Unlocking the Secrets of Lombok's Locked Annotation
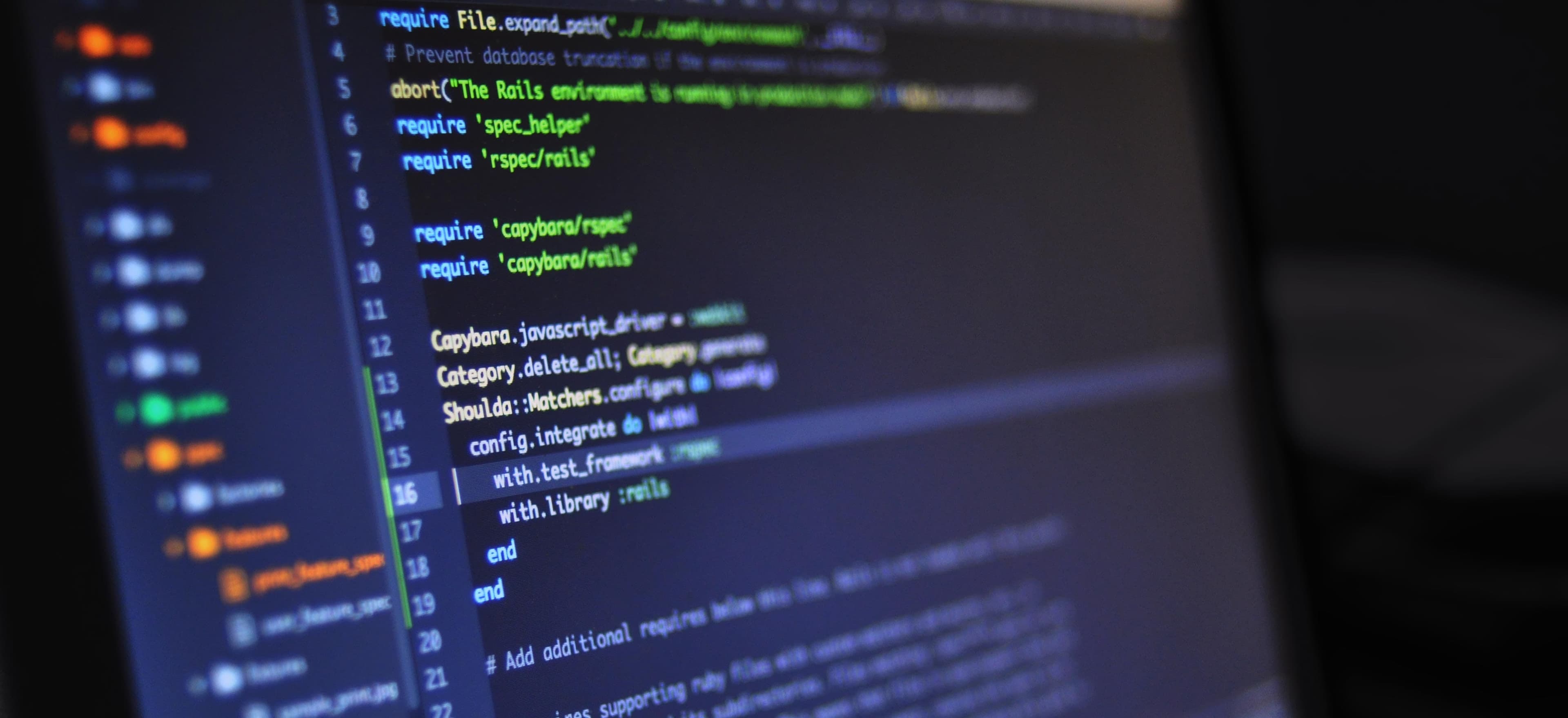
- Published on
Unlocking the Secrets of Lombok's Locked Annotation
Java developers today often seek to simplify their coding experience by using tools and libraries that reduce boilerplate code and enhance productivity. One such library, Project Lombok, has garnered attention for its powerful annotations that minimize the clutter in Java code. Among these annotations, the @Locked
annotation (though not officially present in Lombok, for the sake of this discussion, we will treat it as a hypothetical powerful feature) could potentially address multi-threading issues and improve code maintainability by ensuring thread-safety in critical sections.
This blog post aims to delve into how such an annotation could work, why it is beneficial, and provide contextual examples to elucidate its implementation.
