Mastering JVM Tuning: Common Pitfalls and Solutions
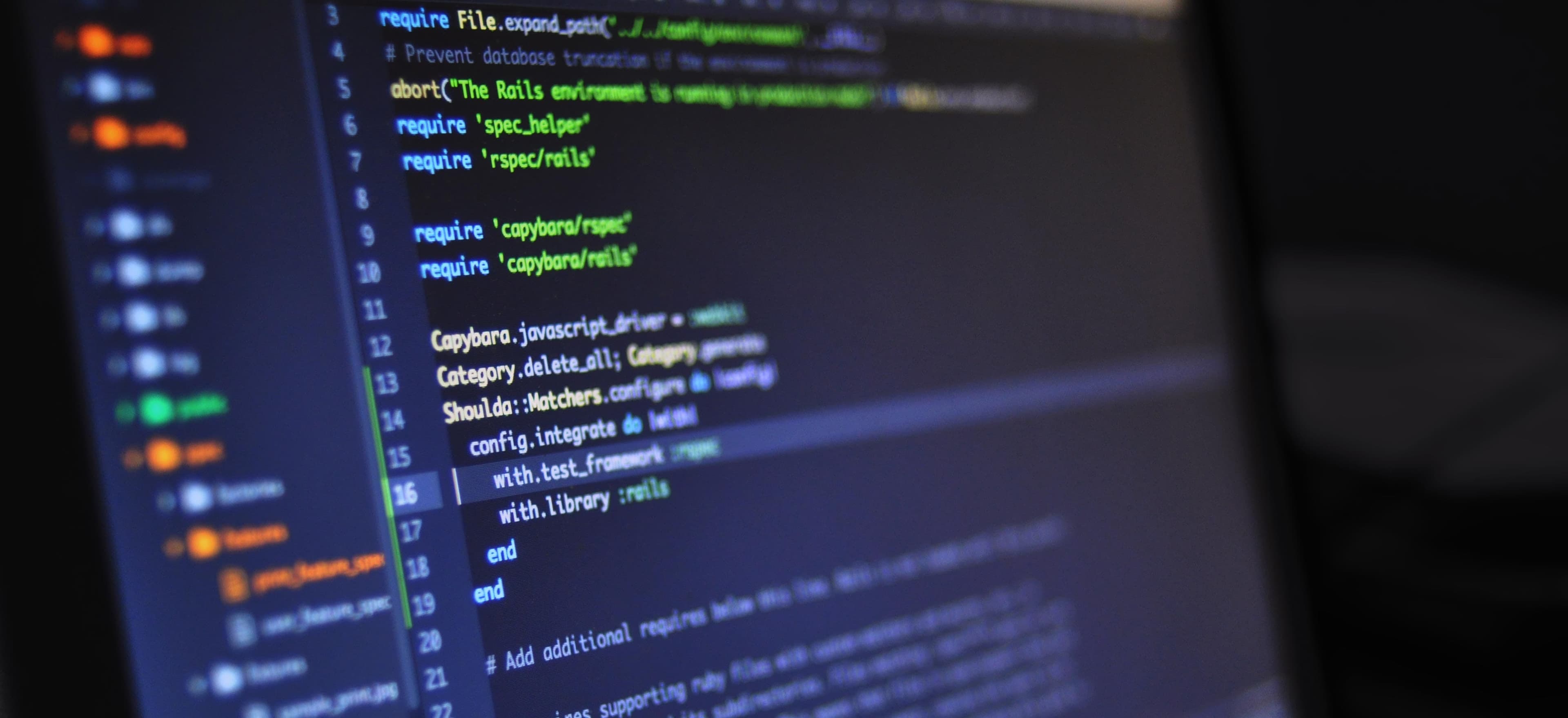
- Published on
Mastering JVM Tuning: Common Pitfalls and Solutions
The Java Virtual Machine (JVM) serves as the backbone of Java applications, influencing their performance and response time significantly. By mastering JVM tuning, developers can ensure their applications operate efficiently, preventing common performance bottlenecks. In this blog post, we'll delve into some common pitfalls encountered during JVM tuning and present actionable solutions to enhance your application's performance.
Understanding the JVM Architecture
Before diving into the intricacies of JVM tuning, it’s crucial to understand its architecture. The JVM consists of several key components, including:
- Class Loader: Loads class files into memory.
- Bytecode Verifier: Ensures that the loaded classes are valid.
- Execution Engine: Executes the bytecode.
- Garbage Collector (GC): Manages memory and object lifecycle.
Understanding these components will guide your tuning efforts as you strive to optimize performance.
