Navigating Chakra Healing with Java-Based Frameworks
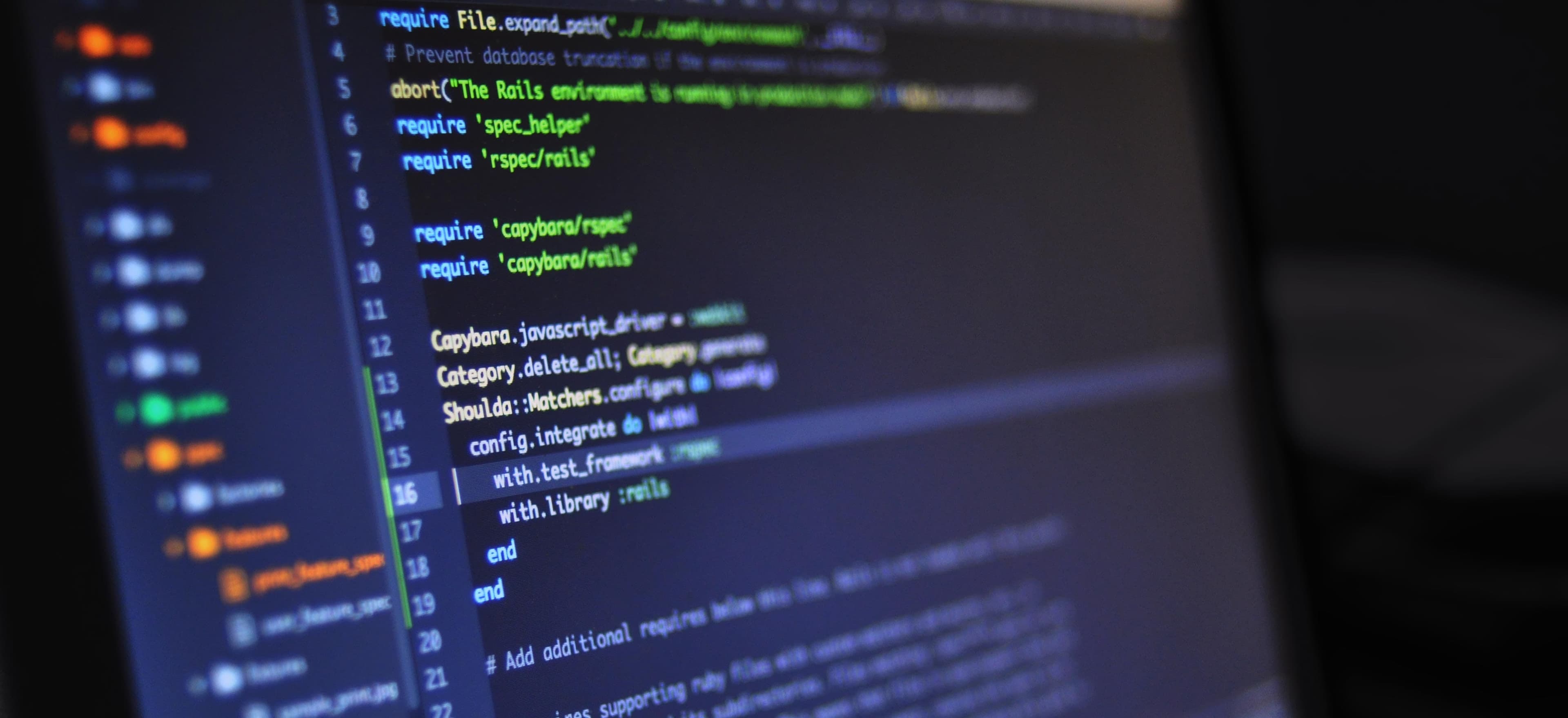
- Published on
Navigating Chakra Healing with Java-Based Frameworks
Chakra healing is gaining popularity as individuals seek holistic ways to improve their mental, emotional, and physical health. For those who are developers and interested in spiritual practices, the combination of technology and healing can be particularly compelling. In this blog post, we will explore how Java-based frameworks can be utilized to create applications centered around chakra healing. We will also discuss factors that contribute to effective application development and provide code snippets that illustrate practical implementations.
Understanding Chakras
Chakras are energy centers in the body that correspond to specific physical and emotional states. There are seven main chakras, each associated with different aspects of our life. Proper chakra alignment is believed to promote well-being and balance.
For more on the philosophical aspects of chakras, refer to the article "Chakren und Altersastrologie: Ein tiefer Einblick" here.
Java Spring Framework: An Overview
One of the best frameworks for building Java applications is Spring Framework. Spring provides a robust platform for developing versatile applications that can connect users with chakra healing practices seamlessly.
Key Features of Spring Framework:
- Dependency Injection: Promotes loose coupling, which is essential for maintainable code.
- Aspect-Oriented Programming (AOP): Suitable for separating cross-cutting concerns like logging, security, and caching.
