Top 7 Java Tools to Boost Developer Productivity in 2023
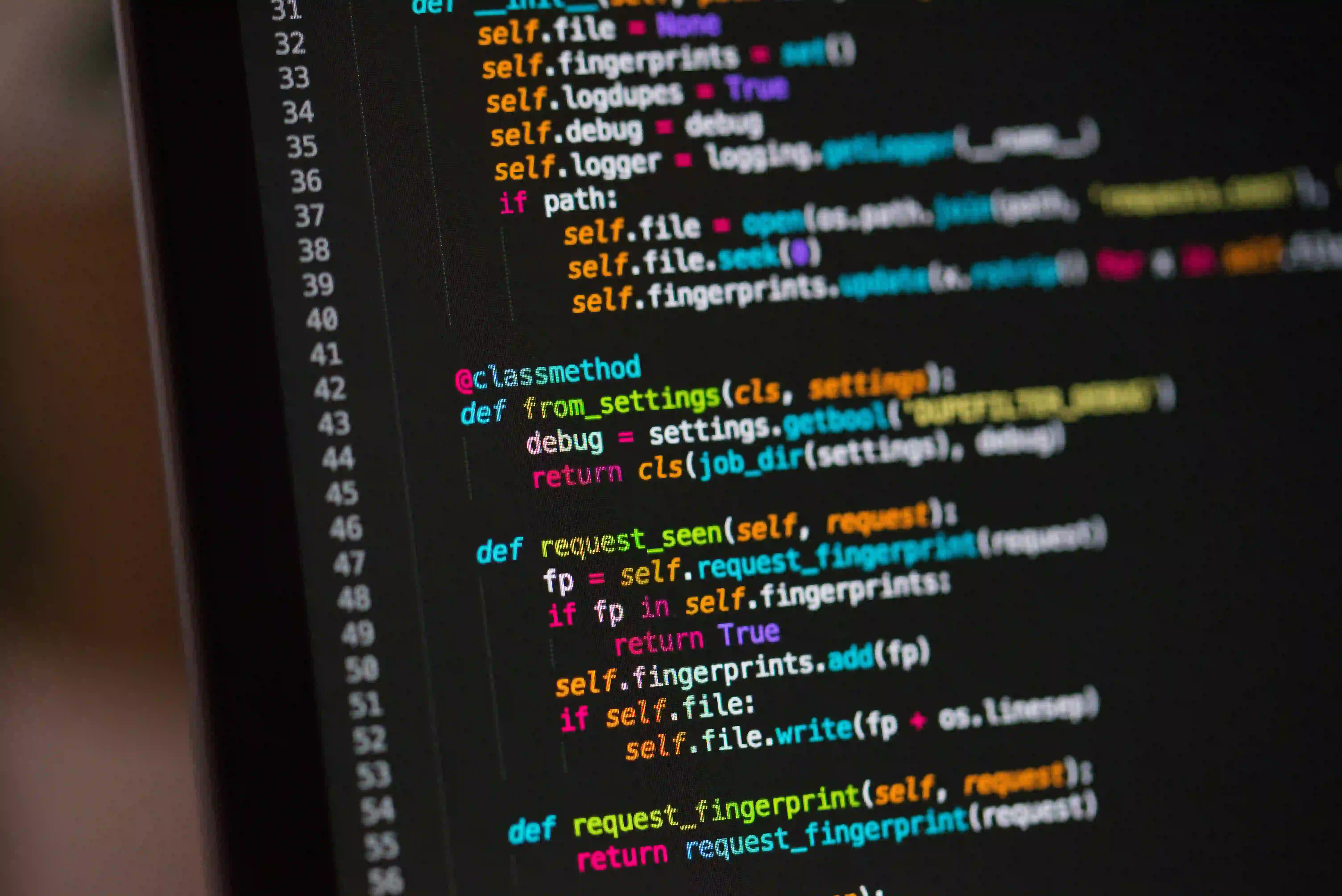
Top 7 Java Tools to Boost Developer Productivity in 2023
Java is one of the most widely used programming languages, and for a good reason. It boasts a robust ecosystem, a vibrant community, and an exhaustive collection of libraries and frameworks. However, the best developers are often those who leverage tools that enhance their productivity. In this blog post, we'll delve into the top 7 Java tools that can help you supercharge your development efforts in 2023.
1. IntelliJ IDEA
JetBrains IntelliJ IDEA is widely regarded as one of the best Integrated Development Environments (IDEs) for Java development.
Why IntelliJ IDEA?
- Smart code completion: It suggests names based on the context, reducing the time spent typing.
