Common Pitfalls When Sending Emails with Akka Actors in Java
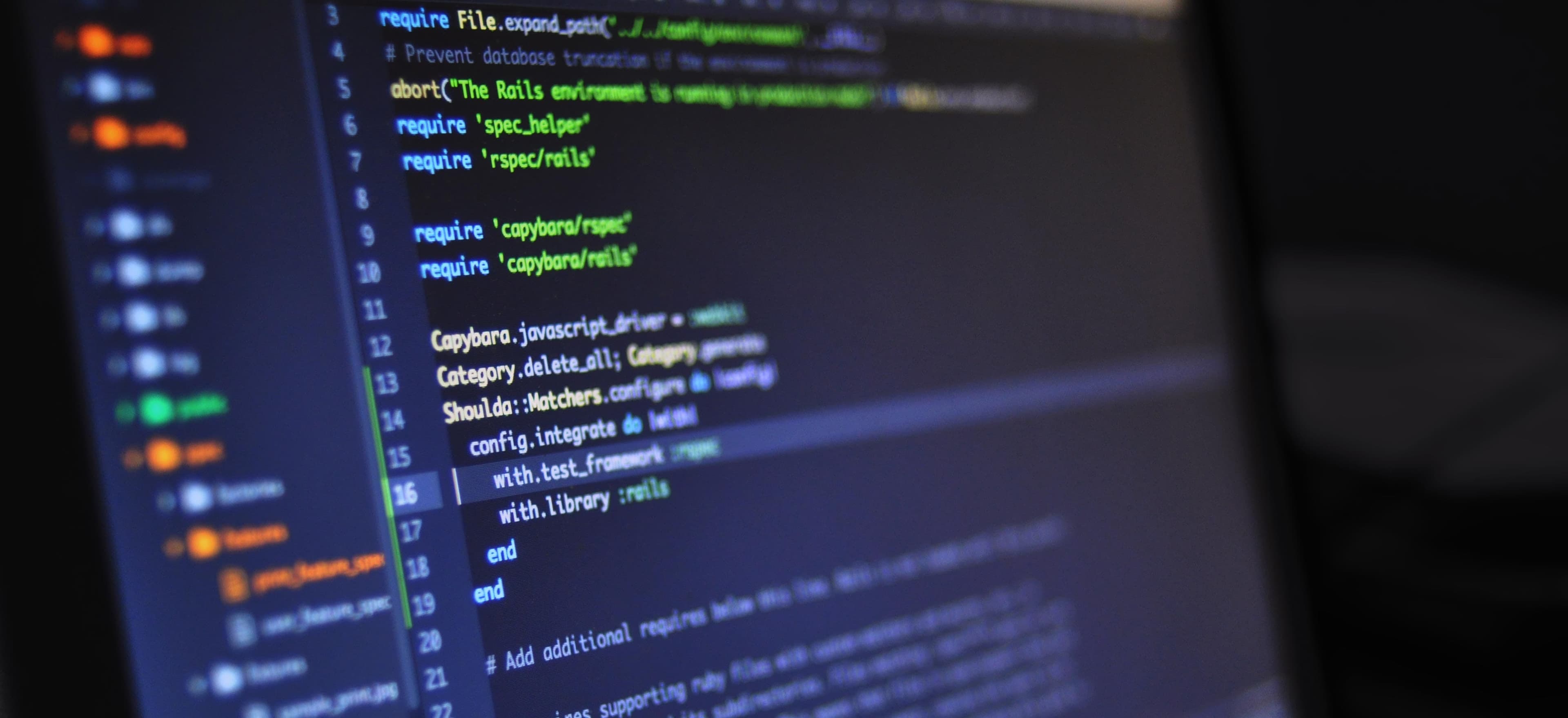
- Published on
Common Pitfalls When Sending Emails with Akka Actors in Java
Email communication is a critical component in many applications. When using Akka actors in Java to send emails, developers must be aware of certain pitfalls that could lead to complications or unexpected behavior. In this article, we will explore those pitfalls, with practical code snippets and explanations to help you avoid them.
Understanding Akka Actors
Before diving into the common pitfalls, let’s briefly understand what Akka actors are.
Akka is a toolkit for building concurrent and distributed applications in Java (and Scala) using the Actor model. Actors encapsulate state and behavior, communicate through messages, and provide an abstraction that helps manage asynchronous programming challenges effectively.
Why Use Akka for Sending Emails?
Using Akka for sending emails allows you to:
- Decouple the email sending process from your application logic.
- Leverage asynchronous processing, which can greatly improve user experience.
- Handle failures gracefully, making your application more robust.
