Understanding CAS: Why Race Conditions Ruin Your Code
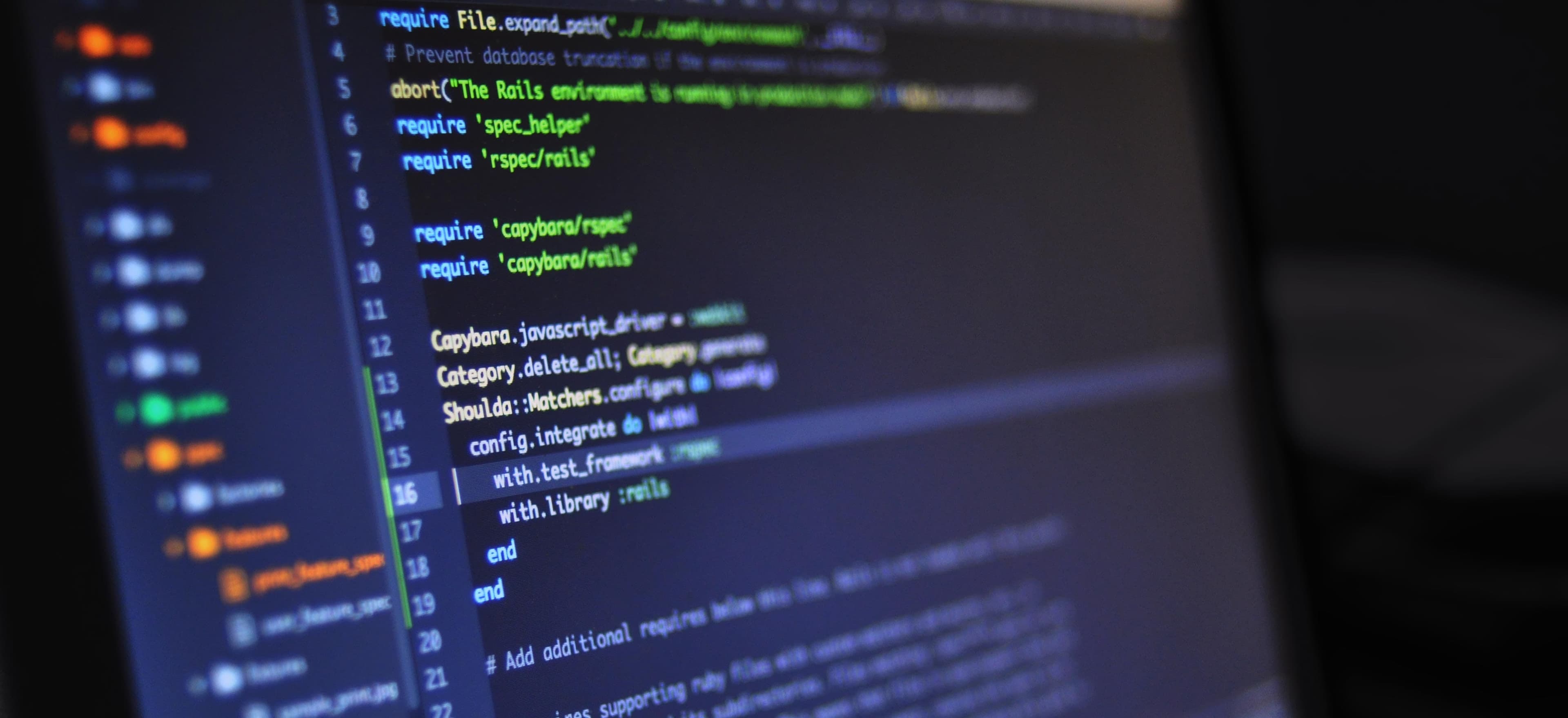
- Published on
Understanding CAS: Why Race Conditions Ruin Your Code
Concurrency is a powerful feature of modern programming, offering the ability to execute multiple sequences of operations simultaneously. However, along with this power comes the responsibility to manage complexity. One of the most pernicious issues in concurrent programming is the race condition, a scenario where two or more threads access shared data and try to change it at the same time. In this post, we'll explore the concept of Compare-And-Swap (CAS), its role in preventing race conditions, and why understanding these concepts is critical for writing robust code.
