Common Issues with Paging Library 3 and Content Providers
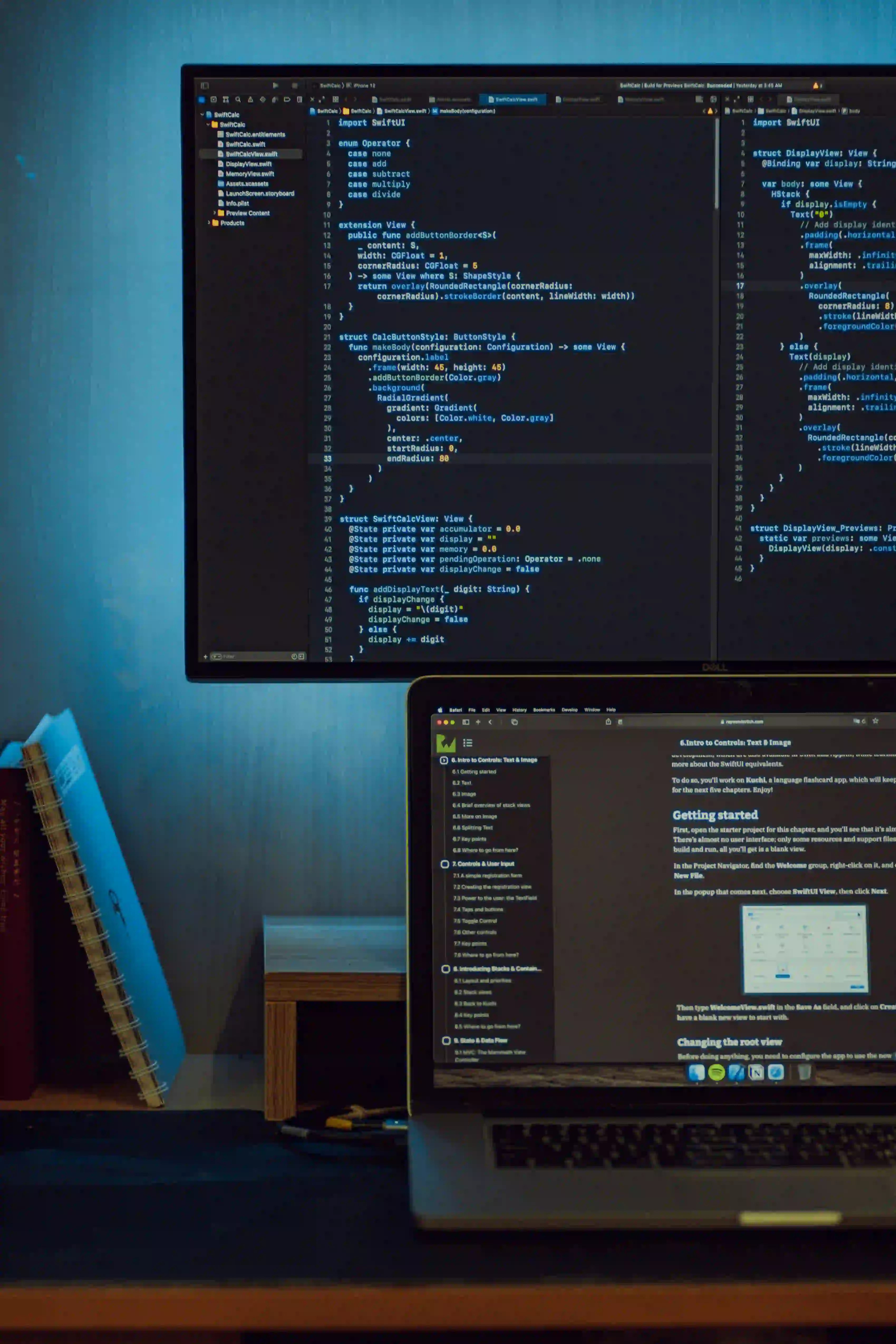
Common Issues with Paging Library 3 and Content Providers
The Android architecture offers various libraries designed to make the development process easier and more efficient. One such library is the Paging Library, now at its third iteration, which provides a streamlined way to load large datasets incrementally. However, integrating the Paging Library with Content Providers can lead to a variety of issues. In this blog post, we'll explore common problems developers face when using Paging Library 3 with Content Providers and how to tackle them head-on.
