Effective Java Strategies for UI Div Toggling Optimization
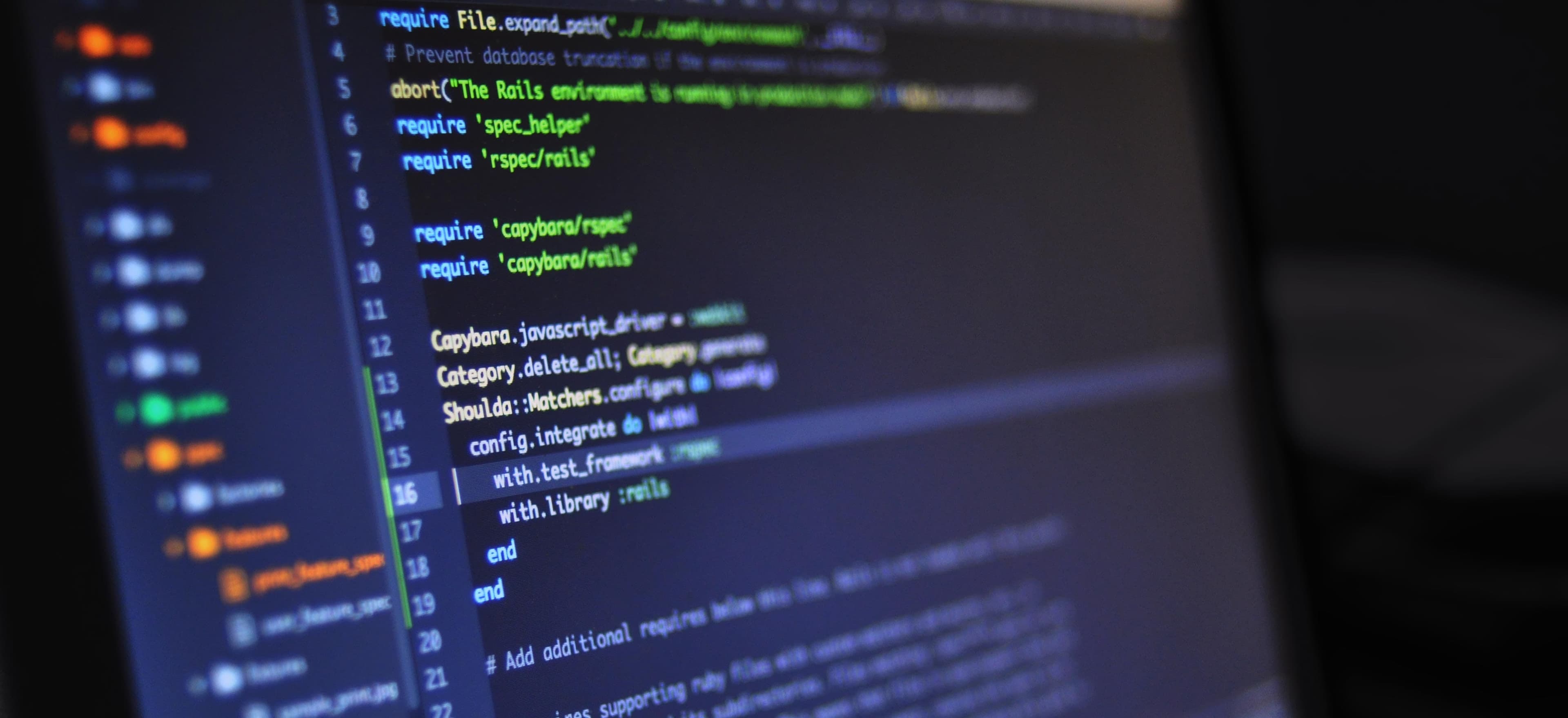
- Published on
Effective Java Strategies for UI Div Toggling Optimization
User interfaces are the first touchpoint for users interacting with applications, and optimizing these experiences is crucial. One common interaction is the toggling of div
elements on and off. This could mean showing and hiding additional information or converting a section of a web application into a more dynamic experience. In this blog post, we'll explore effective Java strategies for optimizing UI div
toggling along with relevant examples.
Understanding Div Toggling
Before we dive into the Java strategies, it's essential to understand what div
toggling entails. When toggling a div
, we want our web application to respond to user actions efficiently. This process usually involves manipulating the Document Object Model (DOM) to show or hide sections of a webpage.
Using Java, either through a server-side context with Java Servlets or using JavaScript for client-side actions, can streamline and optimize this process significantly.