What is a Race Condition?
A race condition occurs when the outcome of a program depends on the timing of uncontrollable events, such as the scheduling of threads by the operating system. In simpler terms, when multiple threads attempt to access shared resources simultaneously, it can lead to unpredictable behavior.
For example, consider a banking application where two threads try to withdraw money from the same account simultaneously:
public class BankAccount {
private int balance;
public BankAccount(int initialBalance) {
this.balance = initialBalance;
}
public synchronized void withdraw(int amount) {
if (balance >= amount) {
balance -= amount;
}
}
public int getBalance() {
return balance;
}
}
Without synchronization, if two threads call the withdraw
method at the same time, it is possible that both could check the balance before either finishes the withdrawal. This could result in both threads allowing a withdrawal that exceeds the current balance, leading to an inconsistent state.
The Role of Compare-And-Swap (CAS)
One of the most efficient ways to handle concurrency and mitigate race conditions is through the use of CAS. CAS is a low-level atomic operation that reads a value from memory, compares it to a known value, and, only if they are the same, updates the memory with a new value.
The general form looks like this:
boolean compareAndSwap(int[] array, int index, int expectedValue, int newValue);
This operation is atomic, meaning that no other threads can alter the value in between the read and the write. The CAS operation returns true
if the swap was successful, and false
otherwise. This means the program can decide to retry the operation or handle the failure as necessary.
Example of CAS
Let's illustrate this with a CAS version of the banking example:
import java.util.concurrent.atomic.AtomicInteger;
public class AtomicBankAccount {
private AtomicInteger balance;
public AtomicBankAccount(int initialBalance) {
this.balance = new AtomicInteger(initialBalance);
}
public boolean withdraw(int amount) {
int currentBalance;
int newBalance;
do {
currentBalance = balance.get();
if (currentBalance < amount) {
return false; // Not enough funds
}
newBalance = currentBalance - amount;
} while (!balance.compareAndSet(currentBalance, newBalance));
return true; // Withdrawal successful
}
public int getBalance() {
return balance.get();
}
}
Explanation of the Code
-
AtomicInteger: This class is part of the
java.util.concurrent.atomic
package, which provides an integer value that may be updated atomically. This ensures thread-safe operations without the use of synchronized blocks. -
compareAndSet(): This method takes two parameters: the expected current value and the new value. It atomically sets the value to the new value if the current value equals the expected current value. It returns a boolean indicating success.
The loop in the withdraw
method repeatedly attempts the withdrawal until it can successfully update the balance.
Why Use CAS Instead of Locks?
CAS offers several advantages over traditional locking mechanisms:
-
Performance: CAS operations are generally faster than acquiring and releasing locks, reducing overhead and context-switching in the operating system.
-
Deadlock Prevention: Since CAS does not require locks, it eliminates the possibility of deadlocks—situations where two or more threads are blocked forever because each thread is waiting for the other to release a lock.
-
Simplicity: The operations are simple to understand and implement. You can easily reason about the state of your variables without worrying about lock contention.
However, ambitious use of CAS can lead to its own problems, like livelihood—a scenario where threads are constantly retrying without success. Thus, while CAS is powerful, it's not a panacea.
The Importance of Understanding CAS and Race Conditions
While CAS provides a means to handle concurrency more effectively, understanding race conditions will lead you to design better, more reliable software. Knowing when to apply CAS patterns can save you from potential pitfalls and guarantee greater application stability.
If you want to dive deeper into concurrency in Java, I recommend checking out the book Java Concurrency in Practice by Brian Goetz, which provides a thorough treatment of the subject, including best practices.
Additionally, the Java documentation on Concurrent Utilities is an invaluable resource for understanding and using Java's concurrency tools effectively.
Bringing It All Together
Race conditions can easily wreak havoc on your applications, leading to inconsistent data and unpredictable behavior. By understanding CAS and applying atomic operations, you can prevent these race conditions and develop high-performing, concurrent programs.
Concurrency is a double-edged sword; with great power comes great responsibility. Mastering CAS is your first step towards conquering the complexities of multithreaded programming.