Struggling with Argument Capture in Mockito Tests?
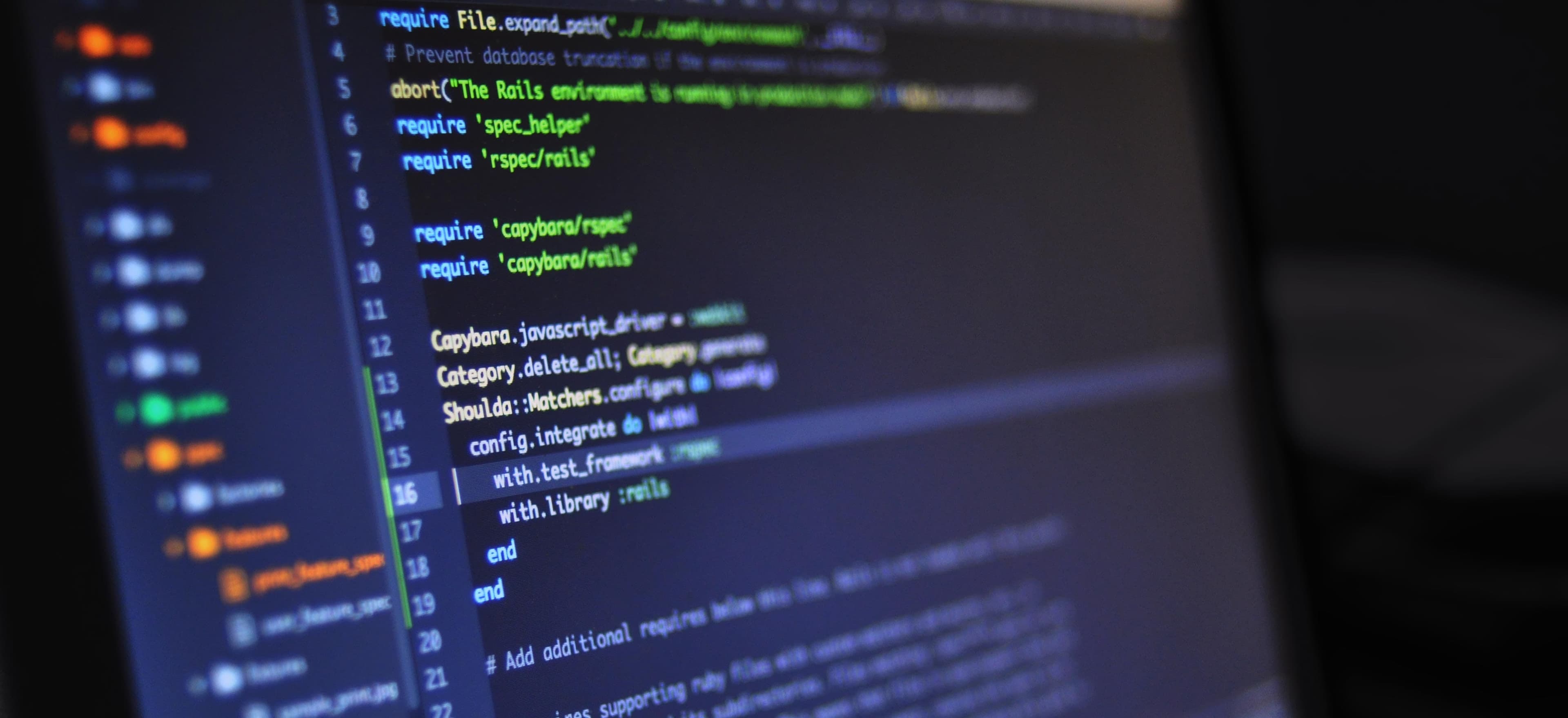
- Published on
Struggling with Argument Capture in Mockito Tests?
Mockito is a powerful framework that allows for easy testing of Java applications. One of its most useful features is the ability to capture method arguments for verification or further investigation. Understanding how to effectively leverage argument captors can significantly enhance your unit tests, making them more reliable and comprehensive.
What is Argument Capturing?
