Common Pitfalls in Unit Testing Spring MVC Controllers
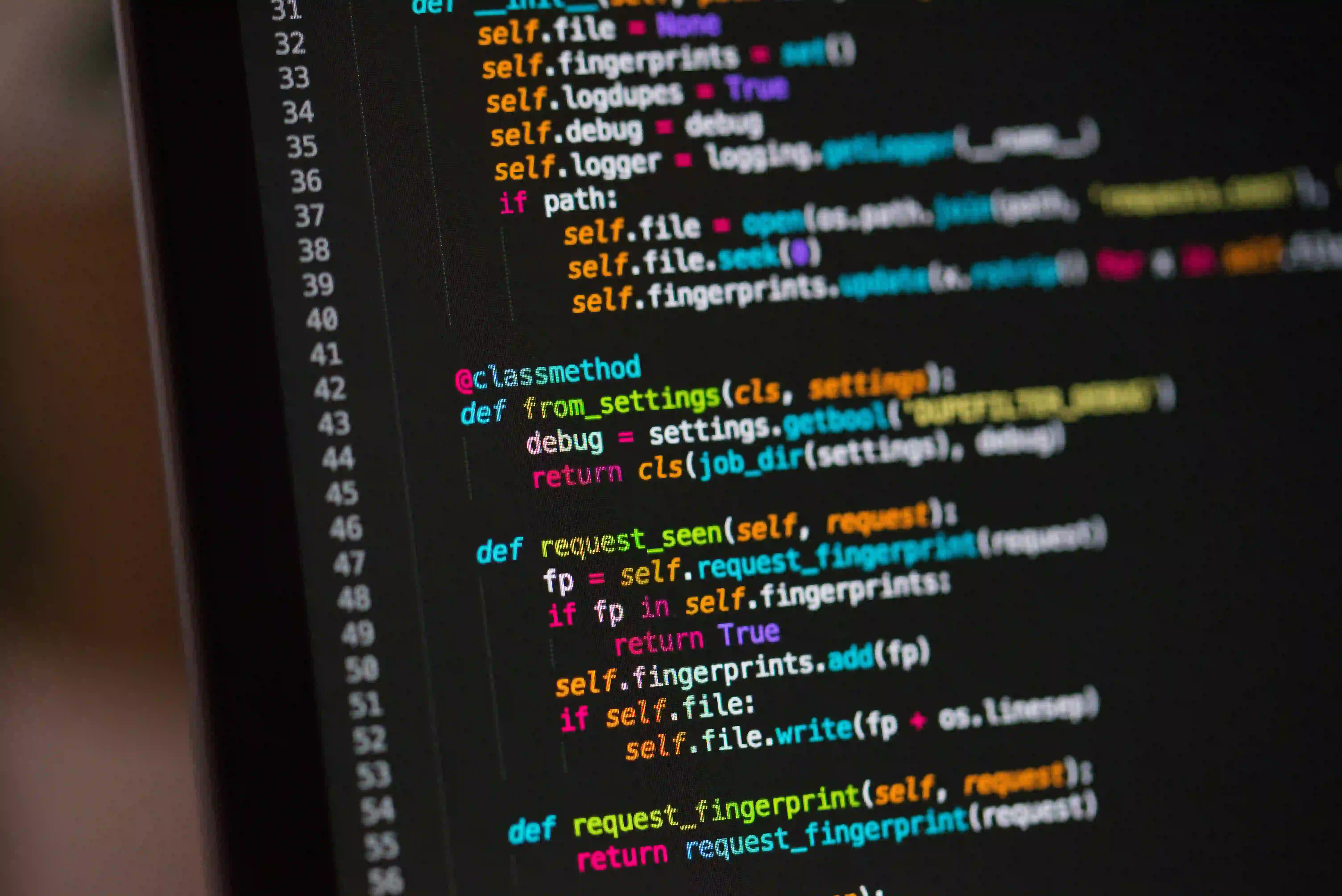
Common Pitfalls in Unit Testing Spring MVC Controllers
Unit testing is an essential practice in software development, particularly when working with Java frameworks like Spring MVC. The goal is to ensure that individual components of your application work as intended. However, pitfalls can occur, especially when testing controllers. Understanding these common issues can enhance your testing efficiency and, as a result, improve your application's overall quality.
Why Unit Testing?
Before diving into the pitfalls, let’s briefly discuss why unit testing is crucial. Unit tests help:
- Identify Bugs Early: Catching bugs during the development phase reduces costs.
- Refactor with Confidence: Well-written tests make refactoring safer.
- Documentation: Tests serve as a form of documentation for your code, helping new developers understand its intended functionality.
If you're new to Spring MVC or just looking to refresh your knowledge, you might find the official Spring MVC Documentation useful.
The Basics: Setting up Spring MVC Tests
To test a Spring MVC controller efficiently, we typically use MockMvc, which allows us to simulate HTTP requests and interact with the framework’s components.
