Mastering Core Java: Overcoming Common Interview Pitfalls
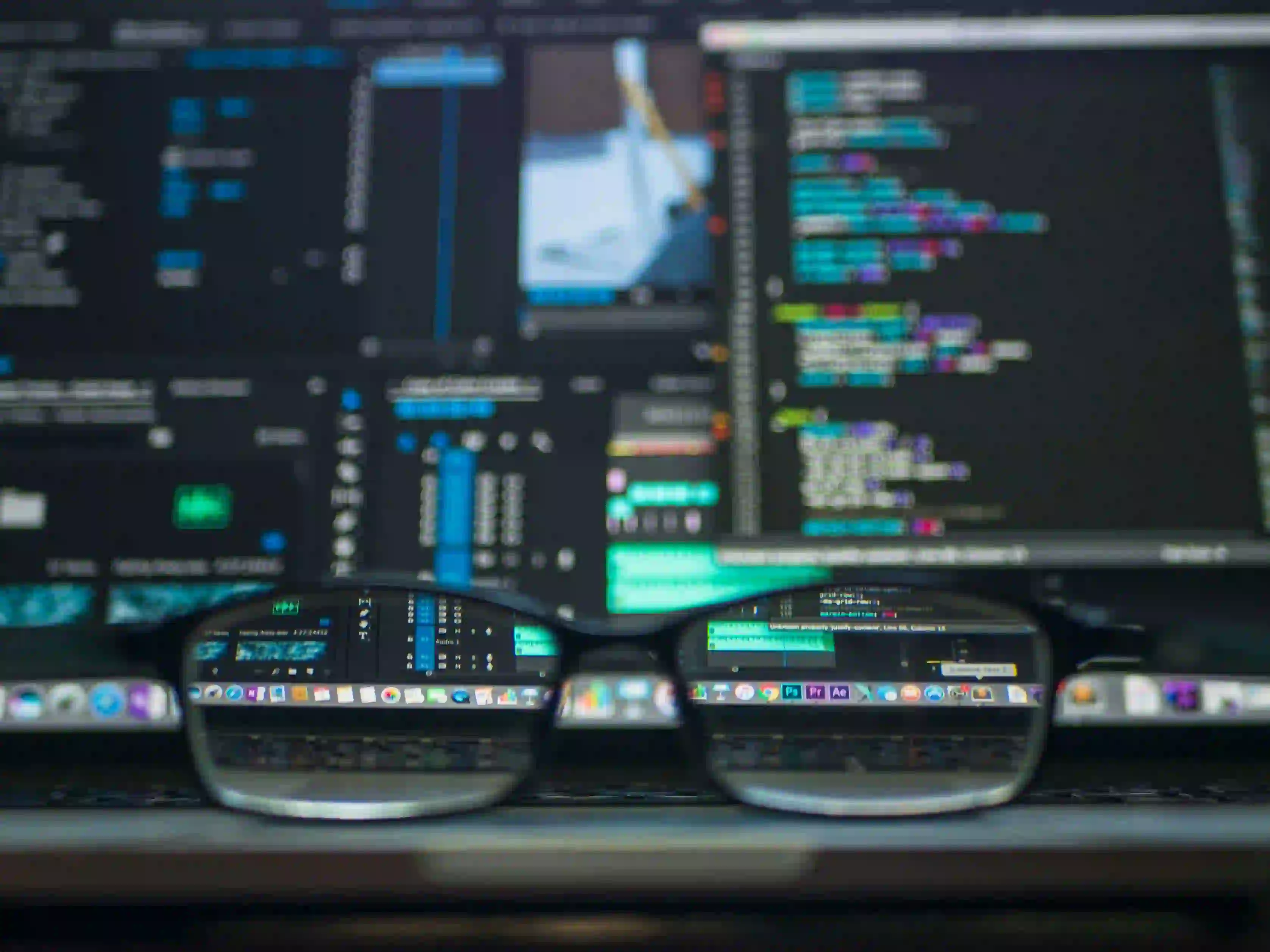
Mastering Core Java: Overcoming Common Interview Pitfalls
Java has long been a staple in the software development industry, and mastering it can significantly boost your career. While Java is lauded for its simplicity and versatility, the reality of mastering it – especially for interviews – can be daunting. In this blog post, we'll explore common pitfalls candidates face during Java interviews and how to overcome them, offering practical tips, code snippets, and resources to enhance your preparation.
Understand the Fundamentals
Before diving into advanced topics, it’s vital to have a strong grasp of core Java concepts. Interviewers often test candidates on their understanding of basic principles, including:
- Object-Oriented Programming (OOP) concepts
- Java Collections Framework
- Exception Handling
- Multithreading
- Java 8 features
Mastering these fundamentals can make a significant difference in your interview performance.
Key OOP Concepts
Understanding OOP is essential in Java as it's a fully object-oriented programming language. The four main pillars of OOP are encapsulation, inheritance, abstraction, and polymorphism.
