Mastering Spring REST Exception Handling: Common Pitfalls
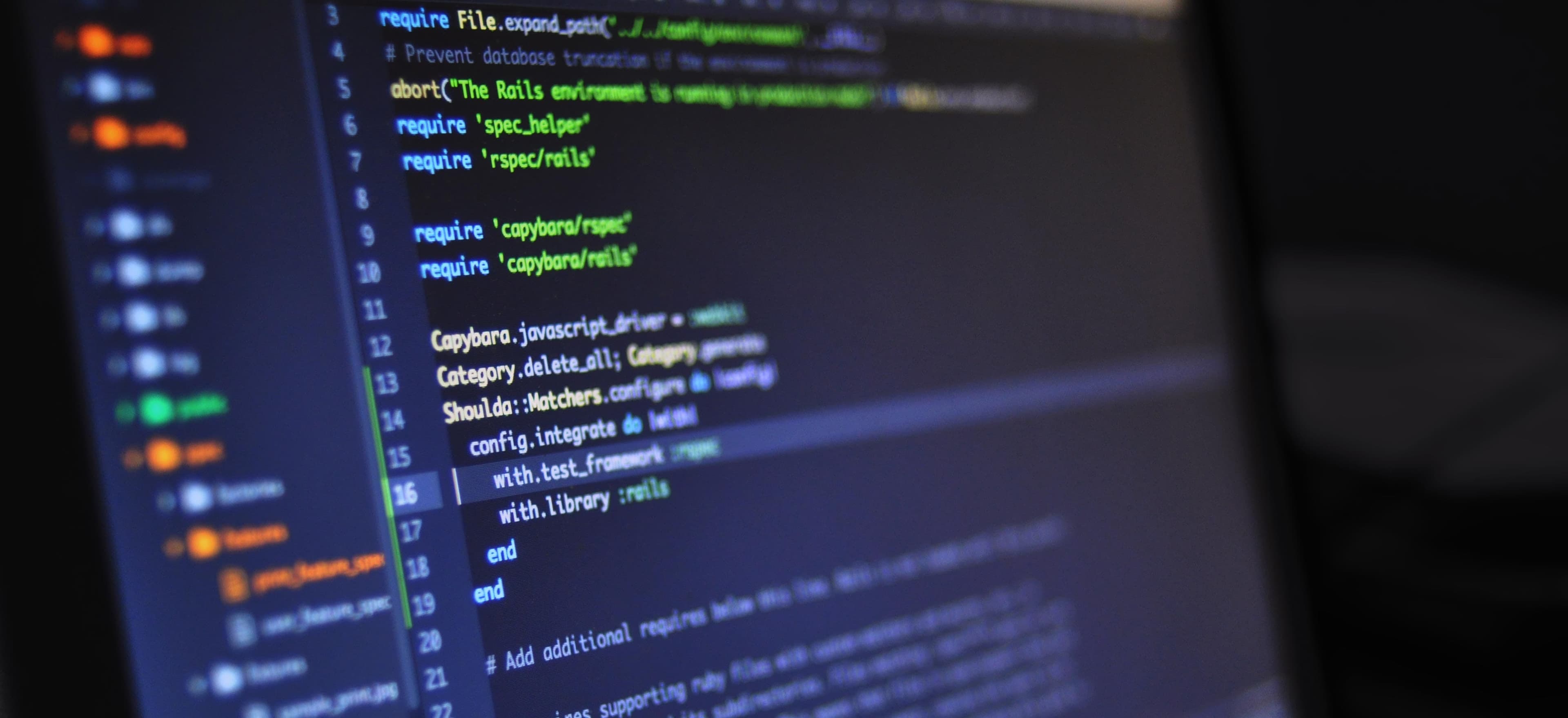
- Published on
Mastering Spring REST Exception Handling: Common Pitfalls
Developing RESTful web services with Spring can be quite rewarding, but it often comes with its own set of challenges. One of the key components that serves to enhance API reliability and user experience is exception handling. Properly managing exceptions can distinguish a robust application from one that is prone to failures. This blog post will discuss common pitfalls in Spring REST exception handling while providing practical examples to elevate your understanding.
What is Exception Handling?
Exception handling is a programming construct that interrupts your flow when an unexpected situation arises. In the context of a REST API, poor exception handling can lead to poor user experience or data integrity issues. In Spring, this can be achieved using various techniques, including @ControllerAdvice
, @ResponseStatus
, and @ExceptionHandler
annotations.
The Importance of Exception Handling
The overarching goal of exception handling in a REST context is to:
- Provide meaningful error messages: Users should not be faced with vague exceptions.
- Ensure consistent responses: Regardless of the error type, clients should receive uniform error response structures.
- Prevent application crashes: Unhandled exceptions often lead to application failure.
Let's dig into common pitfalls you might encounter in Spring REST exception handling and how to avoid them.