Understanding Paging Library and Content Providers
Before diving into the issues, let's briefly review what each component does:
-
Paging Library: This library helps you load data in chunks, significantly reducing memory consumption and increasing performance, especially when dealing with large datasets. It fetches data in pages, which is beneficial for UI performance.
-
Content Providers: They are part of Android's data management framework. Content Providers facilitate access to a structured data set in a way that allows for inter-application data sharing.
Common Issues
1. Initial Load Time
Issue
One of the most common issues developers face is slow initial loading times when integrating the Paging Library with a Content Provider. Users may experience a delay before seeing the initial data on the screen.
Solution
To tackle this issue, ensure that your Content Provider returns data efficiently. Use Room with the Paging Library, as Room is optimized for database operations.
@Query("SELECT * FROM data_table LIMIT :limit OFFSET :offset")
fun getItems(limit: Int, offset: Int): List<DataEntity>
This limits the number of rows fetched, reducing the amount of data transferred initially. The return type, which is used in the Paging source, can be a Flow or LiveData object to observe changes in the data effortlessly.
2. Data Consistency
Issue
When multiple consumers are modifying the data, ensuring data consistency becomes a hassle. Updates from one source may not reflect across the Paging Library.
Solution
To solve this, implement a change tracking mechanism in your Content Provider. When inserting or updating data, notify the observer via the notifyChange
method.
override fun insert(uri: Uri, values: ContentValues?): Uri {
val id = database.insert(TABLE_NAME, null, values)
context?.contentResolver?.notifyChange(uri, null)
return Uri.withAppendedPath(CONTENT_URI, id.toString())
}
This approach ensures that the Paging Library is aware of any changes, prompting it to fetch updated records automatically.
3. Mismatched Paging Source and Data Changes
Issue
Users may face issues when the data in the Content Provider changes after the Paging source has been created but before the data finishes loading. Pages might not correspond to the backend data.
Solution
Use invalidate()
on the PagingSource object to refresh and reload the data when there are any changes in the Content Provider.
class MyPagingSource(private val contentResolver: ContentResolver) : PagingSource<Int, DataEntity>() {
override suspend fun load(params: LoadParams<Int>): LoadResult<Int, DataEntity> {
val pageNumber = params.key ?: 0
// Load data from the Content Provider
// ...
// After updating data, invalidate
invalidate()
}
}
This invalidation will force the Paging source to refetch data, ensuring that it provides the most updated dataset.
4. SQLite Query Performance
Issue
A poorly optimized SQLite query can lead to performance issues, such as excessively long load times or high memory consumption.
Solution
Use indexed queries where possible, and make sure to analyze and optimize your queries. Tools such as the SQLite Query Planner can help identify inefficient queries.
CREATE INDEX idx_data ON data_table(column_name);
Adding an index can significantly speed up data retrieval processes.
5. Handling Network Changes
Issue
In applications that mix local storage and remote data (like a web service), handling network state changes while paging can lead to errors or an unresponsive UI.
Solution
Implement a robust network monitoring mechanism. Use a library such as WorkManager to listen for network state changes and update your content provider accordingly.
val connectivityManager = context.getSystemService(Context.CONNECTIVITY_SERVICE) as ConnectivityManager
connectivityManager.registerNetworkCallback(object : ConnectivityManager.NetworkCallback() {
override fun onAvailable(network: Network) {
// Update Content Provider with new data
}
})
This approach ensures that your data is always current, improving the overall responsiveness of your Paging Library implementation.
6. Missing Bindings With LiveData
Issue
Sometimes developers experience missing LiveData bindings when trying to observe data changes from a Content Provider.
Solution
Ensure you properly implement LiveData
in conjunction with your PagingSource. The LiveData can encapsulate the flow of your paging data and refresh the UI when any changes to the underlying datasets occur.
val liveData = LivePagedListBuilder(pagingSourceFactory, config).build()
liveData.observe(owner, Observer { pagedList ->
// Update UI with the new paged data
})
This guarantees that the UI remains responsive and reflects data changes instantaneously.
Final Thoughts
While integrating Paging Library 3 with Content Providers offers numerous benefits such as memory efficiency and enhanced performance, it's essential to be wary of the common pitfalls discussed in this post. By following the recommended solutions, you can ensure a smooth user experience and maintain optimal data flow within your Android applications.
For further reading, you might find the official Android Paging Documentation and Content Provider Documentation helpful.
Maintaining a combination of best practices while coding can lead to a more robust solution. Happy coding!