Harmonizing Chakra Healing in Java-based Wellness Apps
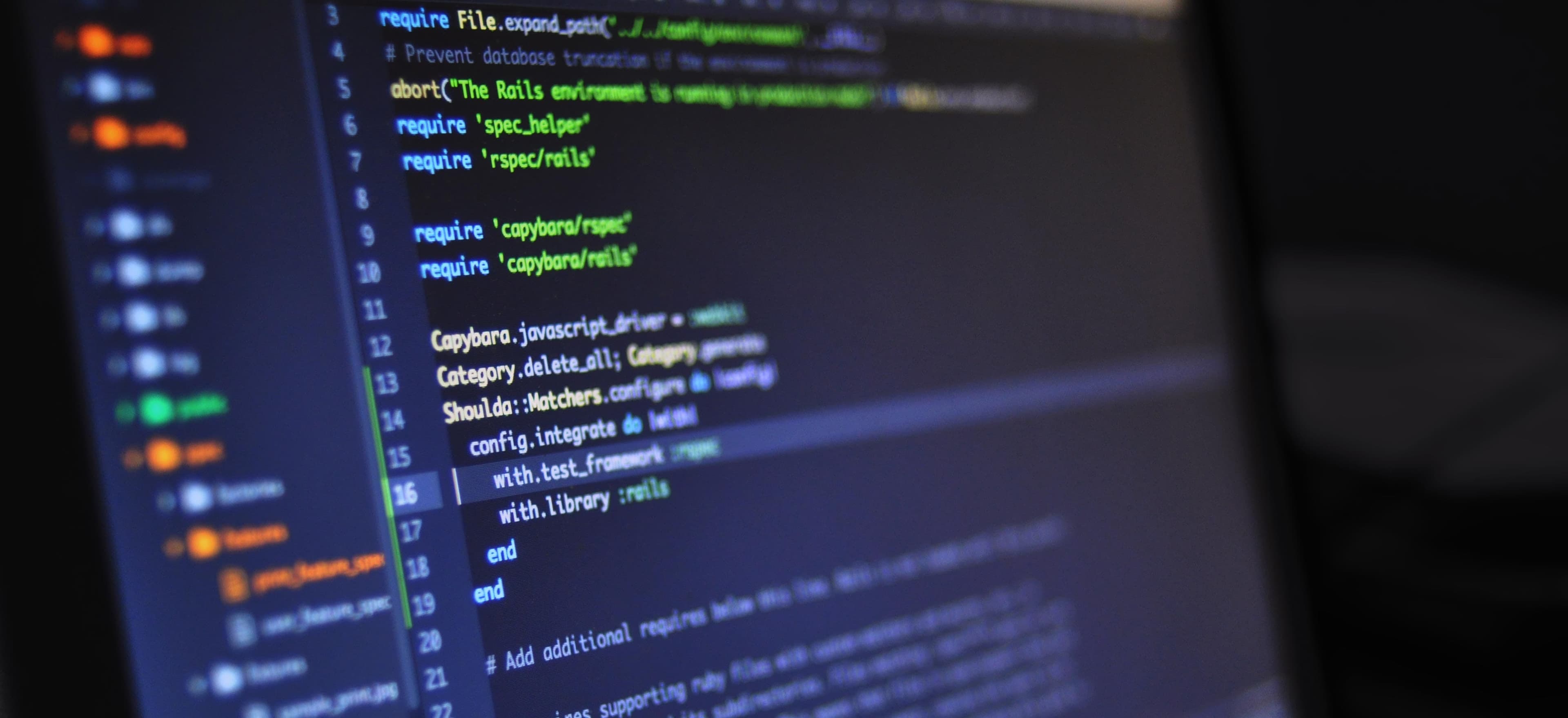
- Published on
Harmonizing Chakra Healing in Java-based Wellness Apps
In an era where wellness and technology intersect, the demand for apps that promote holistic health and spiritual well-being has surged. A popular concept within the wellness community is the understanding of chakras—centers of energy in the body that influence our physical, mental, and emotional health. This blog post will explore how to implement chakra healing concepts in Java-based wellness applications, providing developers with practical insights and code snippets to bring this great idea to life.
Understanding Chakras
Before diving into the coding aspect, let's look at what chakras represent. There are seven main chakras, each associated with specific physical, emotional, and spiritual functions:
- Root Chakra (Muladhara) - Stability and security.
- Sacral Chakra (Svadhisthana) - Creativity and emotional connection.
- Solar Plexus Chakra (Manipura) - Confidence and personal power.
- Heart Chakra (Anahata) - Love and compassion.
- Throat Chakra (Vishuddha) - Communication and truth.
- Third Eye Chakra (Ajna) - Intuition and insight.
- Crown Chakra (Sahasrara) - Spiritual connection.
An excellent resource for further reading on this subject is the article titled Chakren und Altersastrologie: Ein tiefer Einblick, which provides deeper insights into the significance of chakras in astrology and personal growth.
Why Use Java for Wellness Apps?
Java is an excellent choice for developing wellness applications due to its platform independence, extensive libraries, and strong community support. Furthermore, Java's robustness and scalability are ideal for applications that may evolve as user needs grow.
