Overcoming Slow Integration Tests with Smart Simulation Techniques
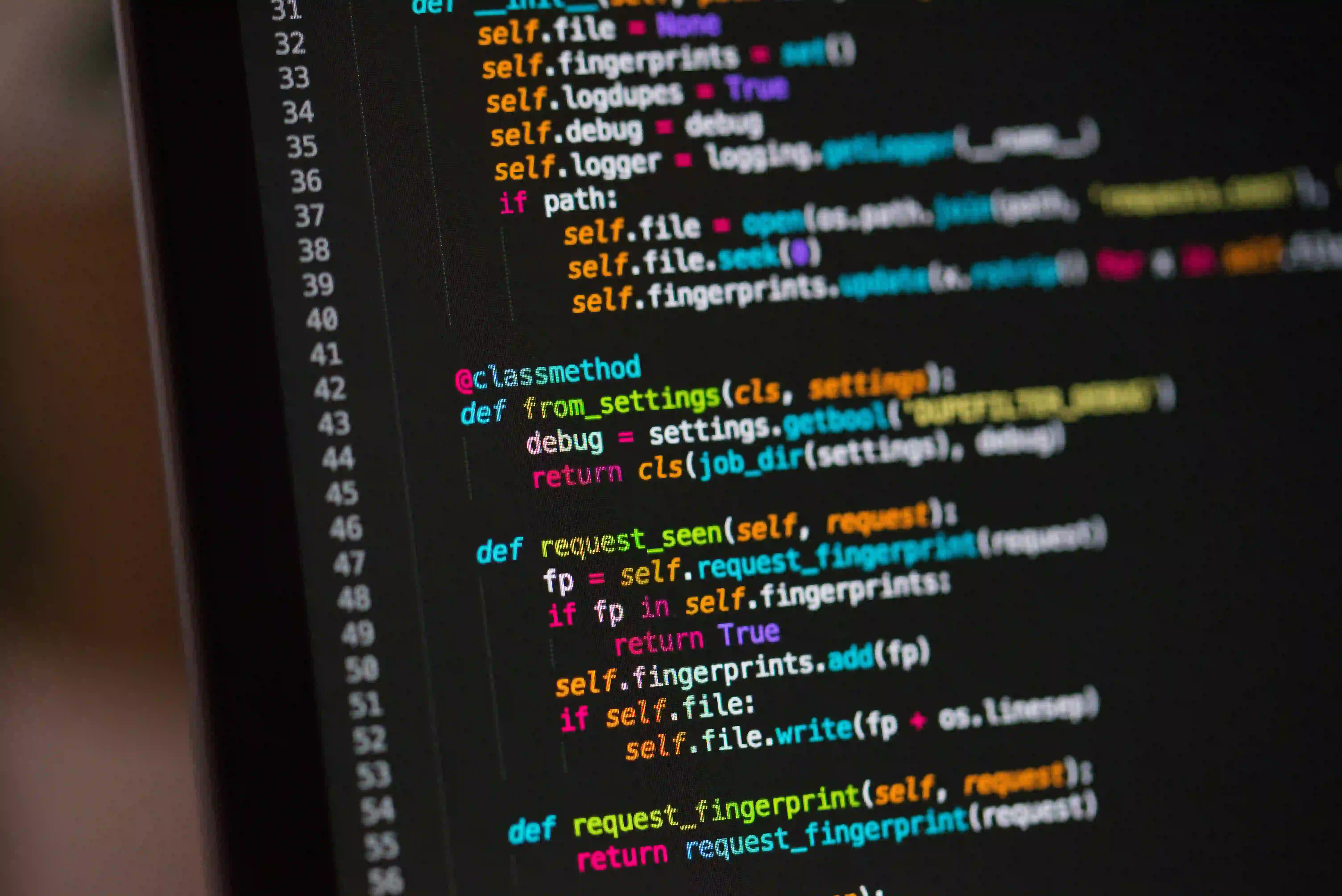
Overcoming Slow Integration Tests with Smart Simulation Techniques
Integration tests are crucial in the software development lifecycle. They ensure that different components of your application work together seamlessly. However, they often come with the cost of extended execution times. In this blog post, we will explore smart simulation techniques that can significantly reduce the time taken for integration tests while still providing comprehensive coverage.
Understanding Integration Tests
Before diving into solutions, let’s briefly discuss what integration tests are. Unlike unit tests that check individual components, integration tests verify the interactions between components or systems. They can involve various stages such as databases, APIs, and external services, making them more complex and time-consuming.
While integration tests are essential for maintaining software quality, they often suffer from slowness due to factors like external service calls and database interactions. Strategies like mocking and simulation can leave you with faster integration tests without compromising on reliability.
Why Use Simulation Techniques?
Simulation techniques help us to mimic the behavior of external systems without actually invoking them. This enables us to:
- Speed Up Test Execution: By reducing the need for real-time calls, we can significantly decrease the execution time of our tests.
- Improve Stability: Mocked components are controllable and predictable, leading to more stable tests.
- Facilitate Resource Management: Simulations can reduce the load on physical resources like databases or APIs.
1. The Basics of Mocking and Stubbing
Mocking and stubbing are common practices in testing that allow you to simulate the behavior of real objects.
- Stubbing: A stub provides predetermined responses to method calls made during the test, without invoking the actual method.
- Mocking: A mock verifies that certain interactions have occurred (like method calls with specific arguments), but it does not provide real implementations.
