Tackling Communication Challenges in Microservices Architectures
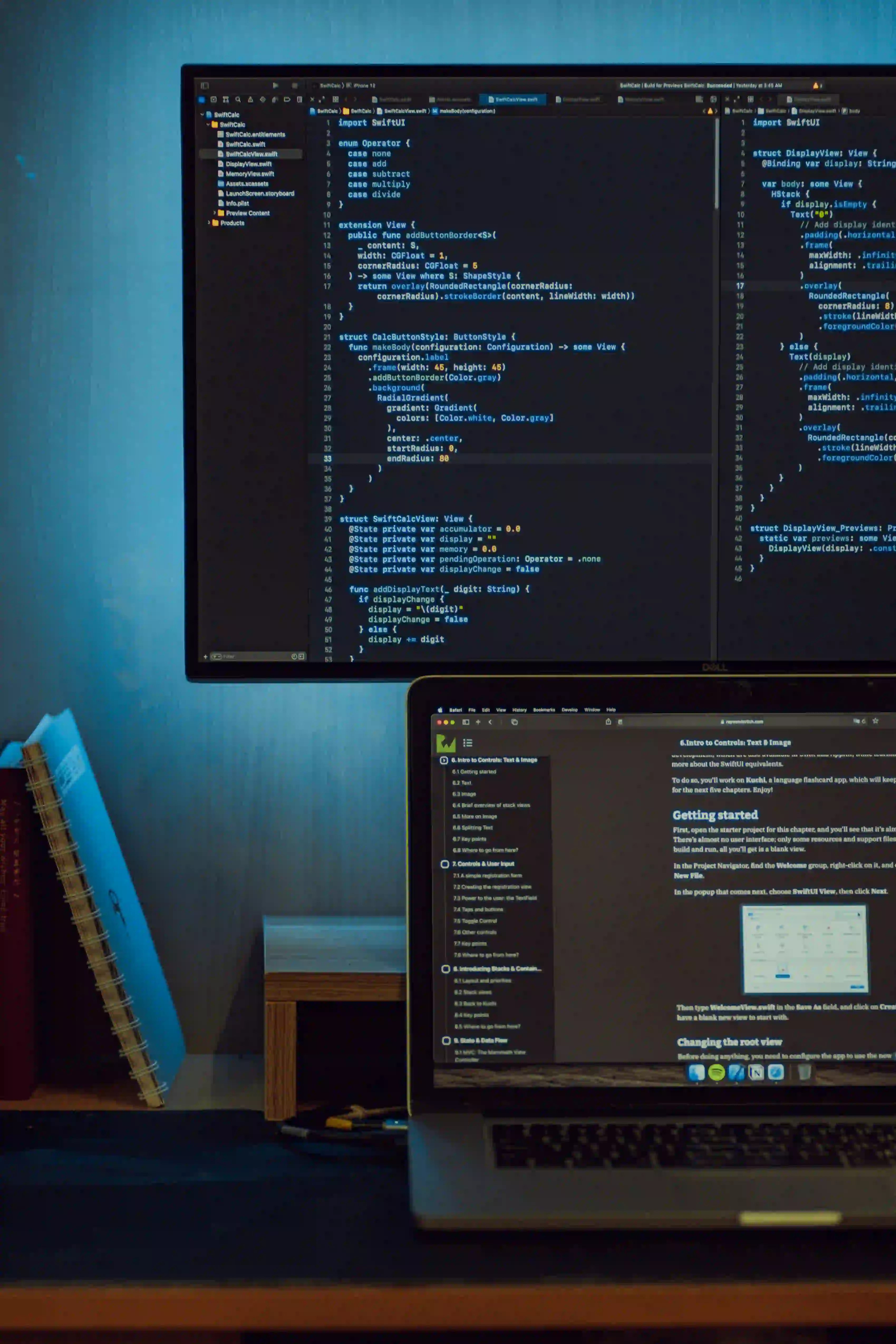
Tackling Communication Challenges in Microservices Architectures
Microservices architecture has become a popular approach for developing large-scale applications. Its advantages include increased flexibility, independent deployments, and improved scalability. However, as organizations transition to microservices, they often encounter communication challenges that can hinder the effectiveness of their system. This blog post will explore these communication challenges and provide strategies to tackle them, ensuring your microservices architecture thrives.
