Mastering Java Regex: Common Pitfalls and Best Practices
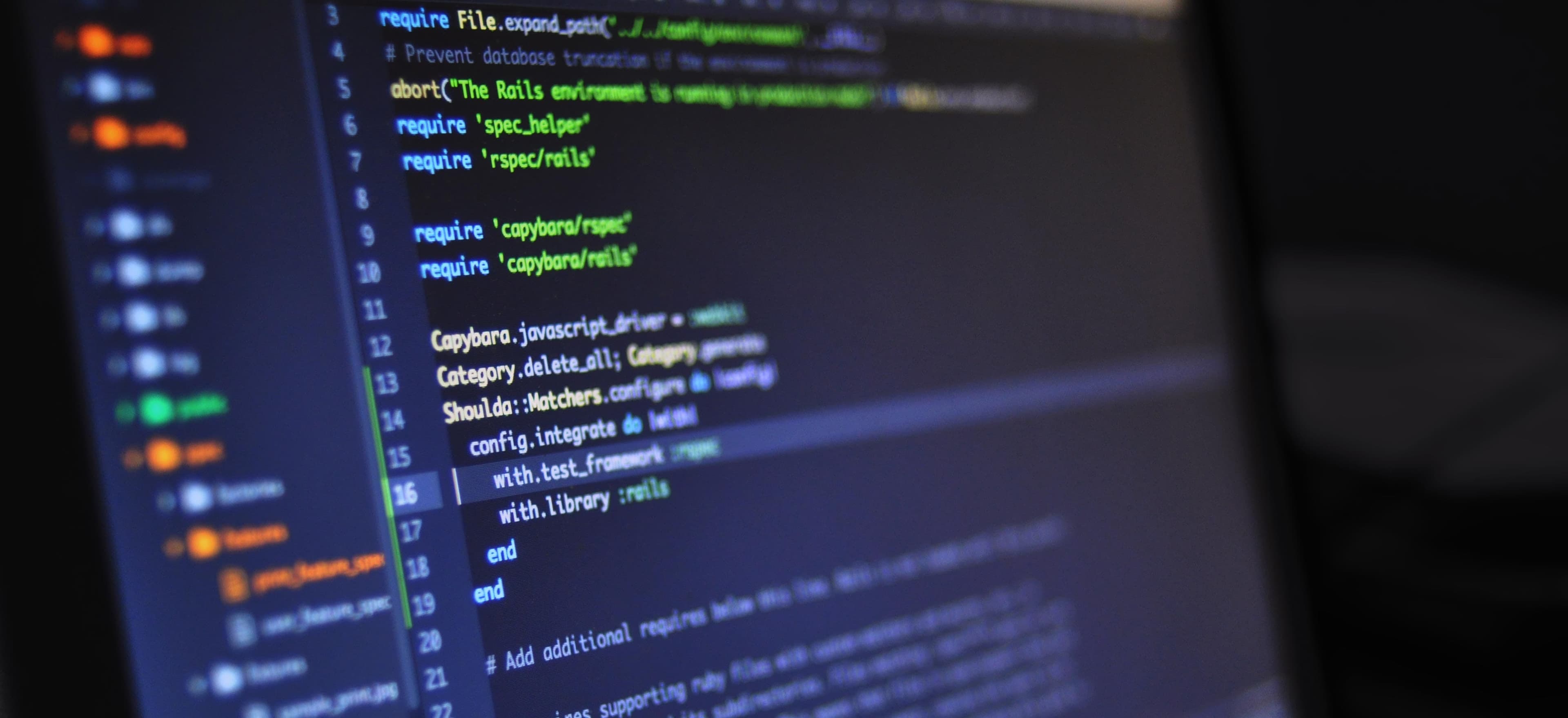
- Published on
Mastering Java Regex: Common Pitfalls and Best Practices
Regular expressions (regex) can be a powerful tool in Java programming for string manipulation, validation, and search functionalities. However, using regex can often feel like walking a tightrope, as it is easy to misstep into common pitfalls. In this blog post, we will discuss best practices for working with Java regex, illuminate common mistakes, and provide illustrative code snippets to solidify your understanding.
What is Regex?
Regular expressions are sequences of characters that form a search pattern. They can be used to perform pattern matching on strings. In Java, regex is part of the java.util.regex
package, which provides classes like Pattern
and Matcher
.
