Overcoming Common Jenkins Pipeline Mistakes for Beginners
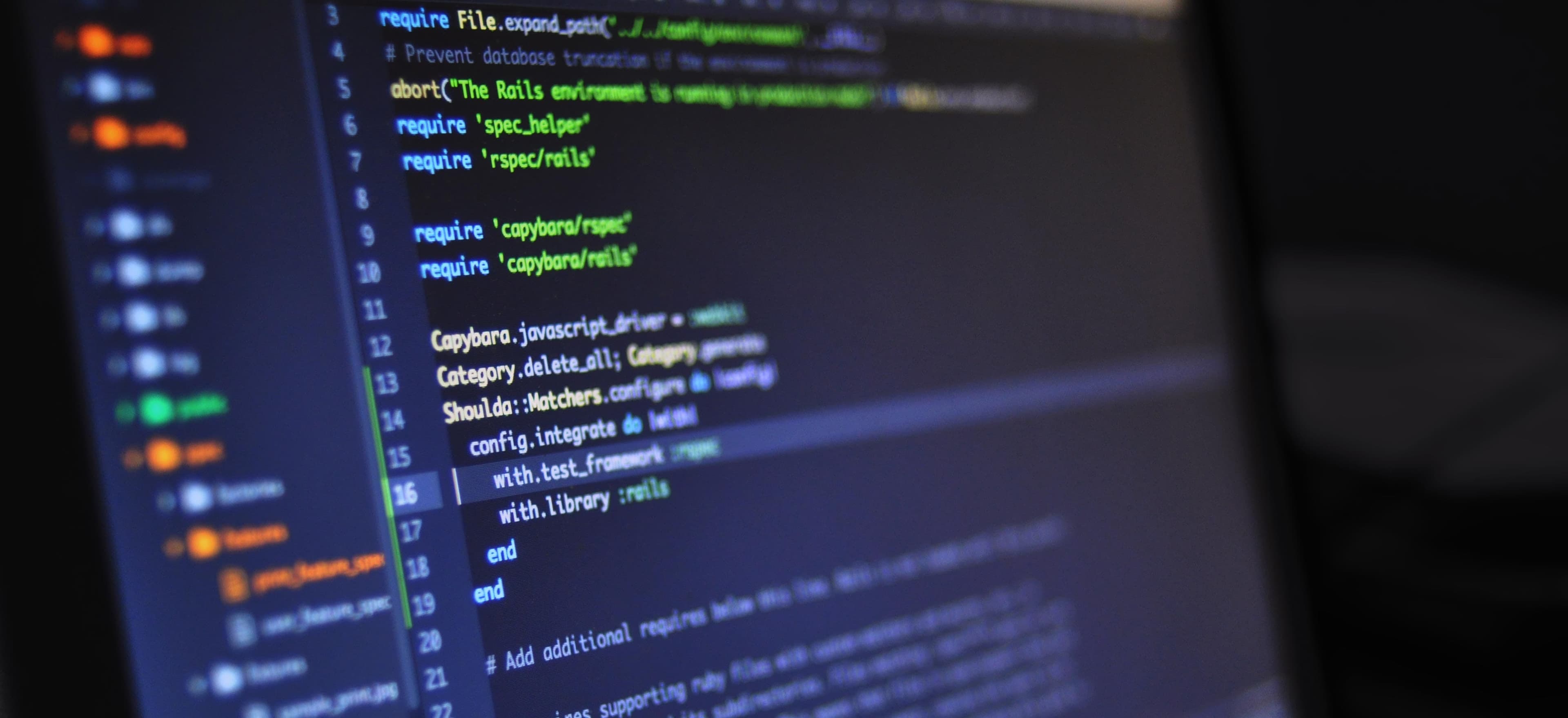
- Published on
Overcoming Common Jenkins Pipeline Mistakes for Beginners
Jenkins is a highly popular open-source automation server that enables developers to build, test, and deploy their software continuously. While utilizing Jenkins can dramatically enhance your development workflow, beginners often stumble through a variety of common pitfalls. This article explores the frequent mistakes in Jenkins Pipelines and offers practical solutions to overcome them.
Understanding Jenkins Pipelines
Jenkins Pipelines are a suite of plugins that support implementing continuous delivery pipelines into Jenkins. A Jenkins Pipeline defines the entire process of building the code and deploying it. Pipelines can be defined as code using a domain-specific language (DSL), which can be stored in your version control system.