Argument capturing is the act of retrieving the actual arguments that were passed to mocked methods during test execution. This is particularly useful when you want to validate interactions with mocked objects without hardcoding method parameters in your assertions.
Why Use Argument Capture?
- Flexibility: You may not always know what values your methods will receive.
- Clarity: Helps in making clear assertions with dynamic data.
- Validation: Good for validating calls that happen in response to some actions.
Setting Up Mockito
Before we dive into argument capturing, ensure you have Mockito added to your project. If you're using Maven, include the following dependency in your pom.xml
:
<dependency>
<groupId>org.mockito</groupId>
<artifactId>mockito-core</artifactId>
<version>4.0.0</version> <!-- Ensure you are using the latest version -->
<scope>test</scope>
</dependency>
For Gradle, add the following:
testImplementation 'org.mockito:mockito-core:4.0.0'
Example Scenario
Let's say we have a UserService
class that sends a welcome email to a user upon registration. To inform a mock of its actions, we need a EmailService
that our UserService
interacts with. Below is the code snippet of these classes:
UserService.java
public class UserService {
private EmailService emailService;
public UserService(EmailService emailService) {
this.emailService = emailService;
}
public void registerUser(String email) {
// Business logic to register user
emailService.sendWelcomeEmail(email);
}
}
EmailService.java
public interface EmailService {
void sendWelcomeEmail(String email);
}
Now, for testing purposes, we need to verify that the sendWelcomeEmail
method is called with the right email address when a user registers.
Testing with Arguments Capture
Now let’s write a unit test using Mockito to verify this behavior.
UserServiceTest.java
import static org.mockito.Mockito.*;
import org.junit.jupiter.api.BeforeEach;
import org.junit.jupiter.api.Test;
import org.mockito.ArgumentCaptor;
public class UserServiceTest {
private EmailService emailService;
private UserService userService;
@BeforeEach
public void setUp() {
emailService = mock(EmailService.class);
userService = new UserService(emailService);
}
@Test
public void testUserRegistrationSendsWelcomeEmail() {
String userEmail = "test@example.com";
// Act
userService.registerUser(userEmail);
// Setup ArgumentCaptor for the email string
ArgumentCaptor<String> emailCaptor = ArgumentCaptor.forClass(String.class);
verify(emailService).sendWelcomeEmail(emailCaptor.capture());
// Assert
assertEquals(userEmail, emailCaptor.getValue());
}
}
Explanation of the Code
-
Mock Creation:
emailService
is a mock of the EmailService interface. This allows us to monitor interactions without relying on actual implementations. -
Instantiate UserService: We pass the mock email service into
UserService
, ensuring our tests focus on the logic ofUserService
. -
Call the Method: The method
registerUser()
is called onuserService
, simulating a user registration. -
Argument Captor: The
ArgumentCaptor
is created for the type String. This will capture any String argument passed tosendWelcomeEmail
. -
Verification: The
verify()
method checks thatsendWelcomeEmail()
was called on theemailService
mock and captures the argument. -
Assertion: Finally, the test asserts that the captured argument matches the expected email. Using
assertEquals
, we compare the actual value from the captor with what we anticipated.
Benefits of Using Argument Captors
- Helps in scenarios where method parameters are not fixed or predictable.
- Provides a clear pathway to validate complex interactions effectively.
Common Pitfalls to Avoid
-
Using Argument Captors with Primitive Types: Always initialize your captors with the correct type. For primitive types, ensure you are using the appropriate wrapper classes.
-
Overusing Verbosity: While it’s great to capture arguments, don’t make tests unnecessarily complex. Keep it simple.
-
Ignoring Object State: If capturing an object, ensure the state of that object is verified correctly as well.
Closing Remarks
Mockito’s argument captors are an essential feature for writing effective unit tests. They provide flexibility and clarity, allowing you to validate the interactions within your service classes without hard-coding method parameters. By implementing argument capturing in your tests, you can achieve a more robust testing strategy that leads to better quality applications.
For more on Mockito, check the official documentation to explore advanced features and best practices.
By mastering argument capturing, you will find that your confidence in writing tests increases, leading to more maintainable and adaptable code. Happy testing!
Checkout our other articles