Example: Mocking with Mockito
Mockito is a widely used framework for creating mocks in Java.
import static org.mockito.Mockito.*;
public class UserServiceTest {
@Test
public void testGetUser() {
UserRepository mockRepository = mock(UserRepository.class);
UserService userService = new UserService(mockRepository);
User testUser = new User("Alice", 30);
when(mockRepository.findById(1)).thenReturn(testUser);
User user = userService.getUser(1);
assertEquals("Alice", user.getName());
verify(mockRepository).findById(1);
}
}
Commentary:
In the example above, we create a mock of the UserRepository
, allowing us to control its behavior during the test of the UserService
. By stubbing findById()
, we ensure that our tests run quickly and deterministically.
2. HTTP Client Simulation
When your integration tests interact with external APIs, you can simulate responses rather than sending actual requests. Libraries such as WireMock or MockServer can be invaluable.
Example: Using WireMock
import static com.github.tomakehurst.wiremock.client.WireMock.*;
import org.junit.After;
import org.junit.Before;
import org.junit.Test;
public class OrderServiceTest {
private WireMockServer wireMockServer;
@Before
public void setup() {
wireMockServer = new WireMockServer(8080);
wireMockServer.start();
configureFor("localhost", 8080);
stubFor(get(urlEqualTo("/api/orders/1"))
.willReturn(aResponse()
.withHeader("Content-Type", "application/json")
.withBody("{\"id\":1,\"product\":\"Laptop\",\"status\":\"shipped\"}")));
}
@Test
public void testGetOrder() {
OrderService orderService = new OrderService("http://localhost:8080/api/orders");
Order order = orderService.getOrder(1);
assertEquals("Laptop", order.getProduct());
}
@After
public void teardown() {
wireMockServer.stop();
}
}
Commentary:
In this example, we start a local WireMock server that returns a predefined JSON response when the order API is called. This allows us to test our OrderService
without actually reaching out to a live server, resulting in much faster and more dependable tests.
3. Database Interaction Simulation
Interacting with a database can slow down integration tests significantly. Instead, consider using in-memory databases like H2 or SQLite or frameworks like Testcontainers for spinning up lightweight, isolated instances of your databases.
Example: Using H2 Database
import org.junit.Test;
import org.springframework.jdbc.core.JdbcTemplate;
import org.springframework.jdbc.datasource.DriverManagerDataSource;
import static org.junit.Assert.*;
public class ProductServiceTest {
@Test
public void testAddProduct() {
DriverManagerDataSource dataSource = new DriverManagerDataSource();
dataSource.setDriverClassName("org.h2.Driver");
dataSource.setUrl("jdbc:h2:mem:testdb");
dataSource.setUsername("sa");
dataSource.setPassword("");
JdbcTemplate jdbcTemplate = new JdbcTemplate(dataSource);
jdbcTemplate.execute("CREATE TABLE products (id INT, name VARCHAR(255))");
ProductService productService = new ProductService(jdbcTemplate);
productService.addProduct(new Product(1, "Smartphone"));
assertEquals(1, productService.getProductsCount());
}
}
Commentary:
Here, we use an H2 in-memory database, which is incredibly fast and allows for self-cleaning between tests. This makes the tests independent and quick.
4. Contract Testing with Pact
Contract testing is a methodology where you define agreements between services, allowing them to evolve independently without breaking integration. Pact is a popular tool for performing contract tests.
Example: Using Pact
import au.com.dius.pact.consumer.junit.PactConsumerTestExt;
import au.com.dius.pact.consumer.junit.PactTestFor;
import au.com.dius.pact.consumer.Pact;
import au.com.dius.pact.consumer.MockServerConfig;
import org.junit.runner.RunWith;
@RunWith(PactConsumerTestExt.class)
@PactTestFor(providerName = "ProductProvider", port = "8080")
public class ProductClientTest {
@Pact(consumer = "ProductClient")
public RequestResponsePact createPact(PactDslWithProvider builder) {
return builder
.uponReceiving("a request for product 1")
.path("/products/1")
.method("GET")
.willRespondWith()
.status(200)
.body("{\"id\":1,\"name\":\"Smartphone\"}")
.toPact();
}
@Test
public void testGetProduct() {
ProductClient client = new ProductClient("http://localhost:$pact.port/products");
Product product = client.getProduct(1);
assertEquals("Smartphone", product.getName());
}
}
Commentary:
In the example above, we use Pact to simulate the expected response for a product request. This allows us to ensure that our consumer service works correctly without depending on the provider.
Best Practices for Integration Test Simulations
-
Keep Tests Independent: Make sure that the simulated components in your tests do not share state, ensuring consistent behavior across test runs.
-
Use Realistic Simulations: Always strive for simulations that mimic real behavior. If your tests are too far removed from reality, they may not catch potential issues.
-
Measure Performance: Regularly measure the performance of your integration tests before and after applying simulations. This helps to quantify the improvement.
-
Review and Refactor: As your application grows, revisit and refactor your tests and simulations. What worked before may not be optimal down the line.
In Conclusion, Here is What Matters
Integration tests are vital for ensuring the resilience of your applications, but their slowness can hinder development cycles. By employing smart simulation techniques, you not only keep tests efficient but also maintain high stability and reliability of your testing suite.
Embrace mocking, stubbing, HTTP client simulations, and contract testing as tools in your testing arsenal. Measure their impact and tailor your approach as your needs evolve. In doing so, you will find a balance between test thoroughness and execution speed, paving the way for quicker deployments and enhanced collaboration across teams.
For further exploration, check out the following resources for more in-depth guidance on testing strategies:
By understanding and implementing these simulation techniques, you can dramatically improve the speed and effectiveness of your integration tests, creating a smoother and more efficient development experience. Happy testing!