Example of a Simple Spring MVC Controller
Consider a simple controller for managing users:
@RestController
@RequestMapping("/users")
public class UserController {
@Autowired
private UserService userService;
@PostMapping
public ResponseEntity<User> createUser(@RequestBody User user) {
User createdUser = userService.createUser(user);
return new ResponseEntity<>(createdUser, HttpStatus.CREATED);
}
}
Setting Up the Unit Test
Using JUnit, Mockito, and MockMvc, we can set up our test:
@SpringBootTest
@AutoConfigureMockMvc
public class UserControllerTest {
@Autowired
private MockMvc mockMvc;
@MockBean
private UserService userService;
@Test
public void testCreateUser() throws Exception {
User user = new User("John", "Doe");
when(userService.createUser(any(User.class))).thenReturn(user);
mockMvc.perform(post("/users")
.contentType(MediaType.APPLICATION_JSON)
.content("{\"firstName\":\"John\", \"lastName\":\"Doe\"}"))
.andExpect(status().isCreated())
.andExpect(jsonPath("$.firstName").value("John"))
.andExpect(jsonPath("$.lastName").value("Doe"));
}
}
This setup allows you to test the createUser
method without starting the entire application. But be wary! There are pitfalls in crafting these tests that could lead to false confidence.
Common Pitfalls in Unit Testing Spring MVC Controllers
1. Over-Mocking
The Problem
While mocking dependencies is essential, over-relying on mocks can lead to brittle tests. If you mock too much, your tests may pass even when the actual implementation has issues.
The Solution
Balance your use of mocks and real objects. For instance, use mocks to isolate your controller, but prefer testing a repository with an in-memory database like H2 for more complex queries.
2. Ignoring Context Configuration
The Problem
Sometimes, developers forget to configure the application context properly, leading to tests that seem valid but fail at runtime.
The Solution
Ensure your test configuration is correct, using annotations like @WebMvcTest
for controller tests or @ContextConfiguration
if necessary. Here’s how to time it right:
@WebMvcTest(UserController.class)
public class UserControllerTest {
// ...
}
This isolates your tests, making them faster and more reliable.
3. Incomplete Test Coverage
The Problem
Another common mistake is failing to test edge cases or error scenarios. A happy path test is inadequate to validate controller functionality comprehensively.
The Solution
Implement tests for various scenarios. Consider the following:
- Successful creation of a user
- Invalid user input
- Database errors
Here’s an example test for handling an invalid user:
@Test
public void testCreateUser_InvalidInput() throws Exception {
mockMvc.perform(post("/users")
.contentType(MediaType.APPLICATION_JSON)
.content("{\"firstName\":\"\", \"lastName\":\"Doe\"}")) // Invalid firstName
.andExpect(status().isBadRequest());
}
4. Using Real Services Instead of Mocks
The Problem
Developers sometimes use real services in what they believe are isolated tests. This leads to tests that are dependent on the database state and can result in flaky tests.
The Solution
Always use mocks for your service layer to ensure your controller tests remain true unit tests. This also enables you to test the controller without needing to manage the service's internal state.
5. Not Considering Exception Handling
The Problem
If a controller method throws an exception and it is not handled correctly, your tests may pass incorrectly or never reach certain assertions.
The Solution
Always include tests for exception cases. For example, if createUser
throws a UserAlreadyExistsException
:
@Test
public void testCreateUser_UserAlreadyExists() throws Exception {
User user = new User("Existing", "User");
when(userService.createUser(any(User.class))).thenThrow(new UserAlreadyExistsException());
mockMvc.perform(post("/users")
.contentType(MediaType.APPLICATION_JSON)
.content("{\"firstName\":\"Existing\", \"lastName\":\"User\"}"))
.andExpect(status().isConflict());
}
6. Not Verifying Interactions with Mocks
The Problem
After performing an action, developers often forget to verify interactions with their mocks, which can lead to untested paths.
The Solution
Use Mockito's verify()
method to ensure that the expected interactions occurred. For instance:
verify(userService).createUser(any(User.class));
This ensures that your service's createUser
method was called during the test.
7. Testing Implementation Instead of Behavior
The Problem
Some tests that check for specific implementation details (like method calls) instead of behavior can lead to issues when refactoring.
The Solution
Focus on what the controller is supposed to do rather than how it does it. Assert the end result instead of the method calls. For example, checking the response status and body is crucial, while verifying service calls should be supplementary.
To Wrap Things Up
Unit testing Spring MVC controllers might seem straightforward, but there are several common pitfalls that can undermine your testing efforts. Avoiding issues like over-mocking, ignoring context, inadequate test coverage, and others is vital for building a robust application.
By adhering to best practices and being mindful of these common pitfalls, you can create effective unit tests that improve your codebase's reliability and maintainability. For further reading on best practices in unit testing, you can check out JUnit 5 User Guide and Mockito Quick Start for more insights.
Remember: unit tests should save you time and effort in the long run, but only if crafted thoughtfully! Happy testing!