Mastering Akka Streams and RxJava Integration Challenges
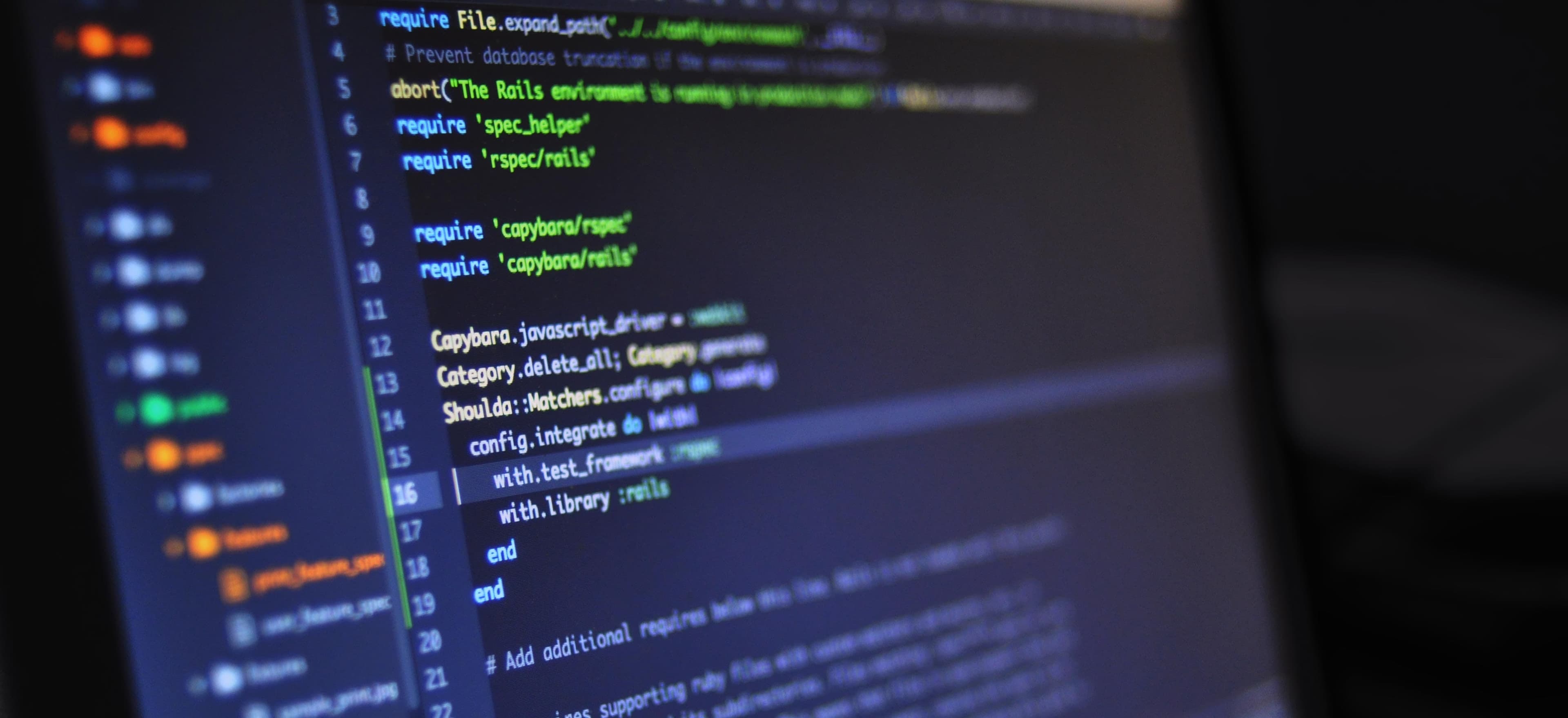
- Published on
Mastering Akka Streams and RxJava Integration Challenges
As the world of reactive programming expands, developers often find themselves navigating through a myriad of tools designed to simplify asynchronous data processing. Two prominent libraries in this space are Akka Streams and RxJava. Each has unique capabilities, but the real challenge arises when one attempts to integrate them efficiently.
In this blog post, we will delve into the intricacies of both frameworks, highlight common integration challenges, and propose effective strategies to master these powerful tools.
What are Akka Streams and RxJava?
Before we dive into integration challenges, it's crucial to understand what Akka Streams and RxJava are and what they provide.
Akka Streams
Akka is a toolkit for building highly concurrent, distributed, and fault-tolerant applications in Java and Scala. Akka Streams is a module that allows users to process streams of data in a non-blocking way. It leverages the Actor model to handle back-pressure, ensuring that systems can manage the flow of data smoothly without overwhelming resources.
