Debugging Train Wreck Patterns in Java 8: A Developer's Guide
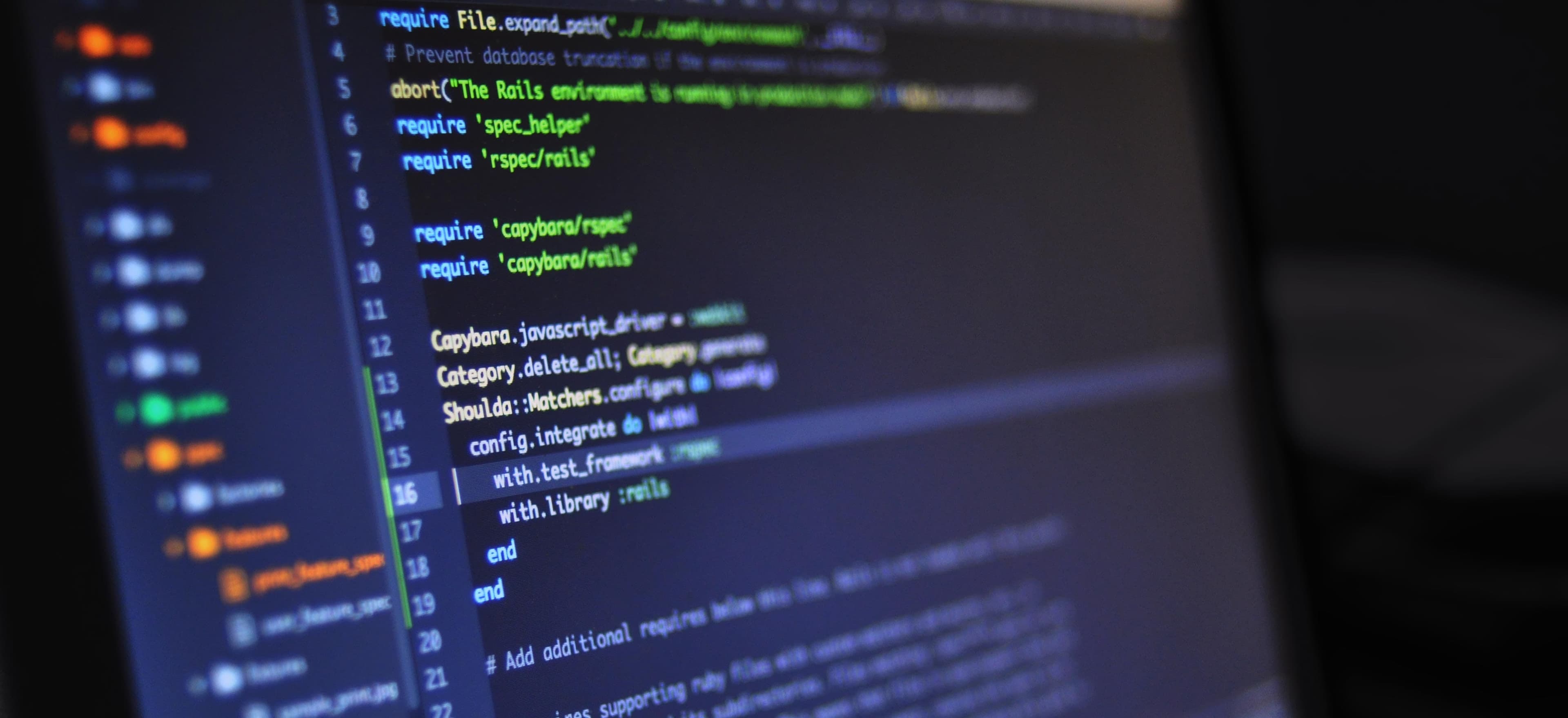
- Published on
Debugging Train Wreck Patterns in Java 8: A Developer's Guide
In the fast-paced world of software development, debugging can often feel like navigating through a dense fog with a limited view. Java 8 introduced numerous enhancements, including lambda expressions and the Stream API, which, while powerful, can also lead to complex, convoluted code patterns often referred to as "train wrecks." This guide will dive into what train wreck patterns are, why they matter, and how you can debug them effectively in your Java applications.
What are Train Wreck Patterns?
Train wreck patterns occur when you have a long chain of method calls, often nested and reliant on the output of previous calls. This can lead to code that is difficult to read, maintain, and debug. The term "train wreck" paints a vivid picture of a messy situation where your code seems to go off the rails.