The Basic Setup
To use Akka actors for sending emails, you first need to set up your actor system and the email-sending actor. Let's consider a basic structure:
import akka.actor.AbstractActor;
import akka.actor.ActorSystem;
import akka.actor.Props;
// Define your Actor
public class EmailActor extends AbstractActor {
@Override
public Receive createReceive() {
return receiveBuilder()
.match(Email.class, this::sendEmail)
.build();
}
private void sendEmail(Email email) {
// Email sending logic goes here
System.out.println("Sending email to: " + email.getRecipient());
}
public static Props props() {
return Props.create(EmailActor.class);
}
}
// Email Message POJO
public class Email {
private final String recipient;
private final String subject;
private final String body;
public Email(String recipient, String subject, String body) {
this.recipient = recipient;
this.subject = subject;
this.body = body;
}
// Getters
public String getRecipient() { return recipient; }
}
This code defines an EmailActor
that listens for messages of type Email
and processes them to send an email.
Common Pitfalls and How to Avoid Them
Now that we have a basic structure, let’s delve into the common pitfalls.
1. Blocking Calls in Actors
Pitfall: Sending emails can often involve blocking calls, such as waiting for a response from an SMTP server. If blocking calls are made inside an actor, it can tie up the actor and prevent it from processing other messages.
Solution: Use non-blocking libraries or delegate the email sending to a separate service. For instance, use CompletableFuture for non-blocking calls:
private void sendEmail(Email email) {
CompletableFuture.runAsync(() -> {
// Simulate sending the email
System.out.println("Sending email to: " + email.getRecipient());
try {
Thread.sleep(1000); // Simulating delay for sending an email
} catch (InterruptedException e) {
Thread.currentThread().interrupt();
}
});
}
This allows the actor to remain responsive while the email is being sent.
2. Incorrect Actor Supervision Strategy
Pitfall: If an email sending fails (e.g., due to an invalid email address), the actor may crash if not handled properly.
Solution: Implement a supervision strategy that allows you to manage failures effectively:
@Override
public SupervisorStrategy supervisorStrategy() {
return new OneForOneStrategy() {
@Override
public Directive handle(Throwable throwable) {
if (throwable instanceof EmailSendException) {
// Log the error and resume
return resume();
}
return super.handle(throwable);
}
};
}
This strategy prevents the actor from crashing when an email fails to send, allowing it to continue processing other messages.
3. Lack of Backoff for Failed Retries
Pitfall: If email delivery fails due to temporary issues (e.g., server overload), continuously retrying immediately might lead to overwhelming the server.
Solution: Use a backoff strategy to implement gradual retries:
private void sendEmailWithRetry(Email email, int attempts) {
if (attempts > 0) {
try {
// Simulate sending the email
System.out.println("Sending email to: " + email.getRecipient());
// Simulate potential failure
if (Math.random() > 0.7) {
throw new EmailSendException("Email failed to send");
}
} catch (EmailSendException e) {
// Log and retry
System.out.println(e.getMessage() + ". Retrying...");
getContext().getSystem().scheduler().scheduleOnce(
Duration.create(1000 * attempts, TimeUnit.MILLISECONDS),
getSelf(),
new EmailRetry(email, attempts - 1),
getContext().dispatcher(),
getSelf()
);
}
}
}
Above, we implemented a simple backoff strategy using Akka’s scheduler to retry sending an email, with the wait time increasing with each attempt.
4. Ignoring Exception Handling in Email Service
Pitfall: Not handling exceptions thrown by the email service could lead to crashes in the actor system.
Solution: Always handle exceptions while sending emails:
private void handleSendEmail(Email email) {
try {
sendEmail(email);
} catch (Exception e) {
// Log the exception and notify the sender
System.err.println("Failed to send email: " + e.getMessage());
// Optionally, you can send an error message back to the user
}
}
5. Overloading the Actor System
Pitfall: Sending multiple emails simultaneously can overload the actor system, leading to performance issues.
Solution: Implement rate limiting to control the number of emails sent in a period:
private static final int MAX_EMAILS_PER_MINUTE = 10;
private int emailCount = 0;
public void sendEmail(Email email) {
if (emailCount >= MAX_EMAILS_PER_MINUTE) {
System.out.println("Rate limit exceeded. Email not sent.");
return;
}
emailCount++;
// Schedule a reset of the count
getContext().getSystem().scheduler().scheduleOnce(
Duration.create(1, TimeUnit.MINUTES),
() -> emailCount = 0,
getContext().dispatcher()
);
// Proceed to send the email...
}
This approach keeps your actor responsive while ensuring it does not overwhelm the email server.
Final Considerations
Using Akka actors to send emails in Java can enhance your application’s performance and architecture. However, it is crucial to be aware of common pitfalls that can lead to issues. By leveraging non-blocking calls, proper exception handling, implementing retries with backoff, and managing rate limits, you can create a robust email-sending mechanism in your application.
For further reading, check out Akka Documentation for detailed insights on concurrency and actor management.
Good luck, and happy coding!