Mastering Drools with Camel and Spring: Common Pitfalls
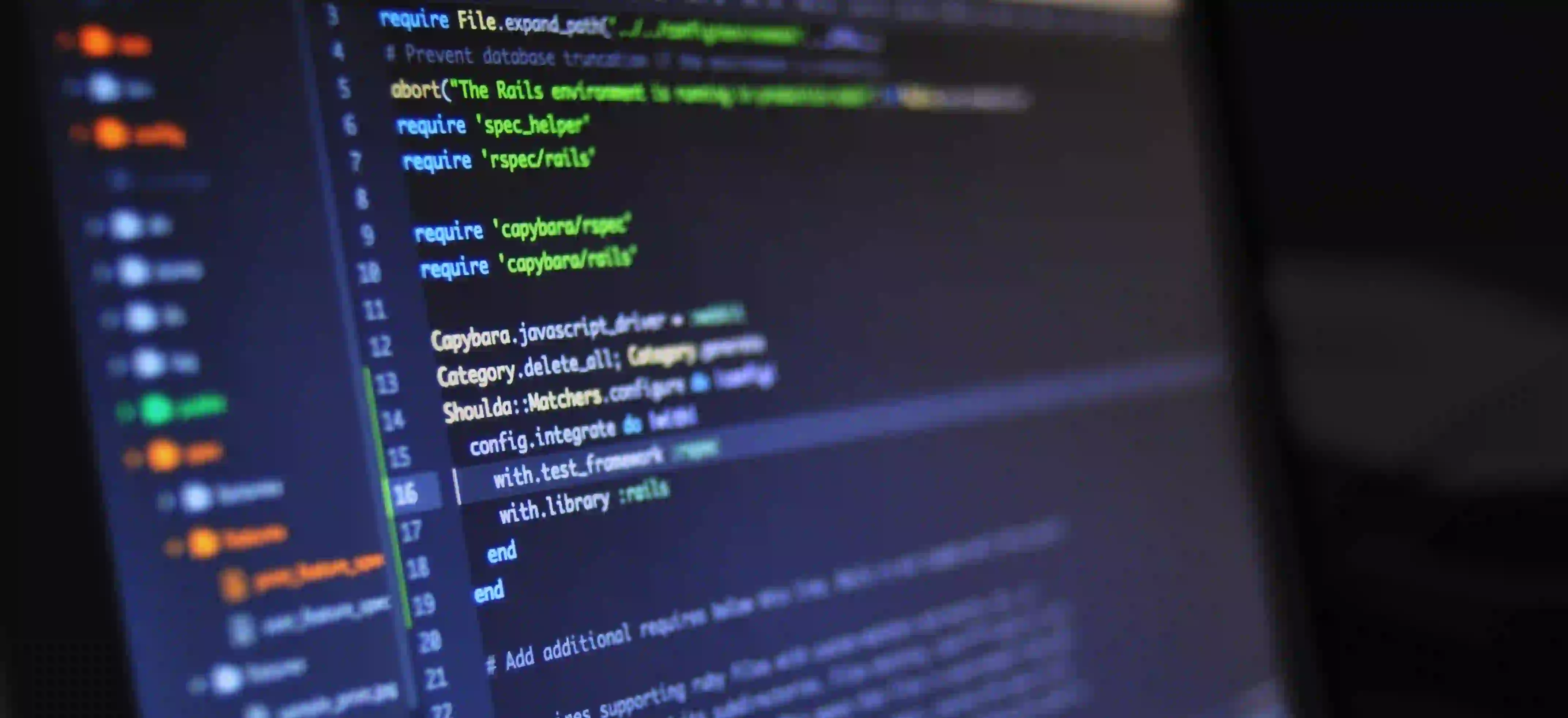
Mastering Drools with Camel and Spring: Common Pitfalls
Drools is a powerful Business Rule Management System (BRMS) that allows you to define, manage, and evaluate business rules within your applications. When integrated with Apache Camel and Spring, it becomes a robust solution that enables dynamic rule execution and complex event processing. However, like any technology stack, there are common pitfalls that developers may encounter. This article aims to address these pitfalls and provide viable solutions to enhance your experience with Drools, Camel, and Spring.
Understanding the Stack
Apache Camel
Apache Camel is an integration framework that enables developers to build applications in a modular way. It simplifies the process of integrating various systems by providing a wide range of connectors and a robust routing engine.
Spring Framework
Spring Framework is a popular framework for building enterprise applications in Java. It offers features such as dependency injection, aspect-oriented programming, and transaction management, making it ideal for creating complex applications.
Drools
Drools is an open-source rule engine that allows for executing rules written in a domain-specific language (DSL). It helps in separating business logic from application code, promoting cleaner, maintainable applications.
