Overcoming CSS Limitations in Android WebView
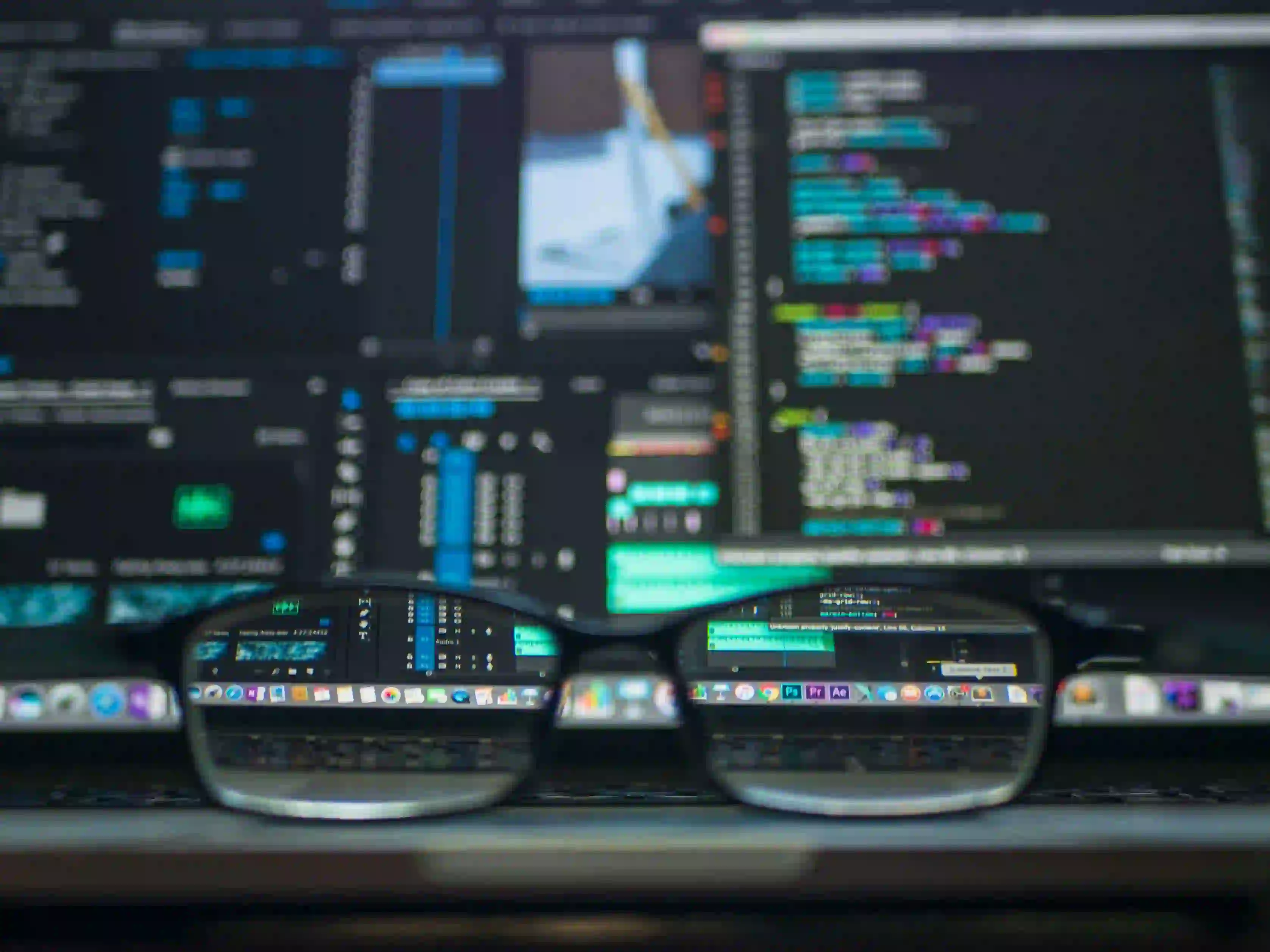
Overcoming CSS Limitations in Android WebView
Android WebView is a powerful component that allows developers to display web content in Android applications. While it leverages Chromium to render web content, developers often face specific limitations when using CSS within WebView. In this post, we will explore these limitations and discuss strategies to effectively work around them.
Understanding WebView and CSS
WebView acts as a mini-browser embedded inside your app. This means that it should ideally support the full range of HTML and CSS features you'd use for any web page. However, there are nuances and constraints that developers must navigate.
