Why Developers Fear JWT: Security Risks Explained
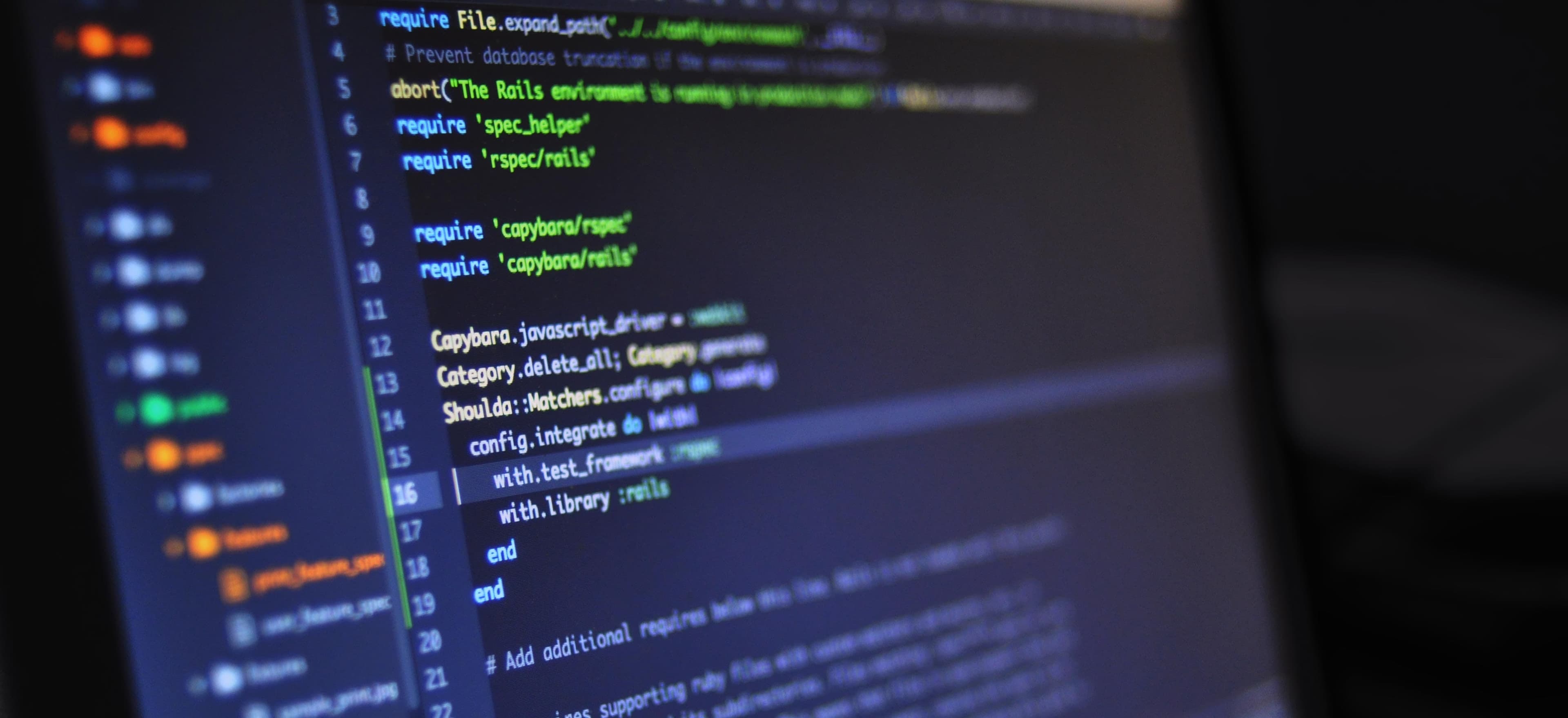
- Published on
Why Developers Fear JWT: Security Risks Explained
JSON Web Tokens (JWT) have become a popular choice for handling authentication and authorization in web applications. However, with this popularity comes a host of concerns, especially regarding security. In this post, we will delve into the reasons why developers often tread carefully around JWTs, breaking down the inherent risks and providing strategies to mitigate them.
Understanding JWT
Before we dive into the security concerns, it's important to grasp what JWTs are and how they function.
JWT is a compact, URL-safe means of representing claims to be transferred between two parties. The claims in a JWT are encoded as a JSON object that is used as the payload of a JSON Web Signature (JWS) structure or as the plaintext of a JSON Web Encryption (JWE) structure, enabling the claims to be digitally signed or integrity protected with a message authentication code.
A typical JWT consists of three parts:
- Header: Typically indicates the type of token (JWT) and the signing algorithm (e.g., HMAC SHA256).
- Payload: Contains the claims, which can be public, private, or registered.
