Boosting Java Apps with Holistic Architecture Insights
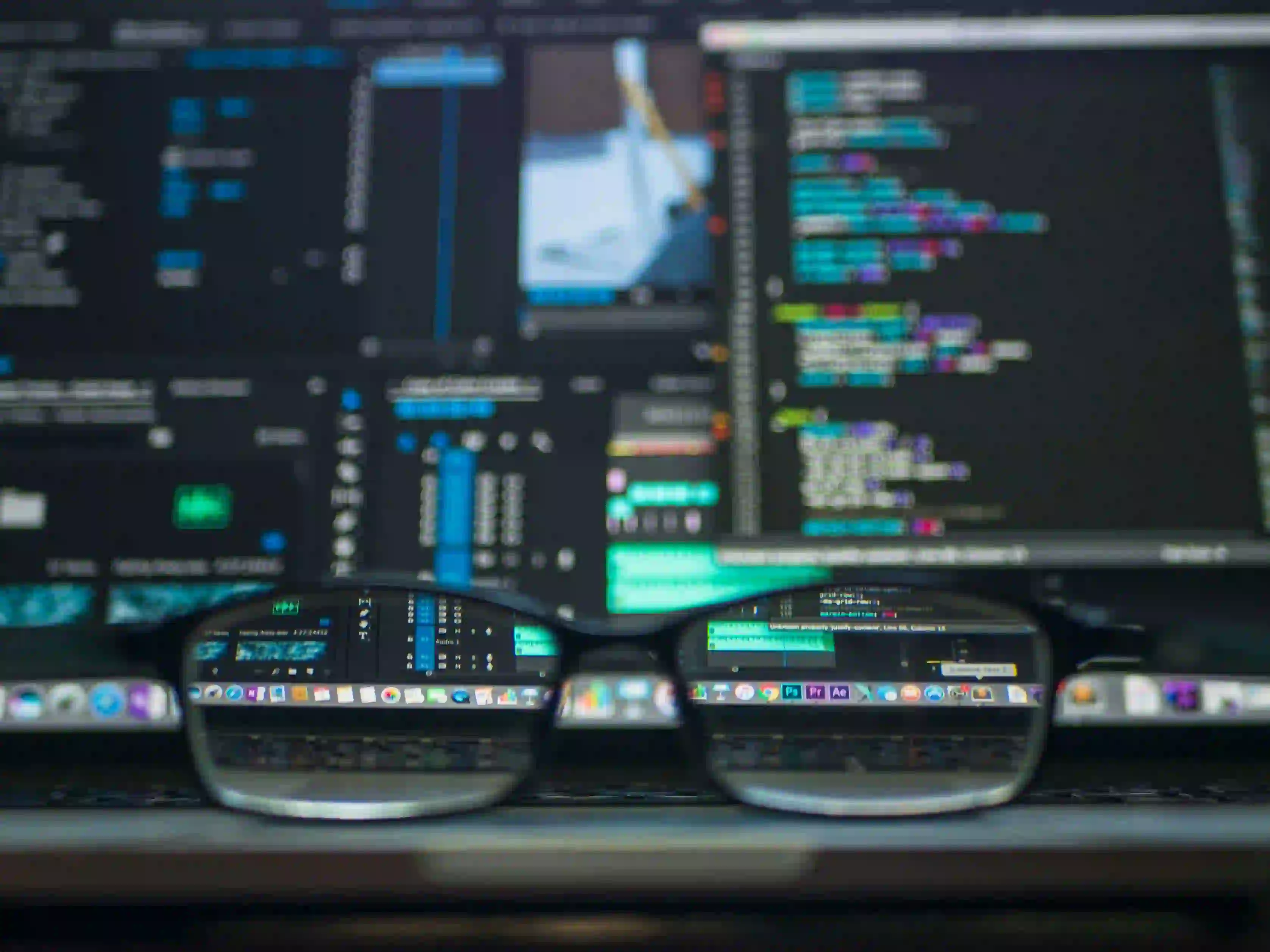
Boosting Java Apps with Holistic Architecture Insights
In the modern software landscape, creating a robust Java application necessitates a keen understanding of holistic architecture. Integrating best practices not only enhances the performance of Java applications but also improves maintainability, scalability, and user experience. In this blog post, we will explore architectural principles, design patterns, and practical coding techniques that can elevate your Java applications.
Understanding Holistic Architecture
Holistic architecture refers to an integrated approach to software design that emphasizes the relationships within component systems. It transcends the boundaries of traditional architecture and incorporates elements from various domains to improve overall software quality. This architectural style encourages a focus on building systems that are not only functionally sound but also align with business goals, user needs, and evolving technologies.
To understand the significance of a holistic approach, consider the article "Chakren und Altersastrologie: Ein tiefer Einblick", which discusses the impacts of various interrelated systems on physical well-being. The essence of holistic thinking applies similarly to software architecture. Just as every chakra influences one's overall health, every module and service in your application affects its overall performance.
You can explore this concept further in the article Chakren und Altersastrologie: Ein tiefer Einblick.
Principles of Holistic Java Architecture
Here are some key principles that should influence your architecture design:
1. Separation of Concerns
Separation of concerns is foundational to building maintainable software. It entails breaking down your application into discrete sections, each responsible for a specific functionality. This modular approach improves readability and makes testing easier.
