Common Pitfalls When Deploying Axon CQRS Microservices
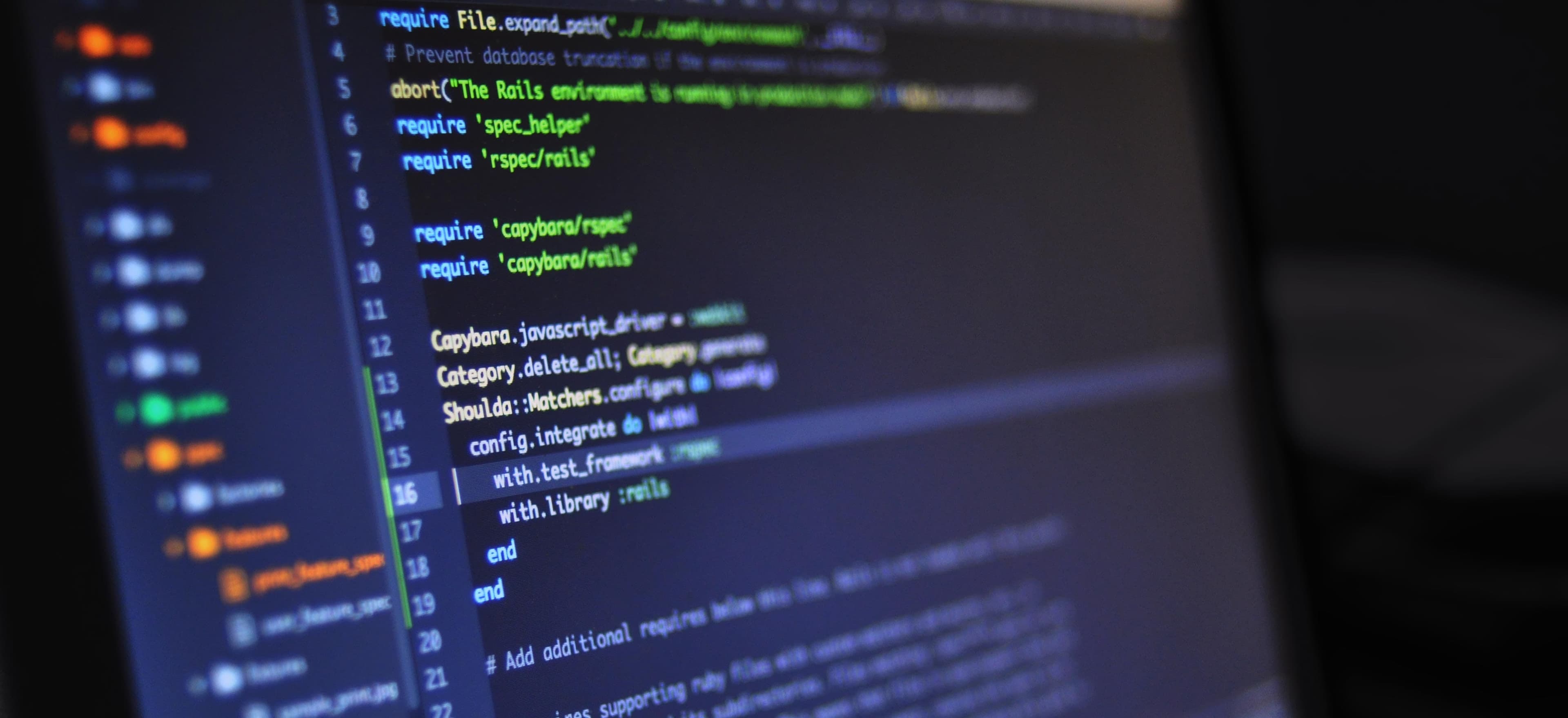
- Published on
Common Pitfalls When Deploying Axon CQRS Microservices
In today's technology-driven landscape, the quest for scalability, resilience, and efficient data management has led many organizations to embrace the Command Query Responsibility Segregation (CQRS) architectural pattern. Axon Framework, specifically designed for building CQRS and Event Sourcing applications, supports developers in creating robust microservices. However, deploying these microservices is not without its challenges. In this blog post, we will explore some common pitfalls developers face when deploying Axon CQRS microservices and provide actionable strategies to overcome them.
Understanding CQRS and Axon Framework
Before diving into the pitfalls, it is crucial to grasp the concepts of CQRS and the Axon Framework. CQRS is a pattern that separates the data modification commands from the data retrieval queries, allowing for optimized performance for each operation. This separation can lead to more scalable applications, especially in microservice environments.
The Axon Framework provides tools and infrastructure suitable for implementing CQRS and Event Sourcing. It handles the heavy lifting of managing commands, queries, events, and aggregates, enabling developers to focus on business logic rather than architectural concerns.