Common CSS Limitations in WebView
- Limited CSS Support: While WebView supports many CSS features, certain advanced styles may not render as expected.
- Performance Issues: Heavy CSS can cause the WebView to lag, impacting user experience.
- Different Rendering Engine: Various versions of Android come with different supports for CSS, which can lead to inconsistencies across devices.
- JavaScript Integration: CSS often interacts closely with JavaScript, and ensuring compatibility can sometimes lead to complications.
Strategies to Overcome Limitations
Now letβs delve into effective strategies to overcome these limitations.
1. Use Resets and Normalization
CSS resets and normalization stylesheets help in producing a consistent baseline across different browsers and platforms. By applying these at the beginning of your CSS, you minimize browser inconsistencies.
Example: CSS Reset
/* A simple CSS reset */
html, body, div, span, applet, object, iframe,
h1, h2, h3, h4, h5, h6, p, blockquote, pre,
a, abbr, acronym, address, big, cite, code,
del, dfn, em, img, ins, kbd, q, s, samp,
small, strike, strong, sub, sup, tt, var,
b, u, i, center {
margin: 0;
padding: 0;
border: 0;
font-size: 100%;
font: inherit;
vertical-align: baseline;
}
This code resets the styles of all elements, ensuring a more uniform appearance across various platforms.
2. Optimize CSS for Performance
Minimizing the size of your CSS can enhance WebView performance. Reducing the number of CSS rules and simplifying styles can have a significant impact.
Example: Combining Styles
Instead of writing separate rules for similar elements, combine them to streamline your stylesheet.
/* Before optimization */
h1 { color: blue; }
h2 { color: blue; }
/* After optimization */
h1, h2 {
color: blue;
}
3. Use CSS Media Queries
Media queries allow you to create responsive designs that adapt to different screen sizes and resolutions. This is particularly useful for WebView, as device diversity can affect how content is displayed.
Example: CSS Media Query
@media (max-width: 600px) {
body {
background-color: lightblue;
}
.responsive-text {
font-size: 14px;
}
}
This code provides a different background color for smaller screens, ensuring usability.
4. Simplify Animation and Transitions
Although CSS animations can create a stunning visual experience, they can also be taxing on WebView performance. Use them judiciously.
Example: Basic CSS Transition
.box {
transition: transform 0.3s ease;
}
.box:hover {
transform: scale(1.1);
}
This transition is light, as it targets a scaling effect rather than complex key-frame animations.
5. Leverage JavaScript for Dynamic Styles
Sometimes, a CSS solution may not suffice. In such cases, consider using JavaScript to manipulate styles dynamically.
Example: JavaScript Modifying Styles
document.querySelector('.my-element').style.cssText = "color: red; font-size: 20px;";
This code allows for dynamic responsiveness based on user interactions, enhancing the overall experience without overwhelming CSS.
Addressing JavaScript Integration Issues
As CSS and JavaScript often go hand-in-hand, challenges may arise when one conflicts with the other.
6. Use WebView's JavaScript Interface
To harmonize CSS and JavaScript, utilize WebView's JavaScript interface. This approach allows your application to send data to the WebView from your Android app.
Example: JavaScript Interface in Android
WebView myWebView = findViewById(R.id.webview);
myWebView.getSettings().setJavaScriptEnabled(true);
myWebView.addJavascriptInterface(new WebAppInterface(this), "Android");
In this code, the JavaScript interface allows communication between the app and the WebView, bridging any functionality gaps.
7. Use Progressive Enhancement
For best practices, apply progressive enhancement principles. Start with a basic layout and then layer on styles and scripts as needed, ensuring basic functionality regardless of CSS or JavaScript support.
Testing Your WebView
Testing is critical. Use tools like BrowserStack or LambdaTest to see how your CSS renders across different devices and variations of WebView.
8. Regular Updates
Keep your WebView component up to date. Older versions may lack support for newer CSS features. You can update the WebView system component via Google Play services.
Closing Remarks
By being mindful of the limitations of CSS in Android WebView, you can create more robust and user-friendly experiences. Applying CSS resets, optimizing styles, using media queries, and incorporating JavaScript as needed, are valuable strategies to enhance your web content within WebView.
Do keep testing across different devices and updating your WebView to ensure consistent performance and appearance. As always, user experience should be paramount in your design and development process.
Useful Resources
Embrace the power of Android WebView, leverage CSS effectively, and turn limitations into opportunities for enhanced user experiences. Happy coding!