Understanding Comparator vs Comparable in Java: Key Differences
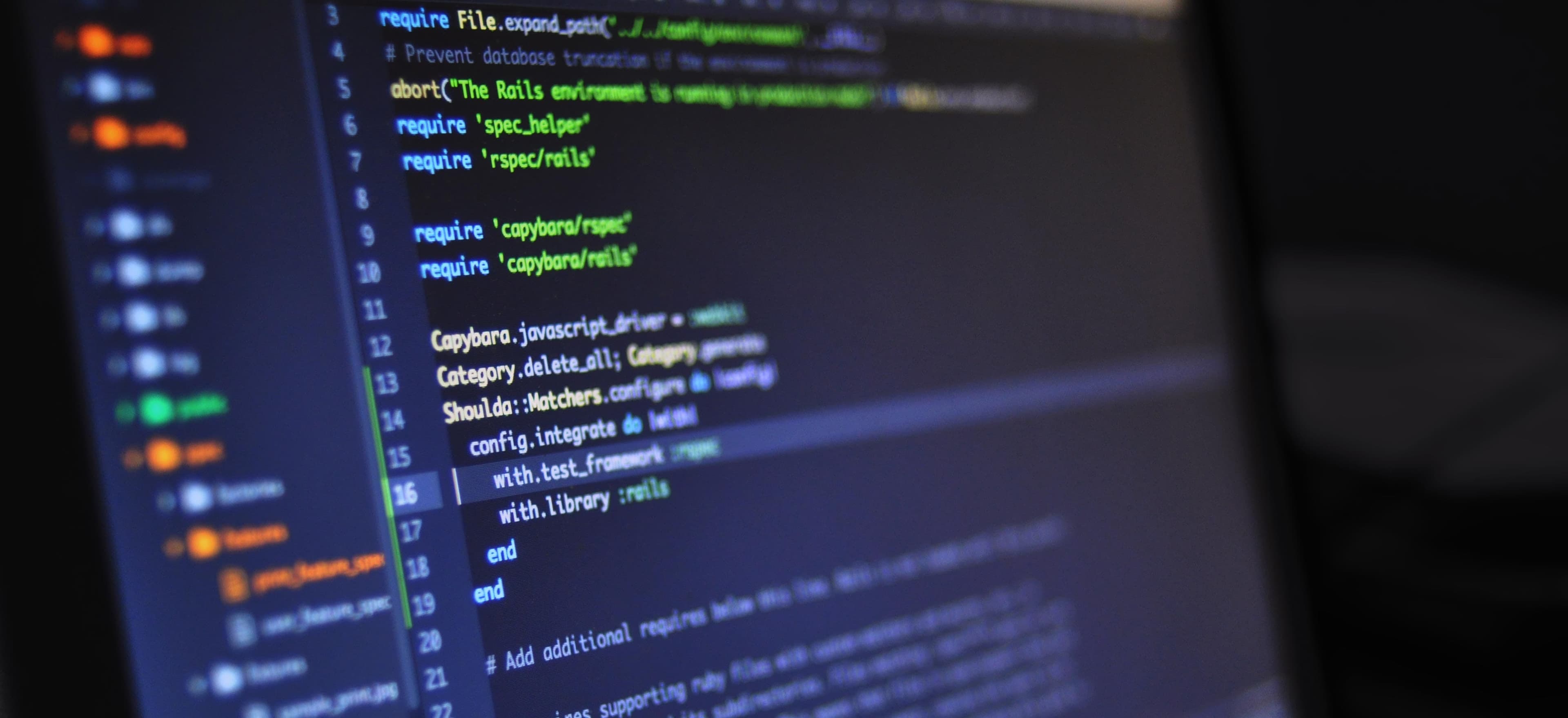
- Published on
Understanding Comparator vs Comparable in Java: Key Differences
When delving into Java and object sorting mechanisms, two critical interfaces often arise: Comparable
and Comparator
. While both interfaces serve the function of sorting, they manifest unique features and functionalities. In this blog post, we'll comprehensively explore the differences between Comparator
and Comparable
, showcasing clear examples and discussing their practical applications.
Table of Contents
- What is Comparable?
- What is Comparator?
- Key Differences between Comparable and Comparator
- Practical Examples of Comparable and Comparator
- When to Use Which?
