Overcoming Twitter API Limits with Spring Social
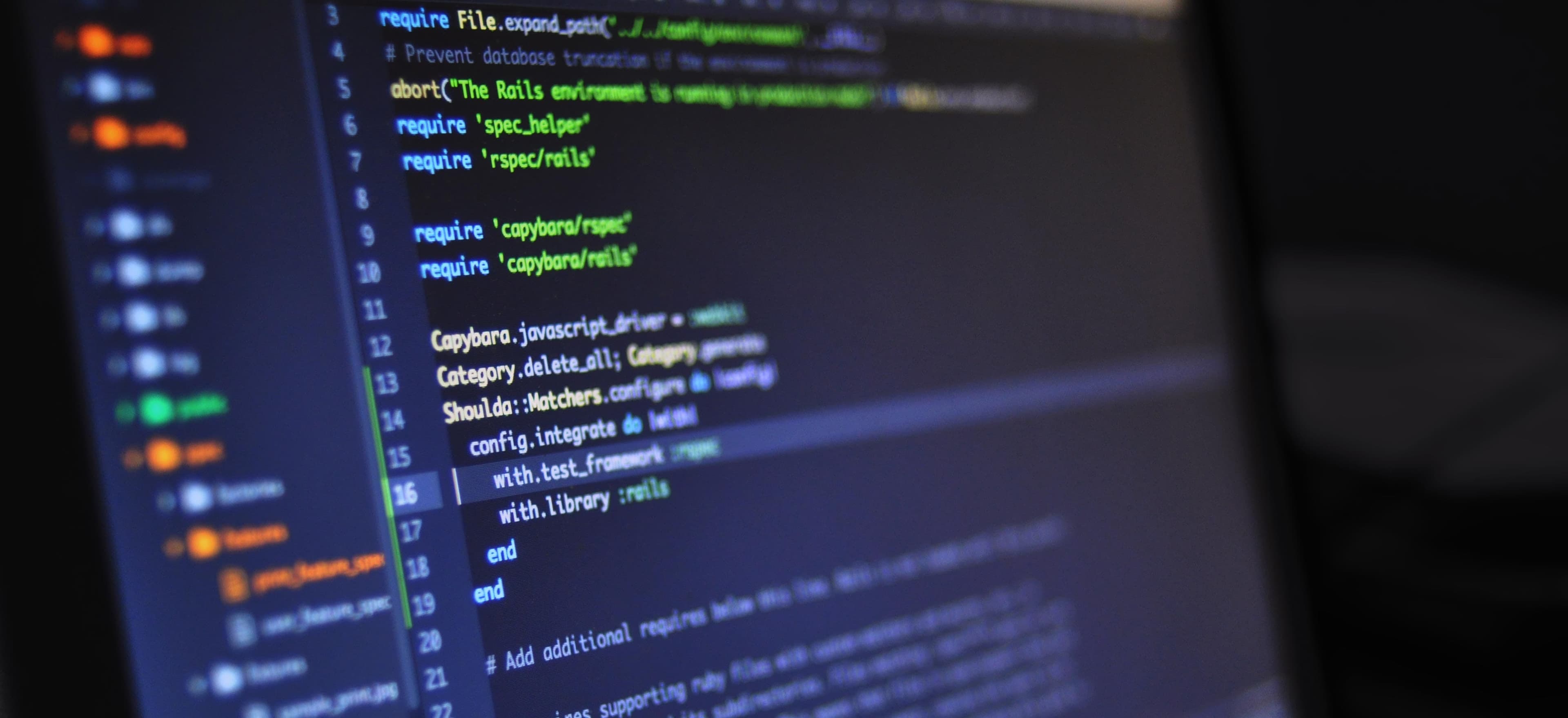
- Published on
Overcoming Twitter API Limits with Spring Social
In today's fast-paced digital environment, social media platforms, particularly Twitter, serve as vital tools for communication and business marketing. However, utilizing these platforms for application development comes with its own set of challenges. One such challenge developers often face is API rate limits. In this blog post, we will explore how to overcome Twitter API limits using Spring Social, a powerful tool for integrating social media networking into Java applications.
Understanding Twitter API Rate Limitations
Before we delve into solutions, it's essential to understand what Twitter's API rate limit entails. Twitter places rate limits on its API to ensure fair usage across its platform. These limits restrict the number of requests an application can make within a specific time window. For example, a typical limit might allow a user to make 900 requests every 15 minutes.
Failing to adhere to these limits can lead to API access restrictions, rendering your application unusable until your limit resets. Therefore, it’s crucial to design your application with these limitations in mind.
Why Spring Social? Spring Social provides a streamlined way to interact with social APIs. It abstracts the complexity of OAuth authentication, exposing simple APIs to handle Twitter endpoints effortlessly. Additionally, it provides resource templates that help you configure and use Twitter services correctly.
For more in-depth information about Twitter's API limits, you can check the Twitter API Rate Limits documentation.
