How to Efficiently Remove Characters Before a Specific One in Java
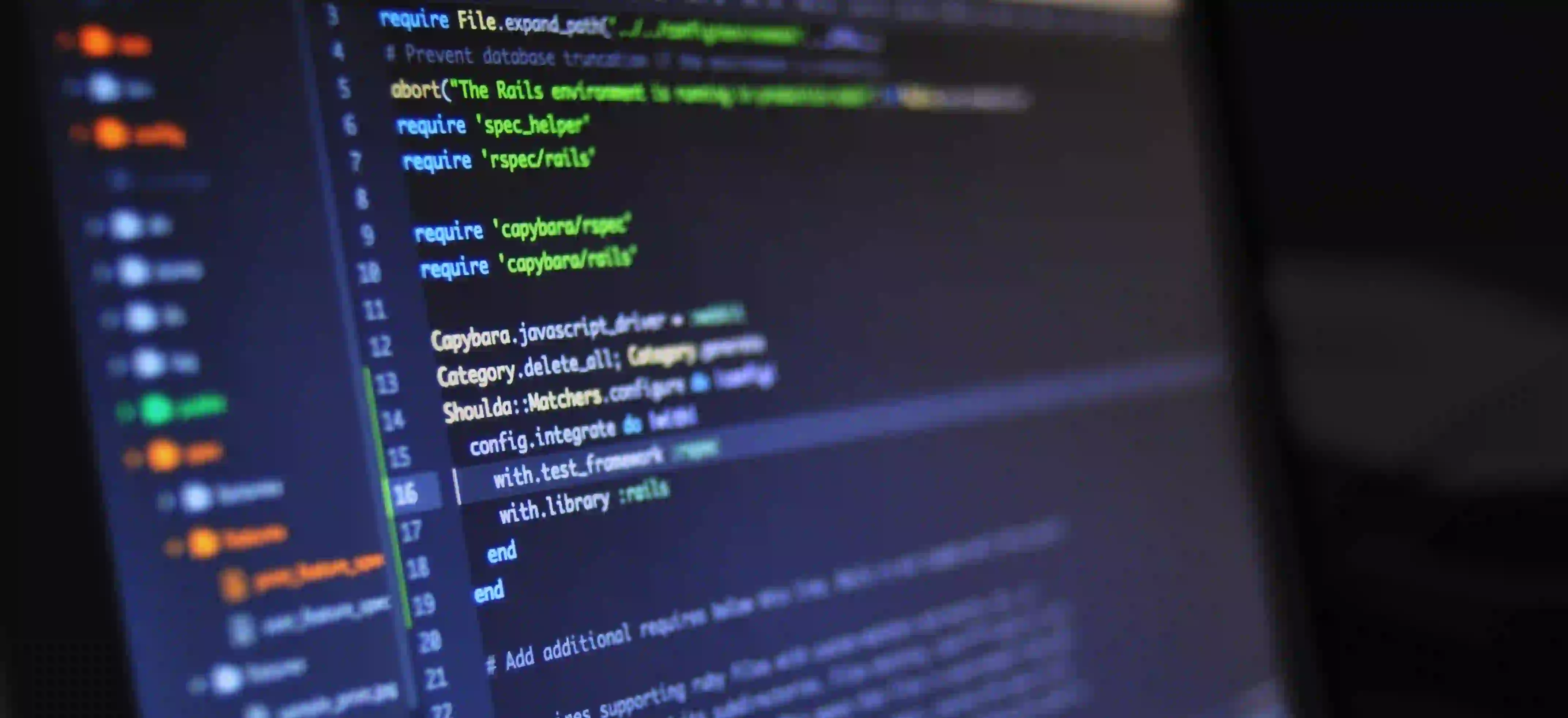
How to Efficiently Remove Characters Before a Specific One in Java
In an era dominated by data processing, effective string manipulation has become an essential skill in programming. Whether you’re dealing with user input, parsing data files, or constructing dynamic content, knowing how to handle strings efficiently can save you time and headaches. In this blog post, we'll dive into how to efficiently remove characters before a specific character in Java.
