Why Good Code Breaks: Unraveling the Mystery
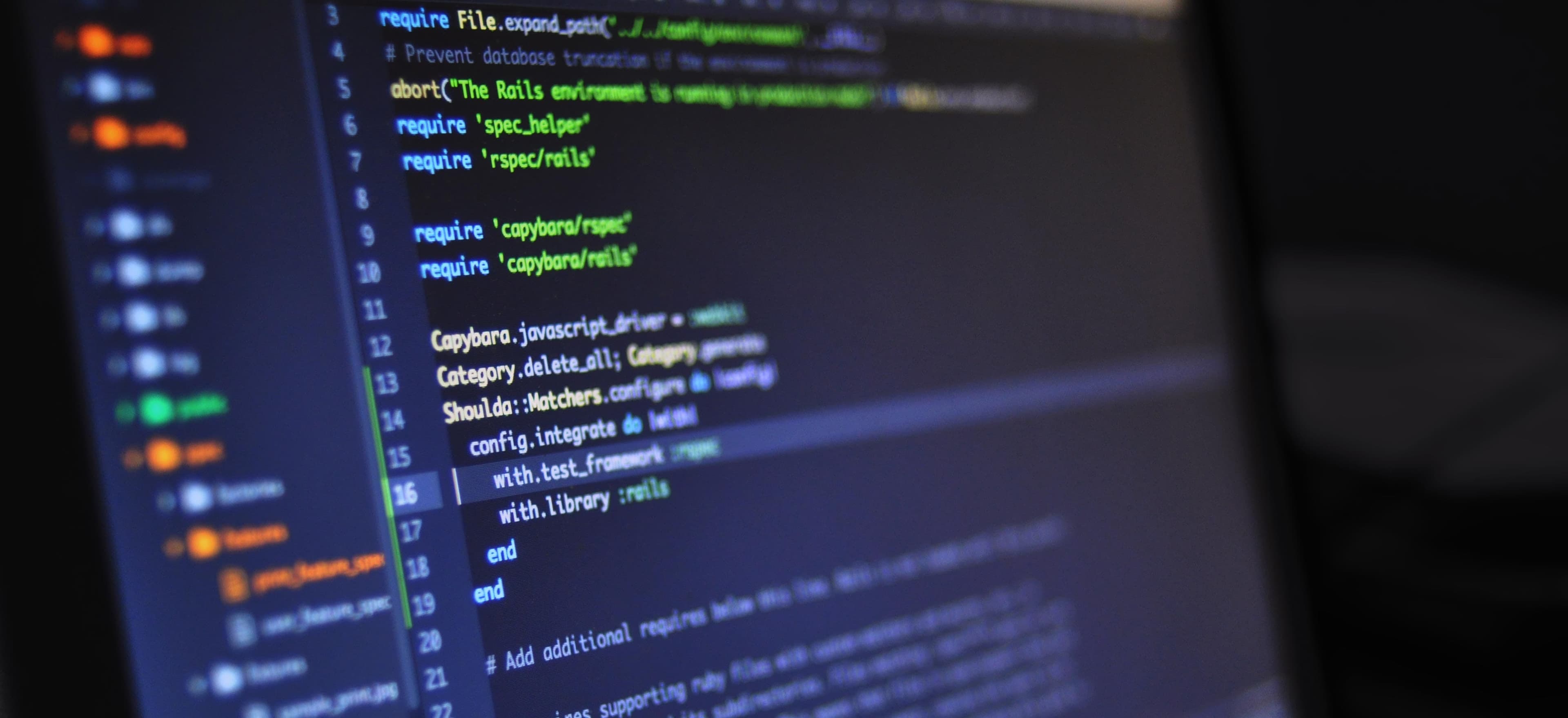
- Published on
Why Good Code Breaks: Unraveling the Mystery
In the world of software development, the conversation often revolves around writing good, maintainable code. However, what frequently comes into play, albeit under-discussed, is the paradox of why seemingly good code tends to break. In this post, we’ll dive into this mystery, examining the factors that contribute to code failure and providing insights to help mitigate these risks.
