Common Pitfalls When Setting Up jOOQ with H2 and Maven
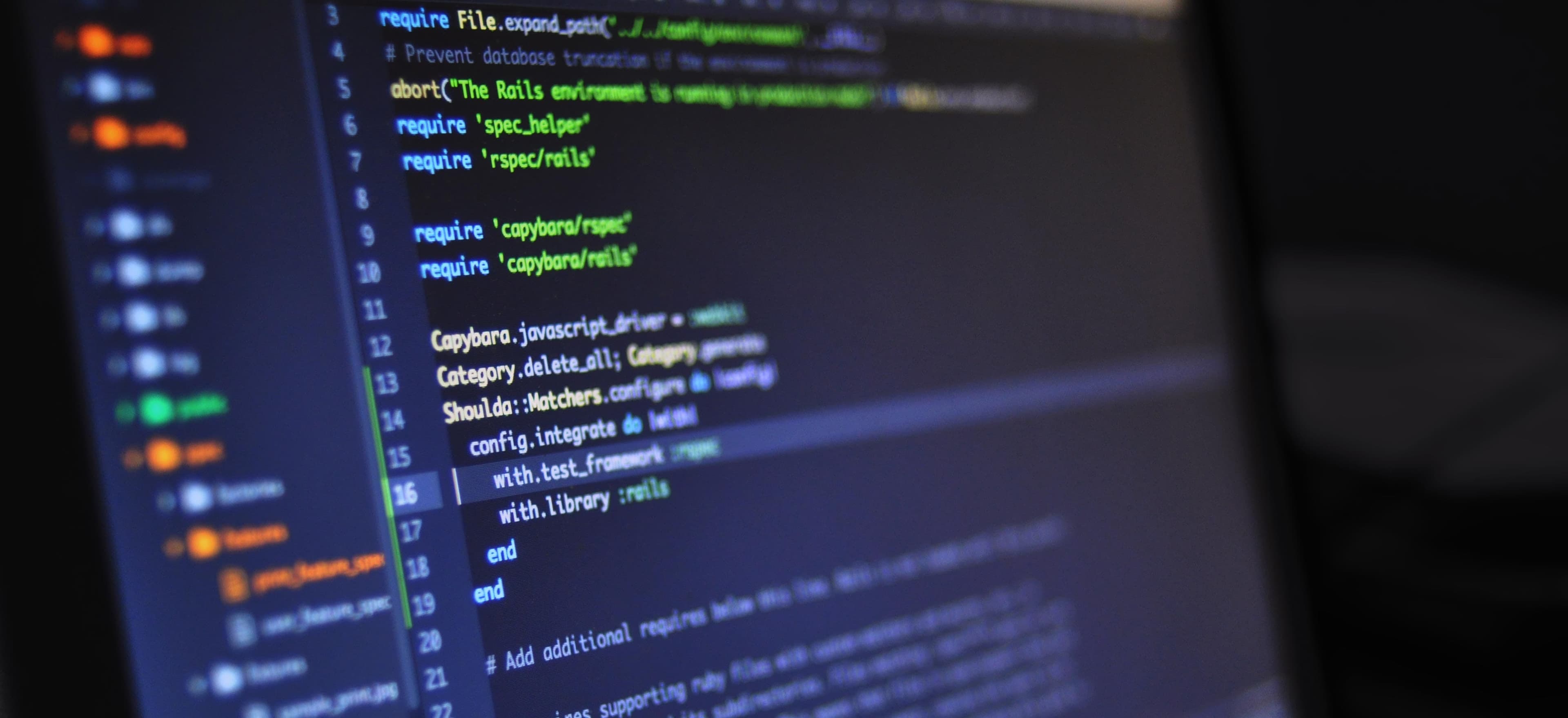
- Published on
Common Pitfalls When Setting Up jOOQ with H2 and Maven
When working with Java's data access layer, jOOQ (Java Object Oriented Querying) is a powerful tool that allows developers to build type-safe SQL queries. When combined with an in-memory database like H2 and managed with Maven, it streamlines database interactions and enhances productivity. However, setting this up can be a little tricky for newcomers or those unacquainted with the nuances of these technologies. In this post, we will explore common pitfalls when configuring jOOQ with H2 and Maven and provide solutions to ensure a seamless development experience.
Understanding jOOQ, H2, and Maven
Before diving into the pitfalls, it's important to understand the context in which these technologies excel. jOOQ provides a powerful and intuitive API for building SQL queries programmatically. H2 is an open-source database engine with a small footprint, ideal for testing and development. Maven is a build automation tool primarily for Java projects, handling dependencies, build cycles, and project management.
Combining these tools can yield a robust development environment, but it's crucial to avoid common mistakes. Let's delve into these pitfalls.
1. Incorrect Dependency Versioning
One of the most prevalent issues developers encounter involves managing Maven dependencies. Using incompatible versions can lead to runtime errors or compilation issues.
