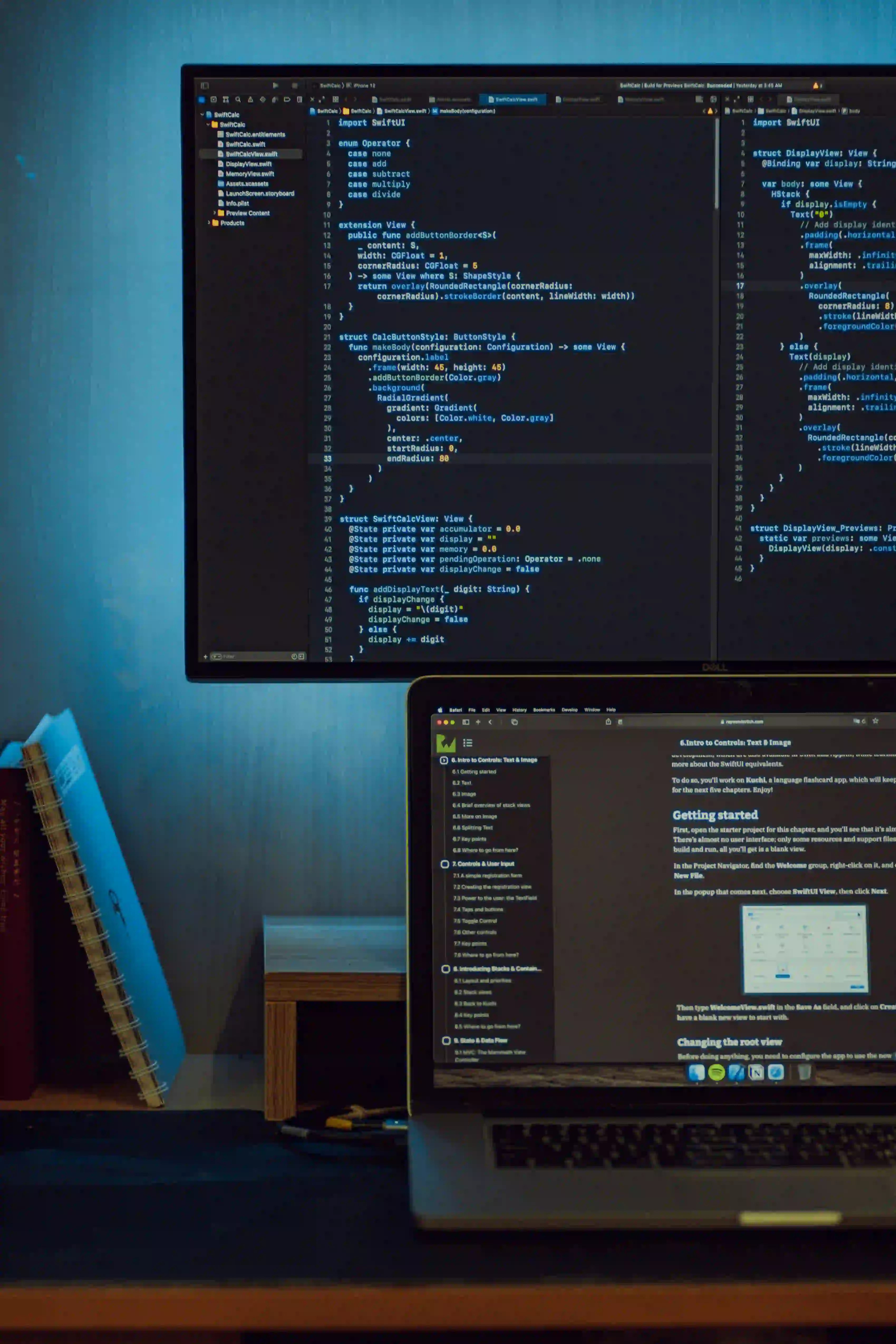
Enhancing Java Web Apps with Div Toggling Mastery
Web applications are increasingly dynamic, requiring efficient user interface (UI) elements that enhance user experience. One such element is the div toggle effect, enabling seamless interaction with content. By incorporating div toggling techniques into Java web applications, you can elevate your application to new heights.
For those seeking to dive deeper into effective div toggling, I highly recommend checking out Mastering UI: Seamless Div Toggling Techniques. This resource provides a thorough overview of div toggling methods which can be extremely beneficial in our Java applications.