Setting Up Your Environment
For this tutorial, we'll need a basic Java setup alongside a minimal HTML template. Let's ensure you have the following:
- Java Development Kit (JDK)
- An IDE (like IntelliJ IDEA or Eclipse)
- A web container (like Apache Tomcat)
- Basic knowledge of HTML and JavaScript
Simple HTML Structure
Let's begin with a simple HTML that we will manipulate using Java.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Div Toggling Example</title>
<style>
.hidden {
display: none;
}
</style>
</head>
<body>
<h1>Div Toggling with Java</h1>
<button id="toggleButton">Show More</button>
<div id="toggleDiv" class="hidden">
<p>This additional content can be toggled!</p>
</div>
<script src="toggle.js"></script>
</body>
</html>
JavaScript for Client-Side Toggling
Here’s a simple JavaScript file (toggle.js
) to manage our div toggling:
const button = document.getElementById("toggleButton");
const div = document.getElementById("toggleDiv");
button.addEventListener("click", () => {
div.classList.toggle("hidden");
button.textContent = div.classList.contains("hidden") ? "Show More" : "Show Less";
});
Why This Approach?
Using classList.toggle
is efficient because it allows us to manage visibility without manipulating style
properties directly, which is more performant in modern browsers.
Optimizing with Java
While client-side JavaScript handles real-time interactions, Java can come into play for server-side rendering or handling more complex scenarios. Below, we’ll discuss how to leverage Java for toggling requests and optimizing performance.
Java Servlet Example
You can handle requests and responses using Java Servlets. Here's an example demonstrating how a simple servlet can toggle the content dynamically:
import javax.servlet.ServletException;
import javax.servlet.annotation.WebServlet;
import javax.servlet.http.HttpServlet;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
import java.io.IOException;
@WebServlet("/toggle")
public class ToggleServlet extends HttpServlet {
protected void doGet(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
String action = request.getParameter("action");
boolean isVisible = "show".equals(action);
request.setAttribute("isVisible", isVisible);
// Forwarding to a JSP page
request.getRequestDispatcher("index.jsp").forward(request, response);
}
}
Frontend Integration
To make our Java ToggleServlet
mobile, we can modify our initial HTML to send a request to the Java backend:
<button id="toggleButton" onclick="toggleDiv()">Show More</button>
<div id="toggleDiv" class="${isVisible ? '' : 'hidden'}">
<p>This additional content can be toggled!</p>
</div>
<script>
function toggleDiv() {
const currentAction = document.getElementById("toggleDiv").classList.contains("hidden") ? "show" : "hide";
fetch(`/toggle?action=${currentAction}`)
.then(response => response.text())
.then(html => {
document.body.innerHTML = html;
});
}
</script>
Why Use Servlets for Toggling?
- Separation of Concerns: The UI is decoupled from business logic, allowing for cleaner code management.
- Scalability: You can improve performance through server-side state management and caching.
Performance Considerations
While optimizing your toggling mechanism, keep the following strategies in mind:
-
Minimize DOM Manipulations: Over-manipulating the DOM is a common bottleneck. Every operation can cause reflows and repaints, which can hinder performance.
-
Use Caching: If specific content does not change often, consider caching it. This way, you won't need to fetch it from the server again and again.
-
Debounce Input Handling: If you are toggling based on input events, use a debounce mechanism to reduce the number of function executions.
Example of Debouncing
Implementing a debounce function in JavaScript can help you optimize performance by limiting the number of calls made to the toggle function.
function debounce(func, delay) {
let timeout;
return function() {
clearTimeout(timeout);
timeout = setTimeout(() => func.apply(this, arguments), delay);
};
}
document.getElementById("toggleButton").addEventListener("click", debounce(() => {
div.classList.toggle("hidden");
button.textContent = div.classList.contains("hidden") ? "Show More" : "Show Less";
}, 300));
The Last Word
With the strategies laid out in this post, you should now have a comprehensive understanding of optimizing UI div
toggling interactions using Java and JavaScript. By employing both server-side and client-side optimizations, you can vastly improve user experience.
For additional techniques related to div
toggling, check out the article Mastering UI: Seamless Div Toggling Techniques, which provides further insights on enhancing user interfaces seamlessly.
Incorporate these strategies into your applications to ensure smooth and efficient user interactions. Always remember that the ultimate goal is to create a seamless user experience by making interfaces not only functional but also engaging. Happy coding!