Spring Security 6.3: Tackling Common Authentication Issues
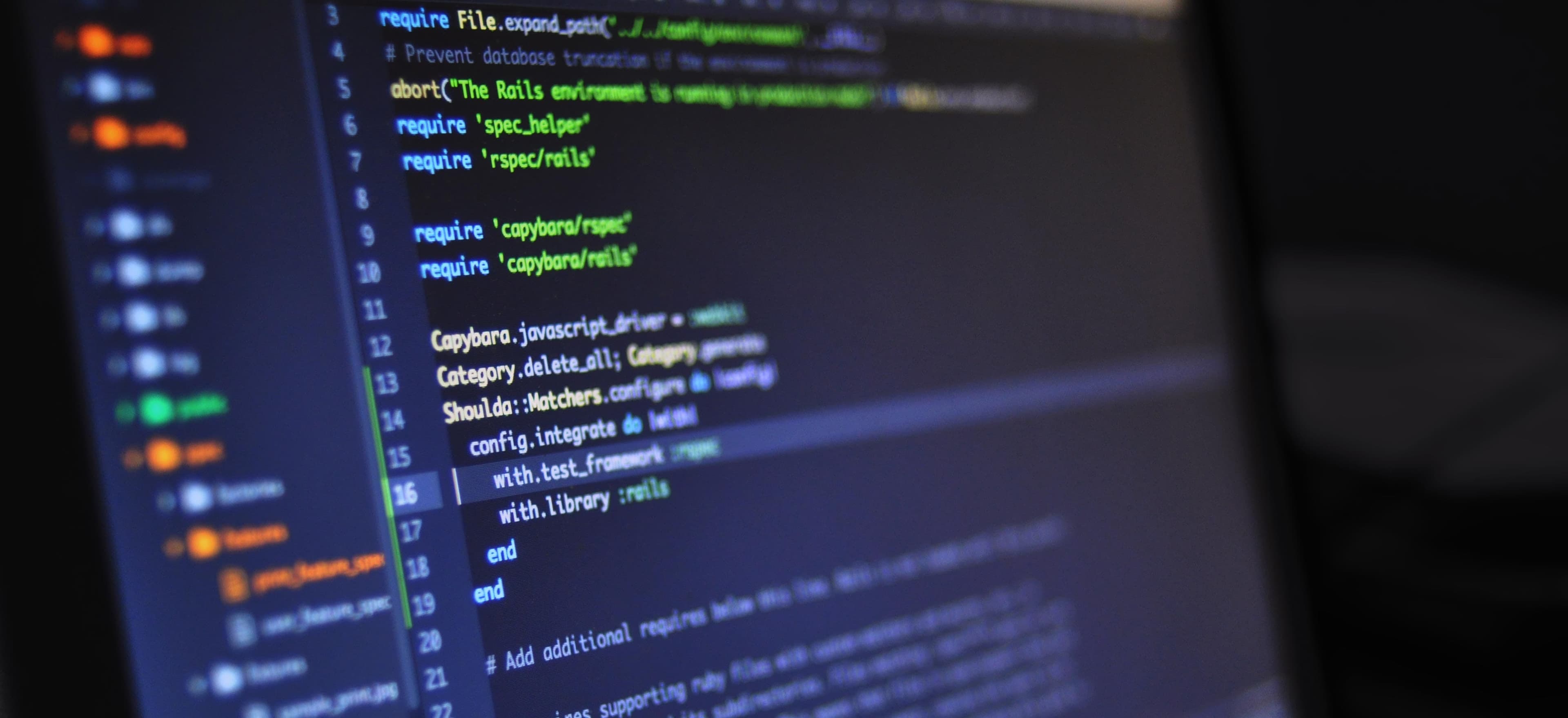
- Published on
Spring Security 6.3: Tackling Common Authentication Issues
As web applications grow in complexity and the demand for robust security measures increases, frameworks like Spring Security continue to evolve. The latest version, Spring Security 6.3, brings improvements that address various authentication challenges developers face. In this blog post, we will discuss common authentication issues and how to efficiently tackle them using the features of Spring Security 6.3.
Understanding Spring Security 6.3
Spring Security is a powerful and customizable authentication and access control framework for Java applications. It provides a comprehensive security solution for both web and enterprise applications. Version 6.3 introduces noteworthy enhancements, focusing on improving usability and security defaults.
Key Features of Spring Security 6.3
- Improved OAuth2 Support: Enhancements for OAuth2 Client and Resource Server, making it easier to integrate third-party authentication.
- Customizable Password Encoders: A more straightforward mechanism for custom password encoders, making it easier to ensure password security.