Understanding Div Toggling
Div toggling is the process of showing or hiding content in a web page without requiring a full page refresh. This can be accomplished through JavaScript, but in the context of Java web applications (like those using JSP or Servlets), server-side responses may dictate the content.
The Structure of a Basic Java Web App
Before we delve deeper into toggling mechanisms, let's set the foundation of a simple Java web app. Here is a simple servlet setup:
import java.io.IOException;
import javax.servlet.ServletException;
import javax.servlet.annotation.WebServlet;
import javax.servlet.http.HttpServlet;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
@WebServlet("/toggle")
public class DivToggleServlet extends HttpServlet {
@Override
protected void doGet(HttpServletRequest request, HttpServletResponse response)
throws ServletException, IOException {
request.getRequestDispatcher("/index.jsp").forward(request, response);
}
}
In this code snippet, a servlet handles HTTP GET requests and forwards the request to an index page where our toggling logic will be implemented.
Creating the User Interface with JSP
Here’s a simple JSP file that includes a button to toggle the visibility of a div:
<%@ taglib uri="http://java.sun.com/jsp/jstl/core" prefix="c" %>
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Div Toggling Example</title>
<style>
.toggled-div {
display: none;
background-color: #f0f0f0;
padding: 20px;
border: 1px solid #ccc;
margin-top: 10px;
}
</style>
</head>
<body>
<h1>Toggle Div Example</h1>
<button id="toggleBtn">Show Content</button>
<div id="toggleDiv" class="toggled-div">
<p>This is the toggleable content!</p>
</div>
<script>
document.getElementById("toggleBtn").onclick = function() {
var div = document.getElementById("toggleDiv");
if (div.style.display === "none" || div.style.display === "") {
div.style.display = "block";
this.innerText = "Hide Content";
} else {
div.style.display = "none";
this.innerText = "Show Content";
}
};
</script>
</body>
</html>
Why This Approach?
-
Simplicity: The JavaScript code directly manipulates CSS styles based on the click event, allowing for a straightforward toggling mechanism.
-
No Reloads: Users can show or hide content seamlessly without disturbing the page state.
-
User Control: Users have explicit control over their interactions, which can lead to improved engagement.
Leveraging AJAX for More Dynamic Interactions
For more advanced applications, incorporating AJAX allows for the fetching of additional content dynamically without a full page refresh. Below is an example that loads data from the server into a div:
<%@ taglib uri="http://java.sun.com/jsp/jstl/core" prefix="c" %>
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>AJAX Example</title>
<style>
.toggled-div {
display: none;
background-color: #f0f0f0;
padding: 20px;
border: 1px solid #ccc;
margin-top: 10px;
}
</style>
</head>
<body>
<h1>AJAX Toggle Example</h1>
<button id="loadBtn">Load Content</button>
<div id="toggleDiv" class="toggled-div"></div>
<script>
document.getElementById("loadBtn").onclick = function() {
var xhr = new XMLHttpRequest();
xhr.onreadystatechange = function() {
if (xhr.readyState === 4 && xhr.status === 200) {
var div = document.getElementById("toggleDiv");
div.innerHTML = xhr.responseText;
div.style.display = div.style.display === "none" ? "block" : "none";
document.getElementById("loadBtn").innerText =
div.style.display === "block" ? "Hide Content" : "Load Content";
}
};
xhr.open("GET", "/your-server-endpoint", true);
xhr.send();
};
</script>
</body>
</html>
Explanation of AJAX Example
-
Asynchronous Requests: Using
XMLHttpRequest
, we send an asynchronous request to fetch data without reloading the page. -
Dynamic Content Update: The server returns HTML content that gets injected into the div. This enables dynamic updates based on the user’s context or actions.
-
User Experience: The toggle button changes text based on the div's state, providing clear feedback to the user.
Lessons Learned
Implementing div toggling in your Java web applications can greatly enhance user experience and application interactivity. By leveraging basic JavaScript and AJAX, you can create an engaging environment for your users, keeping them interested and coming back for more.
If you're keen on honing your UI skills further, be sure to revisit the article Mastering UI: Seamless Div Toggling Techniques.
With these techniques and insights, your Java web applications are well on their way to achieving a refined, modern look and feel, making interactions not just functional, but enjoyable. Happy coding!