Java Solutions for Testing Async Code: A Deep Dive
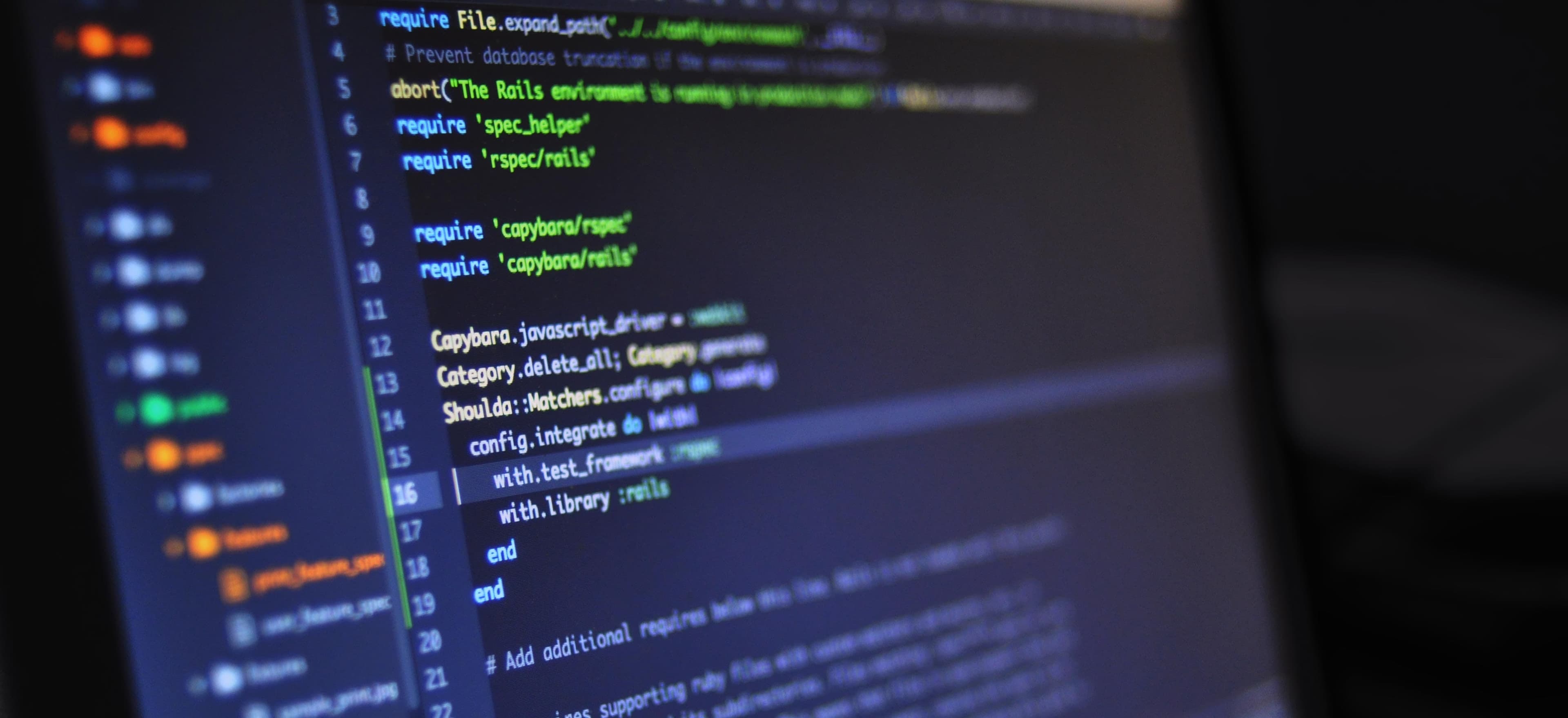
- Published on
Java Solutions for Testing Async Code: A Deep Dive
As software development evolves, writing efficient and reliable asynchronous code has become increasingly crucial. Java, one of the most prevalent programming languages, offers several tools and methodologies for testing asynchronous code. In this post, we delve into various strategies for testing asynchronous code in Java, ensuring your applications remain robust and maintainable.
Understanding Asynchronous Code in Java
Asynchronous programming allows code to perform tasks concurrently. It is an effective approach to improving application performance, especially for I/O-bound operations. In Java, several frameworks such as CompletableFuture, ExecutorService, and libraries like RxJava facilitate asynchronous programming. However, testing asynchronous code introduces unique challenges.
Challenges of Testing Asynchronous Code
When testing async code, developers often face issues such as race conditions, timing issues, and the difficulty of verifying the state after async operations. Without proper testing strategies, bugs may remain hidden until they manifest in production.
Testing Strategies for Asynchronous Code in Java
Below, we outline effective strategies for testing async code in Java, providing code samples to illustrate each method.
