Streamlining XML Querying in Java: Ditching SAX and DOM
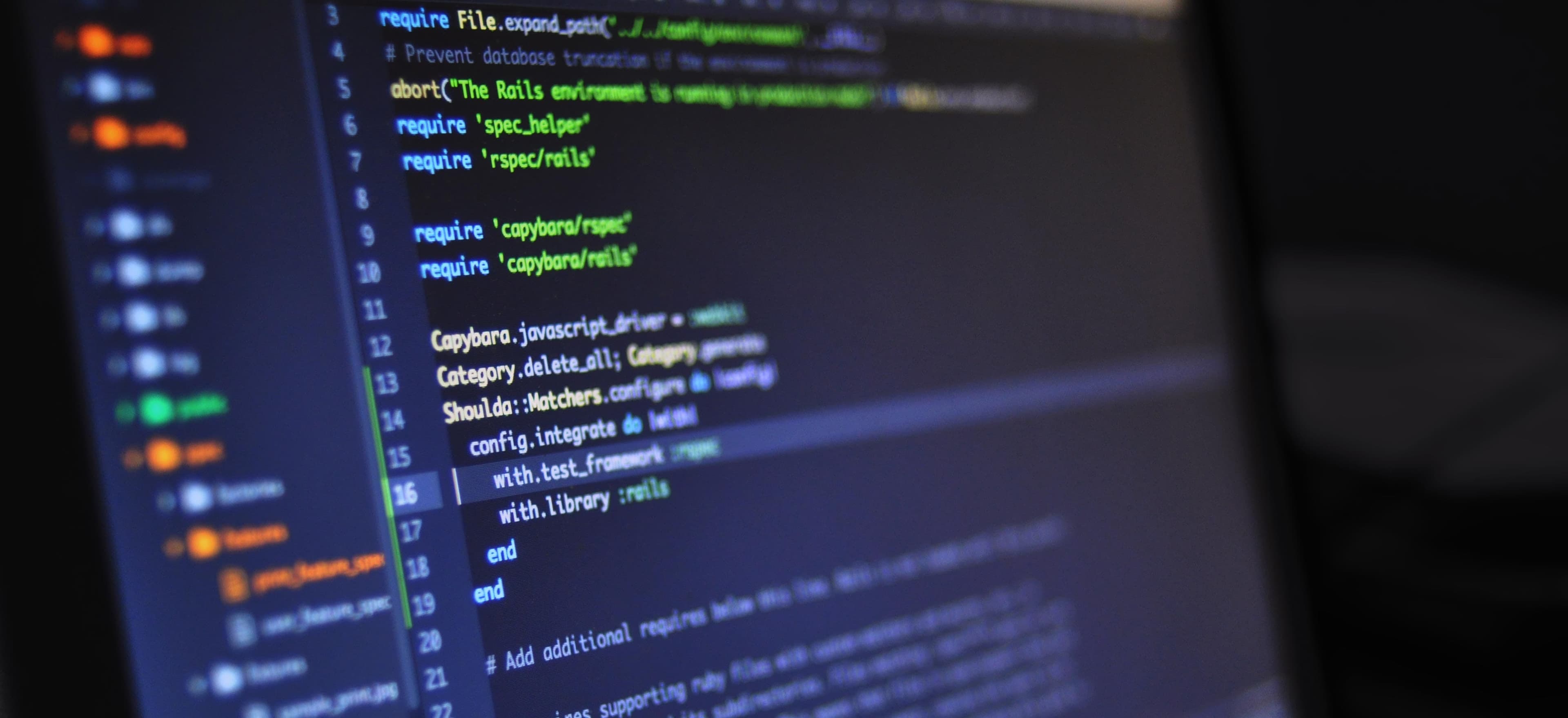
- Published on
Streamlining XML Querying in Java: Ditching SAX and DOM
Handling XML in Java has long been synonymous with two primary approaches: Simple API for XML (SAX) and Document Object Model (DOM). While both have served their purpose, they can often lead to performance bottlenecks and increased complexity in application design. In this blog post, we'll explore more modern alternatives for querying XML in Java. We’ll take a closer look at the Streaming API for XML (StAX) and XPath, showing you how to streamline your XML querying process.
Table of Contents
- Introduction to XML in Java
- The Drawbacks of SAX and DOM
- Modern Alternatives: StAX and XPath
- 3.1 Streaming API for XML (StAX)
- 3.2 XPath
- Code Examples
- 4.1 Using StAX
- 4.2 Using XPath
- Conclusion
A Brief Overview to XML in Java
XML (eXtensible Markup Language) is frequently used to store and transport data, making it pertinent to Java developers. Originally, SAX and DOM were the go-to methods for processing XML, but they have their limitations. Understanding these limitations is crucial as we delve into more efficient methods that can elegantly manage XML files without unnecessary overhead.
