Mastering Pre and Postconditions: Unlocking Use Matchers
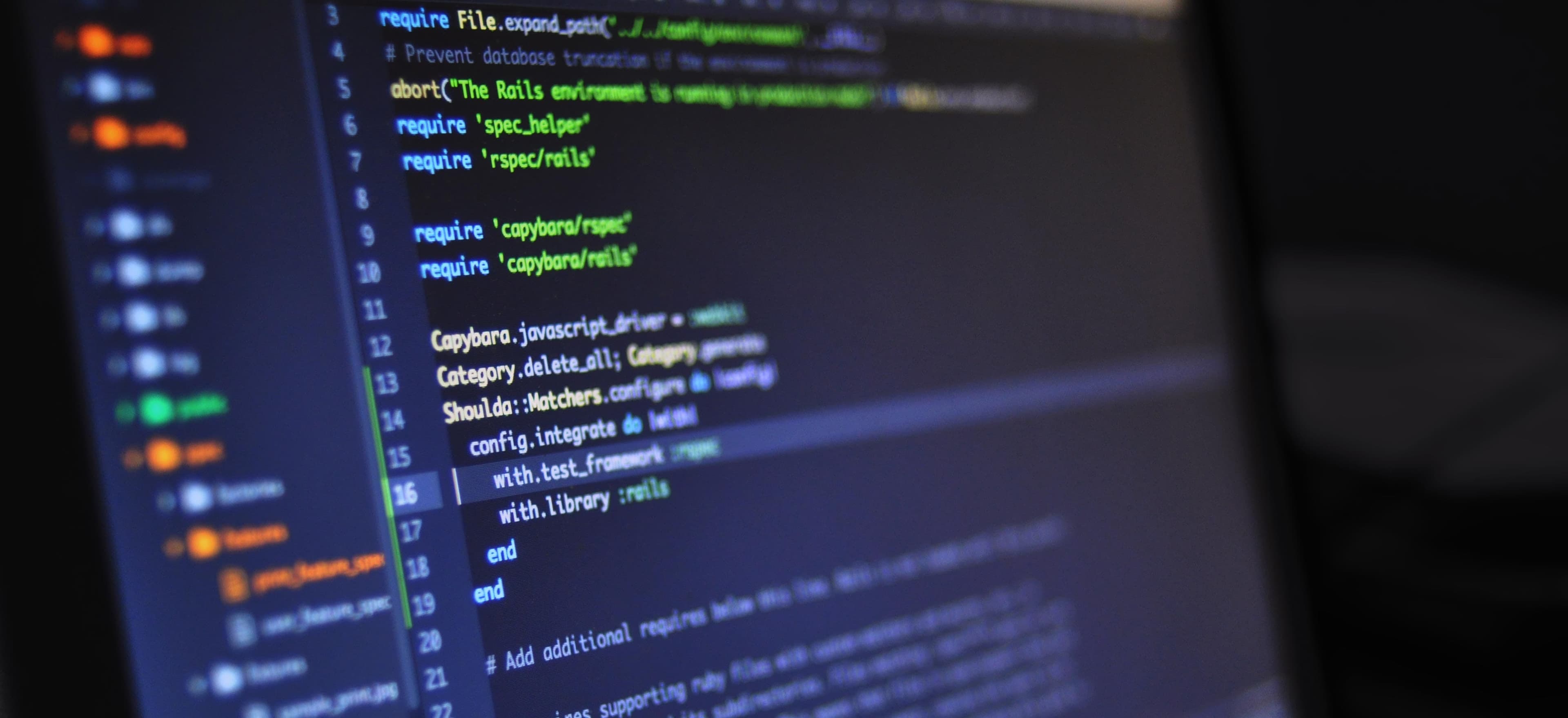
- Published on
Mastering Pre and Postconditions: Unlocking Use Matchers in Java
In the realm of Java programming, achieving robust code often hinges on implementing design by contract principles. A crucial aspect of this principle is the use of pre and postconditions to enforce specific rules that a method must adhere to. By mastering these conditions, developers can unlock the potential of use matchers, making their code more reliable, understandable, and maintainable.
In this blog post, we’ll explore what preconditions and postconditions are, their importance, how to implement them in Java, and how use matchers play a pivotal role in this context.
What are Preconditions and Postconditions?
