Common Mistakes Every Programmer Makes and How to Avoid Them
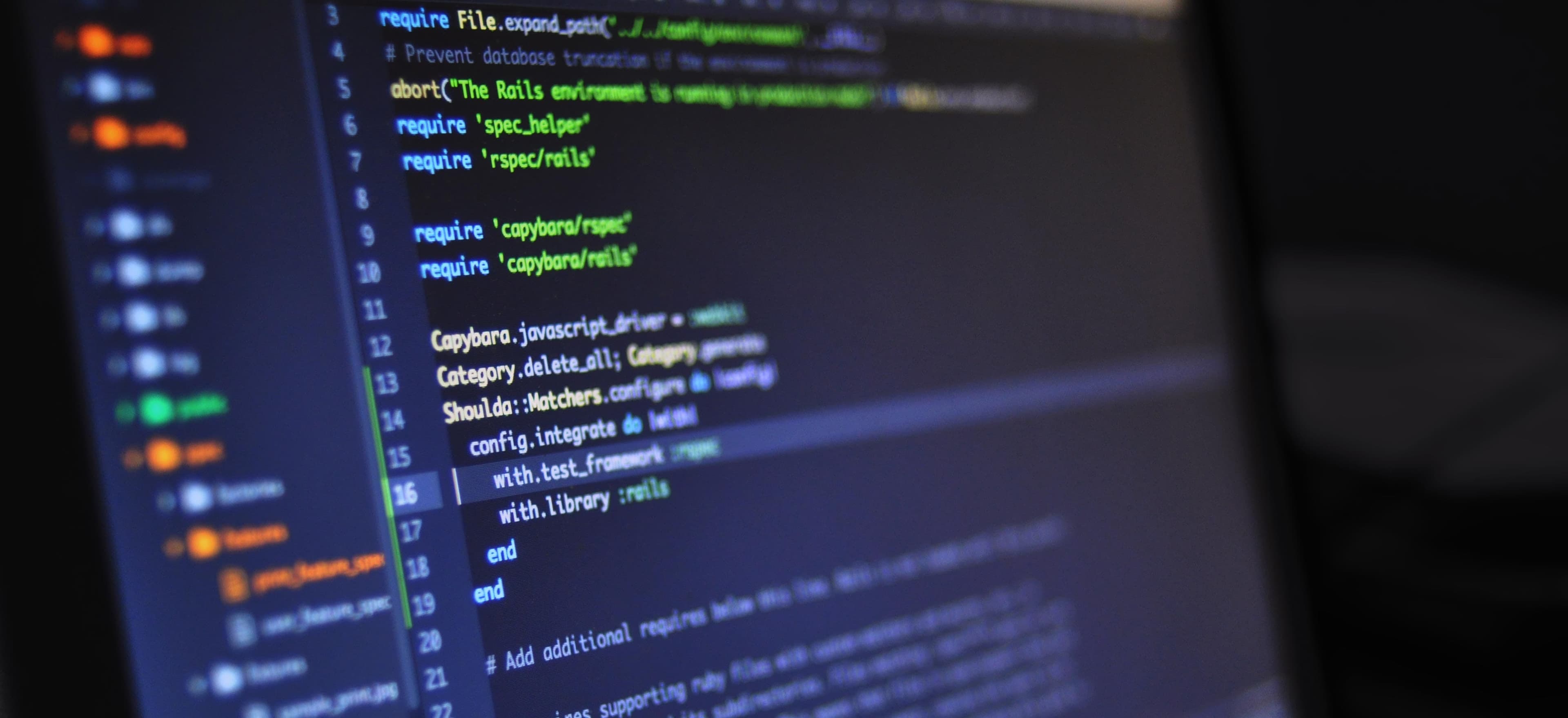
- Published on
Common Mistakes Every Programmer Makes and How to Avoid Them
Programming is an intricate blend of creativity, logic, and problem-solving. Regardless of experience, every programmer—a beginner or a seasoned developer—will inevitably encounter some preventable mistakes along the journey. While the learning process is essential, acknowledging these common pitfalls and understanding how to avoid them can enhance efficiency and produce cleaner, more reliable code.
1. Ignoring Documentation
The Importance of Clear Documentation
One of the most pervasive mistakes developers make is neglecting documentation. Whether it's internal documentation within your code or external documentation for user manuals, clarity and completeness are paramount.
/**
* Calculates the factorial of a number.
* @param n the number to calculate the factorial for
* @return the factorial value of n
*/
public int factorial(int n) {
if (n < 0) {
throw new IllegalArgumentException("Number must be non-negative");
}
return (n == 0) ? 1 : n * factorial(n - 1);
}
Why document at all? Good documentation helps not only you but also your colleagues. When you revisit code after a few months or pass it to someone else, clear comments provide essential insights about functionality and usage.
How to Avoid This Mistake
- Use descriptive comments: Avoid sparse or generic comments. Describe “why” rather than “what.”