Example of Akka Streams
Here’s a simple example demonstrating how to implement a straightforward Akka Streams application:
import akka.NotUsed;
import akka.actor.ActorSystem;
import akka.stream.ActorMaterializer;
import akka.stream.javadsl.Source;
import java.util.Arrays;
public class AkkaStreamsExample {
public static void main(String[] args) {
ActorSystem system = ActorSystem.create("StreamSystem");
ActorMaterializer materializer = ActorMaterializer.create(system);
// Create a Source from a list of integers
Source<Integer, NotUsed> source = Source.from(Arrays.asList(1, 2, 3, 4, 5));
// Printing out each element
source.runForeach(System.out::println, materializer);
// Shut down the actor system after use
system.terminate();
}
}
In this snippet, we create an ActorSystem
and a Source
from a list of integers. We then utilize runForeach
to print each element, demonstrating how to create a simple data stream.
RxJava
On the other hand, RxJava is a Java VM implementation of Reactive Extensions. It enables users to work with asynchronous data streams using a functional programming approach. RxJava's core components are Observables and Observers, with a rich API that allows developers to compose different data-processing operations seamlessly.
Example of RxJava
Here is a quick example showing how to create an observable stream:
import io.reactivex.Observable;
public class RxJavaExample {
public static void main(String[] args) {
// Create an Observable from a list of integers
Observable<Integer> observable = Observable.fromArray(1, 2, 3, 4, 5);
// Subscribe to the observable and print each emitted item
observable.subscribe(System.out::println);
}
}
This example illustrates how to create an observable and subscribe to it, printing each integer emitted from the stream.
Integration Challenges
Challenge 1: Data Flow Compatibility
Integrating Akka Streams with RxJava often leads to compatibility challenges in terms of data flow. When using both libraries, it’s essential to ensure that both frameworks can coexist without data loss or bottlenecking.
Solution: Use custom adapters to bridge the two libraries. For instance, you can create a source in Akka Streams that emits items to RxJava's observable, ensuring a seamless flow.
Challenge 2: Back-Pressure Management
While both Akka Streams and RxJava support back-pressure, they implement different strategies. Managing back-pressure efficiently during integration is critical to maintain system stability.
Solution: Use Akka's back-pressure strategy as the primary controller. You can integrate RxJava streams into Akka Streams by utilizing Source.fromPublisher(...)
. This way, Akka Streams handle flow control effectively, reducing burden on the entire stack.
Challenge 3: Error Handling
Error handling differs significantly in both libraries. Akka Streams operates using supervision strategies, while RxJava employs exceptions and error signals.
Solution: Adopt a consistent error-handling mechanism across both frameworks. You can utilize the onError
and onErrorResume
capabilities of RxJava to manage errors emulated by Akka Streams.
Example of Integration
To integrate Akka Streams and RxJava while addressing the challenges mentioned, consider the following code snippet:
import akka.NotUsed;
import akka.actor.ActorSystem;
import akka.stream.ActorMaterializer;
import akka.stream.javadsl.Source;
import io.reactivex.BackpressureStrategy;
import io.reactivex.Observable;
import org.reactivestreams.Publisher;
public class ReactiveIntegration {
public static void main(String[] args) {
ActorSystem system = ActorSystem.create("ReactiveIntegrationSystem");
ActorMaterializer materializer = ActorMaterializer.create(system);
// Create an Akka Stream Source
Publisher<Integer> akkaSource = Source.range(1, 5).runWith(Sink.asPublisher(AsPublisher.WITHOUT_FANOUT), materializer);
// Create an RxJava Observable from the Akka Publisher
Observable<Integer> rxObservable = Observable.create(emitter -> {
akkaSource.subscribe(emitter::onNext, emitter::onError, emitter::onComplete);
});
// Subscribe to the RxJava observable
rxObservable.subscribe(
item -> System.out.println("RxJava: " + item),
error -> System.err.println("Error: " + error),
() -> System.out.println("Completed")
);
// Shut down the actor system after use
system.terminate();
}
}
In this example, we create an Akka source that emits a range of integers. We then wrap it within an RxJava observable, allowing us to subscribe to it directly. This bridging simplifies data flow and handles both back-pressure and error management effectively.
Best Practices for Integration
- Minimize Context Switching: Limit switching between Akka and RxJava code. Each transition introduces overhead and complexity.
- Centralize the Flow Control: Let either Akka or RxJava manage the data flow. This prevents conflicting back-pressure strategies.
- Handle Errors Consistently: As mentioned earlier, ensure uniform error management across the frameworks.
Additional Resources
- For in-depth knowledge of how to build robust systems with Akka Streams
- To explore more about RxJava
The Bottom Line
Mastering the integration of Akka Streams and RxJava presents unique challenges; however, with thoughtful implementation and proper usage of bridging techniques, developers can harness the strengths of both reactive programming models.
By focusing on compatibility, managing back-pressure, and ensuring consistent error handling, you can create proficient applications that operate seamlessly in real-world environments. With these strategies in hand, you are well-prepared to tackle the intricacies of reactive programming with confidence.
Checkout our other articles