Bridging the Gap: Human Skills in Coding
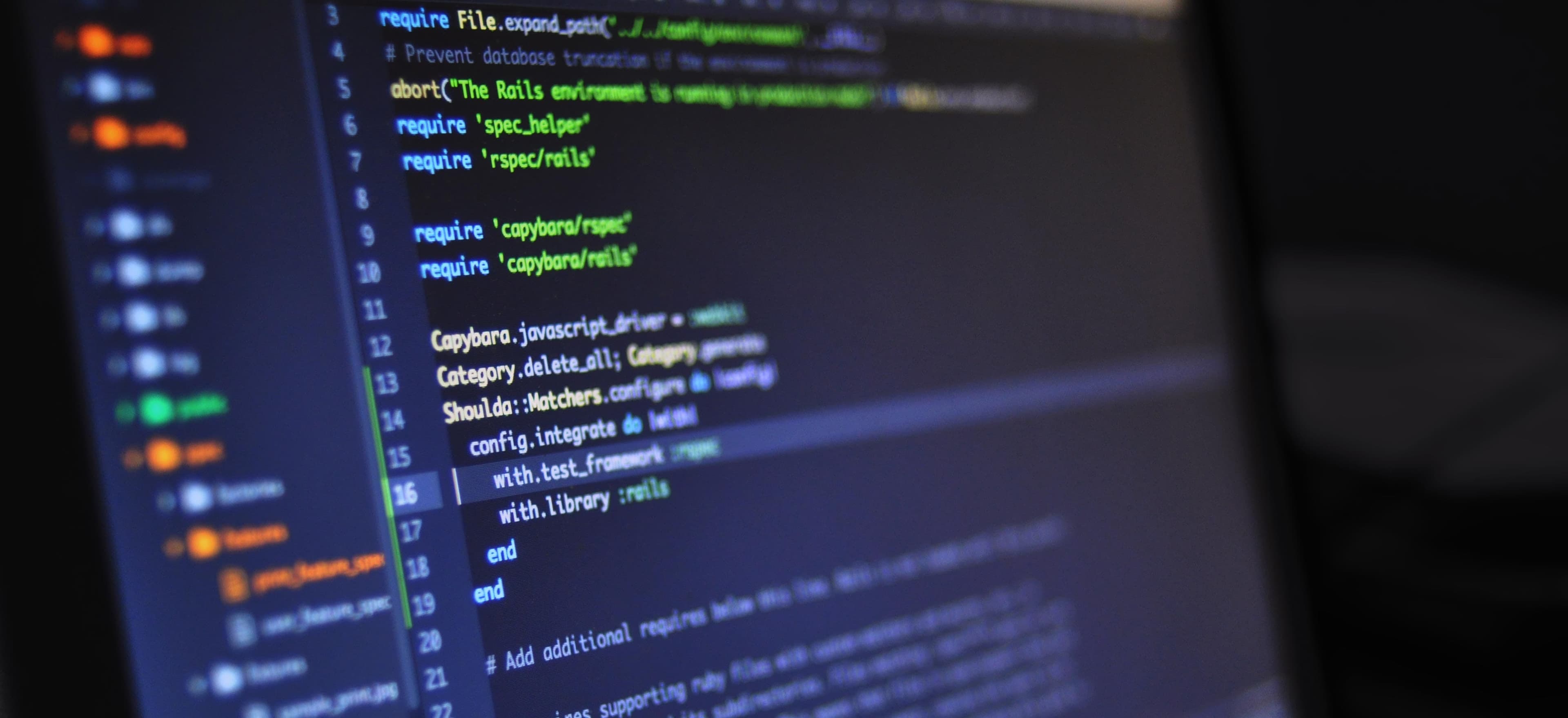
- Published on
Bridging the Gap: Human Skills in Coding
In the world of software development, coding is the heart that keeps the engine running. Yet, while technical skills like programming languages and frameworks are undeniably essential, human skills are equally critical in bridging the gap between mere code and effective, impactful solutions. This article explores the intricate blend of technical and human skills necessary for successful coding in today’s tech landscape.
Understanding Human Skills in Coding
Human skills, often referred to as soft skills, encompass a range of interpersonal and communication abilities that complement technical skills. These can include:
- Communication: The ability to convey ideas clearly, both verbally and in writing.
- Teamwork: Collaborating with others to problem-solve and innovate.
- Adaptability: Adjusting to new challenges, tools, or changes in requirements.
- Empathy: Understanding users' needs to create better user experiences.
- Problem Solving: Tackling issues creatively and effectively.
In the next sections, we will delve into how these human skills can positively impact coding practices while also integrating some essential Java code examples that demonstrate how these concepts can be translated into practical coding scenarios.
The Role of Communication in Coding
Effective communication can make or break a project. While Java might be the language that allows developers to build applications, it is the communication skills that ensure these applications meet customer needs.