Example of a Train Wreck Pattern
Consider this common train wreck example using Java 8 streams:
List<String> names = Arrays.asList("Alice", "Bob", "Charlie", "Dave");
List<String> filteredNames = names.stream()
.filter(name -> name.startsWith("A") || name.startsWith("B"))
.map(String::toUpperCase)
.sorted()
.collect(Collectors.toList());
At a glance, this code appears concise and elegant. However, if we make this slightly more complex—say, adding additional filters or transformations—it can quickly become difficult to follow.
Why Train Wreck Patterns Matter
Train wreck patterns often introduce several issues:
- Readability: Long chains of methods make it hard to quickly grasp the intent of the code.
- Debugging Difficulty: When an error occurs, pinpointing the source of the problem can be like finding a needle in a haystack.
- Performance: Excessively chained method calls can lead to inefficiency as each call processes the dataset.
Techniques for Identifying and Resolving Train Wreck Patterns
1. Break Down Your Code
The first step in debugging train wreck patterns is to break down your code into smaller, more manageable components. This involves extracting segments of your code into separate methods or variables to clarify their purpose.
Refactoring the Example
Let's refactor the earlier example to make it more manageable:
List<String> names = Arrays.asList("Alice", "Bob", "Charlie", "Dave");
List<String> filteredNames = filterNames(names, Arrays.asList("A", "B"));
List<String> upperCaseNames = toUpperCase(filteredNames);
List<String> sortedNames = sortNames(upperCaseNames);
System.out.println(sortedNames);
private static List<String> filterNames(List<String> names, List<String> initials) {
return names.stream()
.filter(name -> initials.stream().anyMatch(name::startsWith))
.collect(Collectors.toList());
}
private static List<String> toUpperCase(List<String> names) {
return names.stream()
.map(String::toUpperCase)
.collect(Collectors.toList());
}
private static List<String> sortNames(List<String> names) {
return names.stream()
.sorted()
.collect(Collectors.toList());
}
Commentary
By breaking the chains into smaller, self-contained methods, we have enhanced the clarity of our code. Each method has a single responsibility—filtering, transforming, and sorting—making it easier to follow the logic and debug as needed.
2. Utilize Intermediate Variables
Instead of chaining everything together in a single expression, use intermediate variables to store results. This gives you points of inspection during debugging.
Example with Intermediate Variables
List<String> names = Arrays.asList("Alice", "Bob", "Charlie", "Dave");
List<String> filteredNames = names.stream()
.filter(name -> name.startsWith("A") || name.startsWith("B"))
.collect(Collectors.toList());
List<String> upperCaseNames = filteredNames.stream()
.map(String::toUpperCase)
.collect(Collectors.toList());
List<String> sortedNames = upperCaseNames.stream()
.sorted()
.collect(Collectors.toList());
System.out.println(sortedNames);
3. Use Debugging Tools
Java IDEs come with powerful debugging tools that allow you to step through your code execution line by line. Use breakpoints to inspect the state of your program at different stages in your method chains.
4. Logging
Effective logging can help track the flow of execution and the state of variables. You can log intermediate results to better understand what's happening at each stage in your chained calls.
Example of Logging
import java.util.logging.Logger;
private static Logger logger = Logger.getLogger("MyLogger");
List<String> sortedNames = names.stream()
.filter(name -> {
boolean condition = name.startsWith("A") || name.startsWith("B");
logger.info("Filtering name: " + name + ", passes filter: " + condition);
return condition;
})
.map(name -> {
String upperName = name.toUpperCase();
logger.info("Transforming name: " + name + " to " + upperName);
return upperName;
})
.sorted()
.collect(Collectors.toList());
5. Explore Java Debugging Techniques
Consider brushing up on Java debugging techniques such as:
- Using Java's built-in debugging tools (jdb): Java provides a command-line debugger that can help sift through your application.
- Stack traces: Understanding and leveraging stack traces can help identify where errors occur.
6. Design Patterns
Implement design patterns such as the Chain of Responsibility or Decorator patterns to give structure to your method chaining. Choosing the right pattern allows the code to remain modular and easier to debug.
The Last Word
Debugging train wreck patterns in Java 8 requires a balanced approach that combines breaking down complex chains of logic, utilizing tools effectively, and employing best practices. By focusing on clarity and maintainability, you can significantly reduce the occurrence of train wreck patterns in your code.
While Java 8 introduced powerful features that can streamline your coding process, they can also complicate your logic if not used judiciously. Always aim for readable code and keep debugging practices at the forefront of your development cycle.
For further reading and resources, check out these links:
By adhering to these techniques, you can navigate the complexities of Java 8 while maintaining clear, manageable, and debug-friendly code. Happy coding!