1. Ignoring the Eventual Consistency Model
One of the most significant pitfalls in a CQRS architecture is failing to understand and design for eventual consistency. In a microservices architecture, different services can have varying models and states, creating scenarios where data is not immediately synchronized across all services.
Solution: Embrace Eventual Consistency
It is essential to educate stakeholders about the trade-offs of eventual consistency versus strong consistency. Use asynchronous messaging systems to propagate events and update read models without blocking the operations.
Example: Event Publication and Subscription
Here is a simplified approach to how an event can be published and handled in Axon:
@Aggregate
public class Order {
@AggregateIdentifier
private String orderId;
public Order() {}
@CommandHandler
public Order(CreateOrderCommand command) {
// Perform validations and business logic
AggregateLifecycle.apply(new OrderCreatedEvent(command.getOrderId()));
}
}
Commentary
In this code snippet, when an order is created, we publish an OrderCreatedEvent
. Downstream services can subscribe to this event to update their read models or trigger other processes. This design allows decoupled services to act on events without directly depending on one another.
2. Overly Complex Event Handling
As applications scale, developers often find themselves dealing with numerous events, leading to complex handling logic. This complexity can create maintenance challenges and reduce performance.
Solution: Simplify Event Handlers
Focus on creating simple, decoupled event handlers. Avoid business logic in event handlers; instead, let them update the projections or trigger workflows.
Example: Simple Event Handler
@Component
public class OrderEventHandler {
@QueryHandler
public OrderProjection handle(OrderCreatedEvent event) {
return new OrderProjection(event.getOrderId(), "Pending");
}
}
Commentary
The OrderEventHandler
listens for OrderCreatedEvent
and creates a simple projection. By keeping the logic straightforward, you enhance readability and maintainability, which is crucial for larger codebases.
3. Not Monitoring Your Microservices
In a distributed system, monitoring becomes more complex yet essential. Lack of proper monitoring tools can result in missed alerts, delayed responses to issues, and downtime.
Solution: Implement Comprehensive Monitoring
Incorporate logging, tracing, and metrics collection into your Axon microservices. Utilize tools like Prometheus and Grafana to visualize application performance and health. Also consider utilizing Axon Server for an out-of-the-box monitoring solution for Axon applications.
4. Poorly Managing Database Transactions
CQRS typically relies on various databases for command and query sides, potentially introducing challenges in consistency. Improper management of transactions can lead to data anomalies.
Solution: Use Sagas for Orchestrating Transactions
Sagas provide a way to manage distributed transactions across microservices. Use Sagas to coordinate related transactions and ensure eventual consistency over long-running processes.
Example: Saga for Order Management
@Saga
public class OrderSaga {
@StartSaga
@CommandHandler
public void handle(CreateOrderCommand command) {
// Logic to create an order and publish events
AggregateLifecycle.apply(new OrderStartedEvent(command.getOrderId()));
}
@SagaEventHandler(associationProperty = "orderId")
public void on(OrderCreatedEvent event) {
// React to order creation
}
}
Commentary
In this example, the saga coordinates the processes involved in creating an order. By managing state transitions and handling compensating transactions, you can maintain data integrity across microservices.
5. Neglecting Security
With the rise of microservices, ensuring robust security measures becomes a pressing need. Vulnerabilities may arise if security is not embedded into the design from the beginning.
Solution: Implement Security Best Practices
Employ standard security practices like HTTPS, validating JSON Web Tokens (JWT), role-based access control, and secret management within microservices.
6. Inadequate Testing
Testing is vital for the reliability of microservices, yet it remains a common pitfall. Budgeting enough time for unit, integration, and end-to-end testing can make a significant difference in your deployment success.
Solution: Emphasize Comprehensive Testing Strategies
Develop a robust testing strategy that includes unit tests for commands and queries, integration tests for service communication, and automated end-to-end tests to cover user journeys.
@SpringBootTest
public class OrderServiceTest {
@Autowired
private CommandGateway commandGateway;
@Test
public void testCreateOrder() {
// Build command
CreateOrderCommand command = new CreateOrderCommand("order-1");
commandGateway.sendAndWait(command);
// Add assertions to validate that order created successfully
}
}
Commentary
In the testing example, CommandGateway
is used to send commands. Always validate that the expected side effects occur, ensuring that your application behaves as intended.
Closing the Chapter
Deploying Axon CQRS microservices offers immense advantages, but it is crucial to navigate the common pitfalls outlined in this post. Remember to embrace eventual consistency, simplify event handling, monitor system performance, manage transactions effectively, prioritize security, and invest time in comprehensive testing.
By applying the solutions discussed, you can streamline your deployment processes and harness the full potential of CQRS and the Axon Framework. For further reading on advanced CQRS practices, consider exploring CQRS by Example with Axon.
As we dive deeper into creating resilient and scalable systems, understanding these pitfalls and how to overcome them will be essential for any software development team looking to leverage the power of Axon in their microservices architecture. Happy coding!
Checkout our other articles