Common Pitfalls in JVM Tuning
1. Ignoring GC Logs
One of the most pervasive mistakes developers make is to ignore Garbage Collection (GC) logs. GC logs provide critical insights into memory management and can reveal problematic behavior that affects application performance.
Solution: Enable GC Logging
To get started, enable GC logging by adding the following JVM arguments:
-XX:+PrintGCDetails -XX:+PrintGCDateStamps -Xloggc:gc.log
These flags will log GC events with timestamps, allowing you to analyze the GC behavior over time. Regularly reviewing these logs can help you identify and rectify memory issues before they escalate.
2. Not Sizing the Heap Correctly
Another common mistake is not properly sizing the heap. A heap that is too small leads to frequent garbage collections, while a heap that is too large can cause long pause times during GC.
Solution: Use Heap Size Options
The default heap sizes may not suit your application. To set the initial and maximum heap sizes, use:
-Xms512m -Xmx4g
In this example, the maximum heap size is set to 4 GB while the initial size is set to 512 MB. The sizes should be determined based on your application’s requirements and memory consumption patterns observed in GC logs.
3. Overlooking the Young Generation Tuning
The JVM uses generational garbage collection, which consists of the young generation and the old generation. A common oversight is neglecting to configure the young generation's size, thus impacting minor GC performance.
Solution: Tune the Young Generation Size
You can specify the size of the young generation with:
-XX:NewSize=256m -XX:MaxNewSize=256m
This setup can reduce minor GC frequency for applications that create and collect many short-lived objects.
4. Misunderstanding GC Algorithms
Yet another pitfall is the lack of knowledge about various GC algorithms and when to use them. The default GC algorithm may not provide the best performance for your specific workload.
Solution: Understand and Choose the Right GC Algorithm
Java provides several GC algorithms like Serial, Parallel, CMS (Concurrent Mark-Sweep), and G1 (Garbage-First). You can choose one by appending the argument:
-XX:+UseG1GC
Understanding the characteristics of each GC algorithm will allow you to select one that matches your application's needs. For instance, the G1 GC works well with larger heaps and aims to minimize pause times.
5. Ignoring Thread Configuration
Another area often overlooked is the configuration of threads. Incorrect thread pool sizes may lead to either underutilization or excessive contention, both of which negatively affect performance.
Solution: Optimize Thread Pool Size
Utilize a thread pool library such as Executors
to efficiently manage threads. Here’s a simple example:
import java.util.concurrent.ExecutorService;
import java.util.concurrent.Executors;
public class ThreadPoolDemo {
public static void main(String[] args) {
int numberOfThreads = Runtime.getRuntime().availableProcessors();
ExecutorService executorService = Executors.newFixedThreadPool(numberOfThreads);
for (int i = 0; i < 10; i++) {
executorService.submit(() -> {
// Task execution logic here
});
}
executorService.shutdown();
}
}
By dynamically adjusting the number of threads based on runtime conditions, you can significantly enhance application performance.
6. Overusing Finalizers
Finalizers in Java allow for cleanup operations before an object is garbage collected. However, over-relying on them can lead to performance degradation and unpredictability.
Solution: Avoid Finalizers
Instead of finalizers, consider implementing the AutoCloseable
interface and leverage try-with-resources to ensure timely resource management:
public class Resource implements AutoCloseable {
public void useResource() {
// Use resource logic here
}
@Override
public void close() {
// Cleanup logic here
}
}
public class Main {
public static void main(String[] args) {
try (Resource resource = new Resource()) {
resource.useResource();
}
}
}
7. Neglecting Native Memory Configuration
Native memory involves everything outside the Java heap memory, including memory used by libraries and native threads. Not configuring native memory parameters can create memory leaks.
Solution: Monitor Native Memory Usage
Java provides tools such as Native Memory Tracking (NMT)
to monitor native memory allocations:
-XX:NativeMemoryTracking=summary
Using this feature, you can get insights into memory usage which can help you identify leaks and make appropriate adjustments.
To Wrap Things Up
JVM tuning is essential for optimizing Java applications for performance and reliability. By avoiding these common pitfalls and implementing the solutions outlined above, you'll be better equipped to enhance your application’s efficiency. Remember that every application is unique; what works for one may not work for another. Thus, continuous monitoring and iteration are key to achieving the best performance outcomes.
For more detailed information on JVM tuning, you can explore resources like the Java Performance Tuning Guide or the JVM Garbage Collection Documentation.
Embrace JVM tuning today, and unlock the potential of your Java applications!
Checkout our other articles