Enhancing Java Applications Through Effective Storytelling
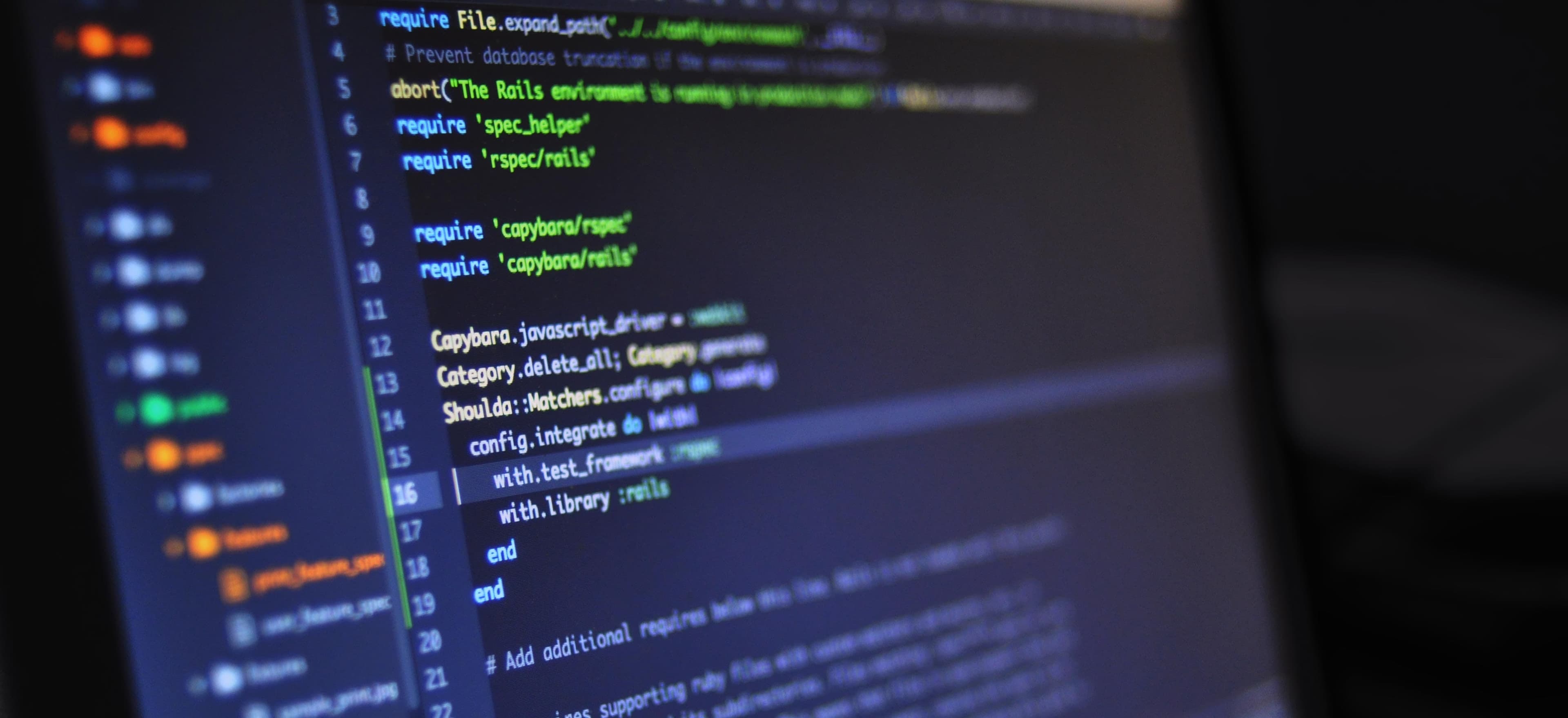
- Published on
Enhancing Java Applications Through Effective Storytelling
Storytelling is often relegated to the realm of literature and entertainment, but its principles can dramatically enhance user experience (UX) design in software applications. In Java development, incorporating storytelling into your application can improve user engagement, retention, and satisfaction. In this blog post, we will explore how effective storytelling can transform your Java applications and provide actionable advice on integrating these principles into your projects.