Together, these three technologies can create powerful applications, but it is crucial to understand their intricacies to avoid common pitfalls.
Common Pitfalls and Solutions
1. Misconfiguration of Drools Rules
Pitfall: One of the most common mistakes is misconfiguring Drools rules, which leads to unexpected behavior in rule evaluation.
Solution: Always validate your rules. The Drools Workbench offers a way to test your rules in a controlled environment. Use unit tests to validate the logic of your rules before deploying them. Here’s an example of a test class in JUnit:
import org.junit.Test;
import org.kie.api.runtime.KieContainer;
import org.kie.api.runtime.KieSession;
import static org.junit.Assert.assertEquals;
public class SimpleRuleTest {
@Test
public void testSimpleRule() {
KieContainer kieContainer = KieServices.Factory.get().getKieClasspathContainer();
KieSession kieSession = kieContainer.newKieSession("ksession-rules");
// Add a fact to the session
TestFact fact = new TestFact();
kieSession.insert(fact);
// Fire all rules
kieSession.fireAllRules();
assertEquals("Expected output", fact.getOutput());
}
}
Commentary:
This unit test initializes a rule session, inserts a fact, and then fires all rules. Validating your rules through such tests ensures your business logic is functioning as expected before integration into larger applications.
2. Overcomplicated Rule Definitions
Pitfall: Developers often write overly complex rules that can lead to a maintenance nightmare and reduced performance.
Solution: Keep your rules simple. Each rule should have a single responsibility—this makes it easier to read and debug. Here’s a sample rule definition:
rule "Check Discount Eligibility"
when
customer: Customer(isLoyal == true)
then
customer.setDiscount(10);
end
Commentary:
This straightforward rule checks if a customer is loyal and applies a discount. Complicated conditions and actions can lead to hard-to-maintain rules. Always strive for simplicity.
3. Not Using the Camel DSL Effectively
Pitfall: Many developers do not leverage Camel's DSL capabilities, missing out on its powerful routing and integration features.
Solution: Utilize Camel routes to achieve better integration points. For instance:
from("direct:start")
.to("drools:rules/myRules.drl")
.to("log:info");
Commentary:
This route takes messages from a direct endpoint, processes them through the Drools rules engine, and then logs the results. By using Camel's DSL effectively, you can streamline your workflows and improve maintainability.
4. Ignoring Transaction Management
Pitfall: Overlooking transaction management can result in data inconsistencies, especially when dealing with multiple interactions.
Solution: Configure transactions correctly using Spring's transaction management features. For example:
@Configuration
@EnableTransactionManagement
public class AppConfig {
@Bean
public PlatformTransactionManager transactionManager() {
return new DataSourceTransactionManager(dataSource());
}
}
Commentary:
Here, the Spring configuration ensures that transactions are managed throughout the application. This is vital when transactions span multiple service calls or database operations.
5. Lack of Monitoring and Logging
Pitfall: Failing to implement sufficient monitoring and logging can make it difficult to troubleshoot issues in production.
Solution: Implement logging throughout your application to track rule execution and data flow. For example, use SLF4J and Logback for structured log outputs.
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
public class RuleService {
private static final Logger logger = LoggerFactory.getLogger(RuleService.class);
public void executeRules(Fact fact) {
logger.info("Executing rules for fact: {}", fact);
// Rule execution logic
}
}
Commentary:
Logging provides valuable insight into the application’s behavior during rule execution, allowing for easier debugging and monitoring of business logic.
6. Not Provisioning Enough Resources
Pitfall: Underestimating the resource requirements for your rules engine can lead to performance bottlenecks, particularly in high-volume environments.
Solution: Profile and test your application under load to identify resource constraints. Consider scaling up or optimizing your environment as necessary. Utilize Apache Camel's built-in capabilities to create asynchronous routes where appropriate:
from("direct:start")
.async().to("drools:rules/myRules.drl");
Commentary:
Asynchronous processing can help manage high workloads efficiently, providing better response times without bogging down the system.
Lessons Learned
Integrating Drools with Camel and Spring can lead to powerful and flexible applications. However, avoiding common pitfalls is crucial for long-term success. By keeping rules simple, using effective configurations, and implementing proper transaction management, logging, and resource management techniques, you can significantly enhance your development experience.
Further Reading
For more in-depth strategies related to Drools, you can refer to the Drools Documentation and for utilizing Apache Camel, consider reviewing the Camel Documentation.
By mastering these techniques and understanding the common pitfalls, you can leverage the full potential of Drools with Camel and Spring, creating robust and maintainable applications that effectively address your business needs.