define a REST controller with
@RestController
. The@RequestMapping
annotation specifies the base path for this controller. -
Handling POST Requests: The
handleDynamicData
method processes data sent as aPOST
request. It expects a list of strings (the dynamic values collected from the frontend). -
Response Construction: The method constructs a response string that echoes the received values. You can extend this method to include logic for storing data in a database or performing other business operations.
Wiring Up the Frontend and Backend
To send the collected data from JavaScript to the Java backend, we can leverage the fetch
API in the submit button's click event.
Here’s how you can modify the submit event in the JavaScript code:
document.getElementById('submit').addEventListener('click', function() {
const inputs = document.getElementsByName('dynamicTextBox[]');
const values = [];
for (let input of inputs) {
values.push(input.value);
}
// Sending the data to the Java backend
fetch('/api/submitDynamicData', {
method: 'POST',
headers: {
'Content-Type': 'application/json'
},
body: JSON.stringify(values)
})
.then(response => response.text())
.then(data => console.log(data))
.catch((error) => console.error('Error:', error));
});
Explanation of the Updated Code
-
Sending Data: We create a
fetch
request to the server, specifying it as aPOST
request. -
Headers and Body: We set the
Content-Type
toapplication/json
and stringify the data to send it in JSON format. -
Handling the Response: We handle the response by logging it to the console.
Key Takeaways
Integrating Java and JavaScript for handling dynamic data enhances the overall functionality of your web application. The discussed methods allow seamless interaction and data management between the front end and back end.
From dynamically collecting input via JavaScript to processing it on a Java server, you can deliver a fully interactive experience for your users. The example discussed illustrates foundational concepts that can be expanded with additional functionalities such as input validation, error handling, and database storage.
By understanding how to efficiently manage dynamic data and integrate your technologies, you can significantly improve user experience and application performance.
For more insights about managing dynamic text box values with JavaScript, you can read the article Storing Dynamic Text Box Values in JavaScript Arrays.
Happy coding!
Checkout our other articles