Overcoming the Complexity of Hamcrest Matchers in Testing
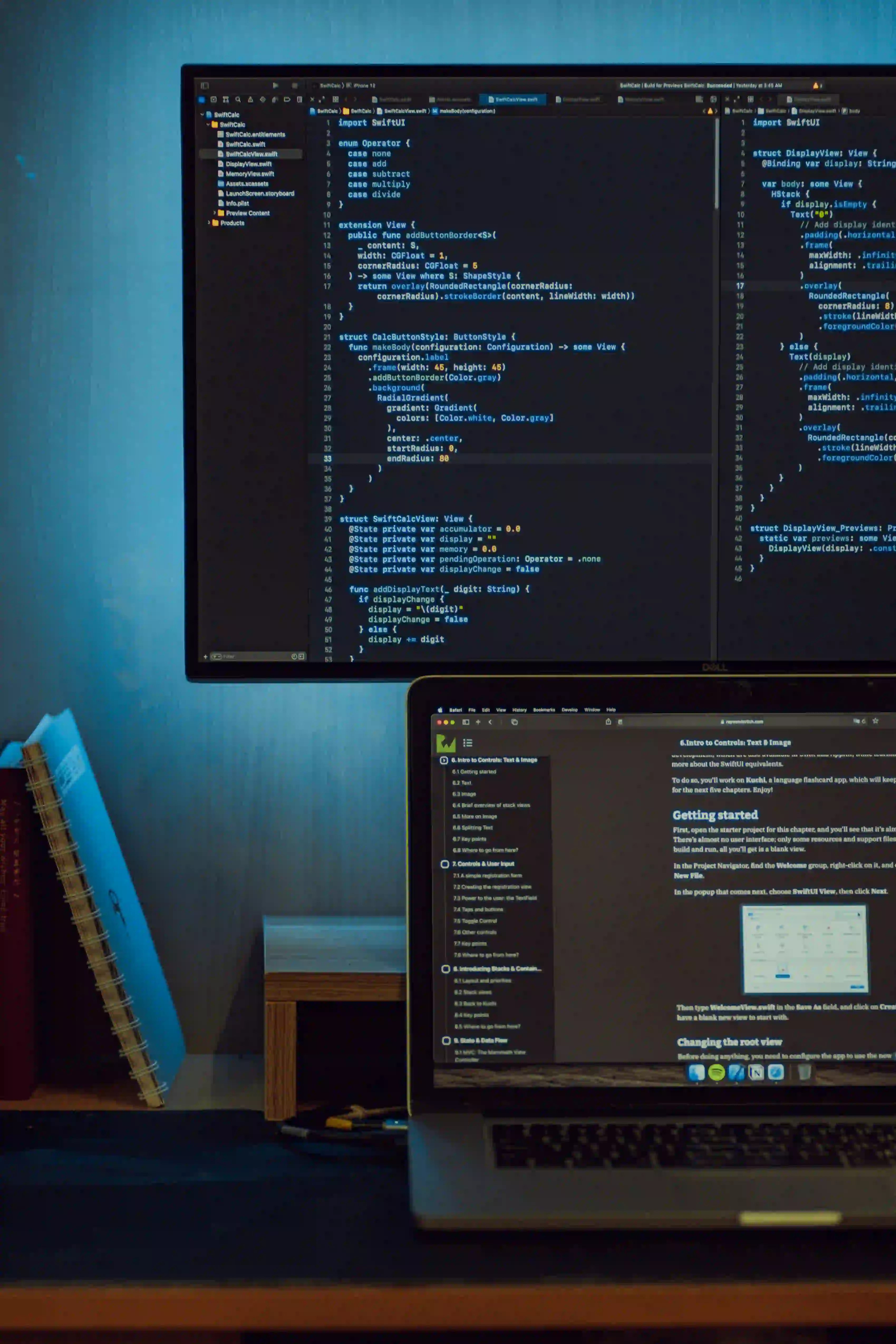
Overcoming the Complexity of Hamcrest Matchers in Testing
Testing is a critical aspect of software development, especially in Java applications. As tests grow in complexity and scope, so does the need for powerful and flexible assertions to validate expected outcomes. Enter Hamcrest — a library that offers a rich set of matchers to facilitate expressive testing.
In this blog post, we will explore the complexity of Hamcrest matchers, demonstrate their usage, and provide tips to simplify testing. We will also highlight best practices for integrating Hamcrest in your Java projects.
What is Hamcrest?
Hamcrest is a library for writing tests in a declarative way. The heart of Hamcrest is its matchers, which allow you to write assertions that read like natural language. This flexibility is valuable when creating unit and integration tests. Rather than using simple assertions that check a single condition, Hamcrest allows you to combine assertions using logical operators, making your tests more expressive.
