Mastering Async State Testing in Java: Tips and Tricks
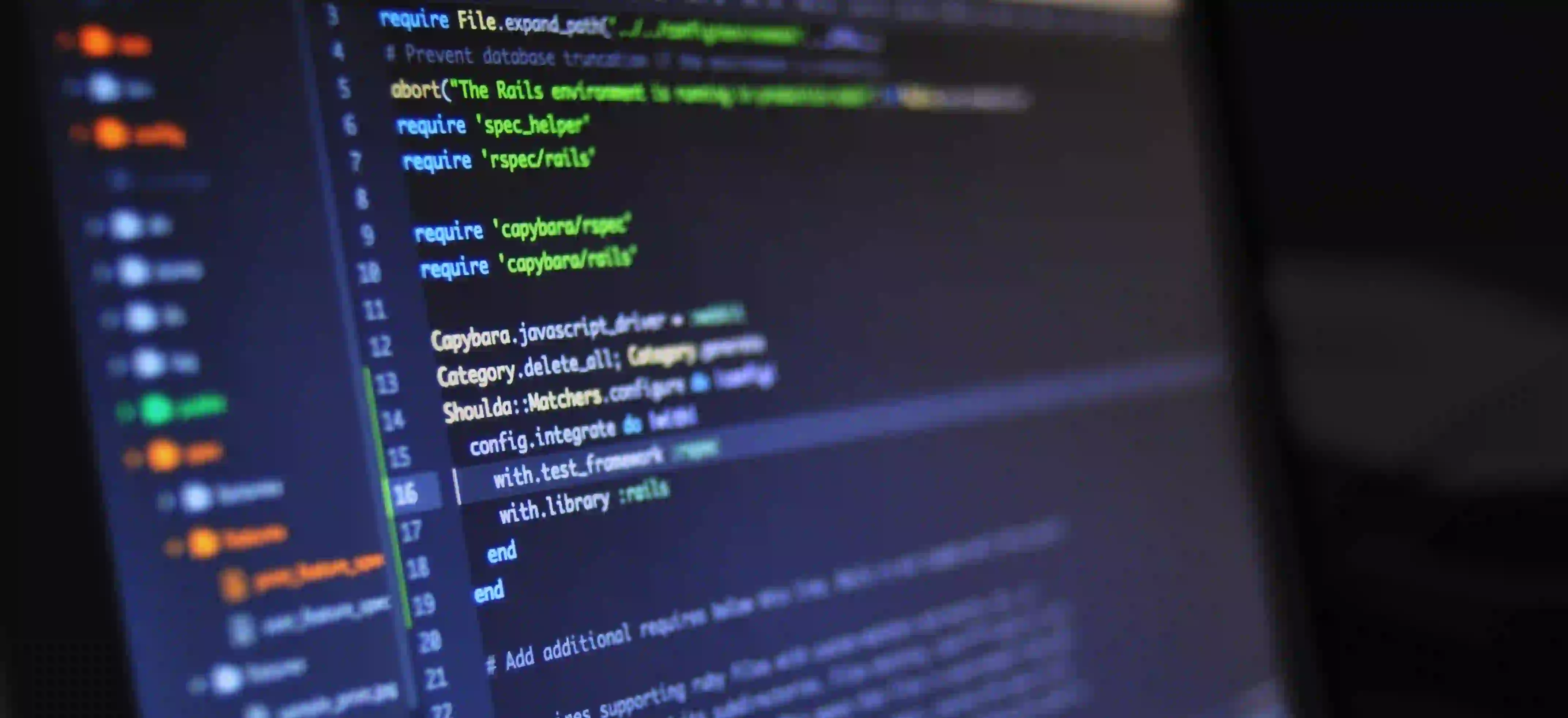
Mastering Async State Testing in Java: Tips and Tricks
As the modern landscape of software development increasingly embraces asynchronous programming, mastering async state testing in Java has become paramount. This blog post aims to equip you with vital strategies, best practices, and practical examples to efficiently test asynchronous state changes in Java applications. Whether you're a seasoned Java developer or just starting out, these insights will bolster your testing capabilities.
Understanding Async State Changes
Before diving deep into the testing techniques, it’s essential to grasp what async state changes entail. In asynchronous programming, methods can return results at a later time, which might reflect a change in the state of an application. This can happen through various mechanisms, like callbacks, promises, or reactive streams. Testing these changes can be tricky due to their non-blocking nature.
The Need for Testing Async State Changes
- Reliability: Asynchronous code can introduce complex interactions; thus, ensuring these interactions work reliably is crucial.
- Debugging: Bugs occurring due to timing issues or missed state updates can be elusive. Testing helps identify these issues early.
- Performance: Ensuring that asynchronous operations don’t degrade performance is vital.
Tools and Libraries for Testing Async State in Java
Java offers several tools and libraries that facilitate asynchronous testing. Here are a couple of popular options:
-
JUnit 5: The default testing framework for Java applications. It provides great utilities for writing clear and concise unit tests, including facilities for asynchronous operations.
-
CompletableFuture: A part of Java’s standard library,
CompletableFuture
is designed for asynchronous programming, allowing for easier composition of asynchronous tasks. -
Mockito: A popular mocking framework that can help isolate components during testing, making it easier to test async behavior.
Let’s explore these in greater detail and reference a few code snippets.