Understanding the Importance of Storytelling in UX Design
To effectively understand why storytelling matters, consider this: stories have an innate ability to evoke emotions. They engage users and provide them with context, helping them navigate their interactions with software. For a deeper dive into how poor storytelling can impact UX design, refer to the article "How Poor Storytelling Can Ruin UX Design" at tech-snags.com/articles/poor-storytelling-ux-design.
When users embark on a journey through your Java application, what you present is crucial. If they encounter a disjointed sequence of features or lack clarity on your application's purpose, they will likely abandon it before reaping any benefits. Here are some ways storytelling can enhance your Java applications:
Elements of Effective Storytelling in Java
-
Character Development
The user is the hero of the story. Ensure that they can relate to the character you create for your application. How do users interact with your software? What challenges do they face? All these questions help shape the narrative.Example Code Snippet: A simple Java class representing a user character can encapsulate this concept.
public class User { private String name; private String role; private int score; public User(String name, String role) { this.name = name; this.role = role; this.score = 0; // initial score } public void increaseScore(int points) { this.score += points; System.out.println(name + " now has a score of " + score); } // Other user-related methods } public static void main(String[] args) { User user = new User("Alice", "Explorer"); user.increaseScore(10); }
Here, we define a 'User' class to serve as the protagonist of the application. This class encapsulates user properties and actions that enhance their narrative experience.
-
Conflict and Resolutions
Every story requires challenges — think of your application's features as obstacles. How do users overcome them? Providing appropriate feedback or tutorials is essential here.For instance, if your application stores user data, consider scenarios where data retrieval might fail. Provide meaningful error messages that guide users back on their journey.
public void fetchData() { try { // simulate data fetching throw new IOException("Data could not be retrieved."); } catch (IOException e) { System.out.println("Error: " + e.getMessage()); System.out.println("Try refreshing your data or check your internet connection."); } }
In this snippet, we demonstrate error handling that respects the user’s journey, offering a path towards resolution rather than despair.
-
Setting
Just as a story needs a backdrop, your application does too. Use design and color themes that resonate with your desired narrative. Java's Swing or JavaFX can help you create visually-appealing interfaces that represent the world of your application effectively.
Crafting the User Journey
Using storytelling principles extends beyond character and conflict; it involves mapping the entire user journey.
-
Onboarding: This should introduce users to the narrative, giving them a sense of purpose.
- Use guided tutorials to unveil functionalities in a story-like format.
- Provide incentives to keep them engaged.
-
Feature Presentation: Each feature must interlock with the overall plot. Don’t just list features; present them as vital steps in the user’s adventure.
-
Feedback Loop: Provide users with rewards and recognition through thoughtful feedback.
public void showFeedback(String message) { System.out.println("Feedback: " + message); // Imagine this being a popup or a notification }
This snippet demonstrates a simple feedback method that could display various messages based on user interaction, encouraging continued engagement.
Utilizing Java Libraries for Storytelling
To enhance storytelling in your Java applications, consider leveraging certain libraries that can assist you in creating compelling narratives:
-
JavaFX: A powerful framework for creating rich Internet applications. JavaFX allows you to design engaging graphical interfaces that can immerse users in your application's story.
-
Apache Commons: For clean coding practices, this library can help streamline your Java code by offering reusable components and utilities.
-
JUnit: To ensure your storytelling components work as intended, rigorous testing is necessary. JUnit allows you to create unit tests that can help verify your narrative components.
Testing Your Story
Once you have implemented storytelling principles in your Java application, testing becomes essential to understand user engagement and satisfaction levels. There’s no better way to ensure that each part of the story is functioning as intended.
- User Testing: Gather feedback from actual users and see if they relate to the narrative you’ve structured.
- A/B Testing: Experiment with different storytelling elements, like onboarding flows or important messages, to see which resonates better with users, enhancing your application's UX.
Bringing It All Together
Effective storytelling in Java applications brings together a rich tapestry of characters, conflicts, settings, and more. When skillfully woven, these elements create a compelling user journey that keeps users engaged. By focusing on character development and user experience, applying narrative-driven design principles, and leveraging Java’s robust libraries, you can build applications that not only fulfill functional requirements but also tell a story worth experiencing.
In the end, engaging users through storytelling is not just about coding; it's about creating an environment where the user feels valued, understood, and intrinsically motivated to continue their journey through your application.
By mastering these elements, your Java applications can serve as powerful narratives that leave lasting impressions.
Checkout our other articles