- MVC Support: Simplifies the creation of web applications through the Model-View-Controller architecture.
Creating a Simple Chakra Healing Application
Let's break down the process of creating a simple web-based chakra healing application using Spring Boot, a popular extension of the Spring framework.
Setting Up Your Environment
Before you dive into coding, ensure you have the following installed:
- JDK 11 or higher
- Maven
- An IDE (like IntelliJ IDEA)
Project Structure
We'll follow a simple directory structure:
chakra-healing
│
├── src
│ ├── main
│ │ ├── java
│ │ │ └── com
│ │ │ └── chakra
│ │ │ ├── ChakraController.java
│ │ │ ├── ChakraService.java
│ │ │ └── Chakra.java
│ │ └── resources
│ │ └── application.properties
└── pom.xml
Step 1: Setting up Maven
Your pom.xml
file is essential for managing dependencies. Here's a simplified example:
<project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd">
<modelVersion>4.0.0</modelVersion>
<groupId>com.chakra</groupId>
<artifactId>chakra-healing</artifactId>
<version>1.0-SNAPSHOT</version>
<dependencies>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter</artifactId>
</dependency>
</dependencies>
</project>
The spring-boot-starter-web
dependency allows you to build web applications quickly without having to configure many components manually.
Step 2: Creating the Model
Next, create a Chakra.java
class to represent the chakras:
package com.chakra;
public class Chakra {
private String name;
private String description;
private String color;
// Constructor
public Chakra(String name, String description, String color) {
this.name = name;
this.description = description;
this.color = color;
}
// Getters and Setters
public String getName() { return name; }
public void setName(String name) { this.name = name; }
public String getDescription() { return description; }
public void setDescription(String description) { this.description = description; }
public String getColor() { return color; }
public void setColor(String color) { this.color = color; }
}
In this model, we encapsulate each chakra's name, description, and color, allowing for easy management and retrieval throughout the application.
Step 3: Creating the Service Layer
The ChakraService.java
class provides business logic. It will contain methods to return chakra information:
package com.chakra;
import org.springframework.stereotype.Service;
import java.util.ArrayList;
import java.util.List;
@Service
public class ChakraService {
private List<Chakra> chakras = new ArrayList<>();
public ChakraService() {
chakras.add(new Chakra("Root Chakra", "Grounding and stability", "Red"));
chakras.add(new Chakra("Sacral Chakra", "Creativity and pleasure", "Orange"));
chakras.add(new Chakra("Solar Plexus Chakra", "Confidence and self-esteem", "Yellow"));
chakras.add(new Chakra("Heart Chakra", "Love and compassion", "Green"));
chakras.add(new Chakra("Throat Chakra", "Communication", "Blue"));
chakras.add(new Chakra("Third Eye Chakra", "Intuition", "Indigo"));
chakras.add(new Chakra("Crown Chakra", "Spiritual connection", "Violet"));
}
public List<Chakra> getChakras() {
return chakras;
}
}
By using a service layer, we isolate our business logic, making it easier to manage and test. This class initializes with a few examples of chakras and could easily be extended to connect to a database.
Step 4: Creating the Controller
The controller will handle incoming HTTP requests and route them accordingly. Here's an example of ChakraController.java
:
package com.chakra;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.RestController;
import java.util.List;
@RestController
public class ChakraController {
@Autowired
private ChakraService chakraService;
@GetMapping("/chakras")
public List<Chakra> getChakras() {
return chakraService.getChakras();
}
}
The ChakraController
uses an HTTP GET request to provide users with a list of available chakras. The simple integration of Spring Dependency Injection (@Autowired
) simplifies the instantiation of service layers, ensuring you retain clean and readable code.
Step 5: Configuration
Last but not least, we will have a simple application.properties
file in your resources
folder:
server.port=8080
This line sets the port for the Spring Boot application to run on, which can be adjusted as needed.
Running the Application
To run your application, navigate to your project directory and execute:
mvn spring-boot:run
Now you can access your chakra information via http://localhost:8080/chakras
.
Bringing It All Together
By leveraging the powerful Spring Framework, you can create engaging applications that guide users in their chakra healing journeys. This blend of technology and spirituality not only enriches the user experience but also creates unique avenues for personal growth.
Using Java for chakra healing applications not only opens up new ideas but encourages developers to think more holistically about the impact of their work. Whether you're helping guide meditation, visualization, or understanding energy flow, Java frameworks serve as an excellent backbone for such innovative applications.
For inspiration and to dive deeper into spiritual concepts, check out "Chakren und Altersastrologie: Ein tiefer Einblick" on Aura Glyphs.
This post serves as a foundation for building chakra healing applications using Java-based frameworks. As technology continues to evolve, the potential to create enriching experiences in spiritual and holistic living becomes ever more exciting. Happy coding!
Checkout our other articles