Let's highlight each with a code snippet:
// Example of Encapsulation
class Employee {
private String name; // private field
public String getName() { // accessor method
return name;
}
public void setName(String name) { // mutator method
this.name = name;
}
}
Why Encapsulation?
Encapsulation helps protect the internal state of an object and ensures that the data is accessed through well-defined methods. This promotes code stability and maintainability.
Navigating Exception Handling
Exception handling is another critical area of focus. Candidates often stumble by either over-simplifying or over-complicating their approaches.
Basic Exception Handling
Here's how you can handle exceptions effectively in Java:
public class ExceptionHandlingExample {
public static void main(String[] args) {
try {
int result = divide(10, 0); // This will throw an exception
} catch (ArithmeticException e) {
System.out.println("Error: " + e.getMessage());
} finally {
System.out.println("Execution completed.");
}
}
public static int divide(int a, int b) {
return a / b;
}
}
Why Use Exception Handling?
Using try
, catch
, and finally
blocks, you can manage errors gracefully without crashing your application. This also demonstrates good programming practices to an interviewer.
Collections Framework: Knowing your Tools
Java’s Collections Framework is one of its strongest features. Familiarity with collections, particularly when to use which container, can set you apart in interviews.
Example: List vs. Set
import java.util.*;
public class CollectionExample {
public static void main(String[] args) {
List<String> list = new ArrayList<>();
list.add("Apple");
list.add("Banana");
list.add("Apple"); // Duplicate elements allowed
Set<String> set = new HashSet<>();
set.add("Apple");
set.add("Banana");
set.add("Apple"); // Duplicate will not be added
System.out.println("List: " + list);
System.out.println("Set: " + set);
}
}
Why Choose Between List and Set?
Understanding the differences helps in selecting the right collection type based on your requirements for duplicates, ordering, and access time.
Multithreading: Concurrency and Challenges
Multithreading can be a tricky subject and is often a focal point in interviews. Address the common pitfalls by familiarizing yourself with thread lifecycles, synchronization, and pitfalls like deadlock.
Simple Thread Example
class MyThread extends Thread {
public void run() {
System.out.println("Thread " + this.getName() + " is running!");
}
}
public class ThreadExample {
public static void main(String[] args) {
MyThread thread1 = new MyThread();
MyThread thread2 = new MyThread();
thread1.start();
thread2.start();
// Join threads to wait for their completion
try {
thread1.join();
thread2.join();
} catch (InterruptedException e) {
System.out.println("Thread interrupted: " + e.getMessage());
}
}
}
Why Use Threads?
Multithreading enhances performance by utilizing CPU resources effectively, especially for I/O-bound tasks. Interviewers are keen to see if you can strike a balance between ease of use and performance optimization.
Java 8 Features: Streams and Lambda Expressions
Java 8 introduced significant features like streams and lambda expressions that simplify programming and improve performance. Interviews frequently explore these concepts.
Using Streams
import java.util.Arrays;
import java.util.List;
public class StreamExample {
public static void main(String[] args) {
List<String> names = Arrays.asList("John", "Jane", "Jack", "Jill");
names.stream()
.filter(name -> name.startsWith("J"))
.forEach(System.out::println);
}
}
Why Use Streams?
Streams support functional-style operations on collections, allowing you to express complex queries concisely. This shows your ability to write expressive and performant code.
Practice Makes Perfect
Regular practice is key to overcoming interview hurdles. Leverage platforms like LeetCode or HackerRank to solve Java problems and familiarize yourself with interview patterns.
Understanding Design Patterns
Design patterns offer proven solutions to common problems. Familiarity with patterns like Singleton, Factory, and Observer can provide a significant edge in interviews.
Singleton Pattern Example
public class Singleton {
private static Singleton instance;
private Singleton() {
// private constructor to restrict instantiation
}
public static Singleton getInstance() {
if (instance == null) {
instance = new Singleton();
}
return instance;
}
}
Why Use the Singleton Pattern?
This pattern ensures that a class has only one instance while providing a global access point to it. It demonstrates your understanding of design principles.
Non-Technical Preparation
While technical skills are crucial, non-technical factors, such as communication and soft skills, play a vital role in interviews as well. Practicing mock interviews and explaining your thought process clearly can greatly improve your performance.
My Closing Thoughts on the Matter
In summary, mastering Core Java requires a solid understanding of the language and its features, regular practice, and awareness of common pitfalls. By focusing on OOP concepts, exception handling, collections, multithreading, Java 8 features, and design patterns, you can enhance your interview readiness.
Embrace the journey of learning and use the above strategies to conquer your next Java interview. Remember, the best preparation combines knowledge, practice, and confidence.
For further reading on Java fundamentals, consider exploring resources on Oracle's Java Documentation or engaging with community discussions on platforms like Stack Overflow.
Happy coding and good luck with your interviews!