A Simple Example
Let's start with a common test case in a Java application. Suppose we have a Calculator
class that adds two numbers together.
public class Calculator {
public int add(int a, int b) {
return a + b;
}
}
The traditional Java assertion for testing this functionality might look like this:
import org.junit.Test;
import static org.junit.Assert.assertEquals;
public class CalculatorTest {
@Test
public void testAddition() {
Calculator calculator = new Calculator();
int result = calculator.add(2, 3);
assertEquals(5, result);
}
}
While this is straightforward, it lacks expressiveness. Now, let’s enhance this test with Hamcrest matchers.
import org.junit.Test;
import static org.hamcrest.MatcherAssert.assertThat;
import static org.hamcrest.Matchers.is;
public class CalculatorTest {
@Test
public void testAddition() {
Calculator calculator = new Calculator();
int result = calculator.add(2, 3);
assertThat("The result should be equal to 5", result, is(5));
}
}
Why Use Hamcrest?
- Expressivity: Matchers enhance readability and maintainability.
- Composability: You can combine multiple matchers to create complex assertions.
- Descriptive Failure Messages: When a test fails, Hamcrest provides context for what went wrong.
Understanding Hamcrest Matchers
As your test requirements grow, understanding the various matchers becomes essential. Below are some commonly used Hamcrest matchers.
Basic Matchers
Equality Matchers: is()
, equalTo()
, sameInstance()
assertThat("Checking equality with is", result, is(5));
assertThat("Checking equality with equalTo", result, equalTo(5));
Collection Matchers: hasItem()
, hasSize()
, contains()
import static org.hamcrest.Matchers.*;
@Test
public void testList() {
List<String> names = Arrays.asList("John", "Jane", "Doe");
assertThat("Names list should contain 'Jane'", names, hasItem("Jane"));
assertThat("Names list should have size 3", names, hasSize(3));
}
Combining Matchers
One of the formidable strengths of Hamcrest is combining matchers for enhanced assertions. For example, you can check if a collection contains an item and has expected size simultaneously.
assertThat("Names list should meet both conditions",
names, allOf(hasItem("Jane"), hasSize(3)));
Custom Matchers
While Hamcrest offers numerous built-in matchers, you may find the need for custom matchers. Creating your own matcher can significantly enhance clarity and reusability.
Here's how you might create a custom matcher that checks if a string is a valid email:
import org.hamcrest.Description;
import org.hamcrest.TypeSafeMatcher;
public class IsValidEmail extends TypeSafeMatcher<String> {
@Override
public void describeTo(Description description) {
description.appendText("a valid email address");
}
@Override
protected boolean matchesSafely(String email) {
String emailRegex = "^[A-Za-z0-9+_.-]+@(.+)$";
return email.matches(emailRegex);
}
}
// Usage:
assertThat("Email validation", "example@test.com", new IsValidEmail());
Challenges and Solutions in Implementing Hamcrest
While Hamcrest offers numerous advantages, it can also introduce complexity if not managed properly. Here are some challenges you might face and how to overcome them:
-
Overcomplication with Nested Matchers: Avoid deep nesting. While it’s tempting to create highly intricate assertions, it can create confusion. Break down your assertions into smaller, more manageable tests.
Instead of:
☕snippet.javaassertThat("Complex nested checks failed", resultList, allOf(hasSize(5), hasItem(startsWith("Sample")), everyItem(containsString("Java"))));
Consider creating separate tests for each condition:
☕snippet.javaassertThat("Result size check", resultList, hasSize(5)); assertThat("List should contain a Sample", resultList, hasItem(startsWith("Sample")));
-
Performance Concerns: Extensive use of matchers can slow down test execution. Keep an eye on performance and optimize where necessary, leveraging simpler assertions for straightforward checks.
-
Lack of Familiarity: If your team is new to Hamcrest, the learning curve can be daunting. Organize training sessions or share resources, such as the Hamcrest documentation to get everyone onboard.
Best Practices for Using Hamcrest
- Combine Built-in and Custom Matchers: Use built-in matchers where possible for simplicity. Reserve custom matchers for scenarios where built-in matchers fall short.
- Be Descriptive: Use meaningful and descriptive messages in your assertions. This practice improves readability and makes debugging easier.
- Stay Consistent: Apply Hamcrest consistently across your test suite to keep the testing framework coherent.
- Minimize Complexity: Each test should focus on a single aspect of behavior. Keep your assertions focused to make failures easier to diagnose.
My Closing Thoughts on the Matter
Hamcrest matchers provide a way to bring clarity and expressiveness to your Java tests. While the library brings remarkable flexibility, it can become complex without careful management.
By knowing the ins and outs of Hamcrest, mastering basic and advanced matchers, and implementing best practices, you can improve your testing suite's readability and maintainability. Remember, the goal of testing is not only to verify functionality but also to create a culture of quality in your codebase.
Feel free to dive deeper into the Hamcrest website to learn more about advanced matchers and features. Happy testing!