Mastering Backpressure in Project Reactor: Common Pitfalls
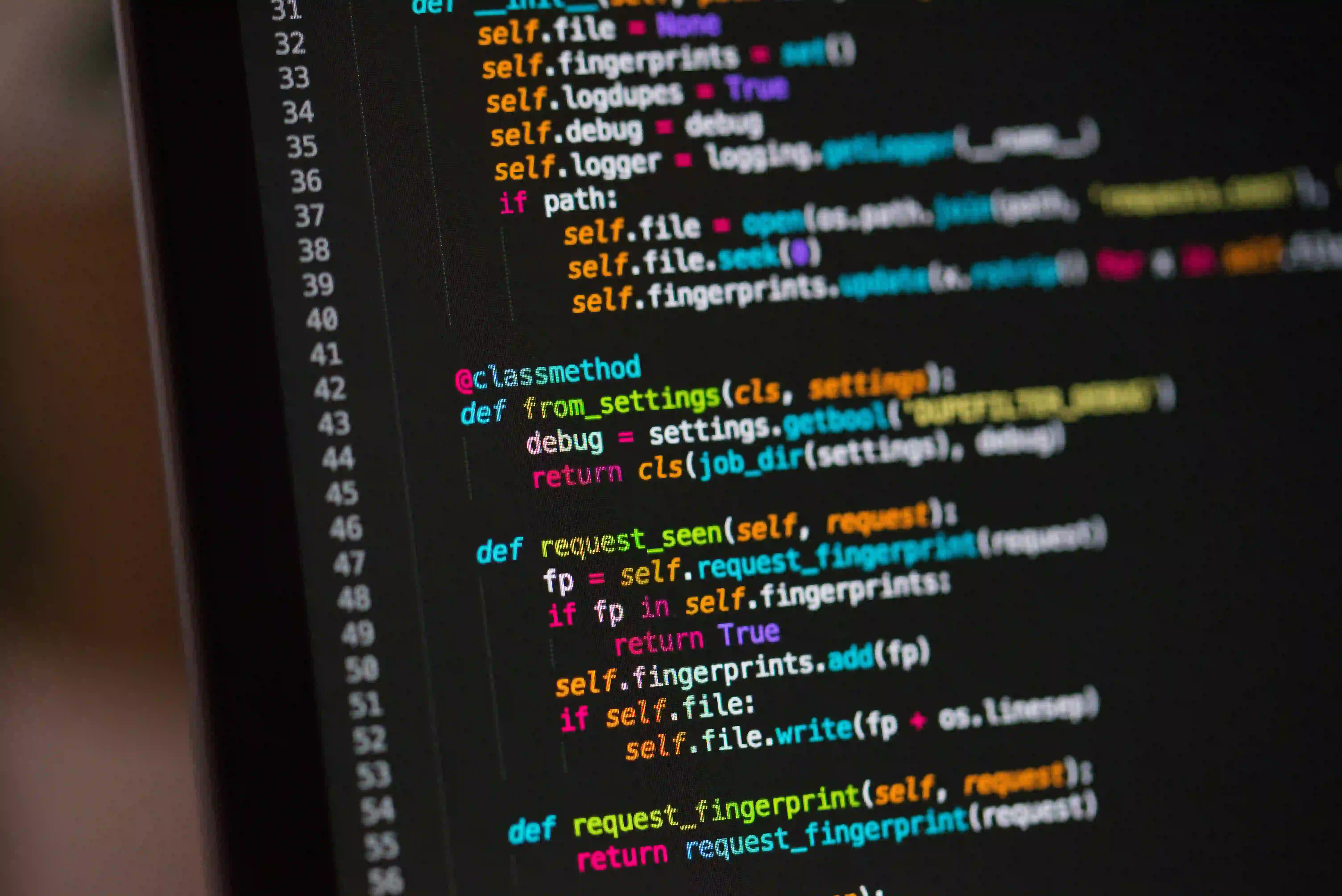
Mastering Backpressure in Project Reactor: Common Pitfalls
Backpressure is a crucial concept in reactive programming, particularly when dealing with data flows that exceed the consumer's processing capacity. In the Java ecosystem, Project Reactor offers powerful tools to handle backpressure. However, mastering backpressure involves navigating common pitfalls. This blog post will guide you through understanding backpressure in the context of Project Reactor, discussing its mechanisms, and highlighting frequent mistakes developers make.
What is Backpressure?
Backpressure refers to a strategy for dealing with the situation when a producer generates data faster than a consumer can process it. Without handling this efficiently, you risk overwhelming your application, resulting in crashes or degraded performance.
Why is Backpressure Important?