The Drawbacks of SAX and DOM
SAX (Simple API for XML)
SAX is an event-driven model that reads XML documents sequentially. Although it’s memory efficient, it can be challenging to implement because you have to manage state manually. Here are some notable drawbacks:
- Complexity: Handling state can get complicated.
- One-pass reading: You can only read the document once, making it difficult to navigate backward.
DOM (Document Object Model)
DOM, on the other hand, parses the entire XML document and loads it into memory as a tree structure. This approach allows random access to the document but suffers from several issues:
- High Memory Consumption: Loading large files can quickly consume memory.
- Performance: The overhead of parsing and building the object model can lag performance.
Both of these methods indicate a need for something better-suited for dynamic and scalable applications.
Modern Alternatives: StAX and XPath
3.1 Streaming API for XML (StAX)
StAX offers a good balance between the benefits of SAX and DOM, allowing for both streaming and event-driven processing. It lets you pull data in a way that is more intuitive and manageable.
Benefits of StAX:
- Pull Parsing: You control when to read data, allowing for more logical workflows.
- Lower Memory Footprint: You do not need to keep the entire XML document in memory.
3.2 XPath
XPath offers a powerful way to query XML documents using a syntax that allows for selections based on XML paths. It operates on any XML structure, regardless of whether it has been parsed into memory.
Benefits of XPath:
- Direct Querying: Easily extract specific nodes or sets of nodes.
- Conciseness: Allows for clearer and more maintainable querying.
Code Examples
4.1 Using StAX
Here is a simple example of using StAX to read an XML file:
import javax.xml.stream.XMLEventReader;
import javax.xml.stream.XMLInputFactory;
import javax.xml.stream.events.XMLEvent;
import java.io.FileInputStream;
public class StAXExample {
public static void main(String[] args) {
try {
XMLInputFactory factory = XMLInputFactory.newInstance();
FileInputStream inputStream = new FileInputStream("example.xml");
XMLEventReader eventReader = factory.createXMLEventReader(inputStream);
while (eventReader.hasNext()) {
XMLEvent event = eventReader.nextEvent();
if (event.isStartElement()) {
System.out.println("Start Element: " + event.asStartElement().getName());
} else if (event.isEndElement()) {
System.out.println("End Element: " + event.asEndElement().getName());
} else if (event.isCharacters()) {
System.out.println("Text: " + event.asCharacters().getData());
}
}
} catch (Exception e) {
e.printStackTrace();
}
}
}
Why StAX?
- The design allows you to extract information dynamically while keeping resource usage low.
- You can build more modular applications by controlling the flow of data retrieval.
4.2 Using XPath
Here’s how to use XPath to extract data from XML:
import javax.xml.parsers.DocumentBuilderFactory;
import javax.xml.parsers.DocumentBuilder;
import javax.xml.xpath.XPath;
import javax.xml.xpath.XPathFactory;
import javax.xml.xpath.XPathExpression;
import javax.xml.xpath.XPathConstants;
import org.w3c.dom.Document;
public class XPathExample {
public static void main(String[] args) {
try {
DocumentBuilderFactory factory = DocumentBuilderFactory.newInstance();
DocumentBuilder builder = factory.newDocumentBuilder();
Document doc = builder.parse("example.xml");
XPathFactory xpathFactory = XPathFactory.newInstance();
XPath xpath = xpathFactory.newXPath();
String expression = "/catalog/book/title";
XPathExpression expr = xpath.compile(expression);
String title = (String) expr.evaluate(doc, XPathConstants.STRING);
System.out.println("Title: " + title);
} catch (Exception e) {
e.printStackTrace();
}
}
}
Why XPath?
- XPath can quickly access specific nodes without having to navigate through the entire structure, making it a suitable choice for retrieving particular data.
The Closing Argument
As XML parsing in Java evolves, alternatives such as StAX and XPath emerge as powerful tools to streamline the fetching and manipulation of XML data. They offer developers improved performance, memory efficiency, and intuitive code structures.
By moving away from SAX and DOM, you can build Java applications that are not only efficient but also easier to maintain. As you embark on your next project that involves XML processing, consider incorporating these modern strategies.
For further reading, you may refer to Java XML Processing for a comprehensive overview of XML processing in Java.
Happy coding!