For instance, consider a simple banking application where we might separate concerns into controllers, services, and repositories. Here’s a Java snippet demonstrating this principle:
// Controller class for handling HTTP requests
@RestController
@RequestMapping("/api/bank")
public class BankController {
private final BankService bankService;
public BankController(BankService bankService) {
this.bankService = bankService;
}
@GetMapping("/{id}")
public ResponseEntity<BankAccount> getAccount(@PathVariable Long id) {
BankAccount account = bankService.findAccountById(id);
return ResponseEntity.ok(account);
}
}
// Service class for business logic
@Service
public class BankService {
private final AccountRepository accountRepository;
public BankService(AccountRepository accountRepository) {
this.accountRepository = accountRepository;
}
public BankAccount findAccountById(Long id) {
return accountRepository.findById(id)
.orElseThrow(() -> new AccountNotFoundException("Account not found"));
}
}
// Repository interface for data access
public interface AccountRepository extends JpaRepository<BankAccount, Long> {}
In the above example, we separated HTTP handling (Controller), business logic (Service), and data access (Repository). This separation not only promotes clarity but also allows developers to work on individual components independently.
2. Use of Design Patterns
Design patterns provide reusable solutions to common problems in software design. Incorporating these patterns enhances flexibility and reduces code repetition.
For instance, the Singleton pattern is commonly used in Java to ensure that a class has only one instance and provides a global point of access to it:
public class DatabaseConnection {
private static DatabaseConnection instance;
private DatabaseConnection() {
// private constructor to prevent instantiation
}
public static synchronized DatabaseConnection getInstance() {
if (instance == null) {
instance = new DatabaseConnection();
}
return instance;
}
public void connect() {
// connect to the database
}
}
In this code, the Singleton pattern ensures a single instance of DatabaseConnection
, thus managing resources efficiently. Utilizing design patterns not only streamlines development but also enhances maintainability across the codebase.
3. Microservices Architecture
Microservices architecture advocates for constructing applications as a suite of small, independent services that can be developed, deployed, and scaled independently. This approach is particularly beneficial for large applications as it allows teams to work concurrently while minimizing dependencies.
When building microservices in Java, frameworks like Spring Boot facilitate the rapid development of standalone services. Below is a simple implementation of a microservice that manages users:
@SpringBootApplication
public class UserServiceApplication {
public static void main(String[] args) {
SpringApplication.run(UserServiceApplication.class, args);
}
}
@RestController
@RequestMapping("/api/users")
public class UserController {
private final UserService userService;
public UserController(UserService userService) {
this.userService = userService;
}
@PostMapping
public ResponseEntity<User> createUser(@RequestBody User user) {
User createdUser = userService.createUser(user);
return ResponseEntity.status(HttpStatus.CREATED).body(createdUser);
}
}
In this microservice, the UserController
handles user-related operations, encapsulating functionality just as services do in holistic architecture.
4. Event-Driven Architecture
Event-driven architecture (EDA) promotes a loosely coupled system where services communicate through events. This approach increases scalability and resilience, as services can operate independently.
Using libraries like Apache Kafka for event handling, an event-driven design can be easily implemented. Below is a simple implementation of producing events using Kafka in a Java application:
@EnableKafka
@Configuration
public class KafkaConfig {
@Bean
public ProducerFactory<String, String> producerFactory() {
Map<String, Object> configProps = new HashMap<>();
configProps.put(ProducerConfig.BOOTSTRAP_SERVERS_CONFIG, "localhost:9092");
configProps.put(ProducerConfig.KEY_SERIALIZER_CLASS_CONFIG, StringSerializer.class);
configProps.put(ProducerConfig.VALUE_SERIALIZER_CLASS_CONFIG, StringSerializer.class);
return new DefaultKafkaProducerFactory<>(configProps);
}
@Bean
public KafkaTemplate<String, String> kafkaTemplate() {
return new KafkaTemplate<>(producerFactory());
}
}
@Service
public class EventProducerService {
private final KafkaTemplate<String, String> kafkaTemplate;
public EventProducerService(KafkaTemplate<String, String> kafkaTemplate) {
this.kafkaTemplate = kafkaTemplate;
}
public void sendEvent(String message) {
kafkaTemplate.send("my-topic", message);
}
}
In this code, the EventProducerService
sends messages to a Kafka topic, allowing other services to react to these events. This decoupling fosters a dynamic environment where components work more efficiently together.
5. Health Monitoring and Metrics Collection
To ensure the longevity of your application, implementing health checks and metrics collection is vital. Tools like Spring Boot Actuator provide built-in endpoints for monitoring application health, which helps detect issues before they escalate into critical problems.
By integrating such monitoring elements, developers can enhance the application’s robustness. Here’s a simple example of how to use Spring Boot Actuator:
@SpringBootApplication
public class ActuatorDemoApplication {}
@EnableActuator
public class ActuatorConfig {
// Additional configuration can be added here
}
With this setup, health and metrics endpoints will automatically be available, giving developers insights into application performance.
Final Thoughts
Adopting a holistic architectural approach can significantly enhance your Java applications by improving their performance, maintainability, and scalability. The principles discussed, from separation of concerns to adopting microservices and event-driven architecture, combine to create a powerful framework for development.
Just as the interconnectedness of chakras influences overall physical health, the various components of your software will directly impact the efficiency of your applications. Implementing these architectural insights will help you build resilient and high-performing Java applications.
For more insights into holistic development, check out the article titled Chakren und Altersastrologie: Ein tiefer Einblick.
By applying these principles, you can ensure your Java applications are not just functional but truly exceptional.