Troubleshooting Transaction Context Issues with Parallel Streams
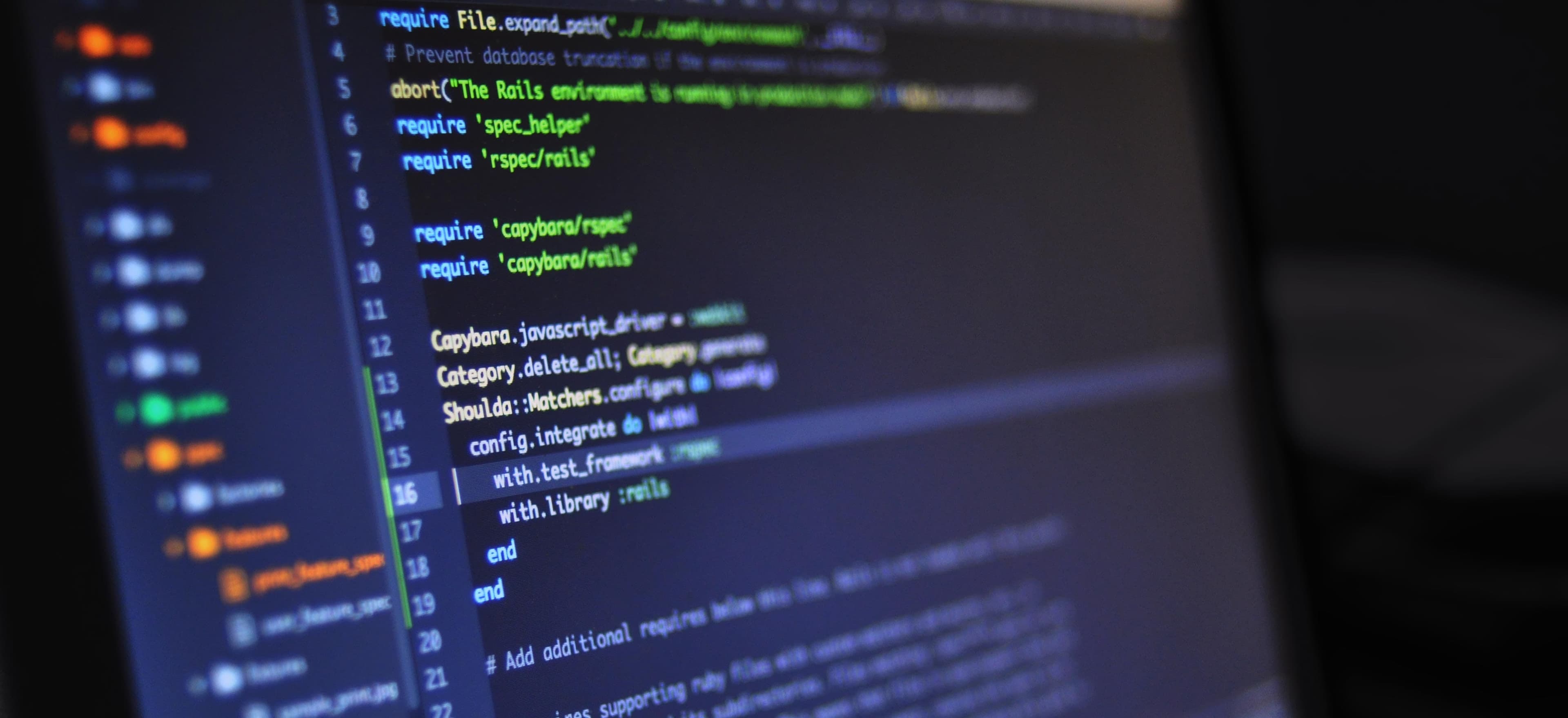
- Published on
Troubleshooting Transaction Context Issues with Parallel Streams in Java
In the ever-evolving world of Java, parallel streams offer a powerful approach to handle data processing tasks efficiently. However, with great power comes great responsibility. One significant challenge developers face when using parallel streams is managing transaction contexts, especially in environments like Spring or Java EE. In this blog post, we will explore the intricacies of transaction contexts in parallel streams, how they affect your applications, and how to troubleshoot common issues effectively.
Understanding Parallel Streams
Java introduced the Stream API in Java 8, enabling developers to process sequences of elements in a functional programming style. Streams can operate in a sequential or parallel mode. While sequential streams process data one element at a time, parallel streams utilize multiple threads to divide and conquer more extensive data sets.