Testing Async Operations with JUnit 5
JUnit 5 allows you to write asynchronous tests using the CompletableFuture
class. The following is a simple example demonstrating how to test an asynchronous method:
Code Snippet: Basic Async Method
import java.util.concurrent.CompletableFuture;
public class AsyncService {
public CompletableFuture<String> fetchData() {
return CompletableFuture.supplyAsync(() -> {
try {
Thread.sleep(1000); // Simulates a delay
} catch (InterruptedException e) {
Thread.currentThread().interrupt();
}
return "Data fetched";
});
}
}
In the above snippet, the method fetchData
simulates an asynchronous operation that fetches data after a delay.
Test Case for Async Method
Now, let’s write a test case for the fetchData
method.
import static org.junit.jupiter.api.Assertions.assertEquals;
import org.junit.jupiter.api.Test;
import java.util.concurrent.CompletableFuture;
public class AsyncServiceTest {
@Test
public void testFetchData() throws Exception {
AsyncService asyncService = new AsyncService();
CompletableFuture<String> future = asyncService.fetchData();
String result = future.get(); // Wait for the future to complete
assertEquals("Data fetched", result);
}
}
Commentary on Transition from Method to Test
- CompletableFuture: It provides a
supplyAsync
method that executes the task in a separate thread. This is useful for simulating async operations. - future.get(): Blocks the current thread until the
CompletableFuture
is complete. This is essential for verifying the result in the unit test.
Testing Async Behavior with Mockito
Mockito can assist in testing asynchronous behavior by allowing you to mock dependencies and verify interactions. Here’s an example.
Code Snippet: Service that Uses a Repository
import java.util.concurrent.CompletableFuture;
public class UserService {
private final UserRepository userRepository;
public UserService(UserRepository userRepository) {
this.userRepository = userRepository;
}
public CompletableFuture<String> getUserData(String userId) {
return userRepository.fetchUser(userId);
}
}
Mocking Async Behavior in Tests
import static org.mockito.Mockito.*;
import static org.junit.jupiter.api.Assertions.*;
import org.junit.jupiter.api.Test;
import java.util.concurrent.CompletableFuture;
public class UserServiceTest {
@Test
public void testGetUserData() {
UserRepository mockRepo = mock(UserRepository.class);
UserService userService = new UserService(mockRepo);
when(mockRepo.fetchUser("123")).thenReturn(CompletableFuture.completedFuture("User Data"));
CompletableFuture<String> future = userService.getUserData("123");
assertEquals("User Data", future.join()); // Use join to get the result
}
}
Deciphering the Components
- Mockito: We create a mock of
UserRepository
to simulate its behavior. - when().thenReturn(): Defines the expected behavior for the mocked method.
- future.join(): A non-blocking way to retrieve the result from
CompletableFuture
.
By leveraging these tools and strategies, you can significantly enhance your async testing capabilities.
Full Stack Example: Async State Changes in Action
For a deeper understanding, let’s take a simple full stack application as an example involving async state changes. Suppose you are developing a web application that fetches user data asynchronously and displays it in the UI.
You might have a controller fetching data from a service:
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.RestController;
import java.util.concurrent.CompletableFuture;
@RestController
public class UserController {
private final UserService userService;
public UserController(UserService userService) {
this.userService = userService;
}
@GetMapping("/user")
public CompletableFuture<String> getUser() {
return userService.getUserData("123");
}
}
Testing Controller Async Endpoint
To test this controller, you can use the Spring Test framework along with JUnit:
import static org.springframework.test.web.servlet.request.MockMvcRequestBuilders.get;
import static org.springframework.test.web.servlet.result.MockMvcResultMatchers.status;
import static org.springframework.test.web.servlet.result.MockMvcResultMatchers.content;
import static org.mockito.Mockito.*;
import org.junit.jupiter.api.BeforeEach;
import org.junit.jupiter.api.Test;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.boot.test.autoconfigure.web.servlet.WebMvcTest;
import org.springframework.test.web.servlet.MockMvc;
import java.util.concurrent.CompletableFuture;
@WebMvcTest(UserController.class)
public class UserControllerTest {
@Autowired
private MockMvc mockMvc;
private UserService userService;
@BeforeEach
public void setUp() {
userService = mock(UserService.class);
}
@Test
public void testGetUser() throws Exception {
when(userService.getUserData("123")).thenReturn(CompletableFuture.completedFuture("User Data"));
mockMvc.perform(get("/user"))
.andExpect(status().isOk())
.andExpect(content().string("User Data"));
}
}
Explanation of the Test Case
- MockMvc: A Spring framework tool that allows you to simulate HTTP requests to the controller.
- CompletableFuture.completedFuture(): Since the repository is mocked, it’s directly supplying the result of the future without needing to wait.
Lessons Learned
Mastering async state testing in Java is not just about getting the right tests, but also about understanding the intricacies of how async operations work. Utilizing tools like JUnit, Mockito, and CompletableFuture can make this process smoother and more reliable.
In modern development, where frameworks like React or Svelte handle async state changes, developers can face challenges. If you want to dive further into testing async state changes, consider reading the article titled "Struggling with Testing Async State Changes in Svelte?" at tech-snags.com/articles/testing-async-state-svelte.
With the practices highlighted in this post, you should now feel more confident in tackling async testing in Java, ensuring your applications remain robust and reliable. Happy coding!