- Enhanced Exception Handling: Better handling of authentication and access denial exceptions, improving user feedback during errors.
Common Authentication Issues
Before diving into solutions, let's discuss the common authentication issues developers encounter:
- Handling Sessions and Security Contexts
- Password Management and Encoding
- OAuth2 Configuration and Issues
- Fine-Grained Access Control
- Exception Handling during Authentication
Typically, these issues arise during the implementation phase of a Spring application. Let's break them down and explore how Spring Security 6.3 effectively addresses each one.
1. Handling Sessions and Security Contexts
Session management is crucial in ensuring that an authenticated user maintains their state across the web application. Inconsistent session management can lead to vulnerabilities, including session fixation and cross-site request forgery (CSRF).
Solution: Configure Session Management
Spring Security allows you to customize session management easily. Here’s an example:
@EnableWebSecurity
public class SecurityConfig extends WebSecurityConfigurerAdapter {
@Override
protected void configure(HttpSecurity http) throws Exception {
http
.sessionManagement()
.sessionCreationPolicy(SessionCreationPolicy.IF_REQUIRED) // control when sessions are created
.maximumSessions(1) // limit the number of sessions per user
.maxSessionsPreventsLogin(true); // prevents a new session if the limit is reached
}
}
Why This Matters: By enforcing maximum sessions, you help mitigate session-related attacks while ensuring a consistent user experience.
2. Password Management and Encoding
With data breaches a common threat, storing user passwords securely is paramount. Plain-text passwords are a developer's nightmare and can lead to severe data loss.
Solution: Use Strong Password Encoders
Spring Security 6.3 allows for the easy integration of various password encoders to hash passwords safely.
@Bean
public PasswordEncoder passwordEncoder() {
return new BCryptPasswordEncoder(); // utilizing BCrypt for password hashing
}
Why This Matters: BCrypt is a strong hashing algorithm, making it impractical to reverse engineer passwords, ensuring that even if a data breach occurs, sensitive user data remains protected.
3. OAuth2 Configuration and Issues
Integrating OAuth2 can be complex, particularly regarding client registration and token management. Spring Security 6.3 simplifies the OAuth2 configuration.
Solution: Configure OAuth2 Client
To create an OAuth2 client, add necessary dependencies to your pom.xml
:
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-oauth2-client</artifactId>
</dependency>
Then, configure the OAuth2 login in your security configuration.
@Override
protected void configure(HttpSecurity http) throws Exception {
http
.oauth2Login()
.loginPage("/login") // specify custom login page
.defaultSuccessUrl("/home", true); // redirect after successful login
}
Why This Matters: Proper OAuth2 setup simplifies the authentication process and enhances security by leveraging trusted third-party providers.
4. Fine-Grained Access Control
Determining who has access to specific resources is critical. Irrespective of strong authentication, incorrect access control can result in unauthorized access.
Solution: Use Method Security
Spring Security allows you to manage permissions on a method level using annotations.
@PreAuthorize("hasRole('ADMIN')")
public void adminOnlyMethod() {
// Access only for ADMIN role
}
Why This Matters: Fine-grained access control helps prevent unauthorized actions by implementing role-specific checks, securing critical operations within your application.
5. Exception Handling During Authentication
User experience can be affected if errors during authentication are not handled effectively. A generic error message can lead to confusion or lack of feedback.
Solution: Custom Authentication Entry Points
Provide a custom entry point to handle unauthorized access attempts smoothly.
@Bean
public AuthenticationEntryPoint authEntryPoint() {
return (req, res, authException) -> {
res.sendError(HttpServletResponse.SC_UNAUTHORIZED, "Unauthorized");
};
}
Why This Matters: Customized feedback allows users to understand why their access was denied and improves overall user experience.
Closing Remarks: Security First
Spring Security 6.3 comes with significant enhancements that address common authentication issues developers face when building web applications. From session management to OAuth2 integration and exception handling, applying these best practices will help you develop a robust security architecture for your application.
For more robust security strategies, consider exploring additional resources from the Spring Security Documentation. Understanding and implementing these concepts will significantly improve the security posture of your applications.
Remember, security is not a one-time task; it’s an ongoing process. Regularly update your libraries, monitor authentication flows, and stay informed about the latest security trends and vulnerabilities. Happy coding!
References
- Spring Security Official Documentation
- Spring Security GitHub Repository
- BCrypt Password Hashing Algorithm
Feel free to share your experiences with Spring Security 6.3 in the comments below or reach out for further discussions on security practices.
Checkout our other articles