Pitfall 1: Generic Exception Handling
What is it?
Using very generic exception catch blocks can muddy the waters. Catching all exceptions with a single handler (e.g., catching Exception.class
) may make debugging more challenging.
Solution: Specificity is Key
It's crucial to define specific handlers that catch only the exceptions you expect and can guide users through troubleshooting. This can improve both the clarity of error messages and maintainability.
@ControllerAdvice
public class GlobalExceptionHandler {
@ExceptionHandler(ResourceNotFoundException.class)
public ResponseEntity<ErrorResponse> handleResourceNotFound(ResourceNotFoundException ex) {
ErrorResponse errorResponse = new ErrorResponse(HttpStatus.NOT_FOUND, ex.getMessage());
return new ResponseEntity<>(errorResponse, HttpStatus.NOT_FOUND);
}
@ExceptionHandler(DataIntegrityViolationException.class)
public ResponseEntity<ErrorResponse> handleDataIntegrityViolation(DataIntegrityViolationException ex) {
ErrorResponse errorResponse = new ErrorResponse(HttpStatus.CONFLICT, ex.getMessage());
return new ResponseEntity<>(errorResponse, HttpStatus.CONFLICT);
}
}
Commentary
By defining specific exception handlers, we ensure that certain exceptions are processed in a targeted manner. Each type of exception returns a custom error response encapsulated in an ErrorResponse
object, providing clarity and context to the client.
Pitfall 2: Failing to Log Exceptions
What is it?
Many developers overlook logging exceptions, especially when implementing an exception handling strategy. While a good response to the client is important, the developer needs insights as well.
Solution: Log Important Exceptions
Make sure to log exceptions at appropriate levels. Use a logging framework such as SLF4J or Log4J for this purpose.
@ExceptionHandler(ResourceNotFoundException.class)
public ResponseEntity<ErrorResponse> handleResourceNotFound(ResourceNotFoundException ex) {
log.error("Resource not found: {}", ex.getMessage()); // Log the exception message
ErrorResponse errorResponse = new ErrorResponse(HttpStatus.NOT_FOUND, ex.getMessage());
return new ResponseEntity<>(errorResponse, HttpStatus.NOT_FOUND);
}
Commentary
Adding logging allows developers to keep track of issues that occur in the API, making it easier to diagnose problems and improve the service's robustness.
Pitfall 3: Not Separating Concerns
What is it?
Mixing the business logic with exception handling leads to code that is difficult to read and maintain. This makes the API fragile and vulnerable to breaking changes.
Solution: Modularize Your Exception Handling
By creating separate classes for your error handling and business logic, you maintain a clear separation of concerns.
public class ErrorResponse {
private HttpStatus status;
private String message;
public ErrorResponse(HttpStatus status, String message) {
this.status = status;
this.message = message;
}
// Getters and Setters
}
Commentary
This ErrorResponse
class serves to neatly encapsulate the response we want to send back to the client. Decoupling the handling and the business logic will make your codebase cleaner and easier to navigate.
Pitfall 4: Ignoring Response Format
What is it?
Inconsistent response formats can frustrate API clients, making it hard to manage errors programmatically.
Solution: Standardize Your Error Responses
Follow a consistent format for all error responses. Here is an example:
{
"status": "NOT_FOUND",
"message": "Resource not found"
}
Implementation
Ensure that the ErrorResponse
class (used in previous examples) adheres to this standardized format. This allows clients to efficiently parse the error messages.
Pitfall 5: Not Testing Your Error Handlers
What is it?
If you do not test your exception handling strategies, you leave your application vulnerable to issues that can arise under load or unexpected usage scenarios.
Solution: Write Unit Tests
Utilize JUnit alongside Spring's testing features to write comprehensive tests for your exception handlers.
@RunWith(SpringRunner.class)
@WebMvcTest(GlobalExceptionHandler.class)
public class GlobalExceptionHandlerTest {
@Autowired
private MockMvc mockMvc;
@Test
public void testHandleResourceNotFound() throws Exception {
mockMvc.perform(get("/api/resource/1"))
.andExpect(status().isNotFound())
.andExpect(jsonPath("$.message").value("Resource not found"));
}
}
Commentary
By writing unit tests, you ensure that your exception handling behaves as expected under various conditions. This mitigates future issues and builds confidence in your error management strategies.
Wrapping Up
Mastering exception handling in Spring REST services can enhance user experience, improve maintainability, and prevent unexpected application failures. By avoiding common pitfalls like generic exception handling, neglecting logging, and not standardizing responses, you can create robust APIs that provide valuable feedback to users and easy insights for developers.
For further reading on Spring's exception management, check out the official Spring documentation and the Spring REST guide.
With the pitfalls laid out and solutions provided, you are well on your way to designing a better, more reliable REST API! Happy coding!
Checkout our other articles