Key Attributes of Jenkins Pipelines:
- Declarative Style: Easier to read and maintain.
- Scripted Style: Offers more flexibility and allows for complex operations.
With that foundational knowledge, let’s dive into the common mistakes and how to fix them!
Common Mistakes in Jenkins Pipelines
1. Ignoring Pipeline Syntax
One of the most common mistakes beginners make is not paying attention to the pipeline syntax. A mistake in syntax will lead to a failed build and can be frustrating for new users.
Solution: Use the built-in syntax checker available in Jenkins.
pipeline {
agent any
stages {
stage('Build') {
steps {
echo 'Building...'
}
}
}
}
Why This Matters: The syntax checker helps you identify errors before executing the pipeline, saving you time and effort.
2. Not Using the Right Agent
Another mistake is not specifying a proper agent. Beginners might assume that using "agent any" is sufficient. This could cause issues when specific nodes are required for certain tasks.
Solution: Specify the correct agent based on your build requirements.
pipeline {
agent {
label 'docker-agent'
}
stages {
stage('Build') {
steps {
echo 'Building on Docker agent...'
}
}
}
}
Why This Matters: Selecting an appropriate agent ensures that the correct environment is set up for various operations, leading to more reliable builds.
3. Lack of Error Handling
Many beginners overlook error handling, which can result in pipelines terminating unexpectedly.
Solution: Use try-catch blocks to gracefully manage errors.
pipeline {
agent any
stages {
stage('Test') {
steps {
script {
try {
sh 'run-tests.sh'
} catch (Exception e) {
currentBuild.result = 'UNSTABLE'
echo "Tests failed: ${e}"
}
}
}
}
}
}
Why This Matters: Handling errors appropriately ensures that you can still capture and log crucial failure information, even if a part of your pipeline fails.
4. Hardcoding Values
New users often hardcode parameters in their builds rather than using variables or parameters.
Solution: Utilize environment variables or pipeline parameters to maintain flexibility.
pipeline {
agent any
parameters {
string(name: 'NODE_ENV', defaultValue: 'production', description: 'Environment to deploy to')
}
stages {
stage('Deploy') {
steps {
echo "Deploying to ${params.NODE_ENV} environment"
}
}
}
}
Why This Matters: This makes your pipeline dynamic. You can simply change variables instead of modifying the code, thus improving maintainability.
5. Overlooking Jenkinsfile
A common mistake is neglecting the Jenkinsfile, either by not version-controlling it or misplacing it. This can lead to confusion over which pipeline is actually running.
Solution: Always keep your Jenkinsfile in version control and ensure it is placed in the root of your repository.
Why This Matters: A well-managed Jenkinsfile helps streamline collaboration and allows for maintenance of your pipeline as code.
6. Not Utilizing Multi-Branch Pipelines
Beginners sometimes build their pipeline for a singular branch, ignoring the potential of multi-branch pipelines.
Solution: Leverage the multi-branch pipeline feature to manage different versions or features of your application.
pipeline {
agent any
stages {
stage('Build') {
steps {
echo 'Building for branch: ${env.BRANCH_NAME}'
}
}
}
}
Why This Matters: Multi-branch pipelines facilitate simultaneous development workflows and ensure that different iterations of your project can be tested independently.
7. Forgetting to Cleanup
Lastly, many beginners fail to include cleanup stages, allowing files and resources to pile up which may lead to inefficient builds over time.
Solution: Always include a cleanup stage.
pipeline {
agent any
stages {
stage('Clean') {
steps {
cleanWs()
}
}
stage('Build') {
steps {
echo 'Building...'
}
}
}
}
Why This Matters: Regular cleanup prevents the accumulation of files, freeing up resources and minimizing build failures caused by leftover files.
Learning Resources
For anyone looking to deepen their understanding of Jenkins, I highly recommend checking out the Jenkins official documentation and exploring Jenkins pipeline examples. These resources provide excellent insights and further elaboration on advanced topics.
The Last Word
By addressing these common mistakes, beginners can significantly improve their experience and efficiency within Jenkins Pipelines. Continuous learning and adaptation will not only make you proficient in Jenkins but will also enhance your overall development workflow.
Remember, pipelines are meant to simplify and automate our manual processes. By eliminating these common pitfalls, you set the stage for smoother, more productive development cycles. Happy coding!