Table of Contents
- Understanding Good Code
- Common Reasons Code Breaks
- Importance of Testing
- Best Practices for Writing Resilient Code
- Conclusion
Understanding Good Code
Good code is often defined by characteristics such as clarity, efficiency, and maintainability. It is structured in such a way that it can be easily understood by others, effectively addresses a problem, and can be adapted to changes in requirements. However, good code does not exist in a vacuum. Various external pressures and internal factors can compromise its functionality.
The Challenge of Perception
Just because code is well-written doesn’t guarantee it will perform correctly under all circumstances. The software development community often emphasizes principles like DRY (Don't Repeat Yourself) and KISS (Keep It Simple, Stupid), yet even the best practices have limitations.
Common Reasons Code Breaks
1. Poor Communication
Communication among team members, including developers, product owners, and stakeholders, is crucial. Misalignment about requirements or misunderstandings can lead to implementing the wrong functionality.
Example:
public class Calculator {
public int add(int a, int b) {
return a + b; // Simple addition
}
// What if someone wanted to add float numbers?
}
In this example, the method add
lacks versatility due to uncommunicated requirements about supporting different data types. A better approach could involve using generic types or overloading the method.
2. Changing Requirements
Agile methodologies emphasize flexibility, but constant changes can create instability. Each modification requires updates to existing code, which can lead to unintended consequences.
Example:
public class User {
private String username;
// Getter and setter methods omitted for brevity
}
// Later, a requirement change demands we store emails too.
If the initial design doesn’t account for such changes, the developer may need to rush through changes, leading to code that may not uphold previous standards.
3. Lack of Documentation
Good code often exists alongside sound documentation. Without documentation, even the clearest code can confuse future maintainers.
Example:
public void calculateDiscount(Customer customer) {
// apply some logic here
}
Without information regarding what "some logic" entails, another developer will struggle to understand the decision-making process. This lack of context can lead to incorrect assumptions or further modifications that break the original logic.
4. Technical Debt
Technical debt is a natural part of software development. Often, developers may opt for shortcuts to meet deadlines. However, the cost of these shortcuts accumulates over time.
Example:
// Using a deprecated API for quick implementation
String userToken = oldAuthService.getToken(username);
Using outdated methods like this can lead to breaking changes when those APIs are eventually removed. Refactoring the code sooner can effectively minimize this type of debt.
Importance of Testing
Testing is an integral component of maintaining code integrity. Various types of tests, from unit tests to integration tests, help identify breakage before deployment.
Example of Simple Unit Test in Java
import static org.junit.jupiter.api.Assertions.assertEquals;
import org.junit.jupiter.api.Test;
public class CalculatorTest {
@Test
public void testAddition() {
Calculator calc = new Calculator();
assertEquals(5, calc.add(2, 3)); // Basic test case
}
}
This unit test ensures the add
function works correctly. Without such tests, changes made later may inadvertently alter this behavior, leading to regressions.
Best Practices for Writing Resilient Code
1. Code Reviews
Implementing regular code reviews encourages knowledge sharing and catches inconsistencies early in the development cycle.
2. Refactoring
Encourage regular refactoring sessions. Code should not become stagnant; adjustments allow it to evolve with changing requirements.
3. Clear Documentation
Maintain clear and concise documentation that gives context to your code. A well-documented codebase reduces the complexity when new developers come on board.
4. Embrace Testing
Incorporate testing as part of the development process. Automated tests should cover as much of the codebase as possible.
5. Use Version Control
Utilize version control systems like Git to keep track of changes. This practice allows for easy rollbacks if new code breaks existing functionality.
Key Takeaways
The reason good code breaks is multifaceted, often stemming from poor communication, changing requirements, lack of documentation, and the inevitable accumulation of technical debt. Understanding these underlying factors can help developers take proactive measures to minimize the likelihood of encountering breakage.
By employing best practices such as thorough testing, excellent documentation, and code reviews, developers can enhance code resilience. Remember, good code doesn’t live in isolation; it thrives in a collaborative, well-communicated, and rigorously tested environment.
For those interested in learning more about resilient coding practices and methodologies, consider exploring Clean Code Principles or Agile Software Development.
To ensure you don’t miss the latest insights and trends in development, subscribe to our newsletter or follow our blog for upcoming posts!
Checkout our other articles