- Conclusion
What is Comparable?
The Comparable
interface is a part of the java.lang
package and allows objects of a class to be compared based on a natural ordering. When a class implements Comparable
, it must override the compareTo
method. The compareTo
method defines the criteria for comparison.
Example of Comparable
public class Student implements Comparable<Student> {
private String name;
private int age;
public Student(String name, int age) {
this.name = name;
this.age = age;
}
public String getName() {
return name;
}
public int getAge() {
return age;
}
@Override
public int compareTo(Student other) {
// Comparing students based on age
return Integer.compare(this.age, other.age);
}
}
Why Use Comparable?
- Natural Order: It defines a 'natural ordering' for the object, making it easier to sort collections without additional mechanisms.
- Ease of Use: Once this interface is implemented, you can directly use sorting methods like
Collections.sort()
.
What is Comparator?
The Comparator
interface, located in the java.util
package, is more flexible than Comparable
. It is used for custom sorting and allows programmers to define multiple sorting criteria without altering the actual class being sorted.
Example of Comparator
import java.util.Comparator;
public class StudentAgeComparator implements Comparator<Student> {
@Override
public int compare(Student s1, Student s2) {
// Comparing students based on age
return Integer.compare(s1.getAge(), s2.getAge());
}
}
public class StudentNameComparator implements Comparator<Student> {
@Override
public int compare(Student s1, Student s2) {
// Comparing students based on name
return s1.getName().compareTo(s2.getName());
}
}
Why Use Comparator?
- Multiple Sorting Criteria: You can implement various comparators to sort objects in multiple ways.
- No Modification Needed: You can sort different classes or the same class without requiring changes to the class itself.
Key Differences between Comparable and Comparator
| Feature | Comparable | Comparator | |--------------------------|--------------------------------------------|--------------------------------------------------| | Package | java.lang | java.util | | Method to Override | compareTo(T o) | compare(T o1, T o2) | | Sorting Criteria | Single, natural ordering | Multiple, custom ordering | | Modification | Modifies the class that implements it | Implemented separately without modifying class | | Use Case | Default sorting | Custom sorting |
Practical Examples of Comparable and Comparator
Let’s see how we can utilize Comparable
and Comparator
in conjunction with Java’s Collections Framework.
Using Comparable
import java.util.ArrayList;
import java.util.Collections;
import java.util.List;
public class ComparableExample {
public static void main(String[] args) {
List<Student> students = new ArrayList<>();
students.add(new Student("Alice", 22));
students.add(new Student("Bob", 20));
students.add(new Student("Charlie", 23));
// Sorting using Comparable's compareTo method
Collections.sort(students);
for (Student student : students) {
System.out.println(student.getName() + " - " + student.getAge());
}
}
}
Using Comparator
import java.util.ArrayList;
import java.util.Collections;
import java.util.List;
public class ComparatorExample {
public static void main(String[] args) {
List<Student> students = new ArrayList<>();
students.add(new Student("Alice", 22));
students.add(new Student("Bob", 20));
students.add(new Student("Charlie", 23));
// Sorting using Comparator based on name
Collections.sort(students, new StudentNameComparator());
for (Student student : students) {
System.out.println(student.getName() + " - " + student.getAge());
}
// Sorting using Comparator based on age
Collections.sort(students, new StudentAgeComparator());
for (Student student : students) {
System.out.println(student.getName() + " - " + student.getAge());
}
}
}
When to Use Which?
Determining when to use Comparable
and Comparator
often depends on the use case:
-
When to use Comparable: Use it when you want a natural ordering (like sorting by name or age) and when the definition of order should be encapsulated within the class itself.
-
When to use Comparator: Opt for
Comparator
when you need to define multiple sorting criteria or when sorting needs to be controlled externally.
The Last Word
Understanding Comparable
and Comparator
is essential for efficient Java programming, especially when dealing with collections. By implementing these interfaces appropriately, you can take full control of how your objects are sorted. While Comparable
is suited for defining natural order, Comparator
gives you the flexibility to create various sorting strategies while keeping your code clean and maintainable.
For further reading, you might explore the Java Documentation for Comparable
and Java Utilities Documentation for Comparator
.
By grasping these distinctions, you can enhance your programming toolkit and write more efficient and organized Java code. Happy coding!
Checkout our other articles