Setting Up Spring Social for Twitter
To begin, ensure your Java development environment is set up with Spring Boot. You can create a new Spring Boot project using Spring Initializr or your preferred IDE. Include the following dependencies in your pom.xml
:
<dependency>
<groupId>org.springframework.social</groupId>
<artifactId>spring-social-twitter</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-security</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.security.oauth.boot</groupId>
<artifactId>spring-security-oauth2-autoconfigure</artifactId>
<version>2.3.0</version>
</dependency>
Twitter API Credentials
You’ll also need to create a Twitter Developer account to access API keys. Once created, configure your application.properties
file with your Twitter API credentials:
spring.social.twitter.appId=yourConsumerKey
spring.social.twitter.appSecret=yourConsumerSecret
spring.twitter.appToken=yourAccessToken
spring.twitter.appTokenSecret=yourAccessTokenSecret
Implementing Rate Limiting Strategy
Now that we have our development environment set up, the next step is implementing a strategy to manage rate limits. The primary focus should be on request management. Before making API calls, we need to check our remaining rate limit and act accordingly.
Code Snippet: Checking Rate Limits
Here is a code snippet showcasing how to check rate limits and handle them appropriately:
import org.springframework.social.twitter.api.Twitter;
import org.springframework.social.twitter.api.TwitterProfile;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Service;
@Service
public class TwitterService {
@Autowired
private Twitter twitter;
public void checkRateLimit() {
try {
// Request the rate limit status
ResponseEntity<Map<String, Object>> response = twitter.getRestOperations()
.getForEntity("https://api.twitter.com/1.1/application/rate_limit_status.json",
Map.class);
Map<String, Object> rateLimits = response.getBody();
int remaining = (int) ((Map) ((Map) rateLimits.get("resources"))
.get("/statuses")).get("/user_timeline").get("remaining");
if (remaining > 0) {
// Proceed with the API call
TwitterProfile profile = twitter.userOperations().getUserProfile();
System.out.println("User Profile: " + profile);
} else {
// Handle the case when the limit has been reached
System.out.println("Rate limit exceeded. Please wait before making more requests.");
}
} catch (Exception e) {
e.printStackTrace();
// Handling exceptions and logging
}
}
}
Commentary: In this snippet, we check if the remaining requests for the user timeline API are more than zero before proceeding. If the limit is reached, we inform the user, helping avoid hitting the limit.
Best Practice: Throttling Requests
If your application frequently requires data from Twitter's API, consider implementing a throttling mechanism. A simple solution is to use Thread.sleep()
based on the remaining seconds until your limit resets.
private void throttleRequests() {
try {
// Logic here to determine when to wait
Thread.sleep(60000); // Sleep for one minute
} catch (InterruptedException e) {
Thread.currentThread().interrupt(); // Restore interrupted state
}
}
Implementation of Caching
Another effective strategy for managing Twitter API limits is caching. By storing frequently requested data locally, you can significantly reduce the number of API calls. You can use libraries such as Ehcache or Spring's built-in caching mechanisms.
Code Snippet: Caching User Profile
Here’s an example of how you might implement a simple cache for user profiles:
import org.springframework.cache.annotation.Cacheable;
@Service
public class CachedTwitterService {
@Autowired
private Twitter twitter;
@Cacheable("userProfile")
public TwitterProfile getUserProfile() {
return twitter.userOperations().getUserProfile();
}
}
Commentary: The @Cacheable
annotation tells Spring to cache the result of the getUserProfile()
method, meaning that subsequent calls will return the cached value instead of making another API request.
Error Handling and Resilience
API calls will occasionally fail due to network issues or transient errors. To create a resilient application:
- Retry Logic: Implement retry mechanisms with exponential backoff strategies to manage transient failures.
- Fallback Methods: Use Circuit Breaker patterns (via libraries like Resilience4j) to provide a fallback response when the API call fails.
Example Code for Resilience4j
Here's how you can implement a fallback method using Resilience4j:
import io.github.resilience4j.retry.Retry;
import io.github.resilience4j.retry.annotation.Retry;
@Service
public class ResilientTwitterService {
@Autowired
private Twitter twitter;
@Retry(name = "twitterRetry", fallbackMethod = "fallbackRetrieveProfile")
public TwitterProfile retrieveProfile() {
return twitter.userOperations().getUserProfile();
}
public TwitterProfile fallbackRetrieveProfile(Throwable t) {
System.out.println("Fallback method called: " + t.getMessage());
// Return cached or default data
return new TwitterProfile("defaultUser", "Unknown", "unknown@example.com");
}
}
Wrapping Up
Managing Twitter API limits can pose challenges for developers, but with the right strategies — checking rate limits, implementing caching, and creating resilient systems — you can overcome these obstacles effectively. Spring Social makes it easier for Java developers to integrate Twitter's powerful functionality without getting bogged down in OAuth complexities.
As you design your application, remember that effective management not only enhances user experience but also keeps your application running smoothly, avoiding unnecessary disruptions due to API limits. For more insights on social media integration with Java, check out java.com for extensive resources.
In closing, always stay updated on Twitter's API specifications and best practices, as this will help your application remain stable and user-friendly in a rapidly evolving digital landscape. Happy coding!
By implementing these strategies and advancements in your application, you'll be well-equipped to handle Twitter's API limits and enhance the overall performance and reliability of your integration.
Checkout our other articles