- Keep it updated: As your code evolves, ensure that documentation is adjusted accordingly.
For more tips on writing effective documentation, check out this guide.
2. Overlooking Code Readability
Clarity over Cleverness
Another common mistake is writing clever but convoluted code. While it can be tempting to show off with clever one-liners, readability should always take priority.
// Bad readability
public void checkEven(int n) {
if (n % 2 == 0) System.out.println("Even");
else System.out.println("Odd");
}
// Improved readability
public void checkEven(int n) {
String result = (n % 2 == 0) ? "Even" : "Odd";
System.out.println(result);
}
Why prioritize readability? Clean code allows for easier maintenance and debugging, which ultimately saves time.
How to Avoid This Mistake
- Follow naming conventions: Variable and method names should be descriptive. For instance, use
totalCost
instead oftc
. - Break complex methods: If a method is too long or does too much, consider dividing it into smaller, manageable functions.
3. Not Version Controlling Your Code
The Perils of Improvisation
Failing to use version control systems such as Git is akin to sailing without a map. Without proper tracking of changes, it becomes almost impossible to manage various iterations of your code effectively.
Essential Git Commands
git init # Initializes a new Git repository
git add . # Adds all changes to the staging area
git commit -m "Initial commit" # Commits changes with a message
git branch # Shows current branches
git checkout -b new-feature # Creates and checks out to a new branch
Why is version control important? It allows you to revert to previous versions, collaborate with others seamlessly, and track progress over time.
How to Avoid This Mistake
- Start with a small project: Familiarize yourself with Git basics.
- Commit frequently: Capture meaningful checkpoints in your development process.
For an in-depth understanding, visit the official Git documentation.
4. Failure to Test
Testing as a Priority
Many programmers, particularly beginners, underestimate the importance of testing. Skipping this step can lead to unforeseen bugs and production issues.
Example of a Simple Unit Test
import org.junit.jupiter.api.Test;
import static org.junit.jupiter.api.Assertions.assertEquals;
public class CalculatorTest {
@Test
public void testAdd() {
Calculator calc = new Calculator();
assertEquals(5, calc.add(2, 3));
}
}
Why run tests? They act as safety nets. By running unit tests before deployment, you catch vulnerabilities early in the development process.
How to Avoid This Mistake
- Write automated tests: Create Unit Tests or integration tests that get run regularly.
- Incorporate testing early on: Adopt a Test-Driven Development (TDD) approach to ensure you consider tests from the outset.
5. Not Seeking Help
The Myth of the Lone Genius
Assuming that you can solve every problem on your own is a common misconception among programmers. Remember, programming communities are vast and equipped with resources that can help you troubleshoot and learn.
Finding Help Efficiently
- Online Forums: Participate in platforms like Stack Overflow for quick queries.
- Local Meetups: Engage with local programming groups for networking and support.
Getting acquainted with the broader community can provide new perspectives and solutions that you may not have considered alone.
How to Avoid This Mistake
- Ask specific questions: When seeking help, clarify your problem statement.
- Encourage collaboration: Discuss challenges with peers and learn from their approaches.
6. Rushing Development
The Trap of Speed Over Quality
Impatience can lead to the rush in development, which often results in sloppy work and oversight of crucial factors such as code quality and efficiency.
Emphasizing Quality
public List<String> getUsers(List<User> users) {
return users.stream()
.filter(user -> user.isActive())
.map(User::getName)
.collect(Collectors.toList());
}
Why take your time? Engaging in thoughtful coding practices embeds quality into your work, laying the foundation for lasting solutions.
How to Avoid This Mistake
- Plan your development: Spend adequate time in the design stage.
- Review and refactor: Regularly revisit your code for improvements.
7. Lack of Consideration for Scalability
Thinking Ahead
Ignoring scalability can lead to significant pitfalls when your program's usage grows. Building code that cannot scale appropriately limits future enhancements.
Designing for Scalability
public class UserProfile {
private List<Post> posts;
public void addPost(Post post) {
this.posts.add(post);
}
}
The above structure directly associates users with their posts; however, this approach may hinder performance if the posts
list grows substantially. A more robust solution would be to leverage database relationships.
How to Avoid This Mistake
- Anticipate user growth: Design with flexibility in mind, allowing for easy modifications or enhancements.
- Utilize appropriate technologies: Consider frameworks or databases that suit large-scale applications.
Closing the Chapter
To sum it up, common pitfalls in programming are easy to fall into, but they can be addressed with attention and practice. Engaging in good documentation practices, prioritizing code readability, harnessing the power of version control, rigorously testing your code, seeking assistance, managing time wisely, and planning for scalability will lead you to improved coding practices and overall better performance.
Continuously learning from your mistakes will not only enhance your skills but also foster a positive coding culture that benefits everyone in the long run. Embrace the journey, and happy coding!
For further reading, check out articles discussing better coding practices that delve deeper into any of these pitfalls.