Preconditions are conditions that must be true before a method is executed. They act as a guarantee that the method has all the necessary information to complete its task successfully. If a precondition is not met, the method should not execute.
Postconditions, on the other hand, are conditions that must be true after the method has completed execution, assuming the preconditions were met. They verify that the method has achieved its intended purpose.
Why Use Preconditions and Postconditions?
Implementing pre and postconditions has several advantages:
- Improved Code Readability: By clearly stating what is required and what will be produced, your code becomes easier to understand.
- Error Handling: They can help identify and catch errors early in the development process, reducing runtime exceptions.
- Facilitating Testing: Unit tests can be more straightforward because they can leverage pre and postconditions to assert expectations.
Implementing Preconditions in Java
Java does not enforce preconditions and postconditions directly but can be simulated using assertions and exceptions. Here’s how:
Using Assertions for Preconditions
Assertions are a tool that can help enforce preconditions. They allow you to verify conditions at runtime and throw an AssertionError
if the condition fails. Here’s an example:
public class Calculator {
public int divide(int numerator, int denominator) {
assert denominator != 0 : "Denominator must not be zero"; // Precondition
return numerator / denominator; // Main operation
}
}
In the above code, we use an assertion to validate that the denominator is not zero. This ensures that the method won't attempt a division by zero, which would throw an ArithmeticException
.
Using Exceptions for Preconditions
For production code, assertions can be disabled, so it might be sensible to explicitly check for conditions using exceptions. Here's an alternate implementation:
public class Calculator {
public int divide(int numerator, int denominator) {
if (denominator == 0) {
throw new IllegalArgumentException("Denominator must not be zero"); // Precondition violation
}
return numerator / denominator; // Main operation
}
}
In this example, the IllegalArgumentException
is used to throw an informative error when the precondition fails.
Implementing Postconditions in Java
To implement postconditions, you can use assertions or return values. Postconditions can often be confirmed by checking the internal state of an object or the return value of a method.
Using Assertions for Postconditions
Here’s how you can assert postconditions, using the earlier division method:
public class Calculator {
public int divide(int numerator, int denominator) {
if (denominator == 0) {
throw new IllegalArgumentException("Denominator must not be zero");
}
int result = numerator / denominator; // Main operation
assert result * denominator == numerator : "Postcondition failed: Invalid division result"; // Postcondition
return result;
}
}
In this example, we check if the result of the division is valid based on the inputs, asserting a postcondition.
Return Values as Postconditions
You can also use the return value of your methods to deduce if postconditions have been satisfactorily met. For instance:
public class BankAccount {
private double balance;
public BankAccount(double initialBalance) {
assert initialBalance >= 0 : "Initial balance must be non-negative"; // Precondition
this.balance = initialBalance;
}
public double withdraw(double amount) {
if (amount < 0) {
throw new IllegalArgumentException("Withdraw amount must be non-negative"); // Precondition
}
double oldBalance = this.balance; // Save old balance
this.balance -= amount; // Main operation
assert this.balance >= 0 : "Postcondition failed: Insufficient funds"; // Postcondition
return oldBalance - this.balance; // Return the amount withdrawn
}
}
In this example, assertions check both the preconditions and postconditions surrounding the withdrawal operation.
Use Matchers and Their Role
Use matchers are a powerful way to verify that a specific contract (made up of preconditions and postconditions) is met when working with tools such as testing frameworks. Libraries like AssertJ or Mockito provide fluent interfaces for assertions that improve readability while enforcing these conditions.
For example, using AssertJ lets you write expressive tests:
import static org.assertj.core.api.Assertions.assertThat;
public class BankAccountTest {
@Test
public void testWithdraw() {
BankAccount account = new BankAccount(100);
int withdrawn = account.withdraw(50);
assertThat(withdrawn).isEqualTo(50); // Assert return value
assertThat(account.getBalance()).isEqualTo(50); // Assert postcondition
}
}
In this test, we check both the return value of the withdrawal and the altered balance, validating both pre and postconditions through clear assertions.
A Final Look
Mastering pre and postconditions in Java helps create more reliable and maintainable code. By framing your methods with these conditions, you provide clear contracts that ensure your code behaves as expected. This not only improves the integrity of your software but also enhances collaboration among developers.
For deeper insights into design by contract in programming, check out articles from Martin Fowler or learn more about AssertJ.
Implement these concepts into your daily programming practices, and unlock the full potential of your code today!