Understanding the Problem
Imagine you have a string that contains a lot of extraneous characters, but you only need the content following a specific delimiter. For example, given the input string:
"2023-10-13: Important meeting details"
If we want to remove everything before the colon (:
), we would need a method to quickly find the colon’s position and extract the substring that follows.
Java String Methods
Java's String
class provides a healthy suite of methods that can help in string manipulation. Specifically, we’ll utilize indexOf()
and substring()
methods.
indexOf()
This method returns the index of the first occurrence of the specified character (or substring) within the string. It’s essential for locating where we need to start extracting our desired substring.
substring()
Once we have the index of the delimiter, we can leverage substring()
to create a new string that only includes characters from the specified index onward.
Code Implementation
public class StringManipulation {
// Method to remove characters before a specified delimiter
public static String removeCharactersBefore(String input, char delimiter) {
// Get the index of the delimiter
int index = input.indexOf(delimiter);
// Check if the delimiter exists
if (index == -1) {
// Return the original string if delimiter not found
return input;
}
// Use substring to get content after the delimiter
return input.substring(index + 1).trim();
}
public static void main(String[] args) {
String input = "2023-10-13: Important meeting details";
char delimiter = ':';
// Remove characters before the delimiter
String result = removeCharactersBefore(input, delimiter);
// Output the result
System.out.println(result); // Outputs: Important meeting details
}
}
Code Breakdown
-
Method Definition: The
removeCharactersBefore
method takes two parameters: the input string and the delimiter character. -
Finding the Index: We utilize
input.indexOf(delimiter)
to determine where the delimiter appears in the string. -
Handling Non-Existent Delimiters: If the delimiter does not exist in the string, we immediately return the original string to avoid any unwanted data manipulation.
-
Trimming and Extracting: We return the substring found after the specified index, ensuring to trim any unnecessary whitespace with the
.trim()
method.
This code provides a clean and efficient way to manipulate strings based on a specified character.
Performance Considerations
Time Complexity
The time complexity of the indexOf()
and substring()
methods is generally O(n), where n represents the length of the string. This means that in the worst-case scenario, both methods will traverse the entire string, making it efficient for moderate-sized strings.
Memory Complexity
Creating a new string with the substring()
method means that you are allocating additional memory proportional to the remaining part of the string. In applications dealing with large amounts of data, consider the impact this could have on performance and memory usage.
Further Enhancements
You could enhance this method in several ways:
-
Multiple Delimiters: Modify the method to accept a list of delimiters and return the string after the first one found.
-
Case Sensitivity: You might implement an option to ignore case sensitivity when searching for the delimiter.
-
Error Handling: Introduce more robust error checks for inputs, such as null or empty strings.
-
Regular Expressions: For more complex patterns, consider using regular expressions with the
Pattern
andMatcher
classes.
Example: Removing Multiple Characters
import java.util.Arrays;
import java.util.List;
public class StringManipulation {
public static String removeCharactersBeforeAny(String input, List<Character> delimiters) {
int minIndex = -1;
for (char delimiter : delimiters) {
int index = input.indexOf(delimiter);
if (index != -1 && (minIndex == -1 || index < minIndex)) {
minIndex = index;
}
}
return minIndex != -1
? input.substring(minIndex + 1).trim()
: input;
}
public static void main(String[] args) {
String input = "2023-10-13: Important meeting details; Note: Bring your agenda.";
List<Character> delimiters = Arrays.asList(':', ';');
String result = removeCharactersBeforeAny(input, delimiters);
System.out.println(result); // Outputs: Important meeting details; Note: Bring your agenda.
}
}
Key Takeaways
String manipulation in Java can appear daunting, but with the right methods and an understanding of their performance characteristics, it becomes much more manageable. Remember, always consider the context of your data and choose the appropriate methods to handle strings effectively. Feel free to adapt the provided examples to suit your specific needs or challenges.
For further reading, check out the official Java documentation to delve deeper into the capabilities of the String
class.
Happy coding!