Example of Refactoring Code with Communication in Mind
Consider an example where a developer writes a method that does too much:
public void processAndSendEmail(String emailDetails, User user) {
// Process email details
String processedDetails = processDetails(emailDetails);
// Send Email
EmailSender emailSender = new EmailSender();
emailSender.sendEmail(processedDetails, user);
// Log Activity
Logger.log(user.getId() + " sent an email.");
}
This method handles multiple responsibilities: processing data, sending an email, and logging information. If the developer communicates with their team, they might discover that splitting responsibilities is more efficient.
By refactoring, the code becomes clearer:
public void processEmail(String emailDetails, User user) {
String processedDetails = processDetails(emailDetails);
sendEmail(processedDetails, user);
}
public void sendEmail(String processedDetails, User user) {
EmailSender emailSender = new EmailSender();
emailSender.sendEmail(processedDetails, user);
Logger.log(user.getId() + " sent an email.");
}
Here, clearer communication about the method’s purpose promotes better code maintenance, readability, and testing flexibility.
Teamwork Makes the Dream Work
Teamwork in coding refers not only to collaboration on code but also to the collective problem-solving capabilities that a group can provide. Agile methodologies, for instance, thrive on teamwork. In Java development, collaborative efforts can lead to innovative solutions that a single developer might miss.
Incorporating Peer Review
Consider implementing a code review process. It enhances both quality and knowledge-sharing among team members. For instance, using Java, developers can pair program to ensure their code meets quality standards.
public void calculateAverageScore(List<Integer> scores) {
int total = 0;
for (Integer score : scores) {
total += score;
}
double average = total / (double) scores.size();
System.out.println("Average Score: " + average);
}
During a code review, a teammate might suggest using streams, which can make the code more concise and expressive:
public void calculateAverageScore(List<Integer> scores) {
double average = scores.stream()
.mapToInt(Integer::intValue)
.average()
.orElse(0.0);
System.out.println("Average Score: " + average);
}
This feedback loop exemplifies how teamwork can enhance coding practices and lead to better solutions.
Adaptability: Embracing Change
The tech field is synonymous with change. New languages, frameworks, or tools emerge rapidly. Adaptability means being open to learning and updating skills continuously.
Learning a New Library in Java
Imagine a scenario where a Java developer must implement a feature using a new library, such as Spring. Here’s how adaptability manifests in coding:
// Before learning Spring
public void createUser(String username, String password) {
// Code to create a user in the database...
}
// After learning Spring Framework
@Service
public class UserService {
public void createUser(User user) {
// Code to create a user using Spring Data
}
}
The first snippet might have been functional, but by adapting to Spring, our developer leverages powerful features like dependency injection, significantly improving code quality and maintainability.
Empathy: A User-Focused Approach
Empathy in coding involves understanding user perspectives and needs. It is essential for creating applications that are not only functional but also user-friendly.
Designing with the User in Mind
When developing a Java application, empathizing with users can influence design decisions. Here’s an example:
public void displayUserDetails(User user) {
System.out.println("Username: " + user.getUsername());
System.out.println("Email: " + user.getEmail());
}
While functional, the output might not be user-friendly. By putting ourselves in the user's shoes, we might change the approach:
public void displayUserDetails(User user) {
System.out.println("User Details:");
System.out.println("---------------");
System.out.printf("Username: %s%n", user.getUsername());
System.out.printf("Email: %s%n", user.getEmail());
}
This format is easier to read, demonstrating how empathy leads to better user experiences.
Problem Solving: A Critical Skill
At its core, coding is about solving problems. A proficient coder can identify issues and create solutions efficiently.
Example of Debugging a Common Issue
Consider a method that needs to handle input correctly:
public int divide(int numerator, int denominator) {
return numerator / denominator; // Potential for ArithmeticException
}
An adept problem solver considers edge cases, such as division by zero:
public int divide(int numerator, int denominator) {
if (denominator == 0) {
throw new IllegalArgumentException("Denominator cannot be zero.");
}
return numerator / denominator;
}
By incorporating error-handling logic, the developer safeguards against common pitfalls, demonstrating problem-solving prowess.
Bringing It All Together
The landscape of coding in Java and beyond is vast and ever-evolving. While strong technical skills are paramount, the importance of human skills—communication, teamwork, adaptability, empathy, and problem-solving—cannot be overstated. By forging a path that integrates these human skills into daily coding practices, developers not only enhance their technical capabilities but also create a more collaborative, efficient, and user-centric programming environment.
In the end, bridging the gap between technical and human skills is about understanding that while machines execute code, human insights drive innovation and user satisfaction. In a world saturated with code, it is our ability to communicate, empathize, and solve problems that will define the future of software development.
For more insights on enhancing your coding skills, check out resources such as Java’s Official Documentation and The 4 Essential Skills for Software Developers.
Happy coding!
Checkout our other articles