1. Using CompletableFuture
Java's CompletableFuture
is a powerful tool for writing asynchronous code. It allows you to build a pipeline of operations that can be run asynchronously. When testing with CompletableFuture
, you can make use of its methods to verify results.
Example: Basic CompletableFuture Testing
import org.junit.jupiter.api.Test;
import java.util.concurrent.CompletableFuture;
import static org.junit.jupiter.api.Assertions.assertEquals;
public class CompletableFutureTest {
@Test
public void testCompletableFuture() {
CompletableFuture<Integer> future = CompletableFuture.supplyAsync(() -> 2)
.thenApplyAsync(result -> result * 2);
Integer result = future.join(); // Wait for the computation to complete
assertEquals(4, result, "The computed value should be 4");
}
}
Commentary
In this example, supplyAsync
runs in a separate thread, while thenApplyAsync
transforms the result. By calling join()
, we wait for the completion of the async computation. This pattern effectively waits for the async task and retrieves its result, allowing for straightforward assertions.
2. Using CountDownLatch
For testing scenarios that involve multiple async tasks, Java provides CountDownLatch
. This is a synchronization aid that allows one or more threads to wait until a set of operations in other threads completes.
Example: CountDownLatch Testing
import org.junit.jupiter.api.Test;
import java.util.concurrent.CountDownLatch;
import static org.junit.jupiter.api.Assertions.assertTrue;
public class CountDownLatchTest {
@Test
public void testCountDownLatch() throws InterruptedException {
CountDownLatch latch = new CountDownLatch(1);
final boolean[] isCompleted = {false};
new Thread(() -> {
// Simulate async work
try {
Thread.sleep(100); // Simulating a delay
} catch (InterruptedException e) {
Thread.currentThread().interrupt();
}
isCompleted[0] = true;
latch.countDown(); // Indicate completion
}).start();
latch.await(); // Wait for the async operation to finish
assertTrue(isCompleted[0], "Async operation should have completed");
}
}
Commentary
In this code, we initialize a CountDownLatch
with a count of 1. When the async operation completes, it calls countDown()
, allowing the main thread to proceed after waiting with await()
. This structure ensures that the test only continues once the async operation is finished.
3. Using Awaitility
While the CountDownLatch
approach is effective, it can lead to cumbersome code if you have multiple async services to wait for. To make your tests cleaner and more expressive, consider using the Awaitility library.
Example: Awaitility Testing
import org.junit.jupiter.api.Test;
import static org.awaitility.Awaitility.*;
import static java.util.concurrent.TimeUnit.SECONDS;
public class AwaitilityTest {
private volatile boolean flag = false; // A flag to simulate async completion
@Test
public void testAwaitility() {
new Thread(() -> {
try {
Thread.sleep(100); // Simulating a delay
} catch (InterruptedException e) {
Thread.currentThread().interrupt();
}
flag = true; // Async completion
}).start();
await().atMost(5, SECONDS).until(() -> flag);
assertTrue(flag, "The async operation should have completed");
}
}
Commentary
Here, we employ Awaitility to succinctly handle the waiting mechanism. It allows us to specify a condition (flag
should be true) and a timeout. The test will keep checking the condition until it is fulfilled or the timeout elapses. This makes our test simpler and more readable.
4. Integration Testing with Async
Often, async code is involved in a larger system, requiring integration tests. A common tool for these tests is TestNG or JUnit 5 combined with Mock libraries like Mockito.
Mocking Async Methods Example
import org.junit.jupiter.api.Test;
import org.mockito.Mockito;
import java.util.concurrent.CompletableFuture;
import static org.junit.jupiter.api.Assertions.assertEquals;
import static org.mockito.Mockito.when;
public class AsyncServiceTest {
public interface AsyncService {
CompletableFuture<String> asyncOperation();
}
@Test
public void testAsyncService() {
AsyncService asyncService = Mockito.mock(AsyncService.class);
when(asyncService.asyncOperation()).thenReturn(CompletableFuture.completedFuture("Mock Result"));
String result = asyncService.asyncOperation().join(); // Wait for the result
assertEquals("Mock Result", result, "The result should be 'Mock Result'");
}
}
Commentary
In this integration test, we mock an asynchronous service using Mockito. By predefining what the async operation returns, we can test the handling of the async method without needing the actual implementation. This not only speeds up tests but also isolates them.
The Closing Argument
Testing asynchronous code in Java does not need to be daunting. Utilizing tools like CompletableFuture, CountDownLatch, Awaitility, and Mockito can significantly enhance your testing strategy.
If you’re also working with frameworks like Svelte, you might find valuable insights in the article Struggling with Testing Async State Changes in Svelte?. Adopting robust techniques to handle async programming will heighten the quality of your code and ensure your applications run smoothly.
Adapting your unit tests to accommodate async operations ensures that you catch potential issues early in the development cycle. Integrating these strategies into your testing suite will improve your application's reliability and maintainability as it scales. Happy coding and testing!