Here's a simple example of how a parallel stream works:
import java.util.Arrays;
public class ParallelStreamExample {
public static void main(String[] args) {
int[] numbers = {1, 2, 3, 4, 5, 6, 7, 8, 9, 10};
int sum = Arrays.stream(numbers)
.parallel()
.map(n -> n * n)
.reduce(0, Integer::sum);
System.out.println("Sum of squares: " + sum);
}
}
In this code, we compute the sum of squares of an array of numbers using a parallel stream. The advantage of the parallel()
method is evident when dealing with larger datasets, where performance gains can be significant.
The Transaction Context
In frameworks like Spring, managing transactions across different threads is crucial. Typically, each thread has its transaction context, which is essential for operations like commits and rollbacks. When running parallel streams, each thread might not automatically inherit the transaction context of the calling thread, leading to potential issues such as lost updates or transaction inconsistency.
Why Transaction Context Matters
- Consistency: Transaction contexts ensure that database operations either succeed or fail completely, preventing data inconsistencies.
- Isolation: They isolate changes made during a transaction, providing safety against concurrent modifications.
- Rollback Mechanism: Should an error occur, transactions can be rolled back to their state before the changes.
Common Issues with Parallel Streams and Transaction Contexts
- Transaction Not Propagating: One of the most common issues is that the transaction context isn't passed correctly to the threads in the pool used by parallel streams.
- Concurrency Issues: Multiple threads may try to modify the same data simultaneously, leading to race conditions.
- Performance Bottlenecks: Incorrect management of transactions can also cause performance issues, as extra locks and waits may be introduced during the execution.
How to Troubleshoot Transaction Context Issues
Step 1: Analyze Your Transaction Management
Understanding your transaction management configuration is the first step. If you are using Spring's declarative transaction management, ensure that it is set up correctly. You might use annotations like @Transactional for methods where transactions should be managed.
Here's an example of how you would annotate a method:
import org.springframework.transaction.annotation.Transactional;
public class UserService {
@Transactional
public void createUser(User user) {
userRepository.save(user);
}
}
Make sure that the transactional boundaries are correctly defined around the operations you wish to perform in parallel.
Step 2: Use a Custom Executor
One way to manage transaction contexts in parallel streams is to use a custom executor service. This service can be configured to manage transactions manually. Here’s how you can create and use a custom executor.
import java.util.concurrent.ExecutorService;
import java.util.concurrent.Executors;
public class CustomExecutor {
public static void main(String[] args) {
ExecutorService executorService = Executors.newFixedThreadPool(4);
try {
parallelProcessing(executorService);
} finally {
executorService.shutdown();
}
}
private static void parallelProcessing(ExecutorService executorService) {
// Your parallel processing logic here
}
}
Using a custom executor allows you to control how threads are managed and how their contexts are propagated.
Step 3: Utilize ThreadLocal
ThreadLocal can help maintain context across threads. In this approach, you can store the transaction context within a ThreadLocal variable and retrieve it when needed.
public class TransactionContext {
private static ThreadLocal<YourTransactionType> transactionContext = new ThreadLocal<>();
public static void setTransaction(YourTransactionType transaction) {
transactionContext.set(transaction);
}
public static YourTransactionType getTransaction() {
return transactionContext.get();
}
public static void clear() {
transactionContext.remove();
}
}
This will ensure that each thread has its transaction context available, mitigating the issues caused by the use of parallel streams.
Step 4: Use Shared Transaction Template
Instead of managing transactions yourself, consider using Spring's TransactionTemplate
. This utility provides an abstraction for transaction management and allows you to execute operations in a defined transaction context more efficiently.
import org.springframework.transaction.PlatformTransactionManager;
import org.springframework.transaction.support.TransactionTemplate;
public class TransactionService {
private TransactionTemplate transactionTemplate;
public TransactionService(PlatformTransactionManager transactionManager) {
this.transactionTemplate = new TransactionTemplate(transactionManager);
}
public void transactionallyExecute(Runnable task) {
transactionTemplate.execute(status -> {
task.run();
return null;
});
}
}
Invoke this method within your parallel stream processing to ensure each task is executed within a transaction.
Final Considerations
While Java's parallel streams can significantly enhance performance and efficiency, they introduce unique challenges regarding transaction contexts. By following best practices and leveraging tools like ThreadLocal, custom executors, and Spring transaction management features, you can mitigate these issues effectively.
For further reading, consider checking out the Java Streams documentation for a deeper understanding of streams. Additionally, the Spring documentation on Transaction Management provides comprehensive insights into handling transactions in Spring applications.
By understanding the underlying mechanics and troubleshooting techniques outlined in this post, you can confidently harness the power of parallel streams while maintaining data integrity and performance in your Java applications.
Checkout our other articles