Solution
Ensure that the versions of jOOQ, H2, and any other related libraries are compatible. As of October 2023, here is an example Maven dependency configuration:
<dependencies>
<dependency>
<groupId>org.jooq</groupId>
<artifactId>jooq</artifactId>
<version>3.16.1</version>
</dependency>
<dependency>
<groupId>com.h2database</groupId>
<artifactId>h2</artifactId>
<version>1.4.200</version>
<scope>test</scope>
</dependency>
<!-- Include the jOOQ code generator if needed -->
<dependency>
<groupId>org.jooq</groupId>
<artifactId>jooq-codegen</artifactId>
<version>3.16.1</version>
</dependency>
<dependency>
<groupId>org.apache.commons</groupId>
<artifactId>commons-lang3</artifactId>
<version>3.12.0</version>
</dependency>
</dependencies>
Make sure to regularly check for the latest versions or any breaking changes in the library's release notes.
2. Misconfigured jOOQ Code Generation
jOOQ can automatically generate Java classes for your database schema, but misconfiguration in this step can lead to failure. It's essential that the configuration matches your H2 setup correctly.
Solution
In your pom.xml
, configure the jOOQ code generation like this:
<plugin>
<groupId>org.jooq</groupId>
<artifactId>jooq-codegen-maven</artifactId>
<version>3.16.1</version>
<executions>
<execution>
<id>generate-sources</id>
<goals>
<goal>generate</goal>
</goals>
<configuration>
<jdbc>
<driver>org.h2.Driver</driver>
<url>jdbc:h2:mem:test;DB_CLOSE_DELAY=-1;DB_CLOSE_ON_EXIT=FALSE</url>
<user>sa</user>
<password></password>
</jdbc>
<generator>
<database>
<name>org.jooq.meta.h2.H2Database</name>
<inputSchema>PUBLIC</inputSchema>
</database>
<target>
<packageName>com.example.jooq.generated</packageName>
<directory>target/generated-sources/jooq</directory>
</target>
</generator>
</configuration>
</execution>
</executions>
</plugin>
Ensure the url
is correct and matches your database configuration. The DB_CLOSE_DELAY=-1
parameter ensures that the database remains available while your application is running.
3. Not Setting Up Correctly for In-Memory Database Usage
H2 can operate as an in-memory database, which is excellent for testing. However, if the application is not set to connect correctly to this in-memory database, it leads to unexpected behavior.
Solution
Make sure that your database connection URL in the application configuration is properly set, as shown above. You might also find it useful to include some initial data setup in your application to allow for more meaningful testing.
Here's an example of initializing the database:
import static org.jooq.impl.DSL.*;
public void setupDatabase() {
try (Connection connection = DriverManager.getConnection("jdbc:h2:mem:test;DB_CLOSE_ON_EXIT=FALSE")) {
DSLContext create = using(connection, SQLDialect.H2);
create.execute("CREATE TABLE IF NOT EXISTS users (id INT PRIMARY KEY, name VARCHAR(255))");
create.execute("INSERT INTO users (id, name) VALUES (1, 'Alice'), (2, 'Bob')");
} catch (SQLException e) {
e.printStackTrace();
}
}
By ensuring your database is initially populated with data, you can execute queries that will meaningfully demonstrate jOOQ's capabilities.
4. Ignoring Thread Safety
JDBC connections and H2 can encounter thread safety issues if not handled correctly within a multi-threaded application. This can happen if a connection is used concurrently across different threads.
Solution
Always ensure that each thread has its own database connection. You can do this with a connection pool (using libraries such as HikariCP). Here’s an example of using HikariCP:
<dependency>
<groupId>com.zaxxer</groupId>
<artifactId>HikariCP</artifactId>
<version>5.0.1</version>
</dependency>
And a basic setup in code:
import com.zaxxer.hikari.HikariConfig;
import com.zaxxer.hikari.HikariDataSource;
public class DataSourceConfig {
private HikariDataSource dataSource;
public DataSourceConfig() {
HikariConfig config = new HikariConfig();
config.setJdbcUrl("jdbc:h2:mem:test;DB_CLOSE_ON_EXIT=FALSE");
config.setUsername("sa");
config.setPassword("");
dataSource = new HikariDataSource(config);
}
public HikariDataSource getDataSource() {
return dataSource;
}
}
This connection pooling ensures your application can handle multiple threads efficiently.
5. Not Understanding SQL Dialects
jOOQ supports various SQL dialects, and failing to specify the correct dialect can lead to incorrect SQL being generated.
Solution
Always explicitly define the dialect you're using. For H2, ensure you use SQLDialect.H2
when creating your DSL context:
DSLContext create = DSL.using(dataSource.getConnection(), SQLDialect.H2);
This ensures jOOQ generates SQL compatible with H2, reducing the risk of errors.
To Wrap Things Up
Setting up jOOQ with H2 and Maven provides a powerful framework for Java developers, but it's essential to navigate common pitfalls effectively. By managing dependencies correctly, configuring code generation accurately, setting up the database connection for in-memory functionality, ensuring thread safety, and using the appropriate SQL dialect, you can avoid most issues you might encounter.
For further reading on jOOQ, the official documentation provides extensive resources on getting started, mapping databases, and advanced querying options. Let us know your experiences and tips for using jOOQ in the comments below!