Getting Started with Java Regex
Before diving into best practices, let's establish a foundational understanding of how to use regex in Java.
Creating a Pattern
To create a regex pattern, you start by compiling it using Pattern.compile()
.
import java.util.regex.Matcher;
import java.util.regex.Pattern;
public class RegexExample {
public static void main(String[] args) {
String text = "sample email: example@test.com";
String regex = "\\S+@\\S+\\.\\S+";
Pattern pattern = Pattern.compile(regex);
Matcher matcher = pattern.matcher(text);
while (matcher.find()) {
System.out.println("Found email: " + matcher.group());
}
}
}
Explanation:
\\S+
matches one or more non-whitespace characters.@
signifies the presence of an '@' symbol in an email format.\\.
escapes the dot, treating it as a character rather than a wildcard.
Why Use Regex?
Regex offers succinct and efficient methods for string searching and manipulation. Here are some reasons to use regex:
- Pattern Matching: Easily search for specific patterns in strings.
- Data Validation: Validate formats (like email, URL, etc.).
- Search and Replace: Effortlessly replace substrings based on patterns.
Common Pitfalls
1. Overcomplicating Patterns
It can be tempting to craft overly complex regex patterns, which not only become hard to read but also challenging to debug. For simplicity:
String regex = "(\\w+)@(\\w+)(\\.\\w+)+";
Best Practice: Start with simple patterns. Build complexity gradually while continuously testing.
2. Forgetting Patterns for Special Characters
Certain characters, such as .
(dot), *
(asterisk), and ?
(question mark), have special meanings in regex. Failing to escape them results in unexpected behavior.
Incorrect Example:
String regex = "test.test"; // This will match 'test' followed by any character and 'test' again.
Correct Example:
String regex = "test\\.test"; // Correctly escaping the period.
3. Using String Literals Directly
In Java, regex patterns are often defined using string literals, resulting in extra backslashes. Many developers fall into the trap of forgetting to escape characters adequately.
Incorrect Example:
String regex = "\d+"; // This will throw a compilation error.
Correct Example:
String regex = "\\d+"; // Use double backslashes to escape.
4. Ignoring Case Sensitivity
Regex matching is case sensitive by default, which can lead to missing valid matches. To combat this, you can use the Pattern.CASE_INSENSITIVE
flag.
Pattern pattern = Pattern.compile("hello", Pattern.CASE_INSENSITIVE);
Best Practices
To become proficient in Java regex, consider these best practices that enhance both performance and clarity.
1. Readable Patterns
Create regex patterns that are easy to read. Consider using comments to explain complex sections.
String regex = "(?<=@)\\w+(?=\\.)";
// Explanation:
// (?<=@): Positive lookbehind for '@'
// \\w+: Matches one or more word characters
// (?=\\.): Positive lookahead for '.'
2. Pre-compile Patterns
When using the same pattern multiple times, compile it once and reuse it. This is particularly beneficial in loops or large-scale applications.
Pattern pattern = Pattern.compile("example");
// Use the 'pattern' instance in multiple Matcher instances.
3. Limit Your Quantifiers
Quantifiers (*, +, ?) can lead to excessive backtracking when they are not defined tightly. It is advisable to be specific about what you are expecting:
Less Efficient:
String regex = "(a+|b+)+";
More Efficient:
String regex = "(a{2,}|b{2,})"; // Matches two or more 'a's or 'b's.
4. Utilize Named Groups
Named capturing groups can significantly increase the readability of your regex patterns, especially for complex expressions.
String regex = "(?<username>\\w+)@(?<domain>\\w+\\.\\w+)";
Pattern pattern = Pattern.compile(regex);
Matcher matcher = pattern.matcher("user@example.com");
if (matcher.find()) {
System.out.println("Username: " + matcher.group("username"));
System.out.println("Domain: " + matcher.group("domain"));
}
5. Testing and Debugging
Always test your regex patterns. Several online tools like Regex101 provide interactive environments for crafting and testing regex.
To Wrap Things Up
Mastering Java regex can substantially improve your string manipulation capabilities. However, pay careful attention to the pitfalls and adopt best practices to avoid common errors. Regular expressions are powerful, but they can also be intricate. The key to success lies in simplicity and clarity.
For further reading, check out the official Java documentation on regex and continue practicing. Happy coding!