Key Features to Implement
In a wellness app, particularly one focused on chakra healing, you might want to consider the following features:
- Chakra Assessment Quiz - Help users evaluate their chakra status.
- Guided Meditations - Offer audio or video guidance for chakra healing.
- Personalized Recommendations - Suggest activities or affirmations to balance chakras.
- Progress Tracking - Allow users to track improvements or changes over time.
Developing a Chakra Assessment Quiz
To begin, let's develop a simple console application to assess the user's chakra status using a quiz format.
Step 1: Setting Up Your Project
Create a new Java project in your preferred IDE. Ensure you have a basic understanding of Java and its syntax.
Step 2: The Code Implementation
Below is a simple implementation of a chakra assessment quiz:
import java.util.HashMap;
import java.util.Map;
import java.util.Scanner;
public class ChakraQuiz {
private final Map<String, Integer> chakraScores;
public ChakraQuiz() {
chakraScores = new HashMap<>();
for (String chakra : getChakras()) {
chakraScores.put(chakra, 0);
}
}
private String[] getChakras() {
return new String[]{"Root", "Sacral", "Solar Plexus", "Heart", "Throat", "Third Eye", "Crown"};
}
public void conductQuiz() {
Scanner scanner = new Scanner(System.in);
for (String chakra : getChakras()) {
System.out.println("On a scale from 1 to 10, how would you rate your " + chakra + " Chakra?");
int score = Integer.parseInt(scanner.nextLine());
chakraScores.put(chakra, score);
}
displayResults();
scanner.close();
}
private void displayResults() {
System.out.println("Your Chakra Scores:");
chakraScores.forEach((chakra, score) -> System.out.println(chakra + " Chakra: " + score));
}
public static void main(String[] args) {
ChakraQuiz quiz = new ChakraQuiz();
quiz.conductQuiz();
}
}
Commentary on the Code
Why Use a HashMap?
The choice of HashMap
for storing chakra scores allows for efficient storage and retrieval of data. Each chakra's name is unique, making it a perfect candidate for HashMap
keys.
Why Use a Scanner?
Using Scanner
for user input is intuitive and straightforward, enabling user interaction via the console. This simple interface encourages users to engage with the app without unnecessary distractions.
Step 3: Improving User Experience
While the console application provides basic functionality, the next step is to enhance user experience—transitioning from a console application to a GUI-based application using JavaFX or Swing. For now, let’s focus on the scoring logic.
Scoring Logic Enhancement
We could enhance the scoring logic to evaluate which chakras are out of balance. Here's an example modification to interpret the scores more meaningfully:
private void displayResults() {
System.out.println("Your Chakra Scores:");
int totalScore = 0;
for (Map.Entry<String, Integer> entry : chakraScores.entrySet()) {
String chakra = entry.getKey();
int score = entry.getValue();
totalScore += score;
System.out.println(chakra + " Chakra: " + score + (score < 5 ? " (Needs Attention)" : ""));
}
System.out.println("Total Chakra Balance Score: " + totalScore);
}
Commentary
This modification adds more context by indicating which chakras are potentially out of balance (scoring below 5). It's essential for users to know not just their scores but also what actions they may need to take.
Integrating Guided Meditations
After assessing chakra health, provide users with guided meditations. This feature could leverage Java's multimedia capabilities. Below is a conceptual outline for integrating audio files for meditation:
Step 1: Java Sound API
Using the Java Sound API, you can easily play audio files within your application. Here’s a snippet demonstrating how to play an audio file:
import javax.sound.sampled.*;
import java.io.File;
import java.io.IOException;
public class MeditationAudio {
public void playMeditation(String filepath) {
try {
AudioInputStream audioInputStream = AudioSystem.getAudioInputStream(new File(filepath));
Clip clip = AudioSystem.getClip();
clip.open(audioInputStream);
clip.start();
System.out.println("Playing meditation audio...");
// Keep the program running until the audio finishes playing
while (clip.isRunning()) {
Thread.sleep(1000);
}
} catch (UnsupportedAudioFileException | IOException | LineUnavailableException | InterruptedException e) {
e.printStackTrace();
}
}
public static void main(String[] args) {
MeditationAudio meditationAudio = new MeditationAudio();
meditationAudio.playMeditation("path/to/your/meditation/file.wav");
}
}
Commentary on Audio Integration
Why Use a Clip?
The Clip
class allows you to efficiently play sound, offering functionalities like looping, pausing, and even stopping audio files. This is crucial for enhancing user experience in a wellness app.
In Conclusion, Here is What Matters
Incorporating chakra healing principles in a Java-based wellness app can create a transformative user experience. By implementing features like chakra assessments and guided meditations, developers not only provide tools for personal growth but also engage users in a meaningful way.
This blog has merely scratched the surface, but the potential for expansion is vast. You could integrate other wellness concepts, such as yoga exercises, nutrition advice, or mindfulness techniques. As technology continues to evolve, the possibilities for creating comprehensive wellness solutions are limitless.
For further insights into the relationship between chakras and personal growth, don't forget to check out the article Chakren und Altersastrologie: Ein tiefer Einblick.
Happy coding, and may your app engage and uplift your users on their wellness journey!
Checkout our other articles