Understanding Microservices Communication
In a microservices architecture, multiple services work together to fulfill a business requirement. This decentralization introduces a variety of communication methods that can be crucial both for coordination and for ensuring data consistency and latency management. Given that services are typically distributed over a network, the manner in which they communicate can significantly affect performance.
Common Communication Protocols in Microservices
- REST (Representational State Transfer): Leveraging HTTP for communication, REST is widely used due to its simplicity and statelessness. It is ideal for CRUD operations.
- gRPC: This high-performance RPC framework, developed by Google, uses HTTP/2 and protobuf for serialization. It's excellent for communication between microservices, especially when performance is a concern.
- Message Brokers (e.g., RabbitMQ, Kafka): These allow asynchronous communication by sending messages between services. They decouple service interactions and facilitate better scalability.
The Communication Challenges
- Network Latency: Communication across the network introduces latency that can slow down service response times.
- Service Discovery: In a dynamic microservices environment, where services can scale up or down, locating the right service can become complex.
- Data Consistency: Maintaining consistency across various microservices that often have their own databases can be tricky.
- Error Handling: Failures in communication can occur, necessitating robust error handling strategies.
- Protocol Overhead: The choice of communication protocol can lead to overhead issues that might slightly impact performance.
Strategies for Overcoming Communication Challenges
1. Use API Gateways
An API Gateway acts as a single entry point for microservices, managing requests and directing them to the appropriate services. This setup can help streamline communication and minimize client-side complexity.
@RestController
@RequestMapping("/api/v1/")
public class APIController {
@Autowired
private UserService userService;
@GetMapping("users/{id}")
public UserDto getUser(@PathVariable Long id) {
return userService.getUserById(id);
}
// Other API endpoints...
}
The API Gateway pattern can reduce direct client-to-service calls, thus improving security and reducing workload on individual services.
2. Implement Service Discovery
Service discovery is essential for allowing services to locate each other dynamically. Tools like Netflix Eureka or Consul can help in this regard.
@EnableEurekaClient
@SpringBootApplication
public class Application {
public static void main(String[] args) {
SpringApplication.run(Application.class, args);
}
}
By annotating your application with @EnableEurekaClient
, it will register automatically with the Eureka server, allowing other services to discover it dynamically.
3. Leverage Asynchronous Communication
Instead of tightly coupling services with synchronous communication, consider using asynchronous messaging patterns. This can improve responsiveness and decouple service dependencies.
@Service
public class OrderService {
@Autowired
private RabbitTemplate rabbitTemplate;
public void createOrder(Order order) {
// Process order
rabbitTemplate.convertAndSend("orderQueue", order);
}
}
In this example, the OrderService
sends a message to a message broker. This allows for non-blocking operations, enabling better scaling and performance.
4. Ensure Data Consistency with Patterns
Use distributed transaction patterns such as Saga or Event Sourcing. These patterns help maintain data consistency without tightly coupling your services.
Example: Saga Pattern
public class OrderSaga {
// Steps in the saga
public void handleOrderCreation(Order order) {
// Step 1: Create order
// Step 2: Reserve inventory
// Step 3: Process payment
// Implement compensatory transactions for each step
}
}
By handling transactions in a series of steps and ensuring compensatory actions are in place, the saga pattern addresses the challenges of distributed data consistency.
5. Implement Robust Error Handling
Implement retries and circuit-breaker patterns to manage transient failures effectively. For instance, using Resilience4j can help in setting up circuit breakers.
@RestController
public class UserController {
@Autowired
private UserService userService;
@CircuitBreaker
public User getUser(Long id) {
return userService.fetchUserFromRemoteService(id);
}
}
A circuit-breaker protects services from failure cascades, enhancing resilience and reliability in communication.
The Closing Argument
While the transition to microservices architecture comes with its share of communication challenges, it also opens doors to immense flexibility and scalability in application development. By leveraging strategies like API gateways, service discovery, asynchronous communication, and robust error handling, organizations can navigate these challenges effectively.
As you advance in implementing microservices, remember that the choice of communication method should align with your organizational goals and technical capabilities. Regularly review your architecture to ensure it adapts to changes in business requirements and technological advancements.
For more resources on microservices best practices, check out the Microservices Patterns book and Spring Cloud documentation.
By addressing these challenges head-on, you not only enhance the efficiency of individual services but also ensure that your microservices ecosystem operates harmoniously as a whole. Happy coding!