- Code analysis: It highlights potential issues in real-time, which fosters a cleaner codebase.
- Built-in version control: Simplifies the process of managing changes with Git, SVN, etc.
Code Snippet Example
Here’s a simple code snippet demonstrating how IntelliJ IDEA helps with code completion:
for (String str : list) {
System.out.println(str);
}
As you type for (
, IntelliJ will suggest String str : list
after identifying the list type, increasing your efficiency.
2. JUnit
JUnit is a unit testing framework for Java that is essential for any serious Java developer.
Why JUnit?
- Test-Driven Development: It supports TDD practices that help ensure your code works as intended before you deploy it.
- Easy assertions: Writing tests becomes straightforward, helping you catch bugs before they become costly problems.
Code Snippet Example
Here's a simple unit test using JUnit:
import org.junit.jupiter.api.Test;
import static org.junit.jupiter.api.Assertions.*;
class CalculatorTest {
@Test
void testAddition() {
Calculator calc = new Calculator();
assertEquals(5, calc.add(2, 3));
}
}
In this example, if you run your tests frequently while developing, JUnit enables you to validate every small change, promoting a reliable codebase.
3. Maven
Maven is a build automation tool used primarily for Java projects.
Why Maven?
- Dependency management: Automatically downloads and manages dependencies for your projects.
- Standardized project structure: Enables a consistent setup that allows developers to quickly understand the layout of any project.
Code Snippet Example
A basic pom.xml
file looks like this:
<project xmlns="http://maven.apache.org/POM/4.0.0"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd">
<modelVersion>4.0.0</modelVersion>
<groupId>com.example</groupId>
<artifactId>my-app</artifactId>
<version>1.0-SNAPSHOT</version>
</project>
Maven’s structure makes it easier for collaborative work, since everyone knows where to find resources.
4. Gradle
Gradle is another powerful build automation tool, which is widely adopted for its flexibility and ease of use.
Why Gradle?
- Performance: It is faster than Maven due to incremental builds.
- Groovy-based DSL: Allows developers to write build scripts in a more natural, declarative language.
Code Snippet Example
Here’s a basic build.gradle
file:
plugins {
id 'java'
}
group 'com.example'
version '1.0-SNAPSHOT'
repositories {
mavenCentral()
}
dependencies {
testImplementation 'junit:junit:4.12'
}
Gradle's scripting capabilities allow for extensive customization and configuration, making it suited for modern-day projects.
5. Lombok
Project Lombok is a Java library that automatically plugs into your editor and builds tools.
Why Lombok?
- Reduce boilerplate code: It generates common methods like getters and setters on compile time.
- Improves readability: Your classes become cleaner and easier to understand.
Code Snippet Example
Instead of manually writing boilerplate code, you can use Lombok like this:
import lombok.Data;
@Data
public class User {
private String name;
private int age;
}
Here, @Data
automatically generates the getters, setters, toString()
, and equals()
methods, simplifying your class structure.
6. Javadoc
Javadoc is the documentation tool for Java that comes integrated with the JDK.
Why Javadoc?
- Built-in: Since it’s part of the Java SDK, no additional setup is required.
- Standardized documentation: Facilitates better understanding across teams, especially in larger projects.
Code Snippet Example
A simple method with Javadoc comments looks like this:
/**
* Adds two integers together.
*
* @param a the first integer
* @param b the second integer
* @return the sum of a and b
*/
public int add(int a, int b) {
return a + b;
}
With Javadoc, you’re not just writing code; you’re creating documentation that other developers can build upon.
7. Docker
Docker is increasingly becoming essential in Java development for containerization.
Why Docker?
- Environment consistency: Ensures applications run the same regardless of where they are hosted.
- Scalability: Makes it easier to scale applications across various environments.
Code Snippet Example
A basic Dockerfile for a Java application:
FROM openjdk:11
VOLUME /tmp
COPY target/myapp.jar myapp.jar
ENTRYPOINT ["java", "-jar", "/myapp.jar"]
With Docker, you can easily ship your application, eliminating "it works on my machine" issues.
Closing Remarks
The right tools can significantly enhance a developer's productivity and the overall quality of your Java applications. By integrating IntelliJ IDEA, JUnit, Maven, Gradle, Lombok, Javadoc, and Docker into your workflow, you can streamline your development process, make fewer mistakes, and maintain cleaner code.
Remember, while these tools are immensely beneficial, the key is to select ones that best cater to your workflow, team size, and project type. As we advance through 2023, staying updated with these tools will not only improve your efficiency but also keep you competitive in the ever-evolving software development landscape.
For more resources, check out Java SE Documentation and Maven Central Repository to dive deeper into these tools!