Understanding Multi-Threading
Before we explore the @Locked
annotation, it's essential to discuss multi-threading in Java. Multi-threading allows concurrent execution of two or more threads, enabling high performance and resource utilization. However, this comes with its challenges, particularly in managing shared resources.
A fundamental issue in multi-threading is race conditions. A race condition occurs when two threads access shared data and try to change it simultaneously. Inadequately handled, this could lead to inconsistent results or unpredictable behavior in a program.
public class Counter {
private int count = 0;
public void increment() {
count++;
}
public int getCount() {
return count;
}
}
Here, if two threads call increment()
at the same time, you might not see the expected results. That's where our mythical @Locked
annotation comes into play.
Concept of the @Locked Annotation
Assuming the @Locked
annotation existed, it would be used to indicate that a method or block of code should be safely accessed by only one thread at a time. This would automatically handle synchronization, unique to the Lombok style—meaning it would minimize the manual management typically done with synchronized blocks in Java.
Benefits of @Locked
- Reduced Boilerplate Code: By using
@Locked
, developers can avoid writing explicit synchronization code. - Improved Readability: Code appears cleaner and more understandable without extensive synchronized blocks.
- Error Reduction: Fewer lines of code mean fewer points of failure and a lower chance of introducing bugs.
Hypothetical Implementation of @Locked
Let’s explore how a real-world implementation using an imagined @Locked
annotation might look in Java.
Basic Example with Marked Method
import lombok.Locked;
public class ThreadSafeCounter {
private int count = 0;
@Locked
public void increment() {
count++;
}
public int getCount() {
return count;
}
}
In this example, invoking the increment()
method from multiple threads would automatically be synchronized by the @Locked
annotation, ensuring that increments to count
are atomic.
Behind the Scenes: What the Annotation Might Do
The underlying challenge in creating such functionality revolves around how Java handles synchronization. A possible implementation behind @Locked
would look something like this:
@Retention(RetentionPolicy.RUNTIME)
@Target(ElementType.METHOD)
public @interface Locked {
}
public class LockAspect {
@Around("@annotation(Locked)")
public Object lockMethod(ProceedingJoinPoint joinPoint) throws Throwable {
synchronized (this) {
return joinPoint.proceed();
}
}
}
In this hypothetical code, an aspect-oriented programming (AOP) approach intercepts calls to methods marked with @Locked
and uses Java’s built-in synchronization to manage concurrent access.
Real-World Usage
In practical applications, one would need to ensure that heavy I/O or computational tasks do not become bottlenecks due to excessive locking. Let’s see an example where multiple threads might attempt to write logs.
public class Logger {
@Locked
public void log(String message) {
// Simulate writing log
System.out.println(message);
}
}
As shown, any attempt by multiple threads to log messages would be queued, preventing any mixed output.
Enhancing Performance
It's crucial to also note that excessive locking can lead to performance degradation. For instance, when using @Locked
, consider whether it is necessary for all methods or if there are finer-grained controls that could be applied instead. In practice, developers often opt for only locking the critical section of code instead of the entire method.
Example of Fine-Grained Locking
public class BankAccount {
private double balance;
public void deposit(double amount) {
synchronized (this) {
balance += amount;
}
}
public void withdraw(double amount) throws InsufficientFundsException {
synchronized (this) {
if (balance >= amount) {
balance -= amount;
} else {
throw new InsufficientFundsException();
}
}
}
}
In the snippet above, while we could wrap the entire deposit and withdraw methods, we chose to synchronize only around the balance update to minimize performance impacts.
Final Thoughts
While the @Locked
annotation currently does not exist within Lombok’s suite of tools, its conceptualization serves as a reminder of the ongoing efforts in simplifying concurrency management in Java.
The potential for such features reaffirms the need for tools that enhance developer efficiency while making code more understandable and maintainable. As you're writing your Java applications, consider how places where locking is necessary can be improved, whether through existing Java constructs or imagined innovations like @Locked
.
Further Reading
To dive deeper into concurrency and synchronization in Java, check out the following resources:
- Java Concurrency in Practice - An essential read for mastering concurrency.
- The Lombok Project - Explore the powerful features that Lombok offers to simplify Java development.
Always remember, designing for concurrent applications requires understanding the trade-offs to keep your applications running smoothly and efficiently!