- Resource Management: Proper handling prevents resource exhaustion and retains system stability.
- Data Integrity: Ensures that no data is lost while maintaining throughput.
- User Experience: Asynchronous processes improve responsiveness and the overall experience for users.
Project Reactor's Backpressure Mechanism
Project Reactor employs the Reactive Streams specification, which provides robust mechanisms for backpressure. The core idea revolves around a Publisher
and a Subscriber
. The publisher emits data to the subscriber, which can control the rate of this emission through a request mechanism.
Basic Components:
- Publisher: The source of data, emits items.
- Subscriber: The sink of data, processes the received items.
- Subscription: Links the publisher to the subscriber.
Here’s a simple code example illustrating the relationship between these components.
import reactor.core.publisher.Flux;
public class BackpressureExample {
public static void main(String[] args) {
Flux<Integer> flux = Flux.range(1, 10) // Creating a range of integers.
.log() // Enables logging for debugging.
.limitRate(2); // This is a simple backpressure strategy.
flux.subscribe(
item -> {
try {
Thread.sleep(100); // Simulate a time-consuming task.
} catch (InterruptedException e) {
Thread.currentThread().interrupt();
}
System.out.println(item);
}
);
}
}
Explanation of the Code:
Flux.range(1, 10)
: Creates a stream of numbers from 1 to 10..log()
: Adds logging to monitor the flow of data..limitRate(2)
: This limits the number of items the subscriber can request, effectively implementing backpressure.
This simple setup ensures that the subscriber only processes a maximum of two items at a time, preventing overload.
Common Pitfalls
While Project Reactor provides tools to manage backpressure, there are numerous pitfalls developers can encounter.
1. Ignoring Backpressure
Problem:
Many developers neglect implementing backpressure entirely, especially in cases with minimal data streams. This is dangerous as it can lead to potential memory issues or API overload.
Solution:
Always consider the need for backpressure, even in small applications. The limitRate()
function mentioned above can help by controlling the flow right from the start.
2. Improperly Configuring limitRate()
Problem:
Another common mistake is not understanding how to configure limitRate()
. Setting an excessively high rate can lead to overloading the consumer, whereas setting it too low can hamper throughput.
Solution:
Analyze your application's processing capability and adjust the rate accordingly. Monitor the application's performance and tweak these values.
3. Overusing Schedulers
Problem:
Schedulers allow you to control threading; however, overusing them, or changing them without understanding can lead to unpredictable behavior and inefficient resource utilization.
Solution:
Use appropriate schedulers based on your application's needs. For example, use Schedulers.parallel()
for CPU-bound tasks and Schedulers.immediate()
for asynchronous tasks.
4. Not Handling Exceptions
Problem:
Failing to handle exceptions in a reactive stream can lead to unexpected application behavior and errors that aren't evident until runtime.
Solution:
Always include error handling in your stream. You can use doOnError()
or onErrorResume()
to catch exceptions effectively.
Here’s an example that shows how to handle errors:
Flux<Integer> flux = Flux.range(1, 10)
.map(i -> {
if (i == 5) throw new RuntimeException("An error occurred!"); // Simulating an error.
return i;
})
.doOnError(e -> System.err.println(e.getMessage())) // Handling error.
.onErrorResume(e -> Flux.just(0)); // Fallback to an alternative flux.
flux.subscribe(System.out::println);
5. Not Using onBackpressureBuffer
Problem:
Using backpressure strategies without the appropriate buffer can lead to dropped elements if the consumer is not fast enough.
Solution:
Employ onBackpressureBuffer()
. It buffers elements when the downstream cannot keep up. However, be careful: excessive buffering can lead to memory issues.
Flux<Integer> source = Flux.range(1, 1000)
.onBackpressureBuffer(100, dropped -> System.out.println("Dropped: " + dropped))
.subscribe(System.out::println);
6. Mixing Hot and Cold Publishers Incorrectly
Problem:
Understanding the nature of hot and cold publishers is critical. Mixing them improperly can lead to data being lost or received in the wrong order.
Solution:
Evaluate whether your source of data should be hot or cold, based on your application's behavior. Hot publishers broadcast data to all subscribers, while cold publishers create independent sequences.
Final Thoughts
Mastering backpressure in Project Reactor requires a balanced approach. Recognizing and navigating common pitfalls contributes significantly to building stable, efficient applications.
By incorporating backpressure, you not only safeguard your application against potential overloads but also enhance user experience through responsive and efficient data handling. Remember, practice makes perfect. Start implementing these strategies in your projects today!
For further reading on Project Reactor and backpressure, consider visiting the official documentation and exploring more about the Reactive Streams specification. Optimize your reactive programming skills and ensure robust application design!