- Signature: Created by combining the encoded header and payload, then signing it with a secret key or a public/private key pair.
Example of a JWT
eyJhbGciOiJIUzI1NiIsInR5cCI6IkpXVCJ9.
eyJzdWIiOiIxMjM0NTY3ODkwIiwibmFtZSI6IkpvaG4gRG9lIiwiaWF0cCI6MTYxNjI3NDA4MH0.
SflKxwRJSMeKKF2QT4fwpMeJf36POk6yJV_adQssw5c
In this example, the JWT consists of the header, payload, and signature, separated by periods.
Security Risks of JWT
1. Token Theft
The most significant risk associated with JWT is token theft. If a JWT is intercepted during transmission, an attacker can impersonate the user. This is particularly concerning if the JWT is not properly secured during transport.
Mitigation:
- Always use HTTPS to encrypt the transmission of sensitive information, including tokens.
- Implement additional measures like SameSite cookies to prevent CSRF attacks.
2. Token Expiry and Revocation
JWTs are stateless and typically have an expiration time. Once issued, they cannot be revoked until they expire. This poses a challenge if a token needs to be invalidated before its intended expiration time—such as in the case of user logout or compromised credentials.
Mitigation:
- Implement short-lived tokens and utilize refresh tokens. A short expiration reduces the time an attacker has to use a stolen token.
- Maintain a blacklist of revoked tokens or adopt a compromise strategy, where older tokens are evaluated against a valid state maintained on the server.
3. Key Management
The security of a JWT relies heavily on the strength of its signing key. If a developer hardcodes a key or if a signing key is compromised, attackers can create legitimate-looking tokens.
Mitigation:
- Rotate signing keys periodically.
- Treat your secret keys like passwords—never expose them in your codebases. Utilize environment variables and secret managers for improved security.
4. Algorithm Manipulation
JWT tokens can use various signing algorithms (HMAC, RSA, etc.). Developers sometimes set their application to accept different algorithms without proper checks. An attacker can exploit this by altering the token's algorithm in the header to none
, allowing them to gain unauthorized access.
Mitigation:
- Always validate the algorithm used in the JWT. Avoid enabling support for the
none
algorithm in your applications.
5. Payload Exposure
Since the payload of a JWT is merely Base64Url-encoded, it can easily be decoded. Sensitive data embedded in the token is exposed to anyone who has access to it, even if it's signed.
Mitigation:
- Avoid storing sensitive data in the payload. Use the JWT primarily for authentication and authorization claims instead.
6. Replay Attacks
If a token is stolen, it can be reused until either the token expires or the user forcibly logs out. A replay attack is a common occurrence in such scenarios, where an attacker intercepts the JWT and uses it to gain unauthorized access.
Mitigation:
- Implement strategies like signed timestamps or nonce values to track the validity of token usage.
Example Strategy: Implementing Short-Lived JWTs with Refresh Tokens
Here's a simple example of how you can implement a short-lived JWT with a refresh token mechanism.
// Generate a short-lived access token
public String generateAccessToken(User user) {
long expirationTime = 15 * 60 * 1000; // 15 minutes
Date now = new Date();
return Jwts.builder()
.setSubject(user.getUsername())
.setIssuedAt(now)
.setExpiration(new Date(now.getTime() + expirationTime))
.signWith(SignatureAlgorithm.HS256, SECRET_KEY)
.compact();
}
// Generate a long-lived refresh token
public String generateRefreshToken(User user) {
long expirationTime = 7 * 24 * 60 * 60 * 1000; // 7 days
Date now = new Date();
return Jwts.builder()
.setSubject(user.getUsername())
.setIssuedAt(now)
.setExpiration(new Date(now.getTime() + expirationTime))
.signWith(SignatureAlgorithm.HS256, SECRET_KEY)
.compact();
}
Explanation:
- Short-lived Access Token: The access token is valid for a brief period (15 minutes), limiting the window an attacker has to utilize a stolen token.
- Long-lived Refresh Token: The refresh token lasts longer (7 days) and should be securely stored. This enables the user to obtain a new access token without requiring them to log back in frequently.
The Bottom Line
While JWTs offer a convenient method for handling stateless authentication, they are not without their risks. Developers should be aware of the security implications and implement best practices to mitigate the potential for abuse. By understanding the nature of these risks and planning accordingly, you can leverage the efficiency of JWT while safeguarding your application.
For further reading on JWT and security best practices, consider visiting OWASP JWT Best Practices and familiarize yourself with secure coding practices to enhance your application's resilience against vulnerabilities.
References
- JWT Introduction
- Secure Server-side Token Storage
- SameSite Cookie Attributes
By following these guidelines and staying informed about